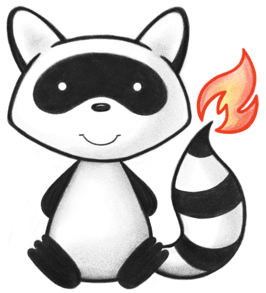
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.SimpleQuantity30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 014import org.hl7.fhir.dstu3.model.Reference; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r4.model.Coding; 017 018public class CarePlan30_40 { 019 020 private static final String CarePlanActivityDetailComponentExtension = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CarePlan.activity.detail.category"; 021 022 public static org.hl7.fhir.dstu3.model.CarePlan convertCarePlan(org.hl7.fhir.r4.model.CarePlan src) throws FHIRException { 023 if (src == null) 024 return null; 025 org.hl7.fhir.dstu3.model.CarePlan tgt = new org.hl7.fhir.dstu3.model.CarePlan(); 026 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 027 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 028 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 029 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) { 030 tgt.addBasedOn(Reference30_40.convertReference(t)); 031 } 032 for (org.hl7.fhir.r4.model.Reference t : src.getReplaces()) { 033 tgt.addReplaces(Reference30_40.convertReference(t)); 034 } 035 for (org.hl7.fhir.r4.model.Reference t : src.getPartOf()) { 036 tgt.addPartOf(Reference30_40.convertReference(t)); 037 } 038 if (src.hasStatus()) { 039 if (src.hasStatus()) 040 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 041 } 042 if (src.hasIntent()) { 043 if (src.hasIntent()) 044 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 045 } 046 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) { 047 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 048 } 049 if (src.hasTitle()) { 050 if (src.hasTitleElement()) 051 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 052 } 053 if (src.hasDescription()) { 054 if (src.hasDescriptionElement()) 055 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 056 } 057 if (src.hasSubject()) { 058 if (src.hasSubject()) 059 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 060 } 061 if (src.hasEncounter()) { 062 if (src.hasEncounter()) 063 tgt.setContext(Reference30_40.convertReference(src.getEncounter())); 064 } 065 if (src.hasPeriod()) { 066 if (src.hasPeriod()) 067 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 068 } 069 if (src.hasAuthor()) { 070 if (src.hasAuthor()) 071 tgt.addAuthor(Reference30_40.convertReference(src.getAuthor())); 072 } 073 for (org.hl7.fhir.r4.model.Reference t : src.getCareTeam()) { 074 tgt.addCareTeam(Reference30_40.convertReference(t)); 075 } 076 for (org.hl7.fhir.r4.model.Reference t : src.getAddresses()) { 077 tgt.addAddresses(Reference30_40.convertReference(t)); 078 } 079 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInfo()) { 080 tgt.addSupportingInfo(Reference30_40.convertReference(t)); 081 } 082 for (org.hl7.fhir.r4.model.Reference t : src.getGoal()) { 083 tgt.addGoal(Reference30_40.convertReference(t)); 084 } 085 for (org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) { 086 tgt.addActivity(convertCarePlanActivityComponent(t)); 087 } 088 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) { 089 tgt.addNote(Annotation30_40.convertAnnotation(t)); 090 } 091 return tgt; 092 } 093 094 public static org.hl7.fhir.r4.model.CarePlan convertCarePlan(org.hl7.fhir.dstu3.model.CarePlan src) throws FHIRException { 095 if (src == null) 096 return null; 097 org.hl7.fhir.r4.model.CarePlan tgt = new org.hl7.fhir.r4.model.CarePlan(); 098 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 099 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 100 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 101 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) { 102 tgt.addBasedOn(Reference30_40.convertReference(t)); 103 } 104 for (org.hl7.fhir.dstu3.model.Reference t : src.getReplaces()) { 105 tgt.addReplaces(Reference30_40.convertReference(t)); 106 } 107 for (org.hl7.fhir.dstu3.model.Reference t : src.getPartOf()) { 108 tgt.addPartOf(Reference30_40.convertReference(t)); 109 } 110 if (src.hasStatus()) { 111 if (src.hasStatus()) 112 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 113 } 114 if (src.hasIntent()) { 115 if (src.hasIntent()) 116 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 117 } 118 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) { 119 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 120 } 121 if (src.hasTitle()) { 122 if (src.hasTitleElement()) 123 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 124 } 125 if (src.hasDescription()) { 126 if (src.hasDescriptionElement()) 127 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 128 } 129 if (src.hasSubject()) { 130 if (src.hasSubject()) 131 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 132 } 133 if (src.hasContext()) { 134 if (src.hasContext()) 135 tgt.setEncounter(Reference30_40.convertReference(src.getContext())); 136 } 137 if (src.hasPeriod()) { 138 if (src.hasPeriod()) 139 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 140 } 141 List<Reference> authors = src.getAuthor(); 142 if (authors.size() > 0) { 143 tgt.setAuthor(Reference30_40.convertReference(authors.get(0))); 144 if (authors.size() > 1) { 145 } 146 } 147 for (org.hl7.fhir.dstu3.model.Reference t : src.getCareTeam()) { 148 tgt.addCareTeam(Reference30_40.convertReference(t)); 149 } 150 for (org.hl7.fhir.dstu3.model.Reference t : src.getAddresses()) { 151 tgt.addAddresses(Reference30_40.convertReference(t)); 152 } 153 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInfo()) { 154 tgt.addSupportingInfo(Reference30_40.convertReference(t)); 155 } 156 for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) { 157 tgt.addGoal(Reference30_40.convertReference(t)); 158 } 159 for (org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) { 160 tgt.addActivity(convertCarePlanActivityComponent(t)); 161 } 162 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) { 163 tgt.addNote(Annotation30_40.convertAnnotation(t)); 164 } 165 return tgt; 166 } 167 168 public static org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 169 if (src == null) 170 return null; 171 org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent(); 172 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 173 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getOutcomeCodeableConcept()) { 174 tgt.addOutcomeCodeableConcept(CodeableConcept30_40.convertCodeableConcept(t)); 175 } 176 for (org.hl7.fhir.dstu3.model.Reference t : src.getOutcomeReference()) { 177 tgt.addOutcomeReference(Reference30_40.convertReference(t)); 178 } 179 for (org.hl7.fhir.dstu3.model.Annotation t : src.getProgress()) { 180 tgt.addProgress(Annotation30_40.convertAnnotation(t)); 181 } 182 if (src.hasReference()) { 183 if (src.hasReference()) 184 tgt.setReference(Reference30_40.convertReference(src.getReference())); 185 } 186 if (src.hasDetail()) { 187 if (src.hasDetail()) 188 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 189 } 190 return tgt; 191 } 192 193 public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 194 if (src == null) 195 return null; 196 org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent(); 197 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 198 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getOutcomeCodeableConcept()) { 199 tgt.addOutcomeCodeableConcept(CodeableConcept30_40.convertCodeableConcept(t)); 200 } 201 for (org.hl7.fhir.r4.model.Reference t : src.getOutcomeReference()) { 202 tgt.addOutcomeReference(Reference30_40.convertReference(t)); 203 } 204 for (org.hl7.fhir.r4.model.Annotation t : src.getProgress()) { 205 tgt.addProgress(Annotation30_40.convertAnnotation(t)); 206 } 207 if (src.hasReference()) { 208 if (src.hasReference()) 209 tgt.setReference(Reference30_40.convertReference(src.getReference())); 210 } 211 if (src.hasDetail()) { 212 if (src.hasDetail()) 213 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 214 } 215 return tgt; 216 } 217 218 public static org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 219 if (src == null) 220 return null; 221 org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent(); 222 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 223 if (src.hasCategory()) { 224 org.hl7.fhir.r4.model.Extension t = new org.hl7.fhir.r4.model.Extension(); 225 t.setUrl(CarePlanActivityDetailComponentExtension); 226 t.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getCategory())); 227 tgt.addExtension(t); 228 } 229 if (src.hasCode()) { 230 if (src.hasCode()) 231 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 232 } 233 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) { 234 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 235 } 236 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) { 237 tgt.addReasonReference(Reference30_40.convertReference(t)); 238 } 239 for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) { 240 tgt.addGoal(Reference30_40.convertReference(t)); 241 } 242 if (src.hasStatus()) { 243 if (src.hasStatus()) 244 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 245 } 246 if (src.hasStatusReason()) { 247 org.hl7.fhir.r4.model.Coding code = new org.hl7.fhir.r4.model.Coding(); 248 code.setCode(src.getStatusReason()); 249 org.hl7.fhir.r4.model.CodeableConcept t = new org.hl7.fhir.r4.model.CodeableConcept(code); 250 tgt.setStatusReason(t); 251 } 252 if (src.hasProhibited()) { 253 if (src.hasProhibitedElement()) 254 tgt.setDoNotPerformElement(Boolean30_40.convertBoolean(src.getProhibitedElement())); 255 } 256 if (src.hasScheduled()) { 257 if (src.hasScheduled()) 258 tgt.setScheduled(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getScheduled())); 259 } 260 if (src.hasLocation()) { 261 if (src.hasLocation()) 262 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 263 } 264 for (org.hl7.fhir.dstu3.model.Reference t : src.getPerformer()) { 265 tgt.addPerformer(Reference30_40.convertReference(t)); 266 } 267 if (src.hasProduct()) { 268 if (src.hasProduct()) 269 tgt.setProduct(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getProduct())); 270 } 271 if (src.hasDailyAmount()) { 272 if (src.hasDailyAmount()) 273 tgt.setDailyAmount(SimpleQuantity30_40.convertSimpleQuantity(src.getDailyAmount())); 274 } 275 if (src.hasQuantity()) { 276 if (src.hasQuantity()) 277 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 278 } 279 if (src.hasDescription()) { 280 if (src.hasDescriptionElement()) 281 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 282 } 283 return tgt; 284 } 285 286 public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 287 if (src == null) 288 return null; 289 org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent(); 290 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 291 if (src.hasExtension()) { 292 org.hl7.fhir.r4.model.Extension extension = src.getExtensionByUrl(CarePlanActivityDetailComponentExtension); 293 if (extension != null) { 294 org.hl7.fhir.r4.model.Type value = extension.getValue(); 295 if (value instanceof org.hl7.fhir.r4.model.CodeableConcept) { 296 tgt.setCategory(CodeableConcept30_40.convertCodeableConcept((org.hl7.fhir.r4.model.CodeableConcept) value)); 297 } 298 } 299 } 300 if (src.hasCode()) { 301 if (src.hasCode()) 302 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 303 } 304 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) { 305 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 306 } 307 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) { 308 tgt.addReasonReference(Reference30_40.convertReference(t)); 309 } 310 for (org.hl7.fhir.r4.model.Reference t : src.getGoal()) { 311 tgt.addGoal(Reference30_40.convertReference(t)); 312 } 313 if (src.hasStatus()) { 314 if (src.hasStatus()) 315 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 316 } 317 if (src.hasStatusReason()) { 318 List<Coding> coding = src.getStatusReason().getCoding(); 319 if (coding.size() > 0) { 320 tgt.setStatusReason(coding.get(0).getCode()); 321 } 322 } 323 if (src.hasDoNotPerform()) { 324 if (src.hasDoNotPerformElement()) 325 tgt.setProhibitedElement(Boolean30_40.convertBoolean(src.getDoNotPerformElement())); 326 } 327 if (src.hasScheduled()) { 328 if (src.hasScheduled()) 329 tgt.setScheduled(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getScheduled())); 330 } 331 if (src.hasLocation()) { 332 if (src.hasLocation()) 333 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 334 } 335 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) { 336 tgt.addPerformer(Reference30_40.convertReference(t)); 337 } 338 if (src.hasProduct()) { 339 if (src.hasProduct()) 340 tgt.setProduct(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getProduct())); 341 } 342 if (src.hasDailyAmount()) { 343 if (src.hasDailyAmount()) 344 tgt.setDailyAmount(SimpleQuantity30_40.convertSimpleQuantity(src.getDailyAmount())); 345 } 346 if (src.hasQuantity()) { 347 if (src.hasQuantity()) 348 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 349 } 350 if (src.hasDescription()) { 351 if (src.hasDescriptionElement()) 352 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 353 } 354 return tgt; 355 } 356 357 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 358 if (src == null || src.isEmpty()) 359 return null; 360 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatusEnumFactory()); 361 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 362 switch (src.getValue()) { 363 case CANCELLED: 364 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.CANCELLED); 365 break; 366 case COMPLETED: 367 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.COMPLETED); 368 break; 369 case INPROGRESS: 370 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 371 break; 372 case NOTSTARTED: 373 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 374 break; 375 case ONHOLD: 376 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.ONHOLD); 377 break; 378 case SCHEDULED: 379 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 380 break; 381 case UNKNOWN: 382 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 383 break; 384 default: 385 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NULL); 386 break; 387 } 388 return tgt; 389 } 390 391 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 392 if (src == null || src.isEmpty()) 393 return null; 394 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatusEnumFactory()); 395 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 396 switch (src.getValue()) { 397 case CANCELLED: 398 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.CANCELLED); 399 break; 400 case COMPLETED: 401 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.COMPLETED); 402 break; 403 case INPROGRESS: 404 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 405 break; 406 case NOTSTARTED: 407 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 408 break; 409 case ONHOLD: 410 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.ONHOLD); 411 break; 412 case SCHEDULED: 413 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 414 break; 415 case UNKNOWN: 416 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 417 break; 418 default: 419 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus.NULL); 420 break; 421 } 422 return tgt; 423 } 424 425 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent> src) throws FHIRException { 426 if (src == null || src.isEmpty()) 427 return null; 428 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanIntent> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CarePlan.CarePlanIntentEnumFactory()); 429 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 430 switch (src.getValue()) { 431 case OPTION: 432 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanIntent.OPTION); 433 break; 434 case ORDER: 435 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanIntent.ORDER); 436 break; 437 case PLAN: 438 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanIntent.PLAN); 439 break; 440 case PROPOSAL: 441 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanIntent.PROPOSAL); 442 break; 443 default: 444 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanIntent.NULL); 445 break; 446 } 447 return tgt; 448 } 449 450 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanIntent> src) throws FHIRException { 451 if (src == null || src.isEmpty()) 452 return null; 453 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntentEnumFactory()); 454 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 455 switch (src.getValue()) { 456 case OPTION: 457 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent.OPTION); 458 break; 459 case ORDER: 460 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent.ORDER); 461 break; 462 case PLAN: 463 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent.PLAN); 464 break; 465 case PROPOSAL: 466 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent.PROPOSAL); 467 break; 468 default: 469 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent.NULL); 470 break; 471 } 472 return tgt; 473 } 474 475 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanStatus> src) throws FHIRException { 476 if (src == null || src.isEmpty()) 477 return null; 478 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatusEnumFactory()); 479 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 480 switch (src.getValue()) { 481 case ACTIVE: 482 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.ACTIVE); 483 break; 484 case REVOKED: 485 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.CANCELLED); 486 break; 487 case COMPLETED: 488 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.COMPLETED); 489 break; 490 case DRAFT: 491 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.DRAFT); 492 break; 493 case ENTEREDINERROR: 494 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.ENTEREDINERROR); 495 break; 496 case ONHOLD: 497 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.SUSPENDED); 498 break; 499 case UNKNOWN: 500 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.UNKNOWN); 501 break; 502 default: 503 tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus.NULL); 504 break; 505 } 506 return tgt; 507 } 508 509 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> src) throws FHIRException { 510 if (src == null || src.isEmpty()) 511 return null; 512 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CarePlan.CarePlanStatusEnumFactory()); 513 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 514 switch (src.getValue()) { 515 case ACTIVE: 516 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.ACTIVE); 517 break; 518 case CANCELLED: 519 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.REVOKED); 520 break; 521 case COMPLETED: 522 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.COMPLETED); 523 break; 524 case DRAFT: 525 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.DRAFT); 526 break; 527 case ENTEREDINERROR: 528 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.ENTEREDINERROR); 529 break; 530 case SUSPENDED: 531 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.ONHOLD); 532 break; 533 case UNKNOWN: 534 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.UNKNOWN); 535 break; 536 default: 537 tgt.setValue(org.hl7.fhir.r4.model.CarePlan.CarePlanStatus.NULL); 538 break; 539 } 540 return tgt; 541 } 542}