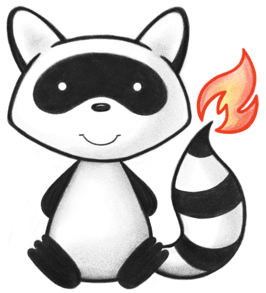
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Signature14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Decimal14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Instant14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.UnsignedInt14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Bundle.LinkRelationTypes; 012 013public class Bundle14_50 { 014 015 public static org.hl7.fhir.r5.model.Bundle convertBundle(org.hl7.fhir.dstu2016may.model.Bundle src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.r5.model.Bundle tgt = new org.hl7.fhir.r5.model.Bundle(); 019 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyResource(src, tgt); 020 if (src.hasType()) 021 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 022 if (src.hasTotal()) 023 tgt.setTotalElement(UnsignedInt14_50.convertUnsignedInt(src.getTotalElement())); 024 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 025 tgt.addLink(convertBundleLinkComponent(t)); 026 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent t : src.getEntry()) 027 tgt.addEntry(convertBundleEntryComponent(t)); 028 if (src.hasSignature()) 029 tgt.setSignature(Signature14_50.convertSignature(src.getSignature())); 030 return tgt; 031 } 032 033 public static org.hl7.fhir.dstu2016may.model.Bundle convertBundle(org.hl7.fhir.r5.model.Bundle src) throws FHIRException { 034 if (src == null || src.isEmpty()) 035 return null; 036 org.hl7.fhir.dstu2016may.model.Bundle tgt = new org.hl7.fhir.dstu2016may.model.Bundle(); 037 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyResource(src, tgt); 038 if (src.hasType()) 039 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 040 if (src.hasTotal()) 041 tgt.setTotalElement(UnsignedInt14_50.convertUnsignedInt(src.getTotalElement())); 042 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 043 for (org.hl7.fhir.r5.model.Bundle.BundleEntryComponent t : src.getEntry()) 044 tgt.addEntry(convertBundleEntryComponent(t)); 045 if (src.hasSignature()) 046 tgt.setSignature(Signature14_50.convertSignature(src.getSignature())); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryComponent src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent(); 054 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 055 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 056 if (src.hasFullUrl()) 057 tgt.setFullUrlElement(Uri14_50.convertUri(src.getFullUrlElement())); 058 if (src.hasResource()) 059 tgt.setResource(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertResource(src.getResource())); 060 if (src.hasSearch()) 061 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 062 if (src.hasRequest()) 063 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 064 if (src.hasResponse()) 065 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.r5.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.r5.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryComponent(); 073 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 074 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 075 tgt.addLink(convertBundleLinkComponent(t)); 076 if (src.hasFullUrl()) 077 tgt.setFullUrlElement(Uri14_50.convertUri(src.getFullUrlElement())); 078 if (src.hasResource()) 079 tgt.setResource(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertResource(src.getResource())); 080 if (src.hasSearch()) 081 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 082 if (src.hasRequest()) 083 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 084 if (src.hasResponse()) 085 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 086 return tgt; 087 } 088 089 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 090 if (src == null || src.isEmpty()) 091 return null; 092 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent(); 093 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 094 if (src.hasMethod()) 095 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 096 if (src.hasUrlElement()) 097 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 098 if (src.hasIfNoneMatch()) 099 tgt.setIfNoneMatchElement(String14_50.convertString(src.getIfNoneMatchElement())); 100 if (src.hasIfModifiedSince()) 101 tgt.setIfModifiedSinceElement(Instant14_50.convertInstant(src.getIfModifiedSinceElement())); 102 if (src.hasIfMatch()) 103 tgt.setIfMatchElement(String14_50.convertString(src.getIfMatchElement())); 104 if (src.hasIfNoneExist()) 105 tgt.setIfNoneExistElement(String14_50.convertString(src.getIfNoneExistElement())); 106 return tgt; 107 } 108 109 public static org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent(); 113 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 114 if (src.hasMethod()) 115 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 116 if (src.hasUrlElement()) 117 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 118 if (src.hasIfNoneMatch()) 119 tgt.setIfNoneMatchElement(String14_50.convertString(src.getIfNoneMatchElement())); 120 if (src.hasIfModifiedSince()) 121 tgt.setIfModifiedSinceElement(Instant14_50.convertInstant(src.getIfModifiedSinceElement())); 122 if (src.hasIfMatch()) 123 tgt.setIfMatchElement(String14_50.convertString(src.getIfMatchElement())); 124 if (src.hasIfNoneExist()) 125 tgt.setIfNoneExistElement(String14_50.convertString(src.getIfNoneExistElement())); 126 return tgt; 127 } 128 129 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 130 if (src == null || src.isEmpty()) 131 return null; 132 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent(); 133 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 134 if (src.hasStatusElement()) 135 tgt.setStatusElement(String14_50.convertString(src.getStatusElement())); 136 if (src.hasLocation()) 137 tgt.setLocationElement(Uri14_50.convertUri(src.getLocationElement())); 138 if (src.hasEtag()) 139 tgt.setEtagElement(String14_50.convertString(src.getEtagElement())); 140 if (src.hasLastModified()) 141 tgt.setLastModifiedElement(Instant14_50.convertInstant(src.getLastModifiedElement())); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent(); 149 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 150 if (src.hasStatusElement()) 151 tgt.setStatusElement(String14_50.convertString(src.getStatusElement())); 152 if (src.hasLocation()) 153 tgt.setLocationElement(Uri14_50.convertUri(src.getLocationElement())); 154 if (src.hasEtag()) 155 tgt.setEtagElement(String14_50.convertString(src.getEtagElement())); 156 if (src.hasLastModified()) 157 tgt.setLastModifiedElement(Instant14_50.convertInstant(src.getLastModifiedElement())); 158 return tgt; 159 } 160 161 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent(); 165 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 166 if (src.hasMode()) 167 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 168 if (src.hasScore()) 169 tgt.setScoreElement(Decimal14_50.convertDecimal(src.getScoreElement())); 170 return tgt; 171 } 172 173 public static org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 174 if (src == null || src.isEmpty()) 175 return null; 176 org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent(); 177 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 178 if (src.hasMode()) 179 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 180 if (src.hasScore()) 181 tgt.setScoreElement(Decimal14_50.convertDecimal(src.getScoreElement())); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r5.model.Bundle.BundleLinkComponent src) throws FHIRException { 186 if (src == null || src.isEmpty()) 187 return null; 188 org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent(); 189 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 190 if (src.hasRelationElement()) 191 tgt.setRelation((src.getRelation().toCode())); 192 if (src.hasUrlElement()) 193 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 194 return tgt; 195 } 196 197 public static org.hl7.fhir.r5.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r5.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleLinkComponent(); 201 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 202 if (src.hasRelationElement()) 203 tgt.setRelation(LinkRelationTypes.fromCode(src.getRelation())); 204 if (src.hasUrlElement()) 205 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 206 return tgt; 207 } 208 209 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> src) throws FHIRException { 210 if (src == null || src.isEmpty()) 211 return null; 212 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.BundleTypeEnumFactory()); 213 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 214 switch (src.getValue()) { 215 case DOCUMENT: 216 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.DOCUMENT); 217 break; 218 case MESSAGE: 219 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.MESSAGE); 220 break; 221 case TRANSACTION: 222 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTION); 223 break; 224 case TRANSACTIONRESPONSE: 225 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTIONRESPONSE); 226 break; 227 case BATCH: 228 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCH); 229 break; 230 case BATCHRESPONSE: 231 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCHRESPONSE); 232 break; 233 case HISTORY: 234 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.HISTORY); 235 break; 236 case SEARCHSET: 237 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.SEARCHSET); 238 break; 239 case COLLECTION: 240 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.COLLECTION); 241 break; 242 default: 243 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.NULL); 244 break; 245 } 246 return tgt; 247 } 248 249 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> src) throws FHIRException { 250 if (src == null || src.isEmpty()) 251 return null; 252 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.BundleTypeEnumFactory()); 253 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 254 switch (src.getValue()) { 255 case DOCUMENT: 256 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.DOCUMENT); 257 break; 258 case MESSAGE: 259 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.MESSAGE); 260 break; 261 case TRANSACTION: 262 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTION); 263 break; 264 case TRANSACTIONRESPONSE: 265 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTIONRESPONSE); 266 break; 267 case BATCH: 268 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCH); 269 break; 270 case BATCHRESPONSE: 271 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCHRESPONSE); 272 break; 273 case HISTORY: 274 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.HISTORY); 275 break; 276 case SEARCHSET: 277 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.SEARCHSET); 278 break; 279 case COLLECTION: 280 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.COLLECTION); 281 break; 282 default: 283 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.NULL); 284 break; 285 } 286 return tgt; 287 } 288 289 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> src) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerbEnumFactory()); 293 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 294 switch (src.getValue()) { 295 case GET: 296 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.GET); 297 break; 298 case POST: 299 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.POST); 300 break; 301 case PUT: 302 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.PUT); 303 break; 304 case DELETE: 305 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.DELETE); 306 break; 307 default: 308 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.NULL); 309 break; 310 } 311 return tgt; 312 } 313 314 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> src) throws FHIRException { 315 if (src == null || src.isEmpty()) 316 return null; 317 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.HTTPVerbEnumFactory()); 318 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 319 switch (src.getValue()) { 320 case GET: 321 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.GET); 322 break; 323 case POST: 324 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.POST); 325 break; 326 case PUT: 327 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.PUT); 328 break; 329 case DELETE: 330 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.DELETE); 331 break; 332 default: 333 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.NULL); 334 break; 335 } 336 return tgt; 337 } 338 339 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> src) throws FHIRException { 340 if (src == null || src.isEmpty()) 341 return null; 342 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryModeEnumFactory()); 343 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 344 switch (src.getValue()) { 345 case MATCH: 346 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.MATCH); 347 break; 348 case INCLUDE: 349 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.INCLUDE); 350 break; 351 case OUTCOME: 352 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.OUTCOME); 353 break; 354 default: 355 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.NULL); 356 break; 357 } 358 return tgt; 359 } 360 361 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> src) throws FHIRException { 362 if (src == null || src.isEmpty()) 363 return null; 364 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.SearchEntryModeEnumFactory()); 365 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 366 switch (src.getValue()) { 367 case MATCH: 368 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.MATCH); 369 break; 370 case INCLUDE: 371 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.INCLUDE); 372 break; 373 case OUTCOME: 374 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.OUTCOME); 375 break; 376 default: 377 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.NULL); 378 break; 379 } 380 return tgt; 381 } 382}