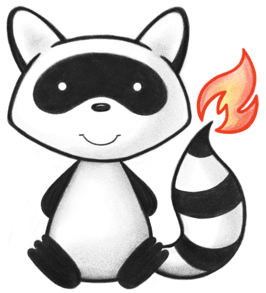
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class CareTeam30_40 { 013 014 public static org.hl7.fhir.dstu3.model.CareTeam convertCareTeam(org.hl7.fhir.r4.model.CareTeam src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.dstu3.model.CareTeam tgt = new org.hl7.fhir.dstu3.model.CareTeam(); 018 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 021 if (src.hasStatus()) 022 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 023 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 024 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 025 if (src.hasName()) 026 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 027 if (src.hasSubject()) 028 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 029 if (src.hasEncounter()) 030 tgt.setContext(Reference30_40.convertReference(src.getEncounter())); 031 if (src.hasPeriod()) 032 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 033 for (org.hl7.fhir.r4.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 034 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 035 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 036 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 037 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 038 tgt.addReasonReference(Reference30_40.convertReference(t)); 039 for (org.hl7.fhir.r4.model.Reference t : src.getManagingOrganization()) 040 tgt.addManagingOrganization(Reference30_40.convertReference(t)); 041 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 042 return tgt; 043 } 044 045 public static org.hl7.fhir.r4.model.CareTeam convertCareTeam(org.hl7.fhir.dstu3.model.CareTeam src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r4.model.CareTeam tgt = new org.hl7.fhir.r4.model.CareTeam(); 049 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 052 if (src.hasStatus()) 053 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 054 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 055 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 056 if (src.hasName()) 057 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 058 if (src.hasSubject()) 059 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 060 if (src.hasContext()) 061 tgt.setEncounter(Reference30_40.convertReference(src.getContext())); 062 if (src.hasPeriod()) 063 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 064 for (org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 065 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 066 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) 067 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 068 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) 069 tgt.addReasonReference(Reference30_40.convertReference(t)); 070 for (org.hl7.fhir.dstu3.model.Reference t : src.getManagingOrganization()) 071 tgt.addManagingOrganization(Reference30_40.convertReference(t)); 072 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.r4.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent(); 080 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 081 if (src.hasRole()) 082 tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getRoleFirstRep())); 083 if (src.hasMember()) 084 tgt.setMember(Reference30_40.convertReference(src.getMember())); 085 if (src.hasOnBehalfOf()) 086 tgt.setOnBehalfOf(Reference30_40.convertReference(src.getOnBehalfOf())); 087 if (src.hasPeriod()) 088 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 089 return tgt; 090 } 091 092 public static org.hl7.fhir.r4.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 093 if (src == null) 094 return null; 095 org.hl7.fhir.r4.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.r4.model.CareTeam.CareTeamParticipantComponent(); 096 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 097 if (src.hasRole()) 098 tgt.addRole(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 099 if (src.hasMember()) 100 tgt.setMember(Reference30_40.convertReference(src.getMember())); 101 if (src.hasOnBehalfOf()) 102 tgt.setOnBehalfOf(Reference30_40.convertReference(src.getOnBehalfOf())); 103 if (src.hasPeriod()) 104 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 105 return tgt; 106 } 107 108 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CareTeam.CareTeamStatus> src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatusEnumFactory()); 112 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 113 switch (src.getValue()) { 114 case PROPOSED: 115 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.PROPOSED); 116 break; 117 case ACTIVE: 118 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.ACTIVE); 119 break; 120 case SUSPENDED: 121 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.SUSPENDED); 122 break; 123 case INACTIVE: 124 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.INACTIVE); 125 break; 126 case ENTEREDINERROR: 127 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.ENTEREDINERROR); 128 break; 129 default: 130 tgt.setValue(org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus.NULL); 131 break; 132 } 133 return tgt; 134 } 135 136 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus> src) throws FHIRException { 137 if (src == null || src.isEmpty()) 138 return null; 139 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CareTeam.CareTeamStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CareTeam.CareTeamStatusEnumFactory()); 140 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 141 switch (src.getValue()) { 142 case PROPOSED: 143 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.PROPOSED); 144 break; 145 case ACTIVE: 146 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.ACTIVE); 147 break; 148 case SUSPENDED: 149 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.SUSPENDED); 150 break; 151 case INACTIVE: 152 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.INACTIVE); 153 break; 154 case ENTEREDINERROR: 155 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.ENTEREDINERROR); 156 break; 157 default: 158 tgt.setValue(org.hl7.fhir.r4.model.CareTeam.CareTeamStatus.NULL); 159 break; 160 } 161 return tgt; 162 } 163}