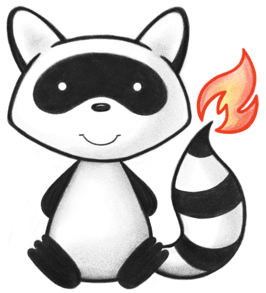
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Instant30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.UnsignedInt30_40; 013import org.hl7.fhir.dstu3.model.Appointment; 014import org.hl7.fhir.dstu3.model.Enumeration; 015import org.hl7.fhir.exceptions.FHIRException; 016 017public class Appointment30_40 { 018 019 public static org.hl7.fhir.r4.model.Appointment convertAppointment(org.hl7.fhir.dstu3.model.Appointment src) throws FHIRException { 020 if (src == null) 021 return null; 022 org.hl7.fhir.r4.model.Appointment tgt = new org.hl7.fhir.r4.model.Appointment(); 023 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 028 if (src.hasServiceCategory()) 029 tgt.addServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategory())); 030 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceType()) 031 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 032 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 033 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 034 if (src.hasAppointmentType()) 035 tgt.setAppointmentType(CodeableConcept30_40.convertCodeableConcept(src.getAppointmentType())); 036 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReason()) 037 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getIndication()) 039 tgt.addReasonReference(Reference30_40.convertReference(t)); 040 if (src.hasPriority()) 041 tgt.setPriorityElement(UnsignedInt30_40.convertUnsignedInt(src.getPriorityElement())); 042 if (src.hasDescription()) 043 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 044 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInformation()) 045 tgt.addSupportingInformation(Reference30_40.convertReference(t)); 046 if (src.hasStart()) 047 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 048 if (src.hasEnd()) 049 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 050 if (src.hasMinutesDuration()) 051 tgt.setMinutesDurationElement(PositiveInt30_40.convertPositiveInt(src.getMinutesDurationElement())); 052 for (org.hl7.fhir.dstu3.model.Reference t : src.getSlot()) tgt.addSlot(Reference30_40.convertReference(t)); 053 if (src.hasCreated()) 054 tgt.setCreatedElement(DateTime30_40.convertDateTime(src.getCreatedElement())); 055 if (src.hasComment()) 056 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 057 for (org.hl7.fhir.dstu3.model.Reference t : src.getIncomingReferral()) 058 tgt.addBasedOn(Reference30_40.convertReference(t)); 059 for (org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 060 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 061 for (org.hl7.fhir.dstu3.model.Period t : src.getRequestedPeriod()) 062 tgt.addRequestedPeriod(Period30_40.convertPeriod(t)); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.dstu3.model.Appointment convertAppointment(org.hl7.fhir.r4.model.Appointment src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.dstu3.model.Appointment tgt = new org.hl7.fhir.dstu3.model.Appointment(); 070 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 071 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 072 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 073 if (src.hasStatus()) 074 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 075 if (src.hasServiceCategory()) 076 tgt.setServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategoryFirstRep())); 077 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceType()) 078 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 079 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) 080 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 081 if (src.hasAppointmentType()) 082 tgt.setAppointmentType(CodeableConcept30_40.convertCodeableConcept(src.getAppointmentType())); 083 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 084 tgt.addReason(CodeableConcept30_40.convertCodeableConcept(t)); 085 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 086 tgt.addIndication(Reference30_40.convertReference(t)); 087 if (src.hasPriority()) 088 tgt.setPriorityElement(UnsignedInt30_40.convertUnsignedInt(src.getPriorityElement())); 089 if (src.hasDescription()) 090 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 091 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInformation()) 092 tgt.addSupportingInformation(Reference30_40.convertReference(t)); 093 if (src.hasStart()) 094 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 095 if (src.hasEnd()) 096 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 097 if (src.hasMinutesDuration()) 098 tgt.setMinutesDurationElement(PositiveInt30_40.convertPositiveInt(src.getMinutesDurationElement())); 099 for (org.hl7.fhir.r4.model.Reference t : src.getSlot()) tgt.addSlot(Reference30_40.convertReference(t)); 100 if (src.hasCreated()) 101 tgt.setCreatedElement(DateTime30_40.convertDateTime(src.getCreatedElement())); 102 if (src.hasComment()) 103 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 104 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) 105 tgt.addIncomingReferral(Reference30_40.convertReference(t)); 106 for (org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 107 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 108 for (org.hl7.fhir.r4.model.Period t : src.getRequestedPeriod()) 109 tgt.addRequestedPeriod(Period30_40.convertPeriod(t)); 110 return tgt; 111 } 112 113 public static org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 114 if (src == null) 115 return null; 116 org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent(); 117 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 118 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 119 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 120 if (src.hasActor()) 121 tgt.setActor(Reference30_40.convertReference(src.getActor())); 122 if (src.hasRequired()) 123 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 124 if (src.hasStatus()) 125 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 126 return tgt; 127 } 128 129 public static org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 130 if (src == null) 131 return null; 132 org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent(); 133 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 134 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 135 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 136 if (src.hasActor()) 137 tgt.setActor(Reference30_40.convertReference(src.getActor())); 138 if (src.hasRequired()) 139 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 140 if (src.hasStatus()) 141 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 142 return tgt; 143 } 144 145 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 Enumeration<Appointment.AppointmentStatus> tgt = new Enumeration<>(new Appointment.AppointmentStatusEnumFactory()); 149 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 150 if (src.getValue() == null) { 151 tgt.setValue(null); 152 } else { 153 switch (src.getValue()) { 154 case PROPOSED: 155 tgt.setValue(Appointment.AppointmentStatus.PROPOSED); 156 break; 157 case PENDING: 158 tgt.setValue(Appointment.AppointmentStatus.PENDING); 159 break; 160 case BOOKED: 161 tgt.setValue(Appointment.AppointmentStatus.BOOKED); 162 break; 163 case ARRIVED: 164 tgt.setValue(Appointment.AppointmentStatus.ARRIVED); 165 break; 166 case FULFILLED: 167 tgt.setValue(Appointment.AppointmentStatus.FULFILLED); 168 break; 169 case CANCELLED: 170 tgt.setValue(Appointment.AppointmentStatus.CANCELLED); 171 break; 172 case NOSHOW: 173 tgt.setValue(Appointment.AppointmentStatus.NOSHOW); 174 break; 175 case ENTEREDINERROR: 176 tgt.setValue(Appointment.AppointmentStatus.ENTEREDINERROR); 177 break; 178 default: 179 tgt.setValue(Appointment.AppointmentStatus.NULL); 180 break; 181 } 182 } 183 return tgt; 184 } 185 186 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.AppointmentStatus> src) throws FHIRException { 187 if (src == null || src.isEmpty()) 188 return null; 189 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.AppointmentStatusEnumFactory()); 190 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 191 if (src.getValue() == null) { 192 tgt.setValue(null); 193 } else { 194 switch (src.getValue()) { 195 case PROPOSED: 196 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PROPOSED); 197 break; 198 case PENDING: 199 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PENDING); 200 break; 201 case BOOKED: 202 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.BOOKED); 203 break; 204 case ARRIVED: 205 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.ARRIVED); 206 break; 207 case FULFILLED: 208 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.FULFILLED); 209 break; 210 case CANCELLED: 211 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.CANCELLED); 212 break; 213 case NOSHOW: 214 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NOSHOW); 215 break; 216 case ENTEREDINERROR: 217 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.ENTEREDINERROR); 218 break; 219 default: 220 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NULL); 221 break; 222 } 223 } 224 return tgt; 225 } 226 227 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> src) throws FHIRException { 228 if (src == null || src.isEmpty()) 229 return null; 230 Enumeration<Appointment.ParticipantRequired> tgt = new Enumeration<>(new Appointment.ParticipantRequiredEnumFactory()); 231 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 232 if (src.getValue() == null) { 233 tgt.setValue(null); 234 } else { 235 switch (src.getValue()) { 236 case REQUIRED: 237 tgt.setValue(Appointment.ParticipantRequired.REQUIRED); 238 break; 239 case OPTIONAL: 240 tgt.setValue(Appointment.ParticipantRequired.OPTIONAL); 241 break; 242 case INFORMATIONONLY: 243 tgt.setValue(Appointment.ParticipantRequired.INFORMATIONONLY); 244 break; 245 default: 246 tgt.setValue(Appointment.ParticipantRequired.NULL); 247 break; 248 } 249 } 250 return tgt; 251 } 252 253 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired> src) throws FHIRException { 254 if (src == null || src.isEmpty()) 255 return null; 256 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipantRequiredEnumFactory()); 257 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 258 if (src.getValue() == null) { 259 tgt.setValue(null); 260 } else { 261 switch (src.getValue()) { 262 case REQUIRED: 263 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.REQUIRED); 264 break; 265 case OPTIONAL: 266 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.OPTIONAL); 267 break; 268 case INFORMATIONONLY: 269 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.INFORMATIONONLY); 270 break; 271 default: 272 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.NULL); 273 break; 274 } 275 } 276 return tgt; 277 } 278 279 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipationStatus> src) throws FHIRException { 280 if (src == null || src.isEmpty()) 281 return null; 282 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipationStatusEnumFactory()); 283 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 284 if (src.getValue() == null) { 285 tgt.setValue(null); 286 } else { 287 switch (src.getValue()) { 288 case ACCEPTED: 289 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.ACCEPTED); 290 break; 291 case DECLINED: 292 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.DECLINED); 293 break; 294 case TENTATIVE: 295 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.TENTATIVE); 296 break; 297 case NEEDSACTION: 298 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NEEDSACTION); 299 break; 300 default: 301 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NULL); 302 break; 303 } 304 } 305 return tgt; 306 } 307 308 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> src) throws FHIRException { 309 if (src == null || src.isEmpty()) 310 return null; 311 Enumeration<Appointment.ParticipationStatus> tgt = new Enumeration<>(new Appointment.ParticipationStatusEnumFactory()); 312 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 313 if (src.getValue() == null) { 314 tgt.setValue(null); 315 } else { 316 switch (src.getValue()) { 317 case ACCEPTED: 318 tgt.setValue(Appointment.ParticipationStatus.ACCEPTED); 319 break; 320 case DECLINED: 321 tgt.setValue(Appointment.ParticipationStatus.DECLINED); 322 break; 323 case TENTATIVE: 324 tgt.setValue(Appointment.ParticipationStatus.TENTATIVE); 325 break; 326 case NEEDSACTION: 327 tgt.setValue(Appointment.ParticipationStatus.NEEDSACTION); 328 break; 329 default: 330 tgt.setValue(Appointment.ParticipationStatus.NULL); 331 break; 332 } 333 } 334 return tgt; 335 } 336}