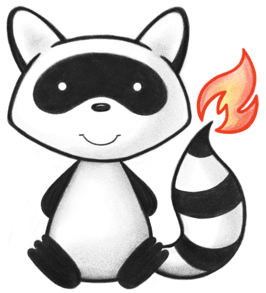
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class ConceptMap30_40 { 017 018 public static org.hl7.fhir.dstu3.model.ConceptMap convertConceptMap(org.hl7.fhir.r4.model.ConceptMap src) throws FHIRException { 019 if (src == null) 020 return null; 021 org.hl7.fhir.dstu3.model.ConceptMap tgt = new org.hl7.fhir.dstu3.model.ConceptMap(); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 023 if (src.hasUrl()) 024 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 025 if (src.hasIdentifier()) 026 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 027 if (src.hasVersion()) 028 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 029 if (src.hasName()) 030 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 031 if (src.hasTitle()) 032 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 033 if (src.hasStatus()) 034 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 035 if (src.hasExperimental()) 036 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 037 if (src.hasDateElement()) 038 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 039 if (src.hasPublisher()) 040 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 041 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 042 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 043 if (src.hasDescription()) 044 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 045 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 046 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 047 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 048 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 049 if (src.hasPurpose()) 050 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 051 if (src.hasCopyright()) 052 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 053 if (src.hasSource()) 054 tgt.setSource(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSource())); 055 if (src.hasTarget()) 056 tgt.setTarget(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getTarget())); 057 for (org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent t : src.getGroup()) 058 tgt.addGroup(convertConceptMapGroupComponent(t)); 059 return tgt; 060 } 061 062 public static org.hl7.fhir.r4.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu3.model.ConceptMap src) throws FHIRException { 063 if (src == null) 064 return null; 065 org.hl7.fhir.r4.model.ConceptMap tgt = new org.hl7.fhir.r4.model.ConceptMap(); 066 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 067 if (src.hasUrl()) 068 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 069 if (src.hasIdentifier()) 070 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 071 if (src.hasVersion()) 072 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 073 if (src.hasName()) 074 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 075 if (src.hasTitle()) 076 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 077 if (src.hasStatus()) 078 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 079 if (src.hasExperimental()) 080 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 081 if (src.hasDateElement()) 082 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 083 if (src.hasPublisher()) 084 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 085 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 086 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 087 if (src.hasDescription()) 088 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 089 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 090 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 091 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 092 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 093 if (src.hasPurpose()) 094 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 095 if (src.hasCopyright()) 096 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 097 if (src.hasSource()) { 098 org.hl7.fhir.r4.model.Type t = ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSource()); 099 tgt.setSource(t instanceof org.hl7.fhir.r4.model.Reference ? new org.hl7.fhir.r4.model.CanonicalType(((org.hl7.fhir.r4.model.Reference) t).getReference()) : t); 100 } 101 if (src.hasTarget()) { 102 org.hl7.fhir.r4.model.Type t = ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getTarget()); 103 tgt.setTarget(t instanceof org.hl7.fhir.r4.model.Reference ? new org.hl7.fhir.r4.model.CanonicalType(((org.hl7.fhir.r4.model.Reference) t).getReference()) : t); 104 } 105 for (org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent t : src.getGroup()) 106 tgt.addGroup(convertConceptMapGroupComponent(t)); 107 return tgt; 108 } 109 110 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 114 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 115 switch (src.getValue()) { 116 case RELATEDTO: 117 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.RELATEDTO); 118 break; 119 case EQUIVALENT: 120 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 121 break; 122 case EQUAL: 123 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.EQUAL); 124 break; 125 case WIDER: 126 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.WIDER); 127 break; 128 case SUBSUMES: 129 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.SUBSUMES); 130 break; 131 case NARROWER: 132 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.NARROWER); 133 break; 134 case SPECIALIZES: 135 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.SPECIALIZES); 136 break; 137 case INEXACT: 138 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.INEXACT); 139 break; 140 case UNMATCHED: 141 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.UNMATCHED); 142 break; 143 case DISJOINT: 144 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.DISJOINT); 145 break; 146 default: 147 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.NULL); 148 break; 149 } 150 return tgt; 151 } 152 153 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 154 if (src == null || src.isEmpty()) 155 return null; 156 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 157 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 158 switch (src.getValue()) { 159 case RELATEDTO: 160 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.RELATEDTO); 161 break; 162 case EQUIVALENT: 163 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 164 break; 165 case EQUAL: 166 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.EQUAL); 167 break; 168 case WIDER: 169 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.WIDER); 170 break; 171 case SUBSUMES: 172 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.SUBSUMES); 173 break; 174 case NARROWER: 175 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.NARROWER); 176 break; 177 case SPECIALIZES: 178 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.SPECIALIZES); 179 break; 180 case INEXACT: 181 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.INEXACT); 182 break; 183 case UNMATCHED: 184 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.UNMATCHED); 185 break; 186 case DISJOINT: 187 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.DISJOINT); 188 break; 189 default: 190 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.NULL); 191 break; 192 } 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent convertConceptMapGroupComponent(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent src) throws FHIRException { 197 if (src == null) 198 return null; 199 org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent(); 200 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 201 if (src.hasSource()) 202 tgt.setSourceElement(Uri30_40.convertUri(src.getSourceElement())); 203 if (src.hasSourceVersion()) 204 tgt.setSourceVersionElement(String30_40.convertString(src.getSourceVersionElement())); 205 if (src.hasTarget()) 206 tgt.setTarget(src.getTarget()); 207 if (src.hasTargetVersion()) 208 tgt.setTargetVersion(src.getTargetVersion()); 209 for (org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent t : src.getElement()) 210 tgt.addElement(convertSourceElementComponent(t)); 211 if (src.hasUnmapped()) 212 tgt.setUnmapped(convertConceptMapGroupUnmappedComponent(src.getUnmapped())); 213 return tgt; 214 } 215 216 public static org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent convertConceptMapGroupComponent(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent src) throws FHIRException { 217 if (src == null) 218 return null; 219 org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent(); 220 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 221 if (src.hasSource()) 222 tgt.setSourceElement(Uri30_40.convertUri(src.getSourceElement())); 223 if (src.hasSourceVersion()) 224 tgt.setSourceVersionElement(String30_40.convertString(src.getSourceVersionElement())); 225 if (src.hasTarget()) 226 tgt.setTarget(src.getTarget()); 227 if (src.hasTargetVersion()) 228 tgt.setTargetVersion(src.getTargetVersion()); 229 for (org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent t : src.getElement()) 230 tgt.addElement(convertSourceElementComponent(t)); 231 if (src.hasUnmapped()) 232 tgt.setUnmapped(convertConceptMapGroupUnmappedComponent(src.getUnmapped())); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent convertConceptMapGroupUnmappedComponent(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedComponent src) throws FHIRException { 237 if (src == null) 238 return null; 239 org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent(); 240 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 241 if (src.hasMode()) 242 tgt.setModeElement(convertConceptMapGroupUnmappedMode(src.getModeElement())); 243 if (src.hasCode()) 244 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 245 if (src.hasDisplay()) 246 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 247 if (src.hasUrl()) 248 tgt.setUrl(src.getUrl()); 249 return tgt; 250 } 251 252 public static org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedComponent convertConceptMapGroupUnmappedComponent(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent src) throws FHIRException { 253 if (src == null) 254 return null; 255 org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedComponent(); 256 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 257 if (src.hasMode()) 258 tgt.setModeElement(convertConceptMapGroupUnmappedMode(src.getModeElement())); 259 if (src.hasCode()) 260 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 261 if (src.hasDisplay()) 262 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 263 if (src.hasUrl()) 264 tgt.setUrl(src.getUrl()); 265 return tgt; 266 } 267 268 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> convertConceptMapGroupUnmappedMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode> src) throws FHIRException { 269 if (src == null || src.isEmpty()) 270 return null; 271 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedModeEnumFactory()); 272 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 273 switch (src.getValue()) { 274 case PROVIDED: 275 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.PROVIDED); 276 break; 277 case FIXED: 278 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.FIXED); 279 break; 280 case OTHERMAP: 281 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.OTHERMAP); 282 break; 283 default: 284 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.NULL); 285 break; 286 } 287 return tgt; 288 } 289 290 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode> convertConceptMapGroupUnmappedMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> src) throws FHIRException { 291 if (src == null || src.isEmpty()) 292 return null; 293 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedModeEnumFactory()); 294 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 295 switch (src.getValue()) { 296 case PROVIDED: 297 tgt.setValue(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode.PROVIDED); 298 break; 299 case FIXED: 300 tgt.setValue(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode.FIXED); 301 break; 302 case OTHERMAP: 303 tgt.setValue(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode.OTHERMAP); 304 break; 305 default: 306 tgt.setValue(org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupUnmappedMode.NULL); 307 break; 308 } 309 return tgt; 310 } 311 312 public static org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent src) throws FHIRException { 313 if (src == null) 314 return null; 315 org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent(); 316 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 317 if (src.hasProperty()) 318 tgt.setPropertyElement(Uri30_40.convertUri(src.getPropertyElement())); 319 if (src.hasSystem()) 320 tgt.setSystem(src.getSystem()); 321 if (src.hasValue()) 322 tgt.setCodeElement(String30_40.convertString(src.getValueElement())); 323 if (src.hasDisplay()) 324 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 325 return tgt; 326 } 327 328 public static org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent src) throws FHIRException { 329 if (src == null) 330 return null; 331 org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent(); 332 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 333 if (src.hasProperty()) 334 tgt.setPropertyElement(Uri30_40.convertUri(src.getPropertyElement())); 335 if (src.hasSystem()) 336 tgt.setSystem(src.getSystem()); 337 if (src.hasCode()) 338 tgt.setValueElement(String30_40.convertString(src.getCodeElement())); 339 if (src.hasDisplay()) 340 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 341 return tgt; 342 } 343 344 public static org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent src) throws FHIRException { 345 if (src == null) 346 return null; 347 org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent(); 348 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 349 if (src.hasCode()) 350 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 351 if (src.hasDisplay()) 352 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 353 for (org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent t : src.getTarget()) 354 tgt.addTarget(convertTargetElementComponent(t)); 355 return tgt; 356 } 357 358 public static org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent src) throws FHIRException { 359 if (src == null) 360 return null; 361 org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent(); 362 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 363 if (src.hasCode()) 364 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 365 if (src.hasDisplay()) 366 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 367 for (org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent t : src.getTarget()) 368 tgt.addTarget(convertTargetElementComponent(t)); 369 return tgt; 370 } 371 372 public static org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent src) throws FHIRException { 373 if (src == null) 374 return null; 375 org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent(); 376 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 377 if (src.hasCode()) 378 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 379 if (src.hasDisplay()) 380 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 381 if (src.hasEquivalence()) 382 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 383 if (src.hasComment()) 384 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 385 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 386 tgt.addDependsOn(convertOtherElementComponent(t)); 387 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getProduct()) 388 tgt.addProduct(convertOtherElementComponent(t)); 389 return tgt; 390 } 391 392 public static org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent src) throws FHIRException { 393 if (src == null) 394 return null; 395 org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent(); 396 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 397 if (src.hasCode()) 398 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 399 if (src.hasDisplay()) 400 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 401 if (src.hasEquivalence()) 402 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 403 if (src.hasComment()) 404 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 405 for (org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 406 tgt.addDependsOn(convertOtherElementComponent(t)); 407 for (org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent t : src.getProduct()) 408 tgt.addProduct(convertOtherElementComponent(t)); 409 return tgt; 410 } 411}