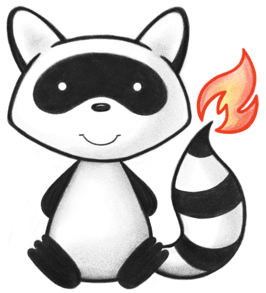
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003 004import org.hl7.fhir.convertors.context.ConversionContext30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.Account; 014import org.hl7.fhir.r4.model.Enumeration; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class Account30_40 { 046 047 public static org.hl7.fhir.r4.model.Account convertAccount(org.hl7.fhir.dstu3.model.Account src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r4.model.Account tgt = new org.hl7.fhir.r4.model.Account(); 051 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 054 if (src.hasStatus()) 055 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 056 if (src.hasType()) 057 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 058 if (src.hasName()) 059 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 060 if (src.hasSubject()) { 061 tgt.addSubject(Reference30_40.convertReference(src.getSubject())); 062 } 063 if (src.hasPeriod()) 064 tgt.setServicePeriod(Period30_40.convertPeriod(src.getPeriod())); 065 for (org.hl7.fhir.dstu3.model.Account.CoverageComponent t : src.getCoverage()) 066 tgt.addCoverage(convertCoverageComponent(t)); 067 if (src.hasOwner()) 068 tgt.setOwner(Reference30_40.convertReference(src.getOwner())); 069 if (src.hasDescription()) 070 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 071 for (org.hl7.fhir.dstu3.model.Account.GuarantorComponent t : src.getGuarantor()) 072 tgt.addGuarantor(convertGuarantorComponent(t)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.Account convertAccount(org.hl7.fhir.r4.model.Account src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.dstu3.model.Account tgt = new org.hl7.fhir.dstu3.model.Account(); 080 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 081 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 082 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 083 if (src.hasStatus()) 084 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 085 if (src.hasType()) 086 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 087 if (src.hasName()) 088 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 089 if (src.getSubject().size() > 0) { 090 tgt.setSubject(Reference30_40.convertReference(src.getSubjectFirstRep())); 091 } 092 if (src.hasServicePeriod()) 093 tgt.setPeriod(Period30_40.convertPeriod(src.getServicePeriod())); 094 for (org.hl7.fhir.r4.model.Account.CoverageComponent t : src.getCoverage()) 095 tgt.addCoverage(convertCoverageComponent(t)); 096 if (src.hasOwner()) 097 tgt.setOwner(Reference30_40.convertReference(src.getOwner())); 098 if (src.hasDescription()) 099 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 100 for (org.hl7.fhir.r4.model.Account.GuarantorComponent t : src.getGuarantor()) 101 tgt.addGuarantor(convertGuarantorComponent(t)); 102 return tgt; 103 } 104 105 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Account.AccountStatus> src) throws FHIRException { 106 if (src == null || src.isEmpty()) 107 return null; 108 Enumeration<Account.AccountStatus> tgt = new Enumeration<>(new Account.AccountStatusEnumFactory()); 109 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 110 if (src.getValue() == null) { 111 tgt.setValue(null); 112 } else { 113 switch (src.getValue()) { 114 case ACTIVE: 115 tgt.setValue(Account.AccountStatus.ACTIVE); 116 break; 117 case INACTIVE: 118 tgt.setValue(Account.AccountStatus.INACTIVE); 119 break; 120 case ENTEREDINERROR: 121 tgt.setValue(Account.AccountStatus.ENTEREDINERROR); 122 break; 123 default: 124 tgt.setValue(Account.AccountStatus.NULL); 125 break; 126 } 127 } 128 return tgt; 129 } 130 131 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Account.AccountStatus> src) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Account.AccountStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Account.AccountStatusEnumFactory()); 135 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 136 if (src.getValue() == null) { 137 tgt.setValue(null); 138 } else { 139 switch (src.getValue()) { 140 case ACTIVE: 141 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.ACTIVE); 142 break; 143 case INACTIVE: 144 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.INACTIVE); 145 break; 146 case ENTEREDINERROR: 147 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.ENTEREDINERROR); 148 break; 149 case ONHOLD: 150 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.ACTIVE); 151 break; 152 case UNKNOWN: 153 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.ACTIVE); 154 break; 155 default: 156 tgt.setValue(org.hl7.fhir.dstu3.model.Account.AccountStatus.NULL); 157 break; 158 } 159 } 160 return tgt; 161 } 162 163 public static org.hl7.fhir.r4.model.Account.CoverageComponent convertCoverageComponent(org.hl7.fhir.dstu3.model.Account.CoverageComponent src) throws FHIRException { 164 if (src == null) 165 return null; 166 org.hl7.fhir.r4.model.Account.CoverageComponent tgt = new org.hl7.fhir.r4.model.Account.CoverageComponent(); 167 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 168 if (src.hasCoverage()) 169 tgt.setCoverage(Reference30_40.convertReference(src.getCoverage())); 170 if (src.hasPriority()) 171 tgt.setPriorityElement(PositiveInt30_40.convertPositiveInt(src.getPriorityElement())); 172 return tgt; 173 } 174 175 public static org.hl7.fhir.dstu3.model.Account.CoverageComponent convertCoverageComponent(org.hl7.fhir.r4.model.Account.CoverageComponent src) throws FHIRException { 176 if (src == null) 177 return null; 178 org.hl7.fhir.dstu3.model.Account.CoverageComponent tgt = new org.hl7.fhir.dstu3.model.Account.CoverageComponent(); 179 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 180 if (src.hasCoverage()) 181 tgt.setCoverage(Reference30_40.convertReference(src.getCoverage())); 182 if (src.hasPriority()) 183 tgt.setPriorityElement(PositiveInt30_40.convertPositiveInt(src.getPriorityElement())); 184 return tgt; 185 } 186 187 public static org.hl7.fhir.r4.model.Account.GuarantorComponent convertGuarantorComponent(org.hl7.fhir.dstu3.model.Account.GuarantorComponent src) throws FHIRException { 188 if (src == null) 189 return null; 190 org.hl7.fhir.r4.model.Account.GuarantorComponent tgt = new org.hl7.fhir.r4.model.Account.GuarantorComponent(); 191 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 192 if (src.hasParty()) 193 tgt.setParty(Reference30_40.convertReference(src.getParty())); 194 if (src.hasOnHold()) 195 tgt.setOnHoldElement(Boolean30_40.convertBoolean(src.getOnHoldElement())); 196 if (src.hasPeriod()) 197 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 198 return tgt; 199 } 200 201 public static org.hl7.fhir.dstu3.model.Account.GuarantorComponent convertGuarantorComponent(org.hl7.fhir.r4.model.Account.GuarantorComponent src) throws FHIRException { 202 if (src == null) 203 return null; 204 org.hl7.fhir.dstu3.model.Account.GuarantorComponent tgt = new org.hl7.fhir.dstu3.model.Account.GuarantorComponent(); 205 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 206 if (src.hasParty()) 207 tgt.setParty(Reference30_40.convertReference(src.getParty())); 208 if (src.hasOnHold()) 209 tgt.setOnHoldElement(Boolean30_40.convertBoolean(src.getOnHoldElement())); 210 if (src.hasPeriod()) 211 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 212 return tgt; 213 } 214}