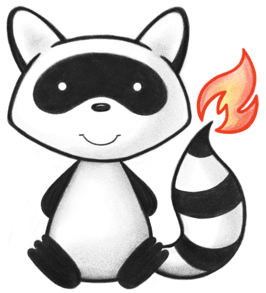
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Duration30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class Encounter30_40 { 014 015 public static org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent src) throws FHIRException { 016 if (src == null) 017 return null; 018 org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent(); 019 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 020 if (src.hasClass_()) 021 tgt.setClass_(Coding30_40.convertCoding(src.getClass_())); 022 if (src.hasPeriod()) 023 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 024 return tgt; 025 } 026 027 public static org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent src) throws FHIRException { 028 if (src == null) 029 return null; 030 org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent(); 031 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 032 if (src.hasClass_()) 033 tgt.setClass_(Coding30_40.convertCoding(src.getClass_())); 034 if (src.hasPeriod()) 035 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 036 return tgt; 037 } 038 039 public static org.hl7.fhir.dstu3.model.Encounter convertEncounter(org.hl7.fhir.r4.model.Encounter src) throws FHIRException { 040 if (src == null) 041 return null; 042 org.hl7.fhir.dstu3.model.Encounter tgt = new org.hl7.fhir.dstu3.model.Encounter(); 043 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 044 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 045 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 046 if (src.hasStatus()) 047 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 048 for (org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 049 tgt.addStatusHistory(convertStatusHistoryComponent(t)); 050 if (src.hasClass_()) 051 tgt.setClass_(Coding30_40.convertCoding(src.getClass_())); 052 for (org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 053 tgt.addClassHistory(convertClassHistoryComponent(t)); 054 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 055 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 056 if (src.hasPriority()) 057 tgt.setPriority(CodeableConcept30_40.convertCodeableConcept(src.getPriority())); 058 if (src.hasSubject()) 059 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 060 for (org.hl7.fhir.r4.model.Reference t : src.getEpisodeOfCare()) 061 tgt.addEpisodeOfCare(Reference30_40.convertReference(t)); 062 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) 063 tgt.addIncomingReferral(Reference30_40.convertReference(t)); 064 for (org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 065 tgt.addParticipant(convertEncounterParticipantComponent(t)); 066 if (src.hasAppointment()) 067 tgt.setAppointment(Reference30_40.convertReference(src.getAppointmentFirstRep())); 068 if (src.hasPeriod()) 069 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 070 if (src.hasLength()) 071 tgt.setLength(Duration30_40.convertDuration(src.getLength())); 072 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 073 tgt.addReason(CodeableConcept30_40.convertCodeableConcept(t)); 074 for (org.hl7.fhir.r4.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 075 tgt.addDiagnosis(convertDiagnosisComponent(t)); 076 for (org.hl7.fhir.r4.model.Reference t : src.getAccount()) tgt.addAccount(Reference30_40.convertReference(t)); 077 if (src.hasHospitalization()) 078 tgt.setHospitalization(convertEncounterHospitalizationComponent(src.getHospitalization())); 079 for (org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent t : src.getLocation()) 080 tgt.addLocation(convertEncounterLocationComponent(t)); 081 if (src.hasServiceProvider()) 082 tgt.setServiceProvider(Reference30_40.convertReference(src.getServiceProvider())); 083 if (src.hasPartOf()) 084 tgt.setPartOf(Reference30_40.convertReference(src.getPartOf())); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.Encounter convertEncounter(org.hl7.fhir.dstu3.model.Encounter src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.r4.model.Encounter tgt = new org.hl7.fhir.r4.model.Encounter(); 092 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 093 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 094 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 095 if (src.hasStatus()) 096 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 097 for (org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 098 tgt.addStatusHistory(convertStatusHistoryComponent(t)); 099 if (src.hasClass_()) 100 tgt.setClass_(Coding30_40.convertCoding(src.getClass_())); 101 for (org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 102 tgt.addClassHistory(convertClassHistoryComponent(t)); 103 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 104 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 105 if (src.hasPriority()) 106 tgt.setPriority(CodeableConcept30_40.convertCodeableConcept(src.getPriority())); 107 if (src.hasSubject()) 108 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 109 for (org.hl7.fhir.dstu3.model.Reference t : src.getEpisodeOfCare()) 110 tgt.addEpisodeOfCare(Reference30_40.convertReference(t)); 111 for (org.hl7.fhir.dstu3.model.Reference t : src.getIncomingReferral()) 112 tgt.addBasedOn(Reference30_40.convertReference(t)); 113 for (org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 114 tgt.addParticipant(convertEncounterParticipantComponent(t)); 115 if (src.hasAppointment()) 116 tgt.addAppointment(Reference30_40.convertReference(src.getAppointment())); 117 if (src.hasPeriod()) 118 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 119 if (src.hasLength()) 120 tgt.setLength(Duration30_40.convertDuration(src.getLength())); 121 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReason()) 122 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 123 for (org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 124 tgt.addDiagnosis(convertDiagnosisComponent(t)); 125 for (org.hl7.fhir.dstu3.model.Reference t : src.getAccount()) tgt.addAccount(Reference30_40.convertReference(t)); 126 if (src.hasHospitalization()) 127 tgt.setHospitalization(convertEncounterHospitalizationComponent(src.getHospitalization())); 128 for (org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent t : src.getLocation()) 129 tgt.addLocation(convertEncounterLocationComponent(t)); 130 if (src.hasServiceProvider()) 131 tgt.setServiceProvider(Reference30_40.convertReference(src.getServiceProvider())); 132 if (src.hasPartOf()) 133 tgt.setPartOf(Reference30_40.convertReference(src.getPartOf())); 134 return tgt; 135 } 136 137 public static org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent convertEncounterHospitalizationComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent src) throws FHIRException { 138 if (src == null) 139 return null; 140 org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent(); 141 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 142 if (src.hasPreAdmissionIdentifier()) 143 tgt.setPreAdmissionIdentifier(Identifier30_40.convertIdentifier(src.getPreAdmissionIdentifier())); 144 if (src.hasOrigin()) 145 tgt.setOrigin(Reference30_40.convertReference(src.getOrigin())); 146 if (src.hasAdmitSource()) 147 tgt.setAdmitSource(CodeableConcept30_40.convertCodeableConcept(src.getAdmitSource())); 148 if (src.hasReAdmission()) 149 tgt.setReAdmission(CodeableConcept30_40.convertCodeableConcept(src.getReAdmission())); 150 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getDietPreference()) 151 tgt.addDietPreference(CodeableConcept30_40.convertCodeableConcept(t)); 152 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialCourtesy()) 153 tgt.addSpecialCourtesy(CodeableConcept30_40.convertCodeableConcept(t)); 154 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialArrangement()) 155 tgt.addSpecialArrangement(CodeableConcept30_40.convertCodeableConcept(t)); 156 if (src.hasDestination()) 157 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 158 if (src.hasDischargeDisposition()) 159 tgt.setDischargeDisposition(CodeableConcept30_40.convertCodeableConcept(src.getDischargeDisposition())); 160 return tgt; 161 } 162 163 public static org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent convertEncounterHospitalizationComponent(org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent src) throws FHIRException { 164 if (src == null) 165 return null; 166 org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent(); 167 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 168 if (src.hasPreAdmissionIdentifier()) 169 tgt.setPreAdmissionIdentifier(Identifier30_40.convertIdentifier(src.getPreAdmissionIdentifier())); 170 if (src.hasOrigin()) 171 tgt.setOrigin(Reference30_40.convertReference(src.getOrigin())); 172 if (src.hasAdmitSource()) 173 tgt.setAdmitSource(CodeableConcept30_40.convertCodeableConcept(src.getAdmitSource())); 174 if (src.hasReAdmission()) 175 tgt.setReAdmission(CodeableConcept30_40.convertCodeableConcept(src.getReAdmission())); 176 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getDietPreference()) 177 tgt.addDietPreference(CodeableConcept30_40.convertCodeableConcept(t)); 178 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialCourtesy()) 179 tgt.addSpecialCourtesy(CodeableConcept30_40.convertCodeableConcept(t)); 180 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialArrangement()) 181 tgt.addSpecialArrangement(CodeableConcept30_40.convertCodeableConcept(t)); 182 if (src.hasDestination()) 183 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 184 if (src.hasDischargeDisposition()) 185 tgt.setDischargeDisposition(CodeableConcept30_40.convertCodeableConcept(src.getDischargeDisposition())); 186 return tgt; 187 } 188 189 public static org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent src) throws FHIRException { 190 if (src == null) 191 return null; 192 org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent(); 193 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 194 if (src.hasLocation()) 195 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 196 if (src.hasStatus()) 197 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 198 if (src.hasPeriod()) 199 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 200 return tgt; 201 } 202 203 public static org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent src) throws FHIRException { 204 if (src == null) 205 return null; 206 org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent(); 207 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 208 if (src.hasLocation()) 209 tgt.setLocation(Reference30_40.convertReference(src.getLocation())); 210 if (src.hasStatus()) 211 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 212 if (src.hasPeriod()) 213 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 214 return tgt; 215 } 216 217 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatusEnumFactory()); 221 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 222 switch (src.getValue()) { 223 case PLANNED: 224 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.PLANNED); 225 break; 226 case ACTIVE: 227 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.ACTIVE); 228 break; 229 case RESERVED: 230 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.RESERVED); 231 break; 232 case COMPLETED: 233 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.COMPLETED); 234 break; 235 default: 236 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.NULL); 237 break; 238 } 239 return tgt; 240 } 241 242 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 243 if (src == null || src.isEmpty()) 244 return null; 245 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Encounter.EncounterLocationStatusEnumFactory()); 246 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 247 switch (src.getValue()) { 248 case PLANNED: 249 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus.PLANNED); 250 break; 251 case ACTIVE: 252 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus.ACTIVE); 253 break; 254 case RESERVED: 255 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus.RESERVED); 256 break; 257 case COMPLETED: 258 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus.COMPLETED); 259 break; 260 default: 261 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus.NULL); 262 break; 263 } 264 return tgt; 265 } 266 267 public static org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 268 if (src == null) 269 return null; 270 org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent(); 271 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 272 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 273 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 274 if (src.hasPeriod()) 275 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 276 if (src.hasIndividual()) 277 tgt.setIndividual(Reference30_40.convertReference(src.getIndividual())); 278 return tgt; 279 } 280 281 public static org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 282 if (src == null) 283 return null; 284 org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent(); 285 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 286 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 287 tgt.addType(CodeableConcept30_40.convertCodeableConcept(t)); 288 if (src.hasPeriod()) 289 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 290 if (src.hasIndividual()) 291 tgt.setIndividual(Reference30_40.convertReference(src.getIndividual())); 292 return tgt; 293 } 294 295 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterStatus> convertEncounterStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> src) throws FHIRException { 296 if (src == null || src.isEmpty()) 297 return null; 298 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Encounter.EncounterStatusEnumFactory()); 299 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 300 switch (src.getValue()) { 301 case PLANNED: 302 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.PLANNED); 303 break; 304 case ARRIVED: 305 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.ARRIVED); 306 break; 307 case TRIAGED: 308 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.TRIAGED); 309 break; 310 case INPROGRESS: 311 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.INPROGRESS); 312 break; 313 case ONLEAVE: 314 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.ONLEAVE); 315 break; 316 case FINISHED: 317 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.FINISHED); 318 break; 319 case CANCELLED: 320 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.CANCELLED); 321 break; 322 case ENTEREDINERROR: 323 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.ENTEREDINERROR); 324 break; 325 case UNKNOWN: 326 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.UNKNOWN); 327 break; 328 default: 329 tgt.setValue(org.hl7.fhir.r4.model.Encounter.EncounterStatus.NULL); 330 break; 331 } 332 return tgt; 333 } 334 335 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> convertEncounterStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterStatus> src) throws FHIRException { 336 if (src == null || src.isEmpty()) 337 return null; 338 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Encounter.EncounterStatusEnumFactory()); 339 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 340 switch (src.getValue()) { 341 case PLANNED: 342 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.PLANNED); 343 break; 344 case ARRIVED: 345 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.ARRIVED); 346 break; 347 case TRIAGED: 348 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.TRIAGED); 349 break; 350 case INPROGRESS: 351 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.INPROGRESS); 352 break; 353 case ONLEAVE: 354 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.ONLEAVE); 355 break; 356 case FINISHED: 357 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.FINISHED); 358 break; 359 case CANCELLED: 360 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.CANCELLED); 361 break; 362 case ENTEREDINERROR: 363 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.ENTEREDINERROR); 364 break; 365 case UNKNOWN: 366 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.UNKNOWN); 367 break; 368 default: 369 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.NULL); 370 break; 371 } 372 return tgt; 373 } 374 375 public static org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent src) throws FHIRException { 376 if (src == null) 377 return null; 378 org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent(); 379 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 380 if (src.hasStatus()) 381 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 382 if (src.hasPeriod()) 383 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 384 return tgt; 385 } 386 387 public static org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent src) throws FHIRException { 388 if (src == null) 389 return null; 390 org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent(); 391 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 392 if (src.hasStatus()) 393 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 394 if (src.hasPeriod()) 395 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 396 return tgt; 397 } 398 399 public static org.hl7.fhir.r4.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent src) throws FHIRException { 400 if (src == null) return null; 401 org.hl7.fhir.r4.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.r4.model.Encounter.DiagnosisComponent(); 402 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 403 if (src.hasCondition()) tgt.setCondition(Reference30_40.convertReference(src.getCondition())); 404 if (src.hasRole()) tgt.setUse(CodeableConcept30_40.convertCodeableConcept(src.getRole())); 405 if (src.hasRank()) tgt.setRankElement(PositiveInt30_40.convertPositiveInt(src.getRankElement())); 406 return tgt; 407 } 408 409 public static org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r4.model.Encounter.DiagnosisComponent src) throws FHIRException { 410 if (src == null) return null; 411 org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent(); 412 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 413 if (src.hasCondition()) tgt.setCondition(Reference30_40.convertReference(src.getCondition())); 414 if (src.hasUse()) tgt.setRole(CodeableConcept30_40.convertCodeableConcept(src.getUse())); 415 if (src.hasRank()) tgt.setRankElement(PositiveInt30_40.convertPositiveInt(src.getRankElement())); 416 return tgt; 417 } 418}