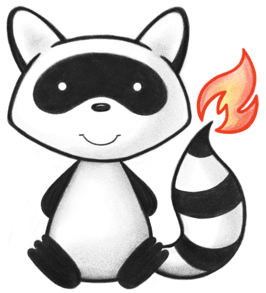
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Reference14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 014import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 015import org.hl7.fhir.dstu2016may.model.ImplementationGuide; 016import org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r5.model.Enumeration; 019import org.hl7.fhir.r5.model.ImplementationGuide.GuidePageGeneration; 020 021public class ImplementationGuide14_50 { 022 023 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.r5.model.ImplementationGuide src) throws FHIRException { 024 if (src == null || src.isEmpty()) 025 return null; 026 org.hl7.fhir.dstu2016may.model.ImplementationGuide tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide(); 027 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 028 if (src.hasUrlElement()) 029 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 030 if (src.hasVersion()) 031 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 032 if (src.hasNameElement()) 033 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 034 if (src.hasStatus()) 035 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 036 if (src.hasExperimental()) 037 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 038 if (src.hasPublisher()) 039 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 040 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 041 tgt.addContact(convertImplementationGuideContactComponent(t)); 042 if (src.hasDate()) 043 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 044 if (src.hasDescription()) 045 tgt.setDescription(src.getDescription()); 046 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 047 if (t.hasValueCodeableConcept()) 048 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 049 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 050 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 051 if (src.hasCopyright()) 052 tgt.setCopyright(src.getCopyright()); 053 if (src.hasFhirVersion()) 054 for (Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> v : src.getFhirVersion()) { 055 tgt.setFhirVersion(v.asStringValue()); 056 } 057 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent t : src.getDependsOn()) 058 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 059 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent t : src.getDefinition().getGrouping()) 060 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 061 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent t : src.getDefinition().getResource()) 062 findPackage(tgt.getPackage(), t.getGroupingId()).addResource(convertImplementationGuidePackageResourceComponent(t)); 063 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 064 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 065 tgt.setPage(convertImplementationGuidePageComponent(src.getDefinition().getPage())); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.r5.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu2016may.model.ImplementationGuide src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.r5.model.ImplementationGuide tgt = new org.hl7.fhir.r5.model.ImplementationGuide(); 073 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 074 if (src.hasUrlElement()) 075 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 076 if (src.hasVersion()) 077 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 078 if (src.hasNameElement()) 079 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 080 if (src.hasStatus()) 081 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 082 if (src.hasExperimental()) 083 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 084 if (src.hasPublisher()) 085 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 086 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent t : src.getContact()) 087 tgt.addContact(convertImplementationGuideContactComponent(t)); 088 if (src.hasDate()) 089 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 090 if (src.hasDescription()) 091 tgt.setDescription(src.getDescription()); 092 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 093 if (CodeableConcept14_50.isJurisdiction(t)) 094 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 095 else 096 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 097 if (src.hasCopyright()) 098 tgt.setCopyright(src.getCopyright()); 099 if (src.hasFhirVersion()) 100 tgt.addFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 101 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 102 tgt.addDependsOn(convertImplementationGuideDependencyComponent(t)); 103 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 104 tgt.getDefinition().addGrouping(convertImplementationGuidePackageComponent(tgt.getDefinition(), t)); 105 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 106 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 107 tgt.getDefinition().setPage(convertImplementationGuidePageComponent(src.getPage())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent convertImplementationGuideContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 112 if (src == null || src.isEmpty()) 113 return null; 114 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent(); 115 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 116 if (src.hasName()) 117 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 118 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 119 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 120 return tgt; 121 } 122 123 public static org.hl7.fhir.r5.model.ContactDetail convertImplementationGuideContactComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent src) throws FHIRException { 124 if (src == null || src.isEmpty()) 125 return null; 126 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 127 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 128 if (src.hasName()) 129 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 130 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 131 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 132 return tgt; 133 } 134 135 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent(); 139 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 140 if (src.hasUri()) 141 tgt.setUri(src.getUri()); 142 if (org.hl7.fhir.dstu2016may.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)) { 143 tgt.setPackageId(org.hl7.fhir.dstu2016may.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)); 144 } 145 if (org.hl7.fhir.dstu2016may.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)) { 146 tgt.setVersion(org.hl7.fhir.dstu2016may.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)); 147 } 148 return tgt; 149 } 150 151 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent src) throws FHIRException { 152 if (src == null || src.isEmpty()) 153 return null; 154 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 155 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 156 tgt.setType(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType.REFERENCE); 157 if (src.hasUri()) 158 tgt.setUri(src.getUri()); 159 if (src.hasPackageId()) 160 tgt.addExtension(new org.hl7.fhir.dstu2016may.model.Extension(VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION, new org.hl7.fhir.dstu2016may.model.IdType(src.getPackageId()))); 161 if (src.hasVersion()) 162 tgt.addExtension(new org.hl7.fhir.dstu2016may.model.Extension(VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION, new org.hl7.fhir.dstu2016may.model.StringType(src.getVersion()))); 163 return tgt; 164 } 165 166 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 167 if (src == null || src.isEmpty()) 168 return null; 169 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 170 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 171 if (tgt.hasType()) { 172 if (src.hasTypeElement()) 173 tgt.setTypeElement(Code14_50.convertCode(src.getTypeElement())); 174 } 175 if (src.hasProfileElement()) 176 tgt.setProfile(Reference14_50.convertCanonicalToReference(src.getProfileElement())); 177 return tgt; 178 } 179 180 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 181 if (src == null || src.isEmpty()) 182 return null; 183 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 184 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 185 if (src.hasTypeElement()) 186 tgt.setTypeElement(Code14_50.convertCode(src.getTypeElement())); 187 if (src.hasProfile()) 188 tgt.setProfileElement(Reference14_50.convertReferenceToCanonical(src.getProfile())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent(); 196 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 197 tgt.setId(src.getId()); 198 if (src.hasNameElement()) 199 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 200 if (src.hasDescription()) 201 tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 202 return tgt; 203 } 204 205 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionComponent context, org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 206 if (src == null || src.isEmpty()) 207 return null; 208 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent(); 209 tgt.setId("p" + (context.getGrouping().size() + 1)); 210 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 211 if (src.hasName()) 212 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 213 if (src.hasDescription()) 214 tgt.setDescriptionElement(String14_50.convertStringToMarkdown(src.getDescriptionElement())); 215 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) { 216 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tn = convertImplementationGuidePackageResourceComponent(t); 217 tn.setGroupingId(tgt.getId()); 218 context.addResource(tn); 219 } 220 return tgt; 221 } 222 223 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent src) throws FHIRException { 224 if (src == null || src.isEmpty()) 225 return null; 226 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 227 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 228 if (src.hasProfile()) { 229 tgt.setExampleFor(Reference14_50.convertCanonicalToReference(src.getProfile().get(0))); 230 tgt.setExample(true); 231 } else if (src.hasIsExample()) 232 tgt.setExample(src.getIsExample()); 233 else 234 tgt.setExample(false); 235 if (src.hasName()) 236 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 237 if (src.hasDescription()) 238 tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 239 if (src.hasReference()) 240 tgt.setSource(Reference14_50.convertReference(src.getReference())); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent(); 248 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 249 if (src.hasExampleFor()) { 250 tgt.getProfile().add(Reference14_50.convertReferenceToCanonical(src.getExampleFor())); 251 } else if (src.hasExample()) 252 tgt.setIsExampleElement(new org.hl7.fhir.r5.model.BooleanType(src.getExample())); 253 if (src.hasName()) 254 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 255 if (src.hasDescription()) 256 tgt.setDescriptionElement(String14_50.convertStringToMarkdown(src.getDescriptionElement())); 257 if (src.hasSourceReference()) 258 tgt.setReference(Reference14_50.convertReference(src.getSourceReference())); 259 else if (src.hasSourceUriType()) 260 tgt.setReference(new org.hl7.fhir.r5.model.Reference(src.getSourceUriType().getValue())); 261 return tgt; 262 } 263 264 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent src) throws FHIRException { 265 if (src == null || src.isEmpty()) 266 return null; 267 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent(); 268 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 269 if (src.hasName()) 270 tgt.setSource(src.getName()); 271 if (src.hasTitleElement()) 272 tgt.setNameElement(String14_50.convertString(src.getTitleElement())); 273 if (src.hasGeneration()) 274 tgt.setKind(convertPageGeneration(src.getGeneration())); 275 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent t : src.getPage()) 276 tgt.addPage(convertImplementationGuidePageComponent(t)); 277 return tgt; 278 } 279 280 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 281 if (src == null || src.isEmpty()) 282 return null; 283 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent(); 284 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 285 if (src.hasSource()) 286 tgt.setNameElement(convertUriToUrl(src.getSourceElement())); 287 if (src.hasNameElement()) 288 tgt.setTitleElement(String14_50.convertString(src.getNameElement())); 289 if (src.hasKind()) 290 tgt.setGeneration(convertPageGeneration(src.getKind())); 291 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 292 tgt.addPage(convertImplementationGuidePageComponent(t)); 293 return tgt; 294 } 295 296 static public GuidePageKind convertPageGeneration(GuidePageGeneration generation) { 297 switch (generation) { 298 case HTML: 299 return GuidePageKind.PAGE; 300 default: 301 return GuidePageKind.RESOURCE; 302 } 303 } 304 305 static public GuidePageGeneration convertPageGeneration(GuidePageKind kind) { 306 switch (kind) { 307 case PAGE: 308 return GuidePageGeneration.HTML; 309 default: 310 return GuidePageGeneration.GENERATED; 311 } 312 } 313 314 public static org.hl7.fhir.r5.model.UrlType convertUriToUrl(org.hl7.fhir.dstu2016may.model.UriType src) throws FHIRException { 315 org.hl7.fhir.r5.model.UrlType tgt = new org.hl7.fhir.r5.model.UrlType(); 316 if (src.hasValue()) 317 tgt.setValue(src.getValue()); 318 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 319 return tgt; 320 } 321 322 static public org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent findPackage(List<ImplementationGuide.ImplementationGuidePackageComponent> definition, String id) { 323 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t : definition) 324 if (t.hasId() && t.getId().equals(id)) 325 return t; 326 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent(); 327 t.setName("Default Package"); 328 t.setId(id); 329 return t; 330 } 331}