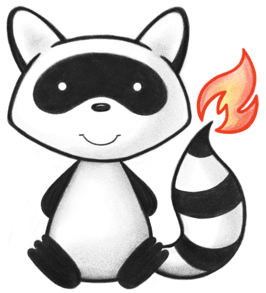
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r4.model.ParameterDefinition; 009 010public class ParameterDefinition30_40 { 011 public static org.hl7.fhir.r4.model.ParameterDefinition convertParameterDefinition(org.hl7.fhir.dstu3.model.ParameterDefinition src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r4.model.ParameterDefinition tgt = new org.hl7.fhir.r4.model.ParameterDefinition(); 014 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 015 if (src.hasName()) tgt.setNameElement(Code30_40.convertCode(src.getNameElement())); 016 if (src.hasUse()) tgt.setUseElement(convertParameterUse(src.getUseElement())); 017 if (src.hasMin()) tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 018 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 019 if (src.hasDocumentation()) tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 020 if (src.hasType()) tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 021 if (src.hasProfile()) { 022 tgt.setProfile(Reference30_40.convertReference(src.getProfile()).getReference()); 023 } 024 return tgt; 025 } 026 027 public static org.hl7.fhir.dstu3.model.ParameterDefinition convertParameterDefinition(org.hl7.fhir.r4.model.ParameterDefinition src) throws FHIRException { 028 if (src == null) return null; 029 org.hl7.fhir.dstu3.model.ParameterDefinition tgt = new org.hl7.fhir.dstu3.model.ParameterDefinition(); 030 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 031 if (src.hasName()) tgt.setNameElement(Code30_40.convertCode(src.getNameElement())); 032 if (src.hasUse()) tgt.setUseElement(convertParameterUse(src.getUseElement())); 033 if (src.hasMin()) tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 034 if (src.hasMax()) tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 035 if (src.hasDocumentation()) tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 036 if (src.hasType()) tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 037 if (src.hasProfile()) tgt.setProfile(new org.hl7.fhir.dstu3.model.Reference(src.getProfile())); 038 return tgt; 039 } 040 041 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ParameterDefinition.ParameterUse> convertParameterUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse> src) throws FHIRException { 042 if (src == null || src.isEmpty()) return null; 043 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ParameterDefinition.ParameterUse> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ParameterDefinition.ParameterUseEnumFactory()); 044 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 045 if (src.getValue() == null) { 046 tgt.setValue(null); 047} else { 048 switch(src.getValue()) { 049 case IN: 050 tgt.setValue(ParameterDefinition.ParameterUse.IN); 051 break; 052 case OUT: 053 tgt.setValue(ParameterDefinition.ParameterUse.OUT); 054 break; 055 default: 056 tgt.setValue(ParameterDefinition.ParameterUse.NULL); 057 break; 058 } 059} 060 return tgt; 061 } 062 063 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse> convertParameterUse(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ParameterDefinition.ParameterUse> src) throws FHIRException { 064 if (src == null || src.isEmpty()) return null; 065 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUseEnumFactory()); 066 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 067 if (src.getValue() == null) { 068 tgt.setValue(null); 069} else { 070 switch(src.getValue()) { 071 case IN: 072 tgt.setValue(org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse.IN); 073 break; 074 case OUT: 075 tgt.setValue(org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse.OUT); 076 break; 077 default: 078 tgt.setValue(org.hl7.fhir.dstu3.model.ParameterDefinition.ParameterUse.NULL); 079 break; 080 } 081} 082 return tgt; 083 } 084}