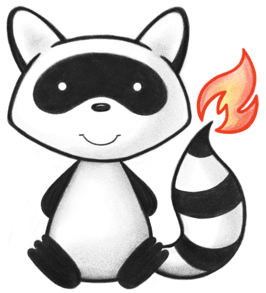
001package org.hl7.fhir.convertors.conv14_50.resources14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.CodeableConcept14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Coding14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.ContactPoint14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Identifier14_50; 008import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.DateTime14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Integer14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.BooleanType; 016 017public class ValueSet14_50 { 018 019 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent(); 023 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 024 if (src.hasCodeElement()) 025 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 026 if (src.hasDisplay()) 027 tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 028 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 029 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 030 return tgt; 031 } 032 033 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 034 if (src == null || src.isEmpty()) 035 return null; 036 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent(); 037 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 038 if (src.hasCodeElement()) 039 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 040 if (src.hasDisplay()) 041 tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 042 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 043 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 044 return tgt; 045 } 046 047 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent(); 051 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 052 if (src.hasLanguage()) 053 tgt.setLanguageElement(Code14_50.convertCode(src.getLanguageElement())); 054 if (src.hasUse()) 055 tgt.setUse(Coding14_50.convertCoding(src.getUse())); 056 if (src.hasValueElement()) 057 tgt.setValueElement(String14_50.convertString(src.getValueElement())); 058 return tgt; 059 } 060 061 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 062 if (src == null || src.isEmpty()) 063 return null; 064 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent(); 065 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 066 if (src.hasLanguage()) 067 tgt.setLanguageElement(Code14_50.convertCode(src.getLanguageElement())); 068 if (src.hasUse()) 069 tgt.setUse(Coding14_50.convertCoding(src.getUse())); 070 if (src.hasValueElement()) 071 tgt.setValueElement(String14_50.convertString(src.getValueElement())); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent src) throws FHIRException { 076 if (src == null || src.isEmpty()) 077 return null; 078 org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent(); 079 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 080 if (src.hasSystemElement()) 081 tgt.setSystemElement(Uri14_50.convertUri(src.getSystemElement())); 082 if (src.hasVersion()) 083 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 084 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 085 tgt.addConcept(convertConceptReferenceComponent(t)); 086 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 087 tgt.addFilter(convertConceptSetFilterComponent(t)); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent(); 095 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 096 if (src.hasSystemElement()) 097 tgt.setSystemElement(Uri14_50.convertUri(src.getSystemElement())); 098 if (src.hasVersion()) 099 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 100 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 101 tgt.addConcept(convertConceptReferenceComponent(t)); 102 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 103 tgt.addFilter(convertConceptSetFilterComponent(t)); 104 return tgt; 105 } 106 107 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent(); 111 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 112 if (src.hasPropertyElement()) 113 tgt.setPropertyElement(Code14_50.convertCode(src.getPropertyElement())); 114 if (src.hasOp()) 115 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 116 if (src.hasValue()) 117 tgt.setValue(src.getValue()); 118 return tgt; 119 } 120 121 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 122 if (src == null || src.isEmpty()) 123 return null; 124 org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent(); 125 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 126 if (src.hasPropertyElement()) 127 tgt.setPropertyElement(Code14_50.convertCode(src.getPropertyElement())); 128 if (src.hasOp()) 129 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 130 if (src.hasValue()) 131 tgt.setValue(src.getValue()); 132 return tgt; 133 } 134 135 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.FilterOperatorEnumFactory()); 139 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 140 switch (src.getValue()) { 141 case EQUAL: 142 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.EQUAL); 143 break; 144 case ISA: 145 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.ISA); 146 break; 147 case ISNOTA: 148 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.ISNOTA); 149 break; 150 case REGEX: 151 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.REGEX); 152 break; 153 case IN: 154 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.IN); 155 break; 156 case NOTIN: 157 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.NOTIN); 158 break; 159 default: 160 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FilterOperator.NULL); 161 break; 162 } 163 return tgt; 164 } 165 166 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> src) throws FHIRException { 167 if (src == null || src.isEmpty()) 168 return null; 169 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperatorEnumFactory()); 170 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 171 switch (src.getValue()) { 172 case EQUAL: 173 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.EQUAL); 174 break; 175 case ISA: 176 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.ISA); 177 break; 178 case ISNOTA: 179 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.ISNOTA); 180 break; 181 case REGEX: 182 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.REGEX); 183 break; 184 case IN: 185 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.IN); 186 break; 187 case NOTIN: 188 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.NOTIN); 189 break; 190 default: 191 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.NULL); 192 break; 193 } 194 return tgt; 195 } 196 197 public static org.hl7.fhir.r5.model.ValueSet convertValueSet(org.hl7.fhir.dstu2016may.model.ValueSet src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r5.model.ValueSet tgt = new org.hl7.fhir.r5.model.ValueSet(); 201 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 202 if (src.hasUrl()) 203 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 204 if (src.hasIdentifier()) 205 tgt.addIdentifier(Identifier14_50.convertIdentifier(src.getIdentifier())); 206 if (src.hasVersion()) 207 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 208 if (src.hasName()) 209 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 210 if (src.hasStatus()) 211 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 212 if (src.hasExperimental()) 213 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 214 if (src.hasPublisher()) 215 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 216 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent t : src.getContact()) 217 tgt.addContact(convertValueSetContactComponent(t)); 218 if (src.hasDate()) 219 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 220 if (src.hasDescription()) 221 tgt.setDescription(src.getDescription()); 222 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 223 if (CodeableConcept14_50.isJurisdiction(t)) 224 tgt.addJurisdiction(CodeableConcept14_50.convertCodeableConcept(t)); 225 else 226 tgt.addUseContext(CodeableConcept14_50.convertCodeableConceptToUsageContext(t)); 227 if (src.hasImmutable()) 228 tgt.setImmutableElement(Boolean14_50.convertBoolean(src.getImmutableElement())); 229 if (src.hasRequirements()) 230 tgt.setPurpose(src.getRequirements()); 231 if (src.hasCopyright()) 232 tgt.setCopyright(src.getCopyright()); 233 if (src.hasExtensible()) 234 tgt.addExtension("http://hl7.org/fhir/StructureDefinition/valueset-extensible", new BooleanType(src.getExtensible())); 235 if (src.hasCompose()) 236 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 237 if (src.hasLockedDate()) 238 tgt.getCompose().setLockedDate(src.getLockedDate()); 239 if (src.hasExpansion()) 240 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.dstu2016may.model.ValueSet convertValueSet(org.hl7.fhir.r5.model.ValueSet src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.dstu2016may.model.ValueSet tgt = new org.hl7.fhir.dstu2016may.model.ValueSet(); 248 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyDomainResource(src, tgt); 249 if (src.hasUrl()) 250 tgt.setUrlElement(Uri14_50.convertUri(src.getUrlElement())); 251 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 252 tgt.setIdentifier(Identifier14_50.convertIdentifier(t)); 253 if (src.hasVersion()) 254 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 255 if (src.hasName()) 256 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 257 if (src.hasStatus()) 258 tgt.setStatusElement(Enumerations14_50.convertConformanceResourceStatus(src.getStatusElement())); 259 if (src.hasExperimental()) 260 tgt.setExperimentalElement(Boolean14_50.convertBoolean(src.getExperimentalElement())); 261 if (src.hasPublisher()) 262 tgt.setPublisherElement(String14_50.convertString(src.getPublisherElement())); 263 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) tgt.addContact(convertValueSetContactComponent(t)); 264 if (src.hasDate()) 265 tgt.setDateElement(DateTime14_50.convertDateTime(src.getDateElement())); 266 if (src.getCompose().hasLockedDate()) 267 tgt.setLockedDate(src.getCompose().getLockedDate()); 268 if (src.hasDescription()) 269 tgt.setDescription(src.getDescription()); 270 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 271 if (t.hasValueCodeableConcept()) 272 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t.getValueCodeableConcept())); 273 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 274 tgt.addUseContext(CodeableConcept14_50.convertCodeableConcept(t)); 275 if (src.hasImmutable()) 276 tgt.setImmutableElement(Boolean14_50.convertBoolean(src.getImmutableElement())); 277 if (src.hasPurpose()) 278 tgt.setRequirements(src.getPurpose()); 279 if (src.hasCopyright()) 280 tgt.setCopyright(src.getCopyright()); 281 if (src.hasExtension("http://hl7.org/fhir/StructureDefinition/valueset-extensible")) 282 tgt.setExtensible(((BooleanType) src.getExtensionByUrl("http://hl7.org/fhir/StructureDefinition/valueset-extensible").getValue()).booleanValue()); 283 if (src.hasCompose()) 284 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 285 if (src.hasExpansion()) 286 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 287 return tgt; 288 } 289 290 public static org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 291 if (src == null || src.isEmpty()) 292 return null; 293 org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent(); 294 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 295 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getImport()) tgt.addInclude().addValueSet(t.getValue()); 296 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent t : src.getInclude()) 297 tgt.addInclude(convertConceptSetComponent(t)); 298 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent t : src.getExclude()) 299 tgt.addExclude(convertConceptSetComponent(t)); 300 return tgt; 301 } 302 303 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 304 if (src == null || src.isEmpty()) 305 return null; 306 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent(); 307 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 308 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getInclude()) { 309 for (org.hl7.fhir.r5.model.UriType ti : t.getValueSet()) tgt.addImport(ti.getValue()); 310 tgt.addInclude(convertConceptSetComponent(t)); 311 } 312 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getExclude()) 313 tgt.addExclude(convertConceptSetComponent(t)); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent convertValueSetContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 318 if (src == null || src.isEmpty()) 319 return null; 320 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent(); 321 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 322 if (src.hasName()) 323 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 324 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 325 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 326 return tgt; 327 } 328 329 public static org.hl7.fhir.r5.model.ContactDetail convertValueSetContactComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent src) throws FHIRException { 330 if (src == null || src.isEmpty()) 331 return null; 332 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 333 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 334 if (src.hasName()) 335 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 336 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 337 tgt.addTelecom(ContactPoint14_50.convertContactPoint(t)); 338 return tgt; 339 } 340 341 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 342 if (src == null || src.isEmpty()) 343 return null; 344 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent(); 345 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 346 if (src.hasIdentifierElement()) 347 tgt.setIdentifierElement(Uri14_50.convertUri(src.getIdentifierElement())); 348 if (src.hasTimestampElement()) 349 tgt.setTimestampElement(DateTime14_50.convertDateTime(src.getTimestampElement())); 350 if (src.hasTotal()) 351 tgt.setTotalElement(Integer14_50.convertInteger(src.getTotalElement())); 352 if (src.hasOffset()) 353 tgt.setOffsetElement(Integer14_50.convertInteger(src.getOffsetElement())); 354 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 355 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 356 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 357 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 358 return tgt; 359 } 360 361 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 362 if (src == null || src.isEmpty()) 363 return null; 364 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent(); 365 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 366 if (src.hasIdentifierElement()) 367 tgt.setIdentifierElement(Uri14_50.convertUri(src.getIdentifierElement())); 368 if (src.hasTimestampElement()) 369 tgt.setTimestampElement(DateTime14_50.convertDateTime(src.getTimestampElement())); 370 if (src.hasTotal()) 371 tgt.setTotalElement(Integer14_50.convertInteger(src.getTotalElement())); 372 if (src.hasOffset()) 373 tgt.setOffsetElement(Integer14_50.convertInteger(src.getOffsetElement())); 374 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 375 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 376 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 377 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 378 return tgt; 379 } 380 381 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 382 if (src == null || src.isEmpty()) 383 return null; 384 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent(); 385 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 386 if (src.hasSystem()) 387 tgt.setSystemElement(Uri14_50.convertUri(src.getSystemElement())); 388 if (src.hasAbstract()) 389 tgt.setAbstractElement(Boolean14_50.convertBoolean(src.getAbstractElement())); 390 if (src.hasVersion()) 391 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 392 if (src.hasCode()) 393 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 394 if (src.hasDisplay()) 395 tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 396 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 397 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 398 return tgt; 399 } 400 401 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 402 if (src == null || src.isEmpty()) 403 return null; 404 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent(); 405 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 406 if (src.hasSystem()) 407 tgt.setSystemElement(Uri14_50.convertUri(src.getSystemElement())); 408 if (src.hasAbstract()) 409 tgt.setAbstractElement(Boolean14_50.convertBoolean(src.getAbstractElement())); 410 if (src.hasVersion()) 411 tgt.setVersionElement(String14_50.convertString(src.getVersionElement())); 412 if (src.hasCode()) 413 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 414 if (src.hasDisplay()) 415 tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 416 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 417 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 418 return tgt; 419 } 420 421 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 422 if (src == null || src.isEmpty()) 423 return null; 424 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent(); 425 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 426 if (src.hasNameElement()) 427 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 428 if (src.hasValue()) 429 tgt.setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getValue())); 430 return tgt; 431 } 432 433 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 434 if (src == null || src.isEmpty()) 435 return null; 436 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent(); 437 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyBackboneElement(src,tgt); 438 if (src.hasNameElement()) 439 tgt.setNameElement(String14_50.convertString(src.getNameElement())); 440 if (src.hasValue()) 441 tgt.setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getValue())); 442 return tgt; 443 } 444}