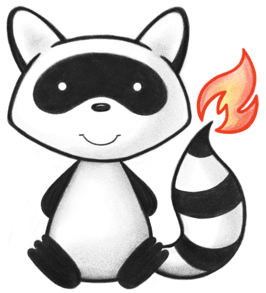
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40; 002 003import java.util.List; 004import java.util.stream.Collectors; 005 006import org.hl7.fhir.convertors.VersionConvertorConstants; 007import org.hl7.fhir.convertors.context.ConversionContext30_40; 008import org.hl7.fhir.convertors.conv30_40.VersionConvertor_30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Id30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.UnsignedInt30_40; 017import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 018import org.hl7.fhir.convertors.conv30_40.resources30_40.Enumerations30_40; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r4.model.ElementDefinition; 021import org.hl7.fhir.r4.model.Type; 022import org.hl7.fhir.utilities.Utilities; 023 024public class ElementDefinition30_40 { 025 public static org.hl7.fhir.r4.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu3.model.ElementDefinition src) throws FHIRException { 026 if (src == null) return null; 027 org.hl7.fhir.r4.model.ElementDefinition tgt = new org.hl7.fhir.r4.model.ElementDefinition(); 028 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 029 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 030 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition30_40::convertPropertyRepresentation).collect(Collectors.toList())); 031 if (src.hasSliceName()) tgt.setSliceNameElement(String30_40.convertString(src.getSliceNameElement())); 032 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 033 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 034 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 035 if (src.hasShort()) tgt.setShortElement(String30_40.convertString(src.getShortElement())); 036 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown30_40.convertMarkdown(src.getDefinitionElement())); 037 if (src.hasComment()) tgt.setCommentElement(MarkDown30_40.convertMarkdown(src.getCommentElement())); 038 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown30_40.convertMarkdown(src.getRequirementsElement())); 039 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 040 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_40.convertUnsignedInt(src.getMinElement())); 041 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 042 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 043 if (src.hasContentReference()) 044 tgt.setContentReferenceElement(Uri30_40.convertUri(src.getContentReferenceElement())); 045 for (org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent t : src.getType()) 046 convertTypeRefComponent(t, tgt.getType()); 047 if (src.hasDefaultValue()) 048 tgt.setDefaultValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDefaultValue())); 049 if (src.hasMeaningWhenMissing()) 050 tgt.setMeaningWhenMissingElement(MarkDown30_40.convertMarkdown(src.getMeaningWhenMissingElement())); 051 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String30_40.convertString(src.getOrderMeaningElement())); 052 if (src.hasFixed()) 053 tgt.setFixed(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getFixed())); 054 if (src.hasPattern()) 055 tgt.setPattern(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getPattern())); 056 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 057 tgt.addExample(convertElementDefinitionExampleComponent(t)); 058 if (src.hasMinValue()) 059 tgt.setMinValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMinValue())); 060 if (src.hasMaxValue()) 061 tgt.setMaxValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMaxValue())); 062 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer30_40.convertInteger(src.getMaxLengthElement())); 063 for (org.hl7.fhir.dstu3.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 064 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 065 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 066 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean30_40.convertBoolean(src.getMustSupportElement())); 067 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean30_40.convertBoolean(src.getIsModifierElement())); 068 if (tgt.getIsModifier()) { 069 String reason = org.hl7.fhir.dstu3.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_MODIFIER_REASON_EXTENSION); 070 if (Utilities.noString(reason)) reason = VersionConvertorConstants.EXT_MODIFIER_REASON_LEGACY; 071 tgt.setIsModifierReason(reason); 072 } 073 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean30_40.convertBoolean(src.getIsSummaryElement())); 074 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 075 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 076 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.dstu3.model.ElementDefinition convertElementDefinition(org.hl7.fhir.r4.model.ElementDefinition src) throws FHIRException { 081 if (src == null) return null; 082 org.hl7.fhir.dstu3.model.ElementDefinition tgt = new org.hl7.fhir.dstu3.model.ElementDefinition(); 083 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 084 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 085 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition30_40::convertPropertyRepresentation).collect(Collectors.toList())); 086 if (src.hasSliceName()) tgt.setSliceNameElement(String30_40.convertString(src.getSliceNameElement())); 087 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 088 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 089 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 090 if (src.hasShort()) tgt.setShortElement(String30_40.convertString(src.getShortElement())); 091 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown30_40.convertMarkdown(src.getDefinitionElement())); 092 if (src.hasComment()) tgt.setCommentElement(MarkDown30_40.convertMarkdown(src.getCommentElement())); 093 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown30_40.convertMarkdown(src.getRequirementsElement())); 094 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 095 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_40.convertUnsignedInt(src.getMinElement())); 096 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 097 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 098 if (src.hasContentReference()) 099 tgt.setContentReferenceElement(Uri30_40.convertUri(src.getContentReferenceElement())); 100 for (org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent t : src.getType()) 101 convertTypeRefComponent(t, tgt.getType()); 102 if (src.hasDefaultValue()) 103 tgt.setDefaultValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDefaultValue())); 104 if (src.hasMeaningWhenMissing()) 105 tgt.setMeaningWhenMissingElement(MarkDown30_40.convertMarkdown(src.getMeaningWhenMissingElement())); 106 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String30_40.convertString(src.getOrderMeaningElement())); 107 if (src.hasFixed()) 108 tgt.setFixed(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getFixed())); 109 if (src.hasPattern()) 110 tgt.setPattern(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getPattern())); 111 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 112 tgt.addExample(convertElementDefinitionExampleComponent(t)); 113 if (src.hasMinValue()) 114 tgt.setMinValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMinValue())); 115 if (src.hasMaxValue()) 116 tgt.setMaxValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMaxValue())); 117 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer30_40.convertInteger(src.getMaxLengthElement())); 118 for (org.hl7.fhir.r4.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 119 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 120 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 121 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean30_40.convertBoolean(src.getMustSupportElement())); 122 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean30_40.convertBoolean(src.getIsModifierElement())); 123 if (src.hasIsModifierReason() && !VersionConvertorConstants.EXT_MODIFIER_REASON_LEGACY.equals(src.getIsModifierReason())) 124 org.hl7.fhir.dstu3.utils.ToolingExtensions.setStringExtension(tgt, VersionConvertorConstants.EXT_MODIFIER_REASON_EXTENSION, src.getIsModifierReason()); 125 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean30_40.convertBoolean(src.getIsSummaryElement())); 126 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 127 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 128 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 133 if (src == null || src.isEmpty()) return null; 134 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentationEnumFactory()); 135 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 136 if (src.getValue() == null) { 137 tgt.setValue(null); 138} else { 139 switch(src.getValue()) { 140 case XMLATTR: 141 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLATTR); 142 break; 143 case XMLTEXT: 144 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLTEXT); 145 break; 146 case TYPEATTR: 147 tgt.setValue(ElementDefinition.PropertyRepresentation.TYPEATTR); 148 break; 149 case CDATEXT: 150 tgt.setValue(ElementDefinition.PropertyRepresentation.CDATEXT); 151 break; 152 case XHTML: 153 tgt.setValue(ElementDefinition.PropertyRepresentation.XHTML); 154 break; 155 default: 156 tgt.setValue(ElementDefinition.PropertyRepresentation.NULL); 157 break; 158 } 159} 160 return tgt; 161 } 162 163 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 164 if (src == null || src.isEmpty()) return null; 165 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentationEnumFactory()); 166 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 167 if (src.getValue() == null) { 168 tgt.setValue(null); 169} else { 170 switch(src.getValue()) { 171 case XMLATTR: 172 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLATTR); 173 break; 174 case XMLTEXT: 175 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 176 break; 177 case TYPEATTR: 178 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 179 break; 180 case CDATEXT: 181 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.CDATEXT); 182 break; 183 case XHTML: 184 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XHTML); 185 break; 186 default: 187 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.NULL); 188 break; 189 } 190} 191 return tgt; 192 } 193 194 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 195 if (src == null) return null; 196 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent(); 197 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 198 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 199 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 200 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 201 if (src.hasOrdered()) tgt.setOrderedElement(Boolean30_40.convertBoolean(src.getOrderedElement())); 202 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 203 return tgt; 204 } 205 206 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 207 if (src == null) return null; 208 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent(); 209 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 210 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 211 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 212 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 213 if (src.hasOrdered()) tgt.setOrderedElement(Boolean30_40.convertBoolean(src.getOrderedElement())); 214 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 215 return tgt; 216 } 217 218 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> src) throws FHIRException { 219 if (src == null || src.isEmpty()) return null; 220 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.SlicingRulesEnumFactory()); 221 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 222 if (src.getValue() == null) { 223 tgt.setValue(null); 224} else { 225 switch(src.getValue()) { 226 case CLOSED: 227 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 228 break; 229 case OPEN: 230 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 231 break; 232 case OPENATEND: 233 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 234 break; 235 default: 236 tgt.setValue(ElementDefinition.SlicingRules.NULL); 237 break; 238 } 239} 240 return tgt; 241 } 242 243 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> src) throws FHIRException { 244 if (src == null || src.isEmpty()) return null; 245 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRulesEnumFactory()); 246 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 247 if (src.getValue() == null) { 248 tgt.setValue(null); 249} else { 250 switch(src.getValue()) { 251 case CLOSED: 252 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.CLOSED); 253 break; 254 case OPEN: 255 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.OPEN); 256 break; 257 case OPENATEND: 258 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.OPENATEND); 259 break; 260 default: 261 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.NULL); 262 break; 263 } 264} 265 return tgt; 266 } 267 268 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 269 if (src == null) return null; 270 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 271 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 272 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 273 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 274 return tgt; 275 } 276 277 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 278 if (src == null) return null; 279 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 280 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 281 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 282 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 283 return tgt; 284 } 285 286 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 287 if (src == null || src.isEmpty()) return null; 288 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 289 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 290 if (src.getValue() == null) { 291 tgt.setValue(null); 292} else { 293 switch(src.getValue()) { 294 case VALUE: 295 tgt.setValue(ElementDefinition.DiscriminatorType.VALUE); 296 break; 297 case EXISTS: 298 tgt.setValue(ElementDefinition.DiscriminatorType.EXISTS); 299 break; 300 case PATTERN: 301 tgt.setValue(ElementDefinition.DiscriminatorType.PATTERN); 302 break; 303 case TYPE: 304 tgt.setValue(ElementDefinition.DiscriminatorType.TYPE); 305 break; 306 case PROFILE: 307 tgt.setValue(ElementDefinition.DiscriminatorType.PROFILE); 308 break; 309 default: 310 tgt.setValue(ElementDefinition.DiscriminatorType.NULL); 311 break; 312 } 313} 314 return tgt; 315 } 316 317 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 318 if (src == null || src.isEmpty()) return null; 319 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 320 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 321 if (src.getValue() == null) { 322 tgt.setValue(null); 323} else { 324 switch(src.getValue()) { 325 case VALUE: 326 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.VALUE); 327 break; 328 case EXISTS: 329 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.EXISTS); 330 break; 331 case PATTERN: 332 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.PATTERN); 333 break; 334 case TYPE: 335 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.TYPE); 336 break; 337 case PROFILE: 338 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.PROFILE); 339 break; 340 default: 341 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.NULL); 342 break; 343 } 344} 345 return tgt; 346 } 347 348 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 349 if (src == null) return null; 350 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent(); 351 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 352 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 353 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_40.convertUnsignedInt(src.getMinElement())); 354 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 355 return tgt; 356 } 357 358 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 359 if (src == null) return null; 360 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent(); 361 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 362 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 363 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_40.convertUnsignedInt(src.getMinElement())); 364 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 365 return tgt; 366 } 367 368 public static void convertTypeRefComponent(org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent src, List<ElementDefinition.TypeRefComponent> list) throws FHIRException { 369 if (src == null) return; 370 ElementDefinition.TypeRefComponent tgt = null; 371 for (ElementDefinition.TypeRefComponent t : list) 372 if (t.getCode().equals(src.getCode())) tgt = t; 373 if (tgt == null) { 374 tgt = new ElementDefinition.TypeRefComponent(); 375 list.add(tgt); 376 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 377 tgt.setCodeElement(Uri30_40.convertUri(src.getCodeElement())); 378 } 379 if (src.hasProfile()) tgt.addProfile(src.getProfile()); 380 if (src.hasTargetProfile()) tgt.addTargetProfile(src.getTargetProfile()); 381 for (org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> t : src.getAggregation()) { 382 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> a = convertAggregationMode(t); 383 if (!tgt.hasAggregation(a.getValue())) 384 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(t, tgt.addAggregation(a.getValue())); 385 } 386 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 387 } 388 389 public static void convertTypeRefComponent(ElementDefinition.TypeRefComponent src, List<org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent> list) throws FHIRException { 390 if (src == null) return; 391 org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 392 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 393 tgt.setCodeElement(Uri30_40.convertUri(src.getCodeElement())); 394 list.add(tgt); 395 if (src.hasTarget()) { 396 if (src.hasProfile()) tgt.setProfile(src.getProfile().get(0).getValue()); 397 for (org.hl7.fhir.r4.model.UriType u : src.getTargetProfile()) { 398 if (tgt.hasTargetProfile()) { 399 tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 400 list.add(tgt); 401 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 402 tgt.setCode(src.getCode()); 403 if (src.hasProfile()) tgt.setProfile(src.getProfile().get(0).getValue()); 404 } 405 tgt.setTargetProfile(u.getValue()); 406 } 407 } else { 408 for (org.hl7.fhir.r4.model.UriType u : src.getProfile()) { 409 if (tgt.hasProfile()) { 410 tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 411 list.add(tgt); 412 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 413 tgt.setCode(src.getCode()); 414 } 415 tgt.setProfile(u.getValue()); 416 } 417 } 418 } 419 420 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> src) throws FHIRException { 421 if (src == null || src.isEmpty()) return null; 422 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 423 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 424 if (src.getValue() == null) { 425 tgt.setValue(null); 426} else { 427 switch(src.getValue()) { 428 case CONTAINED: 429 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 430 break; 431 case REFERENCED: 432 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 433 break; 434 case BUNDLED: 435 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 436 break; 437 default: 438 tgt.setValue(ElementDefinition.AggregationMode.NULL); 439 break; 440 } 441} 442 return tgt; 443 } 444 445 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 446 if (src == null || src.isEmpty()) return null; 447 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.AggregationModeEnumFactory()); 448 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 449 if (src.getValue() == null) { 450 tgt.setValue(null); 451} else { 452 switch(src.getValue()) { 453 case CONTAINED: 454 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.CONTAINED); 455 break; 456 case REFERENCED: 457 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.REFERENCED); 458 break; 459 case BUNDLED: 460 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.BUNDLED); 461 break; 462 default: 463 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.NULL); 464 break; 465 } 466} 467 return tgt; 468 } 469 470 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 471 if (src == null || src.isEmpty()) return null; 472 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.ReferenceVersionRulesEnumFactory()); 473 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 474 if (src.getValue() == null) { 475 tgt.setValue(null); 476} else { 477 switch(src.getValue()) { 478 case EITHER: 479 tgt.setValue(ElementDefinition.ReferenceVersionRules.EITHER); 480 break; 481 case INDEPENDENT: 482 tgt.setValue(ElementDefinition.ReferenceVersionRules.INDEPENDENT); 483 break; 484 case SPECIFIC: 485 tgt.setValue(ElementDefinition.ReferenceVersionRules.SPECIFIC); 486 break; 487 default: 488 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 489 break; 490 } 491} 492 return tgt; 493 } 494 495 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 496 if (src == null || src.isEmpty()) return null; 497 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 498 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 499 if (src.getValue() == null) { 500 tgt.setValue(null); 501} else { 502 switch(src.getValue()) { 503 case EITHER: 504 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.EITHER); 505 break; 506 case INDEPENDENT: 507 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.INDEPENDENT); 508 break; 509 case SPECIFIC: 510 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.SPECIFIC); 511 break; 512 default: 513 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.NULL); 514 break; 515 } 516} 517 return tgt; 518 } 519 520 public static ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 521 if (src == null) return null; 522 ElementDefinition.ElementDefinitionExampleComponent tgt = new ElementDefinition.ElementDefinitionExampleComponent(); 523 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 524 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 525 if (src.hasValue()) 526 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 527 return tgt; 528 } 529 530 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 531 if (src == null) return null; 532 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent(); 533 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 534 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 535 if (src.hasValue()) 536 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 537 return tgt; 538 } 539 540 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 541 if (src == null) return null; 542 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 543 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 544 if (src.hasKey()) tgt.setKeyElement(Id30_40.convertId(src.getKeyElement())); 545 if (src.hasRequirements()) tgt.setRequirementsElement(String30_40.convertString(src.getRequirementsElement())); 546 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 547 if (src.hasHuman()) tgt.setHumanElement(String30_40.convertString(src.getHumanElement())); 548 if (src.hasExpression()) tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 549 if (src.hasXpath()) tgt.setXpathElement(String30_40.convertString(src.getXpathElement())); 550 if (src.hasSource()) tgt.setSource(src.getSource()); 551 return tgt; 552 } 553 554 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 555 if (src == null) return null; 556 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent(); 557 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 558 if (src.hasKey()) tgt.setKeyElement(Id30_40.convertId(src.getKeyElement())); 559 if (src.hasRequirements()) tgt.setRequirementsElement(String30_40.convertString(src.getRequirementsElement())); 560 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 561 if (src.hasHuman()) tgt.setHumanElement(String30_40.convertString(src.getHumanElement())); 562 if (src.hasExpression()) tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 563 if (src.hasXpath()) tgt.setXpathElement(String30_40.convertString(src.getXpathElement())); 564 if (src.hasSource()) tgt.setSource(src.getSource()); 565 return tgt; 566 } 567 568 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 569 if (src == null || src.isEmpty()) return null; 570 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 571 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 572 if (src.getValue() == null) { 573 tgt.setValue(null); 574} else { 575 switch(src.getValue()) { 576 case ERROR: 577 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 578 break; 579 case WARNING: 580 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 581 break; 582 default: 583 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 584 break; 585 } 586} 587 return tgt; 588 } 589 590 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 591 if (src == null || src.isEmpty()) return null; 592 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverityEnumFactory()); 593 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 594 if (src.getValue() == null) { 595 tgt.setValue(null); 596} else { 597 switch(src.getValue()) { 598 case ERROR: 599 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.ERROR); 600 break; 601 case WARNING: 602 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.WARNING); 603 break; 604 default: 605 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.NULL); 606 break; 607 } 608} 609 return tgt; 610 } 611 612 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 613 if (src == null) return null; 614 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 615 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt, VersionConvertor_30_40.EXT_SRC_TYPE); 616 if (src.hasStrength()) tgt.setStrengthElement(Enumerations30_40.convertBindingStrength(src.getStrengthElement())); 617 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 618 if (src.hasValueSet()) { 619 Type t = ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValueSet()); 620 if (t instanceof org.hl7.fhir.r4.model.Reference) { 621 tgt.setValueSet(((org.hl7.fhir.r4.model.Reference) t).getReference()); 622 tgt.getValueSetElement().addExtension(VersionConvertor_30_40.EXT_SRC_TYPE, new org.hl7.fhir.r4.model.UrlType("Reference")); 623 } else { 624 tgt.setValueSet(t.primitiveValue()); 625 tgt.getValueSetElement().addExtension(VersionConvertor_30_40.EXT_SRC_TYPE, new org.hl7.fhir.r4.model.UrlType("uri")); 626 } 627 tgt.setValueSet(VersionConvertorConstants.refToVS(tgt.getValueSet())); 628 } 629 return tgt; 630 } 631 632 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 633 if (src == null) return null; 634 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent(); 635 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt, VersionConvertor_30_40.EXT_SRC_TYPE); 636 if (src.hasStrength()) tgt.setStrengthElement(Enumerations30_40.convertBindingStrength(src.getStrengthElement())); 637 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 638 if (src.hasValueSet()) { 639 org.hl7.fhir.r4.model.Extension ex = src.getValueSetElement().getExtensionByUrl(VersionConvertor_30_40.EXT_SRC_TYPE); 640 String vsr = VersionConvertorConstants.vsToRef(src.getValueSet()); 641 if (ex != null) { 642 if ("uri".equals(ex.getValue().primitiveValue())) { 643 tgt.setValueSet(new org.hl7.fhir.dstu3.model.UriType(vsr == null ? src.getValueSet() : vsr)); 644 } else { 645 tgt.setValueSet(new org.hl7.fhir.dstu3.model.Reference(src.getValueSet())); 646 } 647 } else { 648 if (vsr != null) tgt.setValueSet(new org.hl7.fhir.dstu3.model.UriType(vsr)); 649 else tgt.setValueSet(new org.hl7.fhir.dstu3.model.Reference(src.getValueSet())); 650 } 651 } 652 return tgt; 653 } 654 655 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 656 if (src == null) return null; 657 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 658 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 659 if (src.hasIdentity()) tgt.setIdentityElement(Id30_40.convertId(src.getIdentityElement())); 660 if (src.hasLanguage()) tgt.setLanguageElement(Code30_40.convertCode(src.getLanguageElement())); 661 if (src.hasMap()) tgt.setMapElement(String30_40.convertString(src.getMapElement())); 662 if (src.hasComment()) tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 663 return tgt; 664 } 665 666 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 667 if (src == null) return null; 668 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent(); 669 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 670 if (src.hasIdentity()) tgt.setIdentityElement(Id30_40.convertId(src.getIdentityElement())); 671 if (src.hasLanguage()) tgt.setLanguageElement(Code30_40.convertCode(src.getLanguageElement())); 672 if (src.hasMap()) tgt.setMapElement(String30_40.convertString(src.getMapElement())); 673 if (src.hasComment()) tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 674 return tgt; 675 } 676}