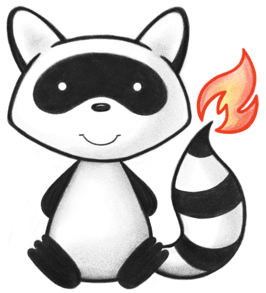
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.SourceElementComponentWrapper; 007import org.hl7.fhir.convertors.VersionConvertorConstants; 008import org.hl7.fhir.convertors.context.ConversionContext14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Reference14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Identifier14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 014import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 015import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 016import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 017import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 018import org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r5.model.CanonicalType; 021import org.hl7.fhir.r5.model.Coding; 022import org.hl7.fhir.r5.model.ConceptMap; 023import org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent; 024import org.hl7.fhir.r5.model.Enumeration; 025import org.hl7.fhir.r5.model.Enumerations; 026import org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship; 027import org.hl7.fhir.r5.utils.ToolingExtensions; 028 029public class ConceptMap14_50 { 030 031 public static org.hl7.fhir.r5.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu2016may.model.ConceptMap src) throws FHIRException { 032 if (src == null || src.isEmpty()) 033 return null; 034 org.hl7.fhir.r5.model.ConceptMap tgt = new org.hl7.fhir.r5.model.ConceptMap(); 035 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 036 if (src.hasUrl()) 037 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 038 if (src.hasIdentifier()) 039 tgt.addIdentifier(Identifier14_50.convertIdentifier(src.getIdentifier())); 040 if (src.hasVersion()) 041 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 042 if (src.hasName()) 043 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 044 if (src.hasStatus()) 045 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 046 if (src.hasExperimental()) 047 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 048 if (src.hasPublisher()) 049 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 050 for (org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent t : src.getContact()) 051 tgt.addContact(convertConceptMapContactComponent(t)); 052 if (src.hasDate()) 053 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 054 if (src.hasDescription()) 055 tgt.setDescription(src.getDescription()); 056 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 057 if (CodeableConcept14_50.isJurisdiction(t)) 058 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 059 else 060 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 061 if (src.hasRequirements()) 062 tgt.setPurpose(src.getRequirements()); 063 if (src.hasCopyright()) 064 tgt.setCopyright(src.getCopyright()); 065 org.hl7.fhir.r5.model.DataType tt = ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getSource()); 066 tgt.setSourceScope(tt instanceof org.hl7.fhir.r5.model.Reference ? new CanonicalType(((org.hl7.fhir.r5.model.Reference) tt).getReference()) : tt); 067 tt = ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getTarget()); 068 tgt.setTargetScope(tt instanceof org.hl7.fhir.r5.model.Reference ? new CanonicalType(((org.hl7.fhir.r5.model.Reference) tt).getReference()) : tt); 069 for (org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent t : src.getElement()) { 070 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> ws = convertSourceElementComponent(t, tgt); 071 for (SourceElementComponentWrapper<ConceptMap.SourceElementComponent> w : ws) 072 getGroup(tgt, w.getSource(), w.getTarget()).addElement(w.getComp()); 073 } 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu2016may.model.ConceptMap convertConceptMap(org.hl7.fhir.r5.model.ConceptMap src) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 org.hl7.fhir.dstu2016may.model.ConceptMap tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap(); 081 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 082 if (src.hasUrl()) 083 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 084 if (src.hasIdentifier()) { 085 if (src.hasIdentifier()) 086 tgt.setIdentifier(Identifier14_50.convertIdentifier(src.getIdentifierFirstRep())); 087 } 088 if (src.hasVersion()) 089 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 090 if (src.hasName()) 091 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 092 if (src.hasStatus()) 093 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 094 if (src.hasExperimental()) 095 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 096 if (src.hasPublisher()) 097 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 098 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) tgt.addContact(convertConceptMapContactComponent(t)); 099 if (src.hasDate()) 100 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 101 if (src.hasDescription()) 102 tgt.setDescription(src.getDescription()); 103 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 104 if (t.hasValueCodeableConcept()) 105 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 106 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 107 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 108 if (src.hasPurpose()) 109 tgt.setRequirements(src.getPurpose()); 110 if (src.hasCopyright()) 111 tgt.setCopyright(src.getCopyright()); 112 if (src.getSourceScope() instanceof CanonicalType) 113 tgt.setSource(Reference14_50.convertCanonicalToReference((CanonicalType) src.getSourceScope())); 114 else if (src.hasSourceScope()) 115 tgt.setSource(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getSourceScope())); 116 if (src.getTargetScope() instanceof CanonicalType) 117 tgt.setTarget(Reference14_50.convertCanonicalToReference((CanonicalType) src.getTargetScope())); 118 else if (src.hasTargetScope()) 119 tgt.setTarget(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getTargetScope())); 120 if (src.hasSourceScope()) 121 tgt.setSource(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getSourceScope())); 122 if (src.hasTargetScope()) 123 tgt.setTarget(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getTargetScope())); 124 for (org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g : src.getGroup()) 125 for (org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent t : g.getElement()) 126 tgt.addElement(convertSourceElementComponent(t, g, src)); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.r5.model.ContactDetail convertConceptMapContactComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 134 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 135 if (src.hasName()) 136 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 137 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 138 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent convertConceptMapContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent(); 146 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 147 if (src.hasName()) 148 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 149 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 150 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 151 return tgt; 152 } 153 154 public static org.hl7.fhir.dstu2016may.model.Enumeration<ConceptMapEquivalence> convertConceptMapEquivalence(Enumeration<ConceptMapRelationship> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent ccm) throws FHIRException { 155 if (src == null || src.isEmpty()) 156 return null; 157 org.hl7.fhir.dstu2016may.model.Enumeration<ConceptMapEquivalence> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<ConceptMapEquivalence>(new org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 158 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 159 if (ccm.hasExtension(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)) { 160 tgt.setValueAsString(ccm.getExtensionString(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)); 161 } else { 162 switch (src.getValue()) { 163 case EQUIVALENT: 164 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 165 break; 166 case SOURCEISNARROWERTHANTARGET: 167 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.WIDER); 168 break; 169 case SOURCEISBROADERTHANTARGET: 170 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.NARROWER); 171 break; 172 case NOTRELATEDTO: 173 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.DISJOINT); 174 break; 175 default: 176 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.NULL); 177 break; 178 } 179 } 180 return tgt; 181 } 182 183 public static Enumeration<ConceptMapRelationship> convertConceptMapRelationship(org.hl7.fhir.dstu2016may.model.Enumeration<ConceptMapEquivalence> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgtCtxt) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 Enumeration<ConceptMapRelationship> tgt = new Enumeration<ConceptMapRelationship>(new Enumerations.ConceptMapRelationshipEnumFactory()); 187 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 188 ToolingExtensions.setCodeExtensionMod(tgtCtxt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE, src.getValueAsString()); 189 switch (src.getValue()) { 190 case EQUIVALENT: 191 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.EQUIVALENT); 192 break; 193 case EQUAL: 194 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.EQUIVALENT); 195 break; 196 case WIDER: 197 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 198 break; 199 case SUBSUMES: 200 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 201 break; 202 case NARROWER: 203 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 204 break; 205 case SPECIALIZES: 206 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 207 break; 208 case INEXACT: 209 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.RELATEDTO); 210 break; 211 case UNMATCHED: 212 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.NULL); 213 break; 214 case DISJOINT: 215 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.NOTRELATEDTO); 216 break; 217 default: 218 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.NULL); 219 break; 220 } 221 return tgt; 222 } 223 224 public static org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent(); 228 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 229 if (src.hasAttribute()) 230 tgt.setElement(srcMap.getAttributeUri(src.getAttribute())); 231 if (src.hasValueCoding()) { 232 tgt.setSystem(src.getValueCoding().getSystem()); 233 tgt.setCode(src.getValueCoding().getCode()); 234 } else if (src.hasValue()) { 235 tgt.setCode(src.getValue().primitiveValue()); 236 } 237 return tgt; 238 } 239 240 public static org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 241 if (src == null || src.isEmpty()) 242 return null; 243 org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent(); 244 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 245 if (src.hasElementElement()) 246 tgt.setAttribute(tgtMap.registerAttribute(src.getElement())); 247 if (src.hasSystem()) { 248 tgt.setValue(new Coding().setSystem(src.getSystem()).setCode(src.getCode())); 249 } else if (src.hasCodeElement()) { 250 tgt.setValue(String14_50.convertString(src.getCodeElement())); 251 } 252 return tgt; 253 } 254 255 public static List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> convertSourceElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 256 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> res = new ArrayList<>(); 257 if (src == null || src.isEmpty()) 258 return res; 259 for (org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent t : src.getTarget()) { 260 org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent(); 261 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 262 if (src.hasCode()) 263 tgt.setCode(src.getCode()); 264 if (t.getEquivalence() == org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.UNMATCHED) { 265 tgt.setNoMap(true); 266 } else { 267 tgt.addTarget(convertTargetElementComponent(t, tgtMap)); 268 } 269 res.add(new SourceElementComponentWrapper<>(tgt, src.getSystem(), t.getSystem())); 270 } 271 return res; 272 } 273 274 public static org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 275 if (src == null || src.isEmpty()) 276 return null; 277 org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent(); 278 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 279 if (g.hasSource()) 280 tgt.setSystem(g.getSource()); 281 if (src.hasCode()) 282 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 283 if (src.hasNoMap() && src.getNoMap() == true) { 284 tgt.addTarget(new org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent().setEquivalence(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.UNMATCHED)); 285 } else { 286 for (org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent t : src.getTarget()) 287 tgt.addTarget(convertTargetElementComponent(t, g, srcMap)); 288 } 289 return tgt; 290 } 291 292 public static org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 293 if (src == null || src.isEmpty()) 294 return null; 295 org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent(); 296 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE); 297 if (g.hasTarget()) 298 tgt.setSystem(g.getTarget()); 299 if (src.hasCode()) 300 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 301 if (src.hasRelationship()) 302 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getRelationshipElement(), src)); 303 if (src.hasComment()) 304 tgt.setCommentsElement(String14_50.convertString(src.getCommentElement())); 305 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 306 tgt.addDependsOn(convertOtherElementComponent(t, srcMap)); 307 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getProduct()) 308 tgt.addProduct(convertOtherElementComponent(t, srcMap)); 309 return tgt; 310 } 311 312 public static org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 313 if (src == null || src.isEmpty()) 314 return null; 315 org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent(); 316 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 317 if (src.hasCode()) 318 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 319 if (src.hasEquivalence()) 320 tgt.setRelationshipElement(convertConceptMapRelationship(src.getEquivalenceElement(), tgt)); 321 if (src.hasComments()) 322 tgt.setCommentElement(String14_50.convertString(src.getCommentsElement())); 323 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 324 tgt.addDependsOn(convertOtherElementComponent(t, tgtMap)); 325 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getProduct()) 326 tgt.addProduct(convertOtherElementComponent(t, tgtMap)); 327 return tgt; 328 } 329 330 static public ConceptMapGroupComponent getGroup(ConceptMap map, String srcs, String tgts) { 331 for (ConceptMapGroupComponent grp : map.getGroup()) { 332 if (grp.hasSource() && grp.getSource().equals(srcs) && grp.getTarget().equals(tgts)) 333 return grp; 334 } 335 ConceptMapGroupComponent grp = map.addGroup(); 336 grp.setSource(srcs); 337 grp.setTarget(tgts); 338 return grp; 339 } 340}