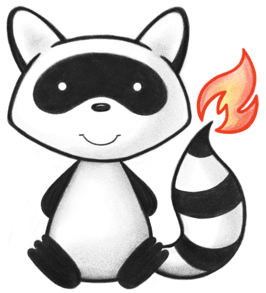
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.ElementDefinition14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Coding14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Identifier14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Id14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.ElementDefinition; 016import org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule; 017import org.hl7.fhir.utilities.Utilities; 018 019public class StructureDefinition14_50 { 020 021 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> convertExtensionContext(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 022 if (src == null || src.isEmpty()) 023 return null; 024 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextTypeEnumFactory()); 025 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 026 switch (src.getValue()) { 027 case RESOURCE: 028 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.ELEMENT); 029 break; 030 case DATATYPE: 031 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.ELEMENT); 032 break; 033 case EXTENSION: 034 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.EXTENSION); 035 break; 036 default: 037 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.NULL); 038 break; 039 } 040 return tgt; 041 } 042 043 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> src, String expression) throws FHIRException { 044 if (src == null || src.isEmpty()) 045 return null; 046 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContextEnumFactory()); 047 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 048 switch (src.getValue()) { 049 case FHIRPATH: 050 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.RESOURCE); 051 break; 052 case ELEMENT: 053 String tn = expression.contains(".") ? expression.substring(0, expression.indexOf(".")) : expression; 054 if (isResource140(tn)) { 055 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.RESOURCE); 056 } else { 057 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.DATATYPE); 058 } 059 break; 060 case EXTENSION: 061 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.EXTENSION); 062 break; 063 default: 064 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.NULL); 065 break; 066 } 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu2016may.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r5.model.StructureDefinition src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.dstu2016may.model.StructureDefinition tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition(); 074 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 075 if (src.hasUrl()) 076 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 077 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 078 tgt.addIdentifier(Identifier14_50.convertIdentifier(t)); 079 if (src.hasVersion()) 080 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 081 if (src.hasNameElement()) 082 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 083 if (src.hasTitle()) 084 tgt.setDisplayElement(String14_50.convertString(src.getTitleElement())); 085 if (src.hasStatus()) 086 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 087 if (src.hasExperimental()) 088 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 089 if (src.hasPublisher()) 090 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 091 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 092 tgt.addContact(convertStructureDefinitionContactComponent(t)); 093 if (src.hasDate()) 094 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 095 if (src.hasDescription()) 096 tgt.setDescription(src.getDescription()); 097 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 098 if (t.hasValueCodeableConcept()) 099 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 100 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 101 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 102 if (src.hasPurpose()) 103 tgt.setRequirements(src.getPurpose()); 104 if (src.hasCopyright()) 105 tgt.setCopyright(src.getCopyright()); 106 for (org.hl7.fhir.r5.model.Coding t : src.getKeyword()) tgt.addCode(Coding14_50.convertCoding(t)); 107 if (src.hasFhirVersion()) 108 tgt.setFhirVersion(src.getFhirVersion().toCode()); 109 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 110 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 111 if (src.hasKind()) 112 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 113 if (src.hasAbstractElement()) 114 tgt.setAbstractElement(Boolean14_50.convertBoolean(src.getAbstractElement())); 115 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) { 116 if (!tgt.hasContextType()) 117 tgt.setContextTypeElement(convertExtensionContext(t.getTypeElement(), t.getExpression())); 118 tgt.addContext("Element".equals(t.getExpression()) ? "*" : t.getExpression()); 119 } 120 if (src.hasBaseDefinition()) 121 tgt.setBaseDefinition(src.getBaseDefinition()); 122 if (src.hasType() && src.getDerivation() == org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.CONSTRAINT) 123 tgt.setBaseType(src.getType()); 124 if (src.hasDerivation()) 125 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 126 if (src.hasSnapshot()) 127 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 128 if (src.hasDifferential()) 129 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.r5.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu2016may.model.StructureDefinition src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.r5.model.StructureDefinition tgt = new org.hl7.fhir.r5.model.StructureDefinition(); 137 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 138 if (src.hasUrl()) 139 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 140 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 141 tgt.addIdentifier(Identifier14_50.convertIdentifier(t)); 142 if (src.hasVersion()) 143 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 144 if (src.hasNameElement()) 145 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 146 if (src.hasDisplay()) 147 tgt.setTitleElement(String14_50.convertString(src.getDisplayElement())); 148 if (src.hasStatus()) 149 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 150 if (src.hasExperimental()) 151 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 152 if (src.hasPublisher()) 153 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 154 for (org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent t : src.getContact()) 155 tgt.addContact(convertStructureDefinitionContactComponent(t)); 156 if (src.hasDate()) 157 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 158 if (src.hasDescription()) 159 tgt.setDescription(src.getDescription()); 160 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 161 if (CodeableConcept14_50.isJurisdiction(t)) 162 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 163 else 164 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 165 if (src.hasRequirements()) 166 tgt.setPurpose(src.getRequirements()); 167 if (src.hasCopyright()) 168 tgt.setCopyright(src.getCopyright()); 169 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getCode()) tgt.addKeyword(Coding14_50.convertCoding(t)); 170 if (src.hasFhirVersion()) 171 tgt.setFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 172 for (org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 173 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 174 if (src.hasKind()) 175 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement(), src.getName())); 176 if (src.hasAbstractElement()) 177 tgt.setAbstractElement(Boolean14_50.convertBoolean(src.getAbstractElement())); 178 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getContext()) { 179 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent ec = tgt.addContext(); 180 ec.setTypeElement(convertExtensionContext(src.getContextTypeElement())); 181 ec.setExpression("*".equals(t.getValue()) ? "Element" : t.getValue()); 182 } 183 if (src.getDerivation() == org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.CONSTRAINT) 184 tgt.setType(src.getBaseType()); 185 else 186 tgt.setType(src.getId()); 187 if (src.hasBaseDefinition()) 188 tgt.setBaseDefinition(src.getBaseDefinition()); 189 if (src.hasDerivation()) 190 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 191 if (src.hasSnapshot()) { 192 if (src.hasSnapshot()) 193 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 194 tgt.getSnapshot().getElementFirstRep().getType().clear(); 195 } 196 if (src.hasDifferential()) { 197 if (src.hasDifferential()) 198 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 199 if (!tgt.getDifferential().getElementFirstRep().getPath().contains(".")) { 200 tgt.getDifferential().getElementFirstRep().getType().clear(); 201 } 202 } 203 if (tgt.getDerivation() == TypeDerivationRule.SPECIALIZATION) { 204 for (ElementDefinition ed : tgt.getSnapshot().getElement()) { 205 if (!ed.hasBase()) { 206 ed.getBase().setPath(ed.getPath()).setMin(ed.getMin()).setMax(ed.getMax()); 207 } 208 } 209 } 210 return tgt; 211 } 212 213 public static org.hl7.fhir.r5.model.ContactDetail convertStructureDefinitionContactComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent src) throws FHIRException { 214 if (src == null || src.isEmpty()) 215 return null; 216 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 217 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 218 if (src.hasName()) 219 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 220 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 221 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 222 return tgt; 223 } 224 225 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent convertStructureDefinitionContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 226 if (src == null || src.isEmpty()) 227 return null; 228 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent(); 229 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 230 if (src.hasName()) 231 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 232 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 233 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 234 return tgt; 235 } 236 237 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 241 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 242 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 243 tgt.addElement(ElementDefinition14_50.convertElementDefinition(t)); 244 return tgt; 245 } 246 247 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 248 if (src == null || src.isEmpty()) 249 return null; 250 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 251 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 252 for (org.hl7.fhir.dstu2016may.model.ElementDefinition t : src.getElement()) 253 tgt.addElement(ElementDefinition14_50.convertElementDefinition(t, src.getElement(), src.getElement().indexOf(t))); 254 return tgt; 255 } 256 257 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 258 if (src == null || src.isEmpty()) 259 return null; 260 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 261 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 262 switch (src.getValue()) { 263 case PRIMITIVETYPE: 264 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 265 break; 266 case COMPLEXTYPE: 267 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 268 break; 269 case RESOURCE: 270 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 271 break; 272 case LOGICAL: 273 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 274 break; 275 default: 276 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.NULL); 277 break; 278 } 279 return tgt; 280 } 281 282 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> src, String name) throws FHIRException { 283 if (src == null || src.isEmpty()) 284 return null; 285 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 286 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 287 switch (src.getValue()) { 288 case DATATYPE: 289 if (name.substring(0, 1).toLowerCase().equals(name.substring(0, 1))) { 290 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 291 } else { 292 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 293 } 294 break; 295 case RESOURCE: 296 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 297 break; 298 case LOGICAL: 299 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 300 break; 301 default: 302 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.NULL); 303 break; 304 } 305 return tgt; 306 } 307 308 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 309 if (src == null || src.isEmpty()) 310 return null; 311 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent(); 312 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 313 if (src.hasIdentityElement()) 314 tgt.setIdentityElement(Id14_50.convertId(src.getIdentityElement())); 315 if (src.hasUri()) 316 tgt.setUriElement(Uri14_50.convertUri(src.getUriElement())); 317 if (src.hasName()) 318 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 319 if (src.hasComments()) 320 tgt.setCommentElement(String14_50.convertString(src.getCommentsElement())); 321 return tgt; 322 } 323 324 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 325 if (src == null || src.isEmpty()) 326 return null; 327 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent(); 328 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 329 if (src.hasIdentityElement()) 330 tgt.setIdentityElement(Id14_50.convertId(src.getIdentityElement())); 331 if (src.hasUri()) 332 tgt.setUriElement(Uri14_50.convertUri(src.getUriElement())); 333 if (src.hasName()) 334 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 335 if (src.hasComment()) 336 tgt.setCommentsElement(String14_50.convertString(src.getCommentElement())); 337 return tgt; 338 } 339 340 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 341 if (src == null || src.isEmpty()) 342 return null; 343 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 344 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 345 for (org.hl7.fhir.dstu2016may.model.ElementDefinition t : src.getElement()) 346 tgt.addElement(ElementDefinition14_50.convertElementDefinition(t, src.getElement(), src.getElement().indexOf(t))); 347 return tgt; 348 } 349 350 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 351 if (src == null || src.isEmpty()) 352 return null; 353 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 354 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 355 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 356 tgt.addElement(ElementDefinition14_50.convertElementDefinition(t)); 357 return tgt; 358 } 359 360 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 361 if (src == null || src.isEmpty()) 362 return null; 363 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 364 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 365 switch (src.getValue()) { 366 case SPECIALIZATION: 367 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 368 break; 369 case CONSTRAINT: 370 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 371 break; 372 default: 373 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.NULL); 374 break; 375 } 376 return tgt; 377 } 378 379 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 380 if (src == null || src.isEmpty()) 381 return null; 382 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 383 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 384 switch (src.getValue()) { 385 case SPECIALIZATION: 386 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 387 break; 388 case CONSTRAINT: 389 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 390 break; 391 default: 392 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.NULL); 393 break; 394 } 395 return tgt; 396 } 397 398 static public boolean isResource140(String tn) { 399 return Utilities.existsInList(tn, "Account", "AllergyIntolerance", "Appointment", "AppointmentResponse", "AuditEvent", "Basic", "Binary", "BodySite", "Bundle", "CarePlan", "CareTeam", "Claim", "ClaimResponse", "ClinicalImpression", "CodeSystem", "Communication", "CommunicationRequest", "CompartmentDefinition", "Composition", "ConceptMap", "Condition", "Conformance", "Contract", "Coverage", "DataElement", "DecisionSupportRule", "DecisionSupportServiceModule", "DetectedIssue", "Device", "DeviceComponent", "DeviceMetric", "DeviceUseRequest", "DeviceUseStatement", "DiagnosticOrder", "DiagnosticReport", "DocumentManifest", "DocumentReference", "EligibilityRequest", "EligibilityResponse", "Encounter", "EnrollmentRequest", "EnrollmentResponse", "EpisodeOfCare", "ExpansionProfile", "ExplanationOfBenefit", "FamilyMemberHistory", "Flag", "Goal", "Group", "GuidanceResponse", "HealthcareService", "ImagingExcerpt", "ImagingObjectSelection", "ImagingStudy", "Immunization", "ImmunizationRecommendation", "ImplementationGuide", "Library", "Linkage", "List", "Location", "Measure", "MeasureReport", "Media", "Medication", "MedicationAdministration", "MedicationDispense", "MedicationOrder", "MedicationStatement", "MessageHeader", "ModuleDefinition", "NamingSystem", "NutritionOrder", "Observation", "OperationDefinition", "OperationOutcome", "Order", "OrderResponse", "OrderSet", "Organization", "Parameters", "Patient", "PaymentNotice", "PaymentReconciliation", "Person", "Practitioner", "PractitionerRole", "Procedure", "ProcedureRequest", "ProcessRequest", "ProcessResponse", "Protocol", "Provenance", "Questionnaire", "QuestionnaireResponse", "ReferralRequest", "RelatedPerson", "RiskAssessment", "Schedule", "SearchParameter", "Sequence", "Slot", "Sequence", "Specimen", "StructureDefinition", "StructureMap", "Subscription", "Substance", "SupplyDelivery", "SupplyRequest", "Task", "TestScript", "ValueSet", "VisionPrescription"); 400 } 401}