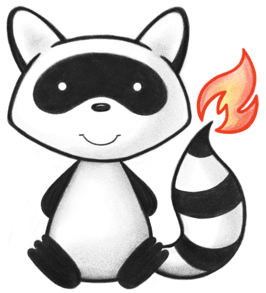
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Reference14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Coding14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.UnsignedInt14_50; 014import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent; 017import org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent; 018 019public class Conformance14_50 { 020 021 public static final String ACCEPT_UNKNOWN_EXTENSION_URL = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CapabilityStatement.acceptUnknown"; 022 023 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 024 ACCEPT_UNKNOWN_EXTENSION_URL 025 }; 026 027 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 028 if (src == null || src.isEmpty()) 029 return null; 030 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatusEnumFactory()); 031 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 032 switch (src.getValue()) { 033 case NOTSUPPORTED: 034 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.NOTSUPPORTED); 035 break; 036 case SINGLE: 037 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.SINGLE); 038 break; 039 case MULTIPLE: 040 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.MULTIPLE); 041 break; 042 default: 043 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.NULL); 044 break; 045 } 046 return tgt; 047 } 048 049 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 053 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 054 switch (src.getValue()) { 055 case NOTSUPPORTED: 056 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 057 break; 058 case SINGLE: 059 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.SINGLE); 060 break; 061 case MULTIPLE: 062 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 063 break; 064 default: 065 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.NULL); 066 break; 067 } 068 return tgt; 069 } 070 071 public static org.hl7.fhir.r5.model.CapabilityStatement convertConformance(org.hl7.fhir.dstu2016may.model.Conformance src) throws FHIRException { 072 if (src == null || src.isEmpty()) 073 return null; 074 org.hl7.fhir.r5.model.CapabilityStatement tgt = new org.hl7.fhir.r5.model.CapabilityStatement(); 075 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 076 if (src.hasUrl()) 077 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 078 if (src.hasVersion()) 079 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 080 if (src.hasName()) 081 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 082 if (src.hasStatus()) 083 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 084 if (src.hasExperimental()) 085 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 086 if (src.hasDate()) 087 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 088 if (src.hasPublisher()) 089 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 090 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent t : src.getContact()) 091 tgt.addContact(convertConformanceContactComponent(t)); 092 if (src.hasDescription()) 093 tgt.setDescription(src.getDescription()); 094 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 095 if (CodeableConcept14_50.isJurisdiction(t)) 096 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 097 else 098 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 099 if (src.hasRequirements()) 100 tgt.setPurpose(src.getRequirements()); 101 if (src.hasCopyright()) 102 tgt.setCopyright(src.getCopyright()); 103 if (src.hasKind()) 104 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 105 if (src.hasSoftware()) 106 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 107 if (src.hasImplementation()) 108 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 109 if (src.hasFhirVersion()) 110 tgt.setFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 111 if (src.hasAcceptUnknown()) 112 tgt.addExtension().setUrl(ACCEPT_UNKNOWN_EXTENSION_URL).setValue(new org.hl7.fhir.r5.model.CodeType(src.getAcceptUnknownElement().asStringValue())); 113 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 114 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent t : src.getRest()) 115 tgt.addRest(convertConformanceRestComponent(t)); 116 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent t : src.getMessaging()) 117 tgt.addMessaging(convertConformanceMessagingComponent(t)); 118 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent t : src.getDocument()) 119 tgt.addDocument(convertConformanceDocumentComponent(t)); 120 return tgt; 121 } 122 123 public static org.hl7.fhir.dstu2016may.model.Conformance convertConformance(org.hl7.fhir.r5.model.CapabilityStatement src) throws FHIRException { 124 if (src == null || src.isEmpty()) 125 return null; 126 org.hl7.fhir.dstu2016may.model.Conformance tgt = new org.hl7.fhir.dstu2016may.model.Conformance(); 127 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 128 if (src.hasUrl()) 129 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 130 if (src.hasVersion()) 131 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 132 if (src.hasName()) 133 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 134 if (src.hasStatus()) 135 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 136 if (src.hasExperimental()) 137 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 138 if (src.hasDate()) 139 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 140 if (src.hasPublisher()) 141 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 142 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 143 tgt.addContact(convertConformanceContactComponent(t)); 144 if (src.hasDescription()) 145 tgt.setDescription(src.getDescription()); 146 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 147 if (t.hasValueCodeableConcept()) 148 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 149 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 150 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 151 if (src.hasPurpose()) 152 tgt.setRequirements(src.getPurpose()); 153 if (src.hasCopyright()) 154 tgt.setCopyright(src.getCopyright()); 155 if (src.hasKind()) 156 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 157 if (src.hasSoftware()) 158 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 159 if (src.hasImplementation()) 160 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 161 tgt.setFhirVersion(src.getFhirVersion().toCode()); 162 if (src.hasExtension(ACCEPT_UNKNOWN_EXTENSION_URL)) 163 tgt.setAcceptUnknown(org.hl7.fhir.dstu2016may.model.Conformance.UnknownContentCode.fromCode(src.getExtensionByUrl(ACCEPT_UNKNOWN_EXTENSION_URL).getValue().primitiveValue())); 164 for (org.hl7.fhir.r5.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 165 for (CapabilityStatementRestComponent r : src.getRest()) 166 for (CapabilityStatementRestResourceComponent rr : r.getResource()) 167 for (org.hl7.fhir.r5.model.CanonicalType t : rr.getSupportedProfile()) 168 tgt.addProfile(Reference14_50.convertCanonicalToReference(t)); 169 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 170 tgt.addRest(convertConformanceRestComponent(t)); 171 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 172 tgt.addMessaging(convertConformanceMessagingComponent(t)); 173 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 174 tgt.addDocument(convertConformanceDocumentComponent(t)); 175 return tgt; 176 } 177 178 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent convertConformanceContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 179 if (src == null || src.isEmpty()) 180 return null; 181 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent(); 182 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 183 if (src.hasName()) 184 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 185 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 186 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 187 return tgt; 188 } 189 190 public static org.hl7.fhir.r5.model.ContactDetail convertConformanceContactComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent src) throws FHIRException { 191 if (src == null || src.isEmpty()) 192 return null; 193 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 194 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 195 if (src.hasName()) 196 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 197 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 198 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 199 return tgt; 200 } 201 202 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent src) throws FHIRException { 203 if (src == null || src.isEmpty()) 204 return null; 205 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 206 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 207 if (src.hasMode()) 208 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 209 if (src.hasDocumentation()) 210 tgt.setDocumentation(src.getDocumentation()); 211 if (src.hasProfile()) 212 tgt.setProfileElement(Reference14_50.convertReferenceToCanonical(src.getProfile())); 213 return tgt; 214 } 215 216 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent(); 220 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 221 if (src.hasMode()) 222 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 223 if (src.hasDocumentation()) 224 tgt.setDocumentation(src.getDocumentation()); 225 if (src.hasProfileElement()) 226 tgt.setProfile(Reference14_50.convertCanonicalToReference(src.getProfileElement())); 227 return tgt; 228 } 229 230 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent src) throws FHIRException { 231 if (src == null || src.isEmpty()) 232 return null; 233 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 234 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 235 if (src.hasDescriptionElement()) 236 tgt.setDescriptionElement(String14_50.convertStringToMarkdown(src.getDescriptionElement())); 237 if (src.hasUrl()) 238 tgt.setUrl(src.getUrl()); 239 return tgt; 240 } 241 242 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 243 if (src == null || src.isEmpty()) 244 return null; 245 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent(); 246 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 247 if (src.hasDescriptionElement()) 248 tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 249 if (src.hasUrl()) 250 tgt.setUrl(src.getUrl()); 251 return tgt; 252 } 253 254 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 255 if (src == null || src.isEmpty()) 256 return null; 257 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent(); 258 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 259 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 260 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 261 if (src.hasReliableCache()) 262 tgt.setReliableCacheElement(UnsignedInt14_50.convertUnsignedInt(src.getReliableCacheElement())); 263 if (src.hasDocumentation()) 264 tgt.setDocumentation(src.getDocumentation()); 265 for (org.hl7.fhir.r5.model.Extension e : src.getExtensionsByUrl(VersionConvertorConstants.IG_CONFORMANCE_MESSAGE_EVENT)) { 266 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent event = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent(); 267 tgt.addEvent(event); 268 event.setCode(Coding14_50.convertCoding((org.hl7.fhir.r5.model.Coding) e.getExtensionByUrl("code").getValue())); 269 if (e.hasExtension("category")) 270 event.setCategory(org.hl7.fhir.dstu2016may.model.Conformance.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 271 event.setMode(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 272 org.hl7.fhir.r5.model.Extension focusE = e.getExtensionByUrl("focus"); 273 if (focusE.getValue().hasPrimitiveValue()) 274 event.setFocus(focusE.getValue().toString()); 275 else { 276 event.setFocusElement(new org.hl7.fhir.dstu2016may.model.CodeType()); 277 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(focusE.getValue(), event.getFocusElement()); 278 } 279 event.setRequest(Reference14_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("request").getValue())); 280 event.setResponse(Reference14_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("response").getValue())); 281 if (e.hasExtension("documentation")) 282 event.setDocumentation(e.getExtensionByUrl("documentation").getValue().toString()); 283 } 284 return tgt; 285 } 286 287 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent src) throws FHIRException { 288 if (src == null || src.isEmpty()) 289 return null; 290 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 291 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 292 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent t : src.getEndpoint()) 293 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 294 if (src.hasReliableCache()) 295 tgt.setReliableCacheElement(UnsignedInt14_50.convertUnsignedInt(src.getReliableCacheElement())); 296 if (src.hasDocumentation()) 297 tgt.setDocumentation(src.getDocumentation()); 298 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent t : src.getEvent()) { 299 org.hl7.fhir.r5.model.Extension e = new org.hl7.fhir.r5.model.Extension(VersionConvertorConstants.IG_CONFORMANCE_MESSAGE_EVENT); 300 e.addExtension(new org.hl7.fhir.r5.model.Extension("code", Coding14_50.convertCoding(t.getCode()))); 301 if (t.hasCategory()) 302 e.addExtension(new org.hl7.fhir.r5.model.Extension("category", new org.hl7.fhir.r5.model.CodeType(t.getCategory().toCode()))); 303 e.addExtension(new org.hl7.fhir.r5.model.Extension("mode", new org.hl7.fhir.r5.model.CodeType(t.getMode().toCode()))); 304 if (t.getFocusElement().hasValue()) 305 e.addExtension(new org.hl7.fhir.r5.model.Extension("focus", new org.hl7.fhir.r5.model.StringType(t.getFocus()))); 306 else { 307 org.hl7.fhir.r5.model.CodeType focus = new org.hl7.fhir.r5.model.CodeType(); 308 org.hl7.fhir.r5.model.Extension focusE = new org.hl7.fhir.r5.model.Extension("focus", focus); 309 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(t.getFocusElement(), focus); 310 e.addExtension(focusE); 311 } 312 e.addExtension(new org.hl7.fhir.r5.model.Extension("request", Reference14_50.convertReference(t.getRequest()))); 313 e.addExtension(new org.hl7.fhir.r5.model.Extension("response", Reference14_50.convertReference(t.getResponse()))); 314 if (t.hasDocumentation()) 315 e.addExtension(new org.hl7.fhir.r5.model.Extension("documentation", new org.hl7.fhir.r5.model.StringType(t.getDocumentation()))); 316 tgt.addExtension(e); 317 } 318 return tgt; 319 } 320 321 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 322 if (src == null || src.isEmpty()) 323 return null; 324 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent(); 325 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 326 if (src.hasProtocol()) 327 tgt.setProtocol(Coding14_50.convertCoding(src.getProtocol())); 328 if (src.hasAddress()) 329 tgt.setAddress(src.getAddress()); 330 return tgt; 331 } 332 333 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent src) throws FHIRException { 334 if (src == null || src.isEmpty()) 335 return null; 336 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 337 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 338 if (src.hasProtocol()) 339 tgt.setProtocol(Coding14_50.convertCoding(src.getProtocol())); 340 if (src.hasAddress()) 341 tgt.setAddress(src.getAddress()); 342 return tgt; 343 } 344 345 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent convertConformanceRestComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent src) throws FHIRException { 346 if (src == null || src.isEmpty()) 347 return null; 348 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent(); 349 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 350 if (src.hasMode()) 351 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 352 if (src.hasDocumentation()) 353 tgt.setDocumentation(src.getDocumentation()); 354 if (src.hasSecurity()) 355 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 356 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent t : src.getResource()) 357 tgt.addResource(convertConformanceRestResourceComponent(t)); 358 for (org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent t : src.getInteraction()) 359 tgt.addInteraction(convertSystemInteractionComponent(t)); 360 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 361 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 362 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent t : src.getOperation()) 363 tgt.addOperation(convertConformanceRestOperationComponent(t)); 364 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 365 return tgt; 366 } 367 368 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent convertConformanceRestComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 369 if (src == null || src.isEmpty()) 370 return null; 371 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent(); 372 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 373 if (src.hasMode()) 374 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 375 if (src.hasDocumentation()) 376 tgt.setDocumentation(src.getDocumentation()); 377 if (src.hasSecurity()) 378 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 379 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 380 tgt.addResource(convertConformanceRestResourceComponent(t)); 381 for (org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) 382 tgt.addInteraction(convertSystemInteractionComponent(t)); 383 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 384 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 385 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent t : src.getOperation()) 386 tgt.addOperation(convertConformanceRestOperationComponent(t)); 387 for (org.hl7.fhir.r5.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 388 return tgt; 389 } 390 391 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent src) throws FHIRException { 392 if (src == null || src.isEmpty()) 393 return null; 394 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent(); 395 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 396 if (src.hasNameElement()) 397 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 398 if (src.hasDefinitionElement()) 399 tgt.setDefinition(Reference14_50.convertCanonicalToReference(src.getDefinitionElement())); 400 return tgt; 401 } 402 403 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent src) throws FHIRException { 404 if (src == null || src.isEmpty()) 405 return null; 406 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent(); 407 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 408 if (src.hasNameElement()) 409 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 410 if (src.hasDefinition()) 411 tgt.setDefinitionElement(Reference14_50.convertReferenceToCanonical(src.getDefinition())); 412 return tgt; 413 } 414 415 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 416 if (src == null || src.isEmpty()) 417 return null; 418 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent(); 419 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 420 if (src.hasType()) { 421 if (src.hasTypeElement()) 422 tgt.setTypeElement(Code14_50.convertCode(src.getTypeElement())); 423 } 424 if (src.hasProfileElement()) 425 tgt.setProfile(Reference14_50.convertCanonicalToReference(src.getProfileElement())); 426 for (org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 427 tgt.addInteraction(convertResourceInteractionComponent(t)); 428 if (src.hasVersioning()) 429 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 430 if (src.hasReadHistory()) 431 tgt.setReadHistoryElement(Boolean14_50.convertBoolean(src.getReadHistoryElement())); 432 if (src.hasUpdateCreate()) 433 tgt.setUpdateCreateElement(Boolean14_50.convertBoolean(src.getUpdateCreateElement())); 434 if (src.hasConditionalCreate()) 435 tgt.setConditionalCreateElement(Boolean14_50.convertBoolean(src.getConditionalCreateElement())); 436 if (src.hasConditionalUpdate()) 437 tgt.setConditionalUpdateElement(Boolean14_50.convertBoolean(src.getConditionalUpdateElement())); 438 if (src.hasConditionalDelete()) 439 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 440 for (org.hl7.fhir.r5.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 441 for (org.hl7.fhir.r5.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 442 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 443 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 444 return tgt; 445 } 446 447 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent src) throws FHIRException { 448 if (src == null || src.isEmpty()) 449 return null; 450 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 451 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 452 if (src.hasTypeElement()) 453 tgt.setTypeElement(Code14_50.convertCode(src.getTypeElement())); 454 if (src.hasProfile()) 455 tgt.setProfileElement(Reference14_50.convertReferenceToCanonical(src.getProfile())); 456 for (org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent t : src.getInteraction()) 457 tgt.addInteraction(convertResourceInteractionComponent(t)); 458 if (src.hasVersioning()) 459 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 460 if (src.hasReadHistory()) 461 tgt.setReadHistoryElement(Boolean14_50.convertBoolean(src.getReadHistoryElement())); 462 if (src.hasUpdateCreate()) 463 tgt.setUpdateCreateElement(Boolean14_50.convertBoolean(src.getUpdateCreateElement())); 464 if (src.hasConditionalCreate()) 465 tgt.setConditionalCreateElement(Boolean14_50.convertBoolean(src.getConditionalCreateElement())); 466 if (src.hasConditionalUpdate()) 467 tgt.setConditionalUpdateElement(Boolean14_50.convertBoolean(src.getConditionalUpdateElement())); 468 if (src.hasConditionalDelete()) 469 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 470 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 471 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 472 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 473 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 474 return tgt; 475 } 476 477 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 478 if (src == null || src.isEmpty()) 479 return null; 480 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent(); 481 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 482 if (src.hasNameElement()) 483 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 484 if (src.hasDefinition()) 485 tgt.setDefinition(src.getDefinition()); 486 if (src.hasType()) 487 tgt.setTypeElement(Enumerations14_50.convertSearchParamType(src.getTypeElement())); 488 if (src.hasDocumentation()) 489 tgt.setDocumentation(src.getDocumentation()); 490 return tgt; 491 } 492 493 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent src) throws FHIRException { 494 if (src == null || src.isEmpty()) 495 return null; 496 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 497 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 498 if (src.hasNameElement()) 499 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 500 if (src.hasDefinition()) 501 tgt.setDefinition(src.getDefinition()); 502 if (src.hasType()) 503 tgt.setTypeElement(Enumerations14_50.convertSearchParamType(src.getTypeElement())); 504 if (src.hasDocumentation()) 505 tgt.setDocumentation(src.getDocumentation()); 506 return tgt; 507 } 508 509 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent src) throws FHIRException { 510 if (src == null || src.isEmpty()) 511 return null; 512 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 513 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 514 if (src.hasCors()) 515 tgt.setCorsElement(Boolean14_50.convertBoolean(src.getCorsElement())); 516 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getService()) 517 tgt.addService(CodeableConcept14_50.convertCodeableConcept(t)); 518 if (src.hasDescription()) 519 tgt.setDescription(src.getDescription()); 520 return tgt; 521 } 522 523 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 524 if (src == null || src.isEmpty()) 525 return null; 526 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent(); 527 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 528 if (src.hasCors()) 529 tgt.setCorsElement(Boolean14_50.convertBoolean(src.getCorsElement())); 530 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getService()) 531 tgt.addService(CodeableConcept14_50.convertCodeableConcept(t)); 532 if (src.hasDescription()) 533 tgt.setDescription(src.getDescription()); 534 return tgt; 535 } 536 537 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent src) throws FHIRException { 538 if (src == null || src.isEmpty()) 539 return null; 540 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 541 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 542 if (src.hasNameElement()) 543 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 544 if (src.hasVersion()) 545 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 546 if (src.hasReleaseDate()) 547 tgt.setReleaseDateElement(DateTime14_50.convertDateTime(src.getReleaseDateElement())); 548 return tgt; 549 } 550 551 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 552 if (src == null || src.isEmpty()) 553 return null; 554 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent(); 555 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 556 if (src.hasNameElement()) 557 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 558 if (src.hasVersion()) 559 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 560 if (src.hasReleaseDate()) 561 tgt.setReleaseDateElement(DateTime14_50.convertDateTime(src.getReleaseDateElement())); 562 return tgt; 563 } 564 565 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> convertConformanceStatementKind(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> src) throws FHIRException { 566 if (src == null || src.isEmpty()) 567 return null; 568 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKindEnumFactory()); 569 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 570 switch (src.getValue()) { 571 case INSTANCE: 572 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind.INSTANCE); 573 break; 574 case CAPABILITY: 575 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind.CAPABILITY); 576 break; 577 case REQUIREMENTS: 578 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind.REQUIREMENTS); 579 break; 580 default: 581 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind.NULL); 582 break; 583 } 584 return tgt; 585 } 586 587 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> convertConformanceStatementKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> src) throws FHIRException { 588 if (src == null || src.isEmpty()) 589 return null; 590 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKindEnumFactory()); 591 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 592 switch (src.getValue()) { 593 case INSTANCE: 594 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.INSTANCE); 595 break; 596 case CAPABILITY: 597 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.CAPABILITY); 598 break; 599 case REQUIREMENTS: 600 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.REQUIREMENTS); 601 break; 602 default: 603 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.NULL); 604 break; 605 } 606 return tgt; 607 } 608 609 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> src) throws FHIRException { 610 if (src == null || src.isEmpty()) 611 return null; 612 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.DocumentModeEnumFactory()); 613 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 614 switch (src.getValue()) { 615 case PRODUCER: 616 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.PRODUCER); 617 break; 618 case CONSUMER: 619 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.CONSUMER); 620 break; 621 default: 622 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.NULL); 623 break; 624 } 625 return tgt; 626 } 627 628 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> convertDocumentMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 629 if (src == null || src.isEmpty()) 630 return null; 631 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.DocumentModeEnumFactory()); 632 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 633 switch (src.getValue()) { 634 case PRODUCER: 635 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.PRODUCER); 636 break; 637 case CONSUMER: 638 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.CONSUMER); 639 break; 640 default: 641 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.NULL); 642 break; 643 } 644 return tgt; 645 } 646 647 public static org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent src) throws FHIRException { 648 if (src == null || src.isEmpty()) 649 return null; 650 org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent(); 651 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 652 if (src.hasCode()) 653 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 654 if (src.hasDocumentation()) 655 tgt.setDocumentation(src.getDocumentation()); 656 return tgt; 657 } 658 659 public static org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 660 if (src == null || src.isEmpty()) 661 return null; 662 org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent(); 663 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 664 if (src.hasCode()) 665 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 666 if (src.hasDocumentation()) 667 tgt.setDocumentation(src.getDocumentation()); 668 return tgt; 669 } 670 671 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> src) throws FHIRException { 672 if (src == null || src.isEmpty()) 673 return null; 674 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicyEnumFactory()); 675 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 676 switch (src.getValue()) { 677 case NOVERSION: 678 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.NOVERSION); 679 break; 680 case VERSIONED: 681 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.VERSIONED); 682 break; 683 case VERSIONEDUPDATE: 684 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 685 break; 686 default: 687 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.NULL); 688 break; 689 } 690 return tgt; 691 } 692 693 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 694 if (src == null || src.isEmpty()) 695 return null; 696 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicyEnumFactory()); 697 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 698 switch (src.getValue()) { 699 case NOVERSION: 700 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.NOVERSION); 701 break; 702 case VERSIONED: 703 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.VERSIONED); 704 break; 705 case VERSIONEDUPDATE: 706 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.VERSIONEDUPDATE); 707 break; 708 default: 709 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.NULL); 710 break; 711 } 712 return tgt; 713 } 714 715 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulConformanceMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> src) throws FHIRException { 716 if (src == null || src.isEmpty()) 717 return null; 718 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityModeEnumFactory()); 719 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 720 switch (src.getValue()) { 721 case CLIENT: 722 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.CLIENT); 723 break; 724 case SERVER: 725 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.SERVER); 726 break; 727 default: 728 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.NULL); 729 break; 730 } 731 return tgt; 732 } 733 734 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> convertRestfulConformanceMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 735 if (src == null || src.isEmpty()) 736 return null; 737 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceModeEnumFactory()); 738 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 739 switch (src.getValue()) { 740 case CLIENT: 741 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.CLIENT); 742 break; 743 case SERVER: 744 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.SERVER); 745 break; 746 default: 747 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.NULL); 748 break; 749 } 750 return tgt; 751 } 752 753 public static org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 754 if (src == null || src.isEmpty()) 755 return null; 756 org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent(); 757 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 758 if (src.hasCode()) 759 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 760 if (src.hasDocumentation()) 761 tgt.setDocumentation(src.getDocumentation()); 762 return tgt; 763 } 764 765 public static org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent src) throws FHIRException { 766 if (src == null || src.isEmpty()) 767 return null; 768 org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent(); 769 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 770 if (src.hasCode()) 771 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 772 if (src.hasDocumentation()) 773 tgt.setDocumentation(src.getDocumentation()); 774 return tgt; 775 } 776 777 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> src) throws FHIRException { 778 if (src == null || src.isEmpty()) 779 return null; 780 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteractionEnumFactory()); 781 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 782 switch (src.getValue()) { 783 case TRANSACTION: 784 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.TRANSACTION); 785 break; 786 case SEARCHSYSTEM: 787 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.SEARCHSYSTEM); 788 break; 789 case HISTORYSYSTEM: 790 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.HISTORYSYSTEM); 791 break; 792 default: 793 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.NULL); 794 break; 795 } 796 return tgt; 797 } 798 799 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 800 if (src == null || src.isEmpty()) 801 return null; 802 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteractionEnumFactory()); 803 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 804 switch (src.getValue()) { 805 case TRANSACTION: 806 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.TRANSACTION); 807 break; 808 case SEARCHSYSTEM: 809 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.SEARCHSYSTEM); 810 break; 811 case HISTORYSYSTEM: 812 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.HISTORYSYSTEM); 813 break; 814 default: 815 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.NULL); 816 break; 817 } 818 return tgt; 819 } 820 821 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 822 if (src == null || src.isEmpty()) 823 return null; 824 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteractionEnumFactory()); 825 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 826 switch (src.getValue()) { 827 case READ: 828 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.READ); 829 break; 830 case VREAD: 831 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.VREAD); 832 break; 833 case UPDATE: 834 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.UPDATE); 835 break; 836 case DELETE: 837 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.DELETE); 838 break; 839 case HISTORYINSTANCE: 840 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.HISTORYINSTANCE); 841 break; 842 case HISTORYTYPE: 843 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.HISTORYTYPE); 844 break; 845 case CREATE: 846 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.CREATE); 847 break; 848 case SEARCHTYPE: 849 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.SEARCHTYPE); 850 break; 851 default: 852 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.NULL); 853 break; 854 } 855 return tgt; 856 } 857 858 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> src) throws FHIRException { 859 if (src == null || src.isEmpty()) 860 return null; 861 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteractionEnumFactory()); 862 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 863 switch (src.getValue()) { 864 case READ: 865 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.READ); 866 break; 867 case VREAD: 868 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.VREAD); 869 break; 870 case UPDATE: 871 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.UPDATE); 872 break; 873 case DELETE: 874 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.DELETE); 875 break; 876 case HISTORYINSTANCE: 877 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 878 break; 879 case HISTORYTYPE: 880 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 881 break; 882 case CREATE: 883 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.CREATE); 884 break; 885 case SEARCHTYPE: 886 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 887 break; 888 default: 889 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.NULL); 890 break; 891 } 892 return tgt; 893 } 894 895 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> convertConformanceEventMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> src) throws FHIRException { 896 if (src == null || src.isEmpty()) return null; 897 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityModeEnumFactory()); 898 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 899 if (src.getValue() == null) { 900 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.NULL); 901 } else { 902 switch (src.getValue()) { 903 case SENDER: 904 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.SENDER); 905 break; 906 case RECEIVER: 907 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.RECEIVER); 908 break; 909 default: 910 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.NULL); 911 break; 912 } 913 } 914 return tgt; 915 } 916 917 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> convertConformanceEventMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 918 if (src == null || src.isEmpty()) return null; 919 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventModeEnumFactory()); 920 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 921 if (src.getValue() == null) { 922 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.NULL); 923 } else { 924 switch (src.getValue()) { 925 case SENDER: 926 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.SENDER); 927 break; 928 case RECEIVER: 929 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.RECEIVER); 930 break; 931 default: 932 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.NULL); 933 break; 934 } 935 } 936 return tgt; 937 } 938}