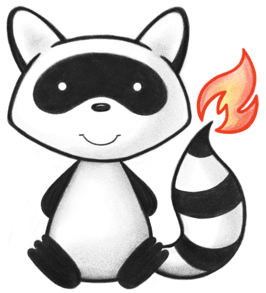
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 009import org.hl7.fhir.exceptions.FHIRException; 010 011public class BodySite30_40 { 012 013 public static org.hl7.fhir.dstu3.model.BodySite convertBodySite(org.hl7.fhir.r4.model.BodyStructure src) throws FHIRException { 014 if (src == null) 015 return null; 016 org.hl7.fhir.dstu3.model.BodySite tgt = new org.hl7.fhir.dstu3.model.BodySite(); 017 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 018 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 019 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 020 if (src.hasActive()) 021 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 022 if (src.hasDescription()) 023 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 024 for (org.hl7.fhir.r4.model.Attachment t : src.getImage()) tgt.addImage(Attachment30_40.convertAttachment(t)); 025 if (src.hasPatient()) 026 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 027 return tgt; 028 } 029 030 public static org.hl7.fhir.r4.model.BodyStructure convertBodySite(org.hl7.fhir.dstu3.model.BodySite src) throws FHIRException { 031 if (src == null) 032 return null; 033 org.hl7.fhir.r4.model.BodyStructure tgt = new org.hl7.fhir.r4.model.BodyStructure(); 034 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 035 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 036 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 037 if (src.hasActive()) 038 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 039 if (src.hasDescription()) 040 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 041 for (org.hl7.fhir.dstu3.model.Attachment t : src.getImage()) tgt.addImage(Attachment30_40.convertAttachment(t)); 042 if (src.hasPatient()) 043 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 044 return tgt; 045 } 046}