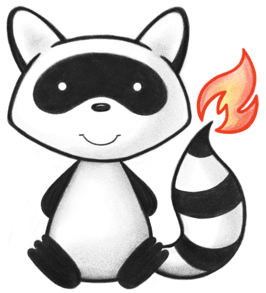
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Coding30_40 { 011 public static org.hl7.fhir.r4.model.Coding convertCoding(org.hl7.fhir.dstu3.model.Coding src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r4.model.Coding tgt = new org.hl7.fhir.r4.model.Coding(); 014 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 015 if (src.hasSystem()) tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 016 if (src.hasVersion()) tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 017 if (src.hasCode()) tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 018 if (src.hasDisplay()) tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 019 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean30_40.convertBoolean(src.getUserSelectedElement())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.r4.model.Coding convertCoding(org.hl7.fhir.dstu3.model.CodeType src) throws FHIRException { 024 if (src == null) return null; 025 org.hl7.fhir.r4.model.Coding tgt = new org.hl7.fhir.r4.model.Coding(); 026 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 027 tgt.setCode(src.getValue()); 028 return tgt; 029 } 030 031 public static org.hl7.fhir.r4.model.Coding convertCoding(org.hl7.fhir.dstu3.model.CodeableConcept src) throws FHIRException { 032 if (src == null) return null; 033 org.hl7.fhir.r4.model.Coding tgt = new org.hl7.fhir.r4.model.Coding(); 034 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 035 if (src.hasCoding()) { 036 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 037 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 038 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 039 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 040 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 041 } 042 return tgt; 043 } 044 045 public static org.hl7.fhir.dstu3.model.Coding convertCoding(org.hl7.fhir.r4.model.CodeableConcept src) throws FHIRException { 046 if (src == null) return null; 047 org.hl7.fhir.dstu3.model.Coding tgt = new org.hl7.fhir.dstu3.model.Coding(); 048 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 049 if (src.hasCoding()) { 050 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 051 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 052 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 053 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 054 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 055 } 056 return tgt; 057 } 058 059 public static org.hl7.fhir.dstu3.model.Coding convertCoding(org.hl7.fhir.r4.model.Coding src) throws FHIRException { 060 if (src == null) return null; 061 org.hl7.fhir.dstu3.model.Coding tgt = new org.hl7.fhir.dstu3.model.Coding(); 062 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 063 if (src.hasSystem()) tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 064 if (src.hasVersion()) tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 065 if (src.hasCode()) tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 066 if (src.hasDisplay()) tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 067 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean30_40.convertBoolean(src.getUserSelectedElement())); 068 return tgt; 069 } 070 071 public static String convertCoding2Uri(org.hl7.fhir.dstu3.model.Coding code) { 072 return code.getSystem() + "/" + code.getCode(); 073 } 074 075 public static org.hl7.fhir.dstu3.model.Coding convertUri2Coding(String uri) { 076 int i = uri.lastIndexOf("/"); 077 return new org.hl7.fhir.dstu3.model.Coding().setSystem(uri.substring(0, i)).setCode(uri.substring(i + 1)); 078 } 079}