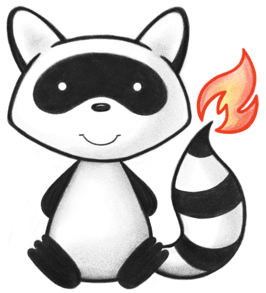
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Signature30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Instant30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.UnsignedInt30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 011import org.hl7.fhir.dstu3.model.Bundle; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class Bundle30_40 { 016 017 public static org.hl7.fhir.r4.model.Bundle convertBundle(org.hl7.fhir.dstu3.model.Bundle src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.r4.model.Bundle tgt = new org.hl7.fhir.r4.model.Bundle(); 021 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyResource(src, tgt); 022 if (src.hasIdentifier()) 023 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 024 if (src.hasType()) 025 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 026 if (src.hasTotal()) 027 tgt.setTotalElement(UnsignedInt30_40.convertUnsignedInt(src.getTotalElement())); 028 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 029 tgt.addLink(convertBundleLinkComponent(t)); 030 for (org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent t : src.getEntry()) 031 tgt.addEntry(convertBundleEntryComponent(t)); 032 if (src.hasSignature()) 033 tgt.setSignature(Signature30_40.convertSignature(src.getSignature())); 034 return tgt; 035 } 036 037 public static org.hl7.fhir.dstu3.model.Bundle convertBundle(org.hl7.fhir.r4.model.Bundle src) throws FHIRException { 038 if (src == null) 039 return null; 040 org.hl7.fhir.dstu3.model.Bundle tgt = new org.hl7.fhir.dstu3.model.Bundle(); 041 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyResource(src, tgt); 042 if (src.hasIdentifier()) 043 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 044 if (src.hasType()) 045 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 046 if (src.hasTotal()) 047 tgt.setTotalElement(UnsignedInt30_40.convertUnsignedInt(src.getTotalElement())); 048 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 049 for (org.hl7.fhir.r4.model.Bundle.BundleEntryComponent t : src.getEntry()) 050 tgt.addEntry(convertBundleEntryComponent(t)); 051 if (src.hasSignature()) 052 tgt.setSignature(Signature30_40.convertSignature(src.getSignature())); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryComponent src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent(); 060 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 061 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 062 if (src.hasFullUrl()) 063 tgt.setFullUrlElement(Uri30_40.convertUri(src.getFullUrlElement())); 064 if (src.hasResource()) 065 tgt.setResource(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertResource(src.getResource())); 066 if (src.hasSearch()) 067 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 068 if (src.hasRequest()) 069 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 070 if (src.hasResponse()) 071 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r4.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent src) throws FHIRException { 076 if (src == null) 077 return null; 078 org.hl7.fhir.r4.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryComponent(); 079 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 080 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 081 tgt.addLink(convertBundleLinkComponent(t)); 082 if (src.hasFullUrl()) 083 tgt.setFullUrlElement(Uri30_40.convertUri(src.getFullUrlElement())); 084 if (src.hasResource()) 085 tgt.setResource(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertResource(src.getResource())); 086 if (src.hasSearch()) 087 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 088 if (src.hasRequest()) 089 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 090 if (src.hasResponse()) 091 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 096 if (src == null) 097 return null; 098 org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent(); 099 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 100 if (src.hasMethod()) 101 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 102 if (src.hasUrl()) 103 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 104 if (src.hasIfNoneMatch()) 105 tgt.setIfNoneMatchElement(String30_40.convertString(src.getIfNoneMatchElement())); 106 if (src.hasIfModifiedSince()) 107 tgt.setIfModifiedSinceElement(Instant30_40.convertInstant(src.getIfModifiedSinceElement())); 108 if (src.hasIfMatch()) 109 tgt.setIfMatchElement(String30_40.convertString(src.getIfMatchElement())); 110 if (src.hasIfNoneExist()) 111 tgt.setIfNoneExistElement(String30_40.convertString(src.getIfNoneExistElement())); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 116 if (src == null) 117 return null; 118 org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent(); 119 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 120 if (src.hasMethod()) 121 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 122 if (src.hasUrl()) 123 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 124 if (src.hasIfNoneMatch()) 125 tgt.setIfNoneMatchElement(String30_40.convertString(src.getIfNoneMatchElement())); 126 if (src.hasIfModifiedSince()) 127 tgt.setIfModifiedSinceElement(Instant30_40.convertInstant(src.getIfModifiedSinceElement())); 128 if (src.hasIfMatch()) 129 tgt.setIfMatchElement(String30_40.convertString(src.getIfMatchElement())); 130 if (src.hasIfNoneExist()) 131 tgt.setIfNoneExistElement(String30_40.convertString(src.getIfNoneExistElement())); 132 return tgt; 133 } 134 135 public static org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 136 if (src == null) 137 return null; 138 org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent(); 139 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 140 if (src.hasStatus()) 141 tgt.setStatusElement(String30_40.convertString(src.getStatusElement())); 142 if (src.hasLocation()) 143 tgt.setLocationElement(Uri30_40.convertUri(src.getLocationElement())); 144 if (src.hasEtag()) 145 tgt.setEtagElement(String30_40.convertString(src.getEtagElement())); 146 if (src.hasLastModified()) 147 tgt.setLastModifiedElement(Instant30_40.convertInstant(src.getLastModifiedElement())); 148 if (src.hasOutcome()) 149 tgt.setOutcome(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertResource(src.getOutcome())); 150 return tgt; 151 } 152 153 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 154 if (src == null) 155 return null; 156 org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent(); 157 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 158 if (src.hasStatus()) 159 tgt.setStatusElement(String30_40.convertString(src.getStatusElement())); 160 if (src.hasLocation()) 161 tgt.setLocationElement(Uri30_40.convertUri(src.getLocationElement())); 162 if (src.hasEtag()) 163 tgt.setEtagElement(String30_40.convertString(src.getEtagElement())); 164 if (src.hasLastModified()) 165 tgt.setLastModifiedElement(Instant30_40.convertInstant(src.getLastModifiedElement())); 166 if (src.hasOutcome()) 167 tgt.setOutcome(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertResource(src.getOutcome())); 168 return tgt; 169 } 170 171 public static org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 172 if (src == null) 173 return null; 174 org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent(); 175 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 176 if (src.hasMode()) 177 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 178 if (src.hasScore()) 179 tgt.setScoreElement(Decimal30_40.convertDecimal(src.getScoreElement())); 180 return tgt; 181 } 182 183 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 184 if (src == null) 185 return null; 186 org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent(); 187 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 188 if (src.hasMode()) 189 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 190 if (src.hasScore()) 191 tgt.setScoreElement(Decimal30_40.convertDecimal(src.getScoreElement())); 192 return tgt; 193 } 194 195 public static org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r4.model.Bundle.BundleLinkComponent src) throws FHIRException { 196 if (src == null) 197 return null; 198 org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent(); 199 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 200 if (src.hasRelation()) 201 tgt.setRelationElement(String30_40.convertString(src.getRelationElement())); 202 if (src.hasUrl()) 203 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 204 return tgt; 205 } 206 207 public static org.hl7.fhir.r4.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent src) throws FHIRException { 208 if (src == null) 209 return null; 210 org.hl7.fhir.r4.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleLinkComponent(); 211 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 212 if (src.hasRelation()) 213 tgt.setRelationElement(String30_40.convertString(src.getRelationElement())); 214 if (src.hasUrl()) 215 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 216 return tgt; 217 } 218 219 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> src) throws FHIRException { 220 if (src == null || src.isEmpty()) 221 return null; 222 Enumeration<Bundle.BundleType> tgt = new Enumeration<>(new Bundle.BundleTypeEnumFactory()); 223 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 224 if (src.getValue() == null) { 225 tgt.setValue(null); 226 } else { 227 switch (src.getValue()) { 228 case DOCUMENT: 229 tgt.setValue(Bundle.BundleType.DOCUMENT); 230 break; 231 case MESSAGE: 232 tgt.setValue(Bundle.BundleType.MESSAGE); 233 break; 234 case TRANSACTION: 235 tgt.setValue(Bundle.BundleType.TRANSACTION); 236 break; 237 case TRANSACTIONRESPONSE: 238 tgt.setValue(Bundle.BundleType.TRANSACTIONRESPONSE); 239 break; 240 case BATCH: 241 tgt.setValue(Bundle.BundleType.BATCH); 242 break; 243 case BATCHRESPONSE: 244 tgt.setValue(Bundle.BundleType.BATCHRESPONSE); 245 break; 246 case HISTORY: 247 tgt.setValue(Bundle.BundleType.HISTORY); 248 break; 249 case SEARCHSET: 250 tgt.setValue(Bundle.BundleType.SEARCHSET); 251 break; 252 case COLLECTION: 253 tgt.setValue(Bundle.BundleType.COLLECTION); 254 break; 255 default: 256 tgt.setValue(Bundle.BundleType.NULL); 257 break; 258 } 259 } 260 return tgt; 261 } 262 263 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> src) throws FHIRException { 264 if (src == null || src.isEmpty()) 265 return null; 266 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.BundleTypeEnumFactory()); 267 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 268 if (src.getValue() == null) { 269 tgt.setValue(null); 270 } else { 271 switch (src.getValue()) { 272 case DOCUMENT: 273 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.DOCUMENT); 274 break; 275 case MESSAGE: 276 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.MESSAGE); 277 break; 278 case TRANSACTION: 279 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTION); 280 break; 281 case TRANSACTIONRESPONSE: 282 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTIONRESPONSE); 283 break; 284 case BATCH: 285 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCH); 286 break; 287 case BATCHRESPONSE: 288 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCHRESPONSE); 289 break; 290 case HISTORY: 291 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.HISTORY); 292 break; 293 case SEARCHSET: 294 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.SEARCHSET); 295 break; 296 case COLLECTION: 297 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.COLLECTION); 298 break; 299 default: 300 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.NULL); 301 break; 302 } 303 } 304 return tgt; 305 } 306 307 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> src) throws FHIRException { 308 if (src == null || src.isEmpty()) 309 return null; 310 Enumeration<Bundle.HTTPVerb> tgt = new Enumeration<>(new Bundle.HTTPVerbEnumFactory()); 311 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 312 if (src.getValue() == null) { 313 tgt.setValue(null); 314 } else { 315 switch (src.getValue()) { 316 case GET: 317 tgt.setValue(Bundle.HTTPVerb.GET); 318 break; 319 case POST: 320 tgt.setValue(Bundle.HTTPVerb.POST); 321 break; 322 case PUT: 323 tgt.setValue(Bundle.HTTPVerb.PUT); 324 break; 325 case DELETE: 326 tgt.setValue(Bundle.HTTPVerb.DELETE); 327 break; 328 default: 329 tgt.setValue(Bundle.HTTPVerb.NULL); 330 break; 331 } 332 } 333 return tgt; 334 } 335 336 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> src) throws FHIRException { 337 if (src == null || src.isEmpty()) 338 return null; 339 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.HTTPVerbEnumFactory()); 340 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 341 if (src.getValue() == null) { 342 tgt.setValue(null); 343 } else { 344 switch (src.getValue()) { 345 case GET: 346 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.GET); 347 break; 348 case POST: 349 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.POST); 350 break; 351 case PUT: 352 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.PUT); 353 break; 354 case DELETE: 355 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.DELETE); 356 break; 357 default: 358 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.NULL); 359 break; 360 } 361 } 362 return tgt; 363 } 364 365 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> src) throws FHIRException { 366 if (src == null || src.isEmpty()) 367 return null; 368 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.SearchEntryModeEnumFactory()); 369 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 370 if (src.getValue() == null) { 371 tgt.setValue(null); 372 } else { 373 switch (src.getValue()) { 374 case MATCH: 375 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.MATCH); 376 break; 377 case INCLUDE: 378 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.INCLUDE); 379 break; 380 case OUTCOME: 381 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.OUTCOME); 382 break; 383 default: 384 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.NULL); 385 break; 386 } 387 } 388 return tgt; 389 } 390 391 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> src) throws FHIRException { 392 if (src == null || src.isEmpty()) 393 return null; 394 Enumeration<Bundle.SearchEntryMode> tgt = new Enumeration<>(new Bundle.SearchEntryModeEnumFactory()); 395 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 396 if (src.getValue() == null) { 397 tgt.setValue(null); 398 } else { 399 switch (src.getValue()) { 400 case MATCH: 401 tgt.setValue(Bundle.SearchEntryMode.MATCH); 402 break; 403 case INCLUDE: 404 tgt.setValue(Bundle.SearchEntryMode.INCLUDE); 405 break; 406 case OUTCOME: 407 tgt.setValue(Bundle.SearchEntryMode.OUTCOME); 408 break; 409 default: 410 tgt.setValue(Bundle.SearchEntryMode.NULL); 411 break; 412 } 413 } 414 return tgt; 415 } 416}