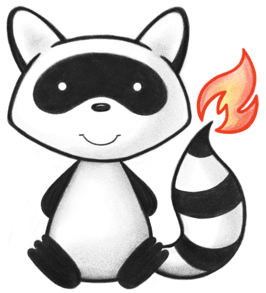
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003 004import java.util.Collections; 005import java.util.stream.Collectors; 006 007import org.hl7.fhir.convertors.context.ConversionContext30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Base64Binary30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 017import org.hl7.fhir.dstu3.model.Device; 018import org.hl7.fhir.exceptions.FHIRException; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class Device30_40 { 050 051 public static org.hl7.fhir.r4.model.Device convertDevice(org.hl7.fhir.dstu3.model.Device src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r4.model.Device tgt = new org.hl7.fhir.r4.model.Device(); 055 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 058 if (src.hasUdi()) { 059 org.hl7.fhir.r4.model.Device.DeviceUdiCarrierComponent carrierComponent = tgt.getUdiCarrierFirstRep(); 060 carrierComponent.setDeviceIdentifierElement(String30_40.convertString(src.getUdi().getDeviceIdentifierElement())); 061 carrierComponent.setJurisdictionElement(Uri30_40.convertUri(src.getUdi().getJurisdictionElement())); 062 carrierComponent.setCarrierHRFElement(String30_40.convertString(src.getUdi().getCarrierHRFElement())); 063 carrierComponent.setCarrierAIDCElement(Base64Binary30_40.convertBase64Binary(src.getUdi().getCarrierAIDCElement())); 064 carrierComponent.setIssuerElement(Uri30_40.convertUri(src.getUdi().getIssuerElement())); 065 carrierComponent.setEntryTypeElement(convertUDIEntryType(src.getUdi().getEntryTypeElement())); 066 tgt.setUdiCarrier(Collections.singletonList(carrierComponent)); 067 org.hl7.fhir.r4.model.Device.DeviceDeviceNameComponent nameComponent = tgt.getDeviceNameFirstRep(); 068 nameComponent.setNameElement(String30_40.convertString(src.getUdi().getNameElement())); 069 nameComponent.setType(org.hl7.fhir.r4.model.Device.DeviceNameType.UDILABELNAME); 070 } 071 if (src.hasStatus()) 072 tgt.setStatusElement(convertFHIRDeviceStatus(src.getStatusElement())); 073 if (src.hasType()) 074 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 075 if (src.hasLotNumber()) 076 tgt.setLotNumberElement(String30_40.convertString(src.getLotNumberElement())); 077 if (src.hasManufacturer()) 078 tgt.setManufacturerElement(String30_40.convertString(src.getManufacturerElement())); 079 if (src.hasManufactureDate()) 080 tgt.setManufactureDateElement(DateTime30_40.convertDateTime(src.getManufactureDateElement())); 081 if (src.hasExpirationDate()) 082 tgt.setExpirationDateElement(DateTime30_40.convertDateTime(src.getExpirationDateElement())); 083 if (src.hasModelElement()) 084 tgt.setModelNumberElement(String30_40.convertString(src.getModelElement())); 085 if (src.hasVersionElement()) 086 tgt.setVersion(Collections.singletonList(tgt.getVersionFirstRep().setValueElement(String30_40.convertString(src.getVersionElement())))); 087 if (src.hasPatient()) 088 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 089 if (src.hasOwner()) 090 tgt.setOwner(Reference30_40.convertReference(src.getOwner())); 091 if (src.hasContact()) 092 tgt.setContact(src.getContact().stream().map(ContactPoint30_40::convertContactPoint).collect(Collectors.toList())); 093 if (src.hasLocation()) 094 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 095 if (src.hasUrl()) 096 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 097 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 098 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSafety()) 099 tgt.addSafety(CodeableConcept30_40.convertCodeableConcept(t)); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.dstu3.model.Device convertDevice(org.hl7.fhir.r4.model.Device src) throws FHIRException { 104 if (src == null) 105 return null; 106 org.hl7.fhir.dstu3.model.Device tgt = new org.hl7.fhir.dstu3.model.Device(); 107 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 108 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 109 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 110 if (src.hasUdiCarrier()) { 111 Device.DeviceUdiComponent udi = tgt.getUdi(); 112 udi.setDeviceIdentifierElement(String30_40.convertString(src.getUdiCarrierFirstRep().getDeviceIdentifierElement())); 113 udi.setJurisdictionElement(Uri30_40.convertUri(src.getUdiCarrierFirstRep().getJurisdictionElement())); 114 udi.setCarrierHRFElement(String30_40.convertString(src.getUdiCarrierFirstRep().getCarrierHRFElement())); 115 udi.setCarrierAIDCElement(Base64Binary30_40.convertBase64Binary(src.getUdiCarrierFirstRep().getCarrierAIDCElement())); 116 udi.setIssuerElement(Uri30_40.convertUri(src.getUdiCarrierFirstRep().getIssuerElement())); 117 udi.setEntryTypeElement(convertUDIEntryType(src.getUdiCarrierFirstRep().getEntryTypeElement())); 118 tgt.setUdi(udi); 119 } 120 if (src.hasStatus()) 121 tgt.setStatusElement(convertFHIRDeviceStatus(src.getStatusElement())); 122 if (src.hasType()) 123 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 124 if (src.hasLotNumber()) 125 tgt.setLotNumberElement(String30_40.convertString(src.getLotNumberElement())); 126 if (src.hasManufacturer()) 127 tgt.setManufacturerElement(String30_40.convertString(src.getManufacturerElement())); 128 if (src.hasManufactureDate()) 129 tgt.setManufactureDateElement(DateTime30_40.convertDateTime(src.getManufactureDateElement())); 130 if (src.hasExpirationDate()) 131 tgt.setExpirationDateElement(DateTime30_40.convertDateTime(src.getExpirationDateElement())); 132 if (src.hasModelNumber()) 133 tgt.setModel(src.getModelNumber()); 134 if (src.hasVersion()) 135 tgt.setVersionElement(String30_40.convertString(src.getVersion().get(0).getValueElement())); 136 if (src.hasDeviceName()) 137 tgt.setUdi(tgt.getUdi().setName(src.getDeviceName().get(0).getName())); 138 if (src.hasPatient()) 139 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 140 if (src.hasOwner()) 141 tgt.setOwner(Reference30_40.convertReference(src.getOwner())); 142 if (src.hasContact()) 143 tgt.setContact(src.getContact().stream().map(ContactPoint30_40::convertContactPoint).collect(Collectors.toList())); 144 if (src.hasLocation()) 145 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 146 for (org.hl7.fhir.r4.model.ContactPoint t : src.getContact()) 147 tgt.addContact(ContactPoint30_40.convertContactPoint(t)); 148 if (src.hasLocation()) 149 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 150 if (src.hasUrl()) 151 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 152 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 153 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSafety()) 154 tgt.addSafety(CodeableConcept30_40.convertCodeableConcept(t)); 155 return tgt; 156 } 157 158 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.FHIRDeviceStatus> convertFHIRDeviceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> src) throws FHIRException { 159 if (src == null || src.isEmpty()) 160 return null; 161 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.FHIRDeviceStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Device.FHIRDeviceStatusEnumFactory()); 162 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 163 switch (src.getValue()) { 164 case ACTIVE: 165 tgt.setValue(org.hl7.fhir.r4.model.Device.FHIRDeviceStatus.ACTIVE); 166 break; 167 case INACTIVE: 168 tgt.setValue(org.hl7.fhir.r4.model.Device.FHIRDeviceStatus.INACTIVE); 169 break; 170 case ENTEREDINERROR: 171 tgt.setValue(org.hl7.fhir.r4.model.Device.FHIRDeviceStatus.ENTEREDINERROR); 172 break; 173 case UNKNOWN: 174 tgt.setValue(org.hl7.fhir.r4.model.Device.FHIRDeviceStatus.UNKNOWN); 175 break; 176 default: 177 tgt.setValue(org.hl7.fhir.r4.model.Device.FHIRDeviceStatus.NULL); 178 break; 179 } 180 return tgt; 181 } 182 183 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> convertFHIRDeviceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.FHIRDeviceStatus> src) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatusEnumFactory()); 187 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 188 switch (src.getValue()) { 189 case ACTIVE: 190 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.ACTIVE); 191 break; 192 case INACTIVE: 193 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.INACTIVE); 194 break; 195 case ENTEREDINERROR: 196 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.ENTEREDINERROR); 197 break; 198 case UNKNOWN: 199 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.UNKNOWN); 200 break; 201 default: 202 tgt.setValue(org.hl7.fhir.dstu3.model.Device.FHIRDeviceStatus.NULL); 203 break; 204 } 205 return tgt; 206 } 207 208 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.UDIEntryType> convertUDIEntryType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.UDIEntryType> src) throws FHIRException { 209 if (src == null || src.isEmpty()) 210 return null; 211 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.UDIEntryType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Device.UDIEntryTypeEnumFactory()); 212 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 213 switch (src.getValue()) { 214 case BARCODE: 215 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.BARCODE); 216 break; 217 case RFID: 218 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.RFID); 219 break; 220 case MANUAL: 221 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.MANUAL); 222 break; 223 case CARD: 224 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.CARD); 225 break; 226 case SELFREPORTED: 227 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.SELFREPORTED); 228 break; 229 case UNKNOWN: 230 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.UNKNOWN); 231 break; 232 default: 233 tgt.setValue(org.hl7.fhir.r4.model.Device.UDIEntryType.NULL); 234 break; 235 } 236 return tgt; 237 } 238 239 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.UDIEntryType> convertUDIEntryType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Device.UDIEntryType> src) throws FHIRException { 240 if (src == null || src.isEmpty()) 241 return null; 242 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Device.UDIEntryType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Device.UDIEntryTypeEnumFactory()); 243 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 244 switch (src.getValue()) { 245 case BARCODE: 246 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.BARCODE); 247 break; 248 case RFID: 249 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.RFID); 250 break; 251 case MANUAL: 252 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.MANUAL); 253 break; 254 case CARD: 255 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.CARD); 256 break; 257 case SELFREPORTED: 258 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.SELFREPORTED); 259 break; 260 case UNKNOWN: 261 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.UNKNOWN); 262 break; 263 default: 264 tgt.setValue(org.hl7.fhir.dstu3.model.Device.UDIEntryType.NULL); 265 break; 266 } 267 return tgt; 268 } 269}