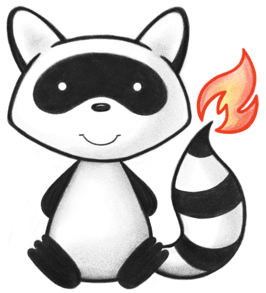
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Reference14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Coding14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Identifier14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Integer14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 014import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 015import org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r5.model.*; 018import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireAnswerConstraint; 019import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator; 020 021public class Questionnaire14_50 { 022 023 public static org.hl7.fhir.r5.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2016may.model.Questionnaire src) throws FHIRException { 024 if (src == null || src.isEmpty()) 025 return null; 026 org.hl7.fhir.r5.model.Questionnaire tgt = new org.hl7.fhir.r5.model.Questionnaire(); 027 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 028 if (src.hasUrl()) 029 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 030 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 031 tgt.addIdentifier(Identifier14_50.convertIdentifier(t)); 032 if (src.hasVersion()) 033 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 034 if (src.hasStatus()) 035 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 036 if (src.hasDate()) 037 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 038 if (src.hasPublisher()) 039 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 040 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 041 tgt.addContact(convertQuestionnaireContactComponent(t)); 042 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 043 if (CodeableConcept14_50.isJurisdiction(t)) 044 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 045 else 046 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 047 if (src.hasTitle()) 048 tgt.setTitleElement(String14_50.convertString(src.getTitleElement())); 049 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Coding14_50.convertCoding(t)); 050 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 051 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 052 tgt.addItem(convertQuestionnaireItemComponent(t)); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu2016may.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r5.model.Questionnaire src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.dstu2016may.model.Questionnaire tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire(); 060 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 061 if (src.hasUrl()) 062 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 063 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 064 tgt.addIdentifier(Identifier14_50.convertIdentifier(t)); 065 if (src.hasVersion()) 066 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 067 if (src.hasStatus()) 068 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 069 if (src.hasDate()) 070 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 071 if (src.hasPublisher()) 072 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 073 for (ContactDetail t : src.getContact()) 074 for (org.hl7.fhir.r5.model.ContactPoint t1 : t.getTelecom()) 075 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t1)); 076 for (UsageContext t : src.getUseContext()) 077 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 078 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 079 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 080 if (src.hasTitle()) 081 tgt.setTitleElement(String14_50.convertString(src.getTitleElement())); 082 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addConcept(Coding14_50.convertCoding(t)); 083 for (CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 084 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 085 tgt.addItem(convertQuestionnaireItemComponent(t)); 086 return tgt; 087 } 088 089 public static org.hl7.fhir.r5.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2016may.model.ContactPoint src) throws FHIRException { 090 if (src == null || src.isEmpty()) 091 return null; 092 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 093 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 094 tgt.addTelecom(ContactPoint14_50.convertContactPoint(src)); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 099 if (src == null || src.isEmpty()) 100 return null; 101 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent(); 102 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 103 if (src.hasLinkId()) 104 tgt.setLinkIdElement(String14_50.convertString(src.getLinkIdElement())); 105 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addConcept(Coding14_50.convertCoding(t)); 106 if (src.hasPrefix()) 107 tgt.setPrefixElement(String14_50.convertString(src.getPrefixElement())); 108 if (src.hasText()) 109 tgt.setTextElement(String14_50.convertString(src.getTextElement())); 110 if (src.hasType()) 111 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement(), src.getAnswerConstraint())); 112 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 113 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 114 if (src.hasRequired()) 115 tgt.setRequiredElement(Boolean14_50.convertBoolean(src.getRequiredElement())); 116 if (src.hasRepeats()) 117 tgt.setRepeatsElement(Boolean14_50.convertBoolean(src.getRepeatsElement())); 118 if (src.hasReadOnly()) 119 tgt.setReadOnlyElement(Boolean14_50.convertBoolean(src.getReadOnlyElement())); 120 if (src.hasMaxLength()) 121 tgt.setMaxLengthElement(Integer14_50.convertInteger(src.getMaxLengthElement())); 122 if (src.hasAnswerValueSetElement()) 123 tgt.setOptions(Reference14_50.convertCanonicalToReference(src.getAnswerValueSetElement())); 124 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 125 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 126 if (src.hasInitial()) 127 tgt.setInitial(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getInitialFirstRep().getValue())); 128 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 129 tgt.addItem(convertQuestionnaireItemComponent(t)); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent(); 137 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 138 if (src.hasLinkId()) 139 tgt.setLinkIdElement(String14_50.convertString(src.getLinkIdElement())); 140 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Coding14_50.convertCoding(t)); 141 if (src.hasPrefix()) 142 tgt.setPrefixElement(String14_50.convertString(src.getPrefixElement())); 143 if (src.hasText()) 144 tgt.setTextElement(String14_50.convertStringToMarkdown(src.getTextElement())); 145 if (src.hasType()) { 146 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 147 if (src.getType() == QuestionnaireItemType.CHOICE) { 148 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSONLY); 149 } else if (src.getType() == QuestionnaireItemType.OPENCHOICE) { 150 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSORSTRING); 151 } 152 } 153 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 154 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 155 if (src.hasRequired()) 156 tgt.setRequiredElement(Boolean14_50.convertBoolean(src.getRequiredElement())); 157 if (src.hasRepeats()) 158 tgt.setRepeatsElement(Boolean14_50.convertBoolean(src.getRepeatsElement())); 159 if (src.hasReadOnly()) 160 tgt.setReadOnlyElement(Boolean14_50.convertBoolean(src.getReadOnlyElement())); 161 if (src.hasMaxLength()) 162 tgt.setMaxLengthElement(Integer14_50.convertInteger(src.getMaxLengthElement())); 163 if (src.hasOptions()) 164 tgt.setAnswerValueSetElement(Reference14_50.convertReferenceToCanonical(src.getOptions())); 165 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 166 tgt.addAnswerOption(convertQuestionnaireItemOptionComponent(t)); 167 if (src.hasInitial()) 168 tgt.addInitial().setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getInitial())); 169 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 170 tgt.addItem(convertQuestionnaireItemComponent(t)); 171 return tgt; 172 } 173 174 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 178 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 179 if (src.hasQuestionElement()) 180 tgt.setQuestionElement(String14_50.convertString(src.getQuestionElement())); 181 if (src.hasAnswered()) { 182 tgt.setOperator(QuestionnaireItemOperator.EXISTS); 183 if (src.hasAnsweredElement()) 184 tgt.setAnswer(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getAnsweredElement())); 185 } 186 if (src.hasAnswer()) 187 tgt.setAnswer(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getAnswer())); 188 return tgt; 189 } 190 191 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 192 if (src == null || src.isEmpty()) 193 return null; 194 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 195 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 196 if (src.hasQuestionElement()) 197 tgt.setQuestionElement(String14_50.convertString(src.getQuestionElement())); 198 if (src.hasOperator() && src.getOperator() == QuestionnaireItemOperator.EXISTS) 199 tgt.setAnswered(src.getAnswerBooleanType().getValue()); 200 else 201 tgt.setAnswer(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getAnswer())); 202 return tgt; 203 } 204 205 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 206 if (src == null || src.isEmpty()) 207 return null; 208 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent(); 209 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 210 if (src.hasValue()) 211 tgt.setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getValue())); 212 return tgt; 213 } 214 215 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 216 if (src == null || src.isEmpty()) 217 return null; 218 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 219 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 220 if (src.hasValue()) 221 tgt.setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getValue())); 222 return tgt; 223 } 224 225 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 226 if (src == null || src.isEmpty()) 227 return null; 228 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 229 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 230 tgt.addExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL, new CodeType(src.getValueAsString())); 231 if (src.getValue() == null) { 232 tgt.setValue(null); 233 } else { 234 switch (src.getValue()) { 235 case GROUP: 236 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 237 break; 238 case DISPLAY: 239 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 240 break; 241 case QUESTION: 242 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 243 break; 244 case BOOLEAN: 245 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 246 break; 247 case DECIMAL: 248 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 249 break; 250 case INTEGER: 251 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 252 break; 253 case DATE: 254 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 255 break; 256 case DATETIME: 257 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 258 break; 259 case INSTANT: 260 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 261 break; 262 case TIME: 263 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 264 break; 265 case STRING: 266 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 267 break; 268 case TEXT: 269 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 270 break; 271 case URL: 272 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 273 break; 274 case CHOICE: 275 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 276 break; 277 case OPENCHOICE: 278 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 279 break; 280 case ATTACHMENT: 281 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 282 break; 283 case REFERENCE: 284 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 285 break; 286 case QUANTITY: 287 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 288 break; 289 default: 290 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 291 break; 292 } 293 } 294 return tgt; 295 } 296 297 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> src, QuestionnaireAnswerConstraint constraint) throws FHIRException { 298 if (src == null || src.isEmpty()) 299 return null; 300 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 301 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt, VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL); 302 if (src.hasExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)) { 303 tgt.setValueAsString(src.getExtensionString(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)); 304 } else { 305 if (src.getValue() == null) { 306 tgt.setValue(null); 307 } else { 308 switch (src.getValue()) { 309 case GROUP: 310 tgt.setValue(QuestionnaireItemType.GROUP); 311 break; 312 case DISPLAY: 313 tgt.setValue(QuestionnaireItemType.DISPLAY); 314 break; 315 case BOOLEAN: 316 tgt.setValue(QuestionnaireItemType.BOOLEAN); 317 break; 318 case DECIMAL: 319 tgt.setValue(QuestionnaireItemType.DECIMAL); 320 break; 321 case INTEGER: 322 tgt.setValue(QuestionnaireItemType.INTEGER); 323 break; 324 case DATE: 325 tgt.setValue(QuestionnaireItemType.DATE); 326 break; 327 case DATETIME: 328 tgt.setValue(QuestionnaireItemType.DATETIME); 329 break; 330 case TIME: 331 tgt.setValue(QuestionnaireItemType.TIME); 332 break; 333 case STRING: 334 tgt.setValue(QuestionnaireItemType.STRING); 335 break; 336 case TEXT: 337 tgt.setValue(QuestionnaireItemType.TEXT); 338 break; 339 case URL: 340 tgt.setValue(QuestionnaireItemType.URL); 341 break; 342 case CODING: 343 if (constraint == QuestionnaireAnswerConstraint.OPTIONSORSTRING) 344 tgt.setValue(QuestionnaireItemType.OPENCHOICE); 345 else 346 tgt.setValue(QuestionnaireItemType.CHOICE); 347 break; 348 case ATTACHMENT: 349 tgt.setValue(QuestionnaireItemType.ATTACHMENT); 350 break; 351 case REFERENCE: 352 tgt.setValue(QuestionnaireItemType.REFERENCE); 353 break; 354 case QUANTITY: 355 tgt.setValue(QuestionnaireItemType.QUANTITY); 356 break; 357 default: 358 tgt.setValue(QuestionnaireItemType.NULL); 359 break; 360 } 361 } 362 } 363 return tgt; 364 } 365 366 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 367 if (src == null || src.isEmpty()) 368 return null; 369 Enumeration<Enumerations.PublicationStatus> tgt = new Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 370 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 371 if (src.getValue() == null) { 372 tgt.setValue(null); 373 } else { 374 switch (src.getValue()) { 375 case DRAFT: 376 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 377 break; 378 case PUBLISHED: 379 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 380 break; 381 case RETIRED: 382 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 383 break; 384 default: 385 tgt.setValue(Enumerations.PublicationStatus.NULL); 386 break; 387 } 388 } 389 return tgt; 390 } 391 392 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> src) throws FHIRException { 393 if (src == null || src.isEmpty()) 394 return null; 395 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatusEnumFactory()); 396 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 397 if (src.getValue() == null) { 398 tgt.setValue(null); 399 } else { 400 switch (src.getValue()) { 401 case DRAFT: 402 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.DRAFT); 403 break; 404 case ACTIVE: 405 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.PUBLISHED); 406 break; 407 case RETIRED: 408 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.RETIRED); 409 break; 410 default: 411 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.NULL); 412 break; 413 } 414 } 415 return tgt; 416 } 417}