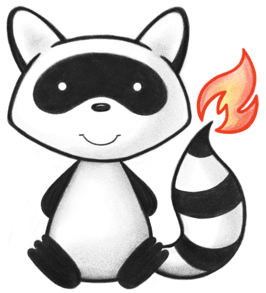
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class ContactPoint30_40 { 009 public static org.hl7.fhir.r4.model.ContactPoint convertContactPoint(org.hl7.fhir.dstu3.model.ContactPoint src) throws FHIRException { 010 if (src == null) return null; 011 org.hl7.fhir.r4.model.ContactPoint tgt = new org.hl7.fhir.r4.model.ContactPoint(); 012 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 013 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 014 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 015 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 016 if (src.hasRank()) tgt.setRankElement(PositiveInt30_40.convertPositiveInt(src.getRankElement())); 017 if (src.hasPeriod()) tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu3.model.ContactPoint convertContactPoint(org.hl7.fhir.r4.model.ContactPoint src) throws FHIRException { 022 if (src == null) return null; 023 org.hl7.fhir.dstu3.model.ContactPoint tgt = new org.hl7.fhir.dstu3.model.ContactPoint(); 024 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 025 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 026 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 027 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 028 if (src.hasRank()) tgt.setRankElement(PositiveInt30_40.convertPositiveInt(src.getRankElement())); 029 if (src.hasPeriod()) tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 030 return tgt; 031 } 032 033 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 034 if (src == null || src.isEmpty()) return null; 035 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ContactPoint.ContactPointSystemEnumFactory()); 036 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 037 if (src.getValue() == null) { 038 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.NULL); 039 } else { 040 switch (src.getValue()) { 041 case PHONE: 042 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.PHONE); 043 break; 044 case FAX: 045 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.FAX); 046 break; 047 case EMAIL: 048 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.EMAIL); 049 break; 050 case PAGER: 051 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.PAGER); 052 break; 053 case URL: 054 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.URL); 055 break; 056 case SMS: 057 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.SMS); 058 break; 059 case OTHER: 060 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.OTHER); 061 break; 062 default: 063 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem.NULL); 064 break; 065 } 066 } 067 return tgt; 068 } 069 070 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 071 if (src == null || src.isEmpty()) return null; 072 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystemEnumFactory()); 073 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 074 if (src.getValue() == null) { 075 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.NULL); 076 } else { 077 switch (src.getValue()) { 078 case PHONE: 079 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.PHONE); 080 break; 081 case FAX: 082 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.FAX); 083 break; 084 case EMAIL: 085 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.EMAIL); 086 break; 087 case PAGER: 088 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.PAGER); 089 break; 090 case URL: 091 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.URL); 092 break; 093 case SMS: 094 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.SMS); 095 break; 096 case OTHER: 097 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.OTHER); 098 break; 099 default: 100 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.NULL); 101 break; 102 } 103 } 104 return tgt; 105 } 106 107 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> src) throws FHIRException { 108 if (src == null || src.isEmpty()) return null; 109 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ContactPoint.ContactPointUseEnumFactory()); 110 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 111 if (src.getValue() == null) { 112 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.NULL); 113 } else { 114 switch (src.getValue()) { 115 case HOME: 116 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.HOME); 117 break; 118 case WORK: 119 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.WORK); 120 break; 121 case TEMP: 122 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.TEMP); 123 break; 124 case OLD: 125 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.OLD); 126 break; 127 case MOBILE: 128 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.MOBILE); 129 break; 130 default: 131 tgt.setValue(org.hl7.fhir.r4.model.ContactPoint.ContactPointUse.NULL); 132 break; 133 } 134 } 135 return tgt; 136 } 137 138 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ContactPoint.ContactPointUse> src) throws FHIRException { 139 if (src == null || src.isEmpty()) return null; 140 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUseEnumFactory()); 141 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 142 if (src.getValue() == null) { 143 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.NULL); 144 } else { 145 switch (src.getValue()) { 146 case HOME: 147 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.HOME); 148 break; 149 case WORK: 150 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.WORK); 151 break; 152 case TEMP: 153 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.TEMP); 154 break; 155 case OLD: 156 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.OLD); 157 break; 158 case MOBILE: 159 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.MOBILE); 160 break; 161 default: 162 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.NULL); 163 break; 164 } 165 } 166 return tgt; 167 } 168}