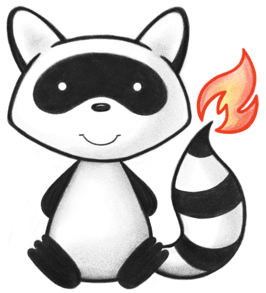
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.r4.model.Quantity; 010 011public class Quantity30_40 { 012 public static void copyQuantity(org.hl7.fhir.dstu3.model.Quantity src, org.hl7.fhir.r4.model.Quantity tgt) throws FHIRException { 013 if (src == null || tgt == null) return; 014 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 015 if (src.hasValue()) tgt.setValue(src.getValue()); 016 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 017 if (src.hasUnit()) tgt.setUnit(src.getUnit()); 018 if (src.hasSystem()) tgt.setSystem(src.getSystem()); 019 if (src.hasCode()) tgt.setCode(src.getCode()); 020 } 021 022 public static void copyQuantity(org.hl7.fhir.r4.model.Quantity src, org.hl7.fhir.dstu3.model.Quantity tgt) throws FHIRException { 023 if (src == null || tgt == null) return; 024 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 025 if (src.hasValue()) tgt.setValue(src.getValue()); 026 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 027 if (src.hasUnit()) tgt.setUnit(src.getUnit()); 028 if (src.hasSystem()) tgt.setSystem(src.getSystem()); 029 if (src.hasCode()) tgt.setCode(src.getCode()); 030 } 031 032 public static org.hl7.fhir.r4.model.Quantity convertQuantity(org.hl7.fhir.dstu3.model.Quantity src) throws FHIRException { 033 if (src == null) return null; 034 org.hl7.fhir.r4.model.Quantity tgt = new org.hl7.fhir.r4.model.Quantity(); 035 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 036 if (src.hasValue()) tgt.setValueElement(Decimal30_40.convertDecimal(src.getValueElement())); 037 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 038 if (src.hasUnit()) tgt.setUnitElement(String30_40.convertString(src.getUnitElement())); 039 if (src.hasSystem()) tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 040 if (src.hasCode()) tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 041 return tgt; 042 } 043 044 public static org.hl7.fhir.dstu3.model.Quantity convertQuantity(org.hl7.fhir.r4.model.Quantity src) throws FHIRException { 045 if (src == null) return null; 046 org.hl7.fhir.dstu3.model.Quantity tgt = new org.hl7.fhir.dstu3.model.Quantity(); 047 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 048 if (src.hasValue()) tgt.setValueElement(Decimal30_40.convertDecimal(src.getValueElement())); 049 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 050 if (src.hasUnit()) tgt.setUnitElement(String30_40.convertString(src.getUnitElement())); 051 if (src.hasSystem()) tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 052 if (src.hasCode()) tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 053 return tgt; 054 } 055 056 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Quantity.QuantityComparator> convertQuantityComparator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> src) throws FHIRException { 057 if (src == null || src.isEmpty()) return null; 058 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Quantity.QuantityComparator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Quantity.QuantityComparatorEnumFactory()); 059 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 060 if (src.getValue() == null) { 061 tgt.setValue(null); 062} else { 063 switch(src.getValue()) { 064 case LESS_THAN: 065 tgt.setValue(Quantity.QuantityComparator.LESS_THAN); 066 break; 067 case LESS_OR_EQUAL: 068 tgt.setValue(Quantity.QuantityComparator.LESS_OR_EQUAL); 069 break; 070 case GREATER_OR_EQUAL: 071 tgt.setValue(Quantity.QuantityComparator.GREATER_OR_EQUAL); 072 break; 073 case GREATER_THAN: 074 tgt.setValue(Quantity.QuantityComparator.GREATER_THAN); 075 break; 076 default: 077 tgt.setValue(Quantity.QuantityComparator.NULL); 078 break; 079 } 080} 081 return tgt; 082 } 083 084 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> convertQuantityComparator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Quantity.QuantityComparator> src) throws FHIRException { 085 if (src == null || src.isEmpty()) return null; 086 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Quantity.QuantityComparatorEnumFactory()); 087 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 088 if (src.getValue() == null) { 089 tgt.setValue(null); 090} else { 091 switch(src.getValue()) { 092 case LESS_THAN: 093 tgt.setValue(org.hl7.fhir.dstu3.model.Quantity.QuantityComparator.LESS_THAN); 094 break; 095 case LESS_OR_EQUAL: 096 tgt.setValue(org.hl7.fhir.dstu3.model.Quantity.QuantityComparator.LESS_OR_EQUAL); 097 break; 098 case GREATER_OR_EQUAL: 099 tgt.setValue(org.hl7.fhir.dstu3.model.Quantity.QuantityComparator.GREATER_OR_EQUAL); 100 break; 101 case GREATER_THAN: 102 tgt.setValue(org.hl7.fhir.dstu3.model.Quantity.QuantityComparator.GREATER_THAN); 103 break; 104 default: 105 tgt.setValue(org.hl7.fhir.dstu3.model.Quantity.QuantityComparator.NULL); 106 break; 107 } 108} 109 return tgt; 110 } 111}