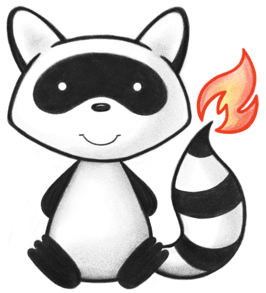
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.VersionConvertor_30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4.model.CodeableConcept; 016import org.hl7.fhir.r4.model.Identifier; 017 018public class Consent30_40 { 019 020 static public org.hl7.fhir.r4.model.Consent convertConsent(org.hl7.fhir.dstu3.model.Consent src) throws FHIRException { 021 if (src == null) 022 return null; 023 org.hl7.fhir.r4.model.Consent tgt = new org.hl7.fhir.r4.model.Consent(); 024 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 025 if (src.hasIdentifier()) 026 tgt.addIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 027 if (src.hasStatus()) 028 tgt.setStatusElement(convertConsentState(src.getStatusElement())); 029 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 030 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 031 if (src.hasPatient()) 032 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 033 if (src.hasDateTime()) 034 tgt.setDateTimeElement(DateTime30_40.convertDateTime(src.getDateTimeElement())); 035 for (org.hl7.fhir.dstu3.model.Reference t : src.getConsentingParty()) 036 tgt.addPerformer(Reference30_40.convertReference(t)); 037 for (org.hl7.fhir.dstu3.model.Reference t : src.getOrganization()) 038 tgt.addOrganization(Reference30_40.convertReference(t)); 039 if (src.hasSource()) 040 tgt.setSource(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSource())); 041 for (org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent t : src.getPolicy()) 042 tgt.addPolicy(convertConsentPolicyComponent(t)); 043 if (src.hasPolicyRule()) { 044 org.hl7.fhir.r4.model.Coding c = new org.hl7.fhir.r4.model.Coding(); 045 c.setSystem(VersionConvertor_30_40.URN_IETF_RFC_3986); 046 c.setCode(src.getPolicyRule()); 047 tgt.setPolicyRule(new CodeableConcept(c)); 048 } 049 if (src.hasSecurityLabel() || src.hasPeriod() || src.hasActor() || src.hasAction() || src.hasPurpose() || src.hasDataPeriod() || src.hasData() || src.hasExcept()) { 050 org.hl7.fhir.r4.model.Consent.provisionComponent pc = new org.hl7.fhir.r4.model.Consent.provisionComponent(); 051 if (src.hasPeriod()) 052 pc.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 053 for (org.hl7.fhir.dstu3.model.Consent.ConsentActorComponent t : src.getActor()) 054 pc.addActor(convertConsentActorComponent(t)); 055 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getAction()) 056 pc.addAction(CodeableConcept30_40.convertCodeableConcept(t)); 057 for (org.hl7.fhir.dstu3.model.Coding t : src.getSecurityLabel()) 058 pc.addSecurityLabel(Coding30_40.convertCoding(t)); 059 for (org.hl7.fhir.dstu3.model.Coding t : src.getPurpose()) pc.addPurpose(Coding30_40.convertCoding(t)); 060 if (src.hasDataPeriod()) 061 pc.setDataPeriod(Period30_40.convertPeriod(src.getDataPeriod())); 062 for (org.hl7.fhir.dstu3.model.Consent.ConsentDataComponent t : src.getData()) 063 pc.addData(convertConsentDataComponent(t)); 064 for (org.hl7.fhir.dstu3.model.Consent.ExceptComponent t : src.getExcept()) 065 pc.addProvision(convertExceptComponent(t)); 066 tgt.setProvision(pc); 067 } 068 return tgt; 069 } 070 071 static public org.hl7.fhir.dstu3.model.Consent convertConsent(org.hl7.fhir.r4.model.Consent src) throws FHIRException { 072 if (src == null) 073 return null; 074 org.hl7.fhir.dstu3.model.Consent tgt = new org.hl7.fhir.dstu3.model.Consent(); 075 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 076 List<Identifier> identifier = src.getIdentifier(); 077 if (identifier.size() > 0) { 078 tgt.setIdentifier(Identifier30_40.convertIdentifier(identifier.get(0))); 079 if (identifier.size() > 1) { 080 } 081 } 082 if (src.hasStatus()) 083 tgt.setStatusElement(convertConsentState(src.getStatusElement())); 084 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 085 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 086 if (src.hasPatient()) 087 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 088 if (src.hasDateTime()) 089 tgt.setDateTimeElement(DateTime30_40.convertDateTime(src.getDateTimeElement())); 090 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) 091 tgt.addConsentingParty(Reference30_40.convertReference(t)); 092 for (org.hl7.fhir.r4.model.Reference t : src.getOrganization()) 093 tgt.addOrganization(Reference30_40.convertReference(t)); 094 if (src.hasSource()) 095 tgt.setSource(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSource())); 096 for (org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent t : src.getPolicy()) 097 tgt.addPolicy(convertConsentPolicyComponent(t)); 098 if (src.hasPolicyRule()) { 099 for (org.hl7.fhir.r4.model.Coding c : src.getPolicyRule().getCoding()) { 100 if (VersionConvertor_30_40.URN_IETF_RFC_3986.equals(c.getSystem())) { 101 tgt.setPolicyRule(c.getCode()); 102 break; 103 } 104 } 105 } 106 if (src.hasProvision()) { 107 org.hl7.fhir.r4.model.Consent.provisionComponent p = src.getProvision(); 108 if (p.hasPeriod()) 109 tgt.setPeriod(Period30_40.convertPeriod(p.getPeriod())); 110 for (org.hl7.fhir.r4.model.Consent.provisionActorComponent t : p.getActor()) 111 tgt.addActor(convertConsentActorComponent(t)); 112 for (org.hl7.fhir.r4.model.CodeableConcept t : p.getAction()) 113 tgt.addAction(CodeableConcept30_40.convertCodeableConcept(t)); 114 for (org.hl7.fhir.r4.model.Coding t : p.getSecurityLabel()) tgt.addSecurityLabel(Coding30_40.convertCoding(t)); 115 for (org.hl7.fhir.r4.model.Coding t : p.getPurpose()) tgt.addPurpose(Coding30_40.convertCoding(t)); 116 if (p.hasDataPeriod()) 117 tgt.setDataPeriod(Period30_40.convertPeriod(p.getDataPeriod())); 118 for (org.hl7.fhir.r4.model.Consent.provisionDataComponent t : p.getData()) 119 tgt.addData(convertConsentDataComponent(t)); 120 for (org.hl7.fhir.r4.model.Consent.provisionComponent t : p.getProvision()) 121 tgt.addExcept(convertExceptComponent(t)); 122 } 123 return tgt; 124 } 125 126 static public org.hl7.fhir.dstu3.model.Consent.ConsentActorComponent convertConsentActorComponent(org.hl7.fhir.r4.model.Consent.provisionActorComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.dstu3.model.Consent.ConsentActorComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ConsentActorComponent(); 130 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 131 if (src.hasRole()) 132 tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 133 if (src.hasReference()) 134 tgt.setReference(Reference30_40.convertReference(src.getReference())); 135 return tgt; 136 } 137 138 static public org.hl7.fhir.r4.model.Consent.provisionActorComponent convertConsentActorComponent(org.hl7.fhir.dstu3.model.Consent.ConsentActorComponent src) throws FHIRException { 139 if (src == null) 140 return null; 141 org.hl7.fhir.r4.model.Consent.provisionActorComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionActorComponent(); 142 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 143 if (src.hasRole()) 144 tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 145 if (src.hasReference()) 146 tgt.setReference(Reference30_40.convertReference(src.getReference())); 147 return tgt; 148 } 149 150 static public org.hl7.fhir.r4.model.Consent.provisionDataComponent convertConsentDataComponent(org.hl7.fhir.dstu3.model.Consent.ConsentDataComponent src) throws FHIRException { 151 if (src == null) 152 return null; 153 org.hl7.fhir.r4.model.Consent.provisionDataComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionDataComponent(); 154 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 155 if (src.hasMeaning()) 156 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 157 if (src.hasReference()) 158 tgt.setReference(Reference30_40.convertReference(src.getReference())); 159 return tgt; 160 } 161 162 static public org.hl7.fhir.dstu3.model.Consent.ConsentDataComponent convertConsentDataComponent(org.hl7.fhir.r4.model.Consent.provisionDataComponent src) throws FHIRException { 163 if (src == null) 164 return null; 165 org.hl7.fhir.dstu3.model.Consent.ConsentDataComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ConsentDataComponent(); 166 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 167 if (src.hasMeaning()) 168 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 169 if (src.hasReference()) 170 tgt.setReference(Reference30_40.convertReference(src.getReference())); 171 return tgt; 172 } 173 174 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentDataMeaningEnumFactory()); 178 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 179 switch (src.getValue()) { 180 case INSTANCE: 181 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.INSTANCE); 182 break; 183 case RELATED: 184 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.RELATED); 185 break; 186 case DEPENDENTS: 187 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.DEPENDENTS); 188 break; 189 case AUTHOREDBY: 190 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.AUTHOREDBY); 191 break; 192 default: 193 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.NULL); 194 break; 195 } 196 return tgt; 197 } 198 199 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> src) throws FHIRException { 200 if (src == null || src.isEmpty()) 201 return null; 202 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaningEnumFactory()); 203 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 204 switch (src.getValue()) { 205 case INSTANCE: 206 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.INSTANCE); 207 break; 208 case RELATED: 209 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.RELATED); 210 break; 211 case DEPENDENTS: 212 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.DEPENDENTS); 213 break; 214 case AUTHOREDBY: 215 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.AUTHOREDBY); 216 break; 217 default: 218 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.NULL); 219 break; 220 } 221 return tgt; 222 } 223 224 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> convertConsentExceptType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentExceptType> src) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentProvisionTypeEnumFactory()); 228 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 229 switch (src.getValue()) { 230 case DENY: 231 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.DENY); 232 break; 233 case PERMIT: 234 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.PERMIT); 235 break; 236 default: 237 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.NULL); 238 break; 239 } 240 return tgt; 241 } 242 243 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentExceptType> convertConsentExceptType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentExceptType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Consent.ConsentExceptTypeEnumFactory()); 247 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 248 switch (src.getValue()) { 249 case DENY: 250 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentExceptType.DENY); 251 break; 252 case PERMIT: 253 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentExceptType.PERMIT); 254 break; 255 default: 256 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentExceptType.NULL); 257 break; 258 } 259 return tgt; 260 } 261 262 static public org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent convertConsentPolicyComponent(org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent src) throws FHIRException { 263 if (src == null) 264 return null; 265 org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent(); 266 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 267 if (src.hasAuthority()) 268 tgt.setAuthorityElement(Uri30_40.convertUri(src.getAuthorityElement())); 269 if (src.hasUri()) 270 tgt.setUriElement(Uri30_40.convertUri(src.getUriElement())); 271 return tgt; 272 } 273 274 static public org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent convertConsentPolicyComponent(org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent src) throws FHIRException { 275 if (src == null) 276 return null; 277 org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent tgt = new org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent(); 278 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 279 if (src.hasAuthority()) 280 tgt.setAuthorityElement(Uri30_40.convertUri(src.getAuthorityElement())); 281 if (src.hasUri()) 282 tgt.setUriElement(Uri30_40.convertUri(src.getUriElement())); 283 return tgt; 284 } 285 286 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentState> convertConsentState(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> src) throws FHIRException { 287 if (src == null || src.isEmpty()) 288 return null; 289 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentState> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Consent.ConsentStateEnumFactory()); 290 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 291 switch (src.getValue()) { 292 case DRAFT: 293 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.DRAFT); 294 break; 295 case PROPOSED: 296 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.PROPOSED); 297 break; 298 case ACTIVE: 299 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.ACTIVE); 300 break; 301 case REJECTED: 302 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.REJECTED); 303 break; 304 case INACTIVE: 305 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.INACTIVE); 306 break; 307 case ENTEREDINERROR: 308 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.ENTEREDINERROR); 309 break; 310 default: 311 tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentState.NULL); 312 break; 313 } 314 return tgt; 315 } 316 317 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> convertConsentState(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentState> src) throws FHIRException { 318 if (src == null || src.isEmpty()) 319 return null; 320 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentStateEnumFactory()); 321 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 322 switch (src.getValue()) { 323 case DRAFT: 324 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.DRAFT); 325 break; 326 case PROPOSED: 327 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.PROPOSED); 328 break; 329 case ACTIVE: 330 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.ACTIVE); 331 break; 332 case REJECTED: 333 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.REJECTED); 334 break; 335 case INACTIVE: 336 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.INACTIVE); 337 break; 338 case ENTEREDINERROR: 339 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.ENTEREDINERROR); 340 break; 341 default: 342 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.NULL); 343 break; 344 } 345 return tgt; 346 } 347 348 static public org.hl7.fhir.dstu3.model.Consent.ExceptActorComponent convertExceptActorComponent(org.hl7.fhir.r4.model.Consent.provisionActorComponent src) throws FHIRException { 349 if (src == null) 350 return null; 351 org.hl7.fhir.dstu3.model.Consent.ExceptActorComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ExceptActorComponent(); 352 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 353 if (src.hasRole()) 354 tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 355 if (src.hasReference()) 356 tgt.setReference(Reference30_40.convertReference(src.getReference())); 357 return tgt; 358 } 359 360 static public org.hl7.fhir.r4.model.Consent.provisionActorComponent convertExceptActorComponent(org.hl7.fhir.dstu3.model.Consent.ExceptActorComponent src) throws FHIRException { 361 if (src == null) 362 return null; 363 org.hl7.fhir.r4.model.Consent.provisionActorComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionActorComponent(); 364 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 365 if (src.hasRole()) 366 tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 367 if (src.hasReference()) 368 tgt.setReference(Reference30_40.convertReference(src.getReference())); 369 return tgt; 370 } 371 372 static public org.hl7.fhir.dstu3.model.Consent.ExceptComponent convertExceptComponent(org.hl7.fhir.r4.model.Consent.provisionComponent src) throws FHIRException { 373 if (src == null) 374 return null; 375 org.hl7.fhir.dstu3.model.Consent.ExceptComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ExceptComponent(); 376 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 377 if (src.hasType()) 378 tgt.setTypeElement(convertConsentExceptType(src.getTypeElement())); 379 if (src.hasPeriod()) 380 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 381 for (org.hl7.fhir.r4.model.Consent.provisionActorComponent t : src.getActor()) 382 tgt.addActor(convertExceptActorComponent(t)); 383 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getAction()) 384 tgt.addAction(CodeableConcept30_40.convertCodeableConcept(t)); 385 for (org.hl7.fhir.r4.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding30_40.convertCoding(t)); 386 for (org.hl7.fhir.r4.model.Coding t : src.getPurpose()) tgt.addPurpose(Coding30_40.convertCoding(t)); 387 for (org.hl7.fhir.r4.model.Coding t : src.getClass_()) tgt.addClass_(Coding30_40.convertCoding(t)); 388 for (CodeableConcept t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 389 if (src.hasDataPeriod()) 390 tgt.setDataPeriod(Period30_40.convertPeriod(src.getDataPeriod())); 391 for (org.hl7.fhir.r4.model.Consent.provisionDataComponent t : src.getData()) 392 tgt.addData(convertExceptDataComponent(t)); 393 return tgt; 394 } 395 396 static public org.hl7.fhir.r4.model.Consent.provisionComponent convertExceptComponent(org.hl7.fhir.dstu3.model.Consent.ExceptComponent src) throws FHIRException { 397 if (src == null) 398 return null; 399 org.hl7.fhir.r4.model.Consent.provisionComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionComponent(); 400 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 401 if (src.hasType()) 402 tgt.setTypeElement(convertConsentExceptType(src.getTypeElement())); 403 if (src.hasPeriod()) 404 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 405 for (org.hl7.fhir.dstu3.model.Consent.ExceptActorComponent t : src.getActor()) 406 tgt.addActor(convertExceptActorComponent(t)); 407 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getAction()) 408 tgt.addAction(CodeableConcept30_40.convertCodeableConcept(t)); 409 for (org.hl7.fhir.dstu3.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding30_40.convertCoding(t)); 410 for (org.hl7.fhir.dstu3.model.Coding t : src.getPurpose()) tgt.addPurpose(Coding30_40.convertCoding(t)); 411 for (org.hl7.fhir.dstu3.model.Coding t : src.getClass_()) tgt.addClass_(Coding30_40.convertCoding(t)); 412 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) 413 tgt.addCode(new CodeableConcept(Coding30_40.convertCoding(t))); 414 if (src.hasDataPeriod()) 415 tgt.setDataPeriod(Period30_40.convertPeriod(src.getDataPeriod())); 416 for (org.hl7.fhir.dstu3.model.Consent.ExceptDataComponent t : src.getData()) 417 tgt.addData(convertExceptDataComponent(t)); 418 return tgt; 419 } 420 421 static public org.hl7.fhir.dstu3.model.Consent.ExceptDataComponent convertExceptDataComponent(org.hl7.fhir.r4.model.Consent.provisionDataComponent src) throws FHIRException { 422 if (src == null) 423 return null; 424 org.hl7.fhir.dstu3.model.Consent.ExceptDataComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ExceptDataComponent(); 425 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 426 if (src.hasMeaning()) 427 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 428 if (src.hasReference()) 429 tgt.setReference(Reference30_40.convertReference(src.getReference())); 430 return tgt; 431 } 432 433 static public org.hl7.fhir.r4.model.Consent.provisionDataComponent convertExceptDataComponent(org.hl7.fhir.dstu3.model.Consent.ExceptDataComponent src) throws FHIRException { 434 if (src == null) 435 return null; 436 org.hl7.fhir.r4.model.Consent.provisionDataComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionDataComponent(); 437 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 438 if (src.hasMeaning()) 439 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 440 if (src.hasReference()) 441 tgt.setReference(Reference30_40.convertReference(src.getReference())); 442 return tgt; 443 } 444}