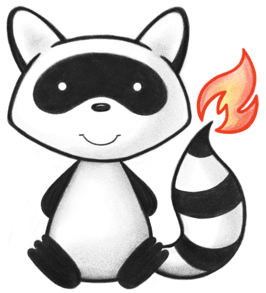
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 005import org.hl7.fhir.exceptions.FHIRException; 006 007public class Contributor30_40 { 008 public static org.hl7.fhir.r4.model.Contributor convertContributor(org.hl7.fhir.dstu3.model.Contributor src) throws FHIRException { 009 if (src == null) return null; 010 org.hl7.fhir.r4.model.Contributor tgt = new org.hl7.fhir.r4.model.Contributor(); 011 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 012 if (src.hasType()) tgt.setTypeElement(convertContributorType(src.getTypeElement())); 013 if (src.hasName()) tgt.setNameElement(String30_40.convertString(src.getNameElement())); 014 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 015 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.dstu3.model.Contributor convertContributor(org.hl7.fhir.r4.model.Contributor src) throws FHIRException { 020 if (src == null) return null; 021 org.hl7.fhir.dstu3.model.Contributor tgt = new org.hl7.fhir.dstu3.model.Contributor(); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 023 if (src.hasType()) tgt.setTypeElement(convertContributorType(src.getTypeElement())); 024 if (src.hasName()) tgt.setNameElement(String30_40.convertString(src.getNameElement())); 025 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 026 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 027 return tgt; 028 } 029 030 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Contributor.ContributorType> convertContributorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Contributor.ContributorType> src) throws FHIRException { 031 if (src == null || src.isEmpty()) return null; 032 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Contributor.ContributorType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Contributor.ContributorTypeEnumFactory()); 033 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 034 if (src.getValue() == null) { 035 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.NULL); 036 } else { 037 switch (src.getValue()) { 038 case AUTHOR: 039 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.AUTHOR); 040 break; 041 case EDITOR: 042 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.EDITOR); 043 break; 044 case REVIEWER: 045 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.REVIEWER); 046 break; 047 case ENDORSER: 048 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.ENDORSER); 049 break; 050 default: 051 tgt.setValue(org.hl7.fhir.r4.model.Contributor.ContributorType.NULL); 052 break; 053 } 054 } 055 return tgt; 056 } 057 058 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Contributor.ContributorType> convertContributorType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Contributor.ContributorType> src) throws FHIRException { 059 if (src == null || src.isEmpty()) return null; 060 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Contributor.ContributorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Contributor.ContributorTypeEnumFactory()); 061 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 062 if (src.getValue() == null) { 063 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.NULL); 064 } else { 065 switch (src.getValue()) { 066 case AUTHOR: 067 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.AUTHOR); 068 break; 069 case EDITOR: 070 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.EDITOR); 071 break; 072 case REVIEWER: 073 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.REVIEWER); 074 break; 075 case ENDORSER: 076 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.ENDORSER); 077 break; 078 default: 079 tgt.setValue(org.hl7.fhir.dstu3.model.Contributor.ContributorType.NULL); 080 break; 081 } 082 } 083 return tgt; 084 } 085}