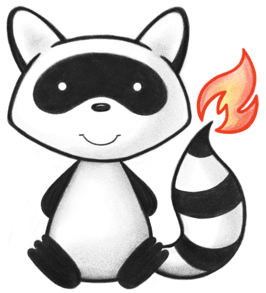
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class Reference30_40 { 009 public static org.hl7.fhir.r4.model.Reference convertReference(org.hl7.fhir.dstu3.model.Reference src) throws FHIRException { 010 if (src == null) return null; 011 org.hl7.fhir.r4.model.Reference tgt = new org.hl7.fhir.r4.model.Reference(); 012 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 013 if (src.hasReference()) tgt.setReference(src.getReference()); 014 if (src.hasIdentifier()) tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 015 if (src.hasDisplay()) tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.dstu3.model.Reference convertReference(org.hl7.fhir.r4.model.Reference src) throws FHIRException { 020 if (src == null) return null; 021 org.hl7.fhir.dstu3.model.Reference tgt = new org.hl7.fhir.dstu3.model.Reference(); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 023 if (src.hasReference()) tgt.setReference(src.getReference()); 024 if (src.hasIdentifier()) tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 025 if (src.hasDisplay()) tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 026 return tgt; 027 } 028 029 static public org.hl7.fhir.r4.model.CanonicalType convertReferenceToCanonical(org.hl7.fhir.dstu3.model.Reference src) throws FHIRException { 030 org.hl7.fhir.r4.model.CanonicalType dst = new org.hl7.fhir.r4.model.CanonicalType(src.getReference()); 031 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, dst); 032 return dst; 033 } 034 035 static public org.hl7.fhir.dstu3.model.Reference convertCanonicalToReference(org.hl7.fhir.r4.model.CanonicalType src) throws FHIRException { 036 org.hl7.fhir.dstu3.model.Reference dst = new org.hl7.fhir.dstu3.model.Reference(src.getValue()); 037 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, dst); 038 return dst; 039 } 040}