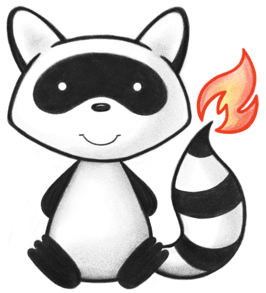
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Instant30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 009import org.hl7.fhir.exceptions.FHIRException; 010 011public class AppointmentResponse30_40 { 012 013 public static org.hl7.fhir.r4.model.AppointmentResponse convertAppointmentResponse(org.hl7.fhir.dstu3.model.AppointmentResponse src) throws FHIRException { 014 if (src == null) 015 return null; 016 org.hl7.fhir.r4.model.AppointmentResponse tgt = new org.hl7.fhir.r4.model.AppointmentResponse(); 017 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 018 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 019 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 020 if (src.hasAppointment()) 021 tgt.setAppointment(Reference30_40.convertReference(src.getAppointment())); 022 if (src.hasStart()) 023 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 024 if (src.hasEnd()) 025 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 026 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getParticipantType()) 027 tgt.addParticipantType(CodeableConcept30_40.convertCodeableConcept(t)); 028 if (src.hasActor()) 029 tgt.setActor(Reference30_40.convertReference(src.getActor())); 030 if (src.hasParticipantStatus()) 031 tgt.setParticipantStatusElement(convertParticipantStatus(src.getParticipantStatusElement())); 032 if (src.hasComment()) 033 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 034 return tgt; 035 } 036 037 public static org.hl7.fhir.dstu3.model.AppointmentResponse convertAppointmentResponse(org.hl7.fhir.r4.model.AppointmentResponse src) throws FHIRException { 038 if (src == null) 039 return null; 040 org.hl7.fhir.dstu3.model.AppointmentResponse tgt = new org.hl7.fhir.dstu3.model.AppointmentResponse(); 041 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 042 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 043 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 044 if (src.hasAppointment()) 045 tgt.setAppointment(Reference30_40.convertReference(src.getAppointment())); 046 if (src.hasStart()) 047 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 048 if (src.hasEnd()) 049 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 050 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getParticipantType()) 051 tgt.addParticipantType(CodeableConcept30_40.convertCodeableConcept(t)); 052 if (src.hasActor()) 053 tgt.setActor(Reference30_40.convertReference(src.getActor())); 054 if (src.hasParticipantStatus()) 055 tgt.setParticipantStatusElement(convertParticipantStatus(src.getParticipantStatusElement())); 056 if (src.hasComment()) 057 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 058 return tgt; 059 } 060 061 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus> convertParticipantStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> src) throws FHIRException { 062 if (src == null || src.isEmpty()) 063 return null; 064 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatusEnumFactory()); 065 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 066 switch (src.getValue()) { 067 case ACCEPTED: 068 tgt.setValue(org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus.ACCEPTED); 069 break; 070 case DECLINED: 071 tgt.setValue(org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus.DECLINED); 072 break; 073 case TENTATIVE: 074 tgt.setValue(org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus.TENTATIVE); 075 break; 076 case NEEDSACTION: 077 tgt.setValue(org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus.NEEDSACTION); 078 break; 079 default: 080 tgt.setValue(org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus.NULL); 081 break; 082 } 083 return tgt; 084 } 085 086 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> convertParticipantStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AppointmentResponse.ParticipantStatus> src) throws FHIRException { 087 if (src == null || src.isEmpty()) 088 return null; 089 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatusEnumFactory()); 090 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 091 switch (src.getValue()) { 092 case ACCEPTED: 093 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.ACCEPTED); 094 break; 095 case DECLINED: 096 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.DECLINED); 097 break; 098 case TENTATIVE: 099 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.TENTATIVE); 100 break; 101 case NEEDSACTION: 102 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.NEEDSACTION); 103 break; 104 default: 105 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.NULL); 106 break; 107 } 108 return tgt; 109 } 110}