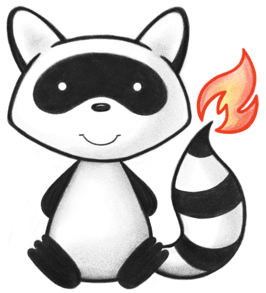
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.ElementDefinition14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 012import org.hl7.fhir.dstu2016may.model.SearchParameter; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAll; 016import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAllEnumFactory; 017 018public class SearchParameter14_50 { 019 020 public static org.hl7.fhir.dstu2016may.model.SearchParameter convertSearchParameter(org.hl7.fhir.r5.model.SearchParameter src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.dstu2016may.model.SearchParameter tgt = new org.hl7.fhir.dstu2016may.model.SearchParameter(); 024 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 025 if (src.hasUrlElement()) 026 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 027 if (src.hasNameElement()) 028 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 029 if (src.hasStatus()) 030 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 031 if (src.hasExperimental()) 032 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 033 if (src.hasDate()) 034 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 035 if (src.hasPublisher()) 036 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 037 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 038 tgt.addContact(convertSearchParameterContactComponent(t)); 039 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 040 if (t.hasValueCodeableConcept()) 041 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 042 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 043 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 044 if (src.hasPurpose()) 045 tgt.setRequirements(src.getPurpose()); 046 if (src.hasCodeElement()) 047 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 048 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getBase()) tgt.setBaseElement(Code14_50.convertCode(t.getCodeType())); 049 if (src.hasType()) 050 tgt.setTypeElement(Enumerations14_50.convertSearchParamType(src.getTypeElement())); 051 if (src.hasDescription()) 052 tgt.setDescription(src.getDescription()); 053 if (src.hasExpression()) 054 tgt.setExpression(ElementDefinition14_50.convertTo2016MayExpression(src.getExpression())); 055// if (src.hasXpath()) 056// tgt.setXpathElement(String14_50.convertString(src.getXpathElement())); 057 if (src.hasProcessingMode()) 058 tgt.setXpathUsageElement(convertXPathUsageType(src.getProcessingModeElement())); 059 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getTarget()) tgt.getTarget().add(Code14_50.convertCode(t.getCodeType())); 060 return tgt; 061 } 062 063 public static org.hl7.fhir.r5.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu2016may.model.SearchParameter src) throws FHIRException { 064 if (src == null || src.isEmpty()) 065 return null; 066 org.hl7.fhir.r5.model.SearchParameter tgt = new org.hl7.fhir.r5.model.SearchParameter(); 067 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 068 if (src.hasUrlElement()) 069 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 070 if (src.hasNameElement()) 071 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 072 if (src.hasStatus()) 073 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 074 if (src.hasExperimental()) 075 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 076 if (src.hasDate()) 077 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 078 if (src.hasPublisher()) 079 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 080 for (org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent t : src.getContact()) 081 tgt.addContact(convertSearchParameterContactComponent(t)); 082 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 083 if (CodeableConcept14_50.isJurisdiction(t)) 084 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 085 else 086 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 087 if (src.hasRequirements()) 088 tgt.setPurpose(src.getRequirements()); 089 if (src.hasCodeElement()) 090 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 091 tgt.getBase().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), Code14_50.convertCode(src.getBaseElement()))); 092 if (src.hasType()) 093 tgt.setTypeElement(Enumerations14_50.convertSearchParamType(src.getTypeElement())); 094 if (src.hasDescription()) 095 tgt.setDescription(src.getDescription()); 096 if (src.hasExpression()) 097 tgt.setExpression(ElementDefinition14_50.convertToR4Expression(src.getExpression())); 098// if (src.hasXpath()) 099// tgt.setXpathElement(String14_50.convertString(src.getXpathElement())); 100 if (src.hasXpathUsage()) 101 tgt.setProcessingModeElement(convertXPathUsageType(src.getXpathUsageElement())); 102 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getTarget()) tgt.getTarget().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), t.getValue())); 103 return tgt; 104 } 105 106 public static org.hl7.fhir.r5.model.ContactDetail convertSearchParameterContactComponent(org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent src) throws FHIRException { 107 if (src == null || src.isEmpty()) 108 return null; 109 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 110 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 111 if (src.hasName()) 112 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 113 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 114 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 115 return tgt; 116 } 117 118 public static org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent convertSearchParameterContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 119 if (src == null || src.isEmpty()) 120 return null; 121 org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent tgt = new org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent(); 122 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 123 if (src.hasName()) 124 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 125 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 126 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 127 return tgt; 128 } 129 130 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu2016may.model.Enumeration<SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new SearchParameter.XPathUsageTypeEnumFactory()); 134 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 135 if (src.getValue() == null) { 136 tgt.setValue(null); 137 } else { 138 switch (src.getValue()) { 139 case NORMAL: 140 tgt.setValue(SearchParameter.XPathUsageType.NORMAL); 141 break; 142 case PHONETIC: 143 tgt.setValue(SearchParameter.XPathUsageType.PHONETIC); 144 break; 145 146 case OTHER: 147 tgt.setValue(SearchParameter.XPathUsageType.OTHER); 148 break; 149 default: 150 tgt.setValue(SearchParameter.XPathUsageType.NULL); 151 break; 152 } 153 } 154 return tgt; 155 } 156 157 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> convertXPathUsageType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType> src) throws FHIRException { 158 if (src == null || src.isEmpty()) 159 return null; 160 Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> tgt = new Enumeration<>(new org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeTypeEnumFactory()); 161 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 162 if (src.getValue() == null) { 163 tgt.setValue(null); 164 } else { 165 switch (src.getValue()) { 166 case NORMAL: 167 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.NORMAL); 168 break; 169 case PHONETIC: 170 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.PHONETIC); 171 break; 172 case NEARBY: 173 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 174 break; 175 case DISTANCE: 176 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 177 break; 178 case OTHER: 179 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 180 break; 181 default: 182 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.NULL); 183 break; 184 } 185 } 186 return tgt; 187 } 188}