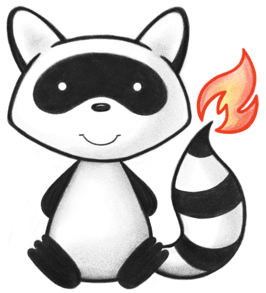
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Instant30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.DiagnosticReport; 012import org.hl7.fhir.r4.model.Enumeration; 013 014public class DiagnosticReport30_40 { 015 016 public static org.hl7.fhir.r4.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu3.model.DiagnosticReport src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r4.model.DiagnosticReport tgt = new org.hl7.fhir.r4.model.DiagnosticReport(); 020 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 023 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_40.convertReference(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 026 if (src.hasCategory()) 027 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(src.getCategory())); 028 if (src.hasCode()) 029 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 030 if (src.hasSubject()) 031 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 032 if (src.hasContext()) 033 tgt.setEncounter(Reference30_40.convertReference(src.getContext())); 034 if (src.hasEffective()) 035 tgt.setEffective(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getEffective())); 036 if (src.hasIssued()) 037 tgt.setIssuedElement(Instant30_40.convertInstant(src.getIssuedElement())); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference30_40.convertReference(t)); 039 for (org.hl7.fhir.dstu3.model.Reference t : src.getResult()) tgt.addResult(Reference30_40.convertReference(t)); 040 for (org.hl7.fhir.dstu3.model.Reference t : src.getImagingStudy()) 041 tgt.addImagingStudy(Reference30_40.convertReference(t)); 042 for (org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 043 tgt.addMedia(convertDiagnosticReportImageComponent(t)); 044 if (src.hasConclusion()) 045 tgt.setConclusionElement(String30_40.convertString(src.getConclusionElement())); 046 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCodedDiagnosis()) 047 tgt.addConclusionCode(CodeableConcept30_40.convertCodeableConcept(t)); 048 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPresentedForm()) 049 tgt.addPresentedForm(Attachment30_40.convertAttachment(t)); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.dstu3.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r4.model.DiagnosticReport src) throws FHIRException { 054 if (src == null) 055 return null; 056 org.hl7.fhir.dstu3.model.DiagnosticReport tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport(); 057 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 058 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 059 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 060 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_40.convertReference(t)); 061 if (src.hasStatus()) 062 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 063 if (src.hasCategory()) 064 tgt.setCategory(CodeableConcept30_40.convertCodeableConcept(src.getCategoryFirstRep())); 065 if (src.hasCode()) 066 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 067 if (src.hasSubject()) 068 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 069 if (src.hasEncounter()) 070 tgt.setContext(Reference30_40.convertReference(src.getEncounter())); 071 if (src.hasEffective()) 072 tgt.setEffective(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getEffective())); 073 if (src.hasIssued()) 074 tgt.setIssuedElement(Instant30_40.convertInstant(src.getIssuedElement())); 075 for (org.hl7.fhir.r4.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference30_40.convertReference(t)); 076 for (org.hl7.fhir.r4.model.Reference t : src.getResult()) tgt.addResult(Reference30_40.convertReference(t)); 077 for (org.hl7.fhir.r4.model.Reference t : src.getImagingStudy()) 078 tgt.addImagingStudy(Reference30_40.convertReference(t)); 079 for (org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 080 tgt.addImage(convertDiagnosticReportImageComponent(t)); 081 if (src.hasConclusion()) 082 tgt.setConclusionElement(String30_40.convertString(src.getConclusionElement())); 083 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getConclusionCode()) 084 tgt.addCodedDiagnosis(CodeableConcept30_40.convertCodeableConcept(t)); 085 for (org.hl7.fhir.r4.model.Attachment t : src.getPresentedForm()) 086 tgt.addPresentedForm(Attachment30_40.convertAttachment(t)); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 091 if (src == null) 092 return null; 093 org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent(); 094 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 095 if (src.hasComment()) 096 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 097 if (src.hasLink()) 098 tgt.setLink(Reference30_40.convertReference(src.getLink())); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 103 if (src == null) 104 return null; 105 org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent(); 106 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 107 if (src.hasComment()) 108 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 109 if (src.hasLink()) 110 tgt.setLink(Reference30_40.convertReference(src.getLink())); 111 return tgt; 112 } 113 114 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 115 if (src == null || src.isEmpty()) 116 return null; 117 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 118 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 119 if (src.getValue() == null) { 120 tgt.setValue(null); 121 } else { 122 switch (src.getValue()) { 123 case REGISTERED: 124 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 125 break; 126 case PARTIAL: 127 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 128 break; 129 case PRELIMINARY: 130 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 131 break; 132 case FINAL: 133 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 134 break; 135 case AMENDED: 136 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.AMENDED); 137 break; 138 case CORRECTED: 139 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 140 break; 141 case APPENDED: 142 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 143 break; 144 case CANCELLED: 145 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 146 break; 147 case ENTEREDINERROR: 148 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 149 break; 150 case UNKNOWN: 151 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 152 break; 153 default: 154 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 155 break; 156 } 157 } 158 return tgt; 159 } 160 161 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 165 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 166 if (src.getValue() == null) { 167 tgt.setValue(null); 168 } else { 169 switch (src.getValue()) { 170 case REGISTERED: 171 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 172 break; 173 case PARTIAL: 174 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 175 break; 176 case PRELIMINARY: 177 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 178 break; 179 case FINAL: 180 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 181 break; 182 case AMENDED: 183 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.AMENDED); 184 break; 185 case CORRECTED: 186 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 187 break; 188 case APPENDED: 189 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 190 break; 191 case CANCELLED: 192 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 193 break; 194 case ENTEREDINERROR: 195 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 196 break; 197 case UNKNOWN: 198 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 199 break; 200 default: 201 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.NULL); 202 break; 203 } 204 } 205 return tgt; 206 } 207}