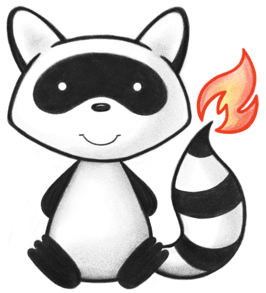
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class SampledData30_40 { 010 public static org.hl7.fhir.r4.model.SampledData convertSampledData(org.hl7.fhir.dstu3.model.SampledData src) throws FHIRException { 011 if (src == null) return null; 012 org.hl7.fhir.r4.model.SampledData tgt = new org.hl7.fhir.r4.model.SampledData(); 013 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 014 if (src.hasOrigin()) tgt.setOrigin(SimpleQuantity30_40.convertSimpleQuantity(src.getOrigin())); 015 if (src.hasPeriod()) tgt.setPeriodElement(Decimal30_40.convertDecimal(src.getPeriodElement())); 016 if (src.hasFactor()) tgt.setFactorElement(Decimal30_40.convertDecimal(src.getFactorElement())); 017 if (src.hasLowerLimit()) tgt.setLowerLimitElement(Decimal30_40.convertDecimal(src.getLowerLimitElement())); 018 if (src.hasUpperLimit()) tgt.setUpperLimitElement(Decimal30_40.convertDecimal(src.getUpperLimitElement())); 019 if (src.hasDimensions()) tgt.setDimensionsElement(PositiveInt30_40.convertPositiveInt(src.getDimensionsElement())); 020 if (src.hasData()) tgt.setDataElement(String30_40.convertString(src.getDataElement())); 021 return tgt; 022 } 023 024 public static org.hl7.fhir.dstu3.model.SampledData convertSampledData(org.hl7.fhir.r4.model.SampledData src) throws FHIRException { 025 if (src == null) return null; 026 org.hl7.fhir.dstu3.model.SampledData tgt = new org.hl7.fhir.dstu3.model.SampledData(); 027 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 028 if (src.hasOrigin()) tgt.setOrigin(SimpleQuantity30_40.convertSimpleQuantity(src.getOrigin())); 029 if (src.hasPeriod()) tgt.setPeriodElement(Decimal30_40.convertDecimal(src.getPeriodElement())); 030 if (src.hasFactor()) tgt.setFactorElement(Decimal30_40.convertDecimal(src.getFactorElement())); 031 if (src.hasLowerLimit()) tgt.setLowerLimitElement(Decimal30_40.convertDecimal(src.getLowerLimitElement())); 032 if (src.hasUpperLimit()) tgt.setUpperLimitElement(Decimal30_40.convertDecimal(src.getUpperLimitElement())); 033 if (src.hasDimensions()) tgt.setDimensionsElement(PositiveInt30_40.convertPositiveInt(src.getDimensionsElement())); 034 if (src.hasData()) tgt.setDataElement(String30_40.convertString(src.getDataElement())); 035 return tgt; 036 } 037}