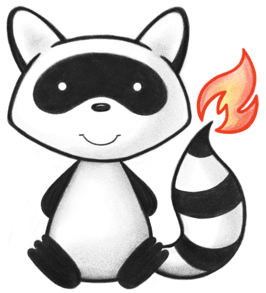
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Goal30_40 { 013 014 public static org.hl7.fhir.dstu3.model.Goal convertGoal(org.hl7.fhir.r4.model.Goal src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.dstu3.model.Goal tgt = new org.hl7.fhir.dstu3.model.Goal(); 018 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 021 if (src.hasLifecycleStatus()) 022 tgt.setStatusElement(convertGoalStatus(src.getLifecycleStatusElement())); 023 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 024 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 025 if (src.hasPriority()) 026 tgt.setPriority(CodeableConcept30_40.convertCodeableConcept(src.getPriority())); 027 if (src.hasDescription()) 028 tgt.setDescription(CodeableConcept30_40.convertCodeableConcept(src.getDescription())); 029 if (src.hasSubject()) 030 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 031 if (src.hasStart()) 032 tgt.setStart(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getStart())); 033 if (src.hasTarget()) 034 tgt.setTarget(convertGoalTargetComponent(src.getTargetFirstRep())); 035 if (src.hasStatusDate()) 036 tgt.setStatusDateElement(Date30_40.convertDate(src.getStatusDateElement())); 037 if (src.hasStatusReason()) 038 tgt.setStatusReasonElement(String30_40.convertString(src.getStatusReasonElement())); 039 if (src.hasExpressedBy()) 040 tgt.setExpressedBy(Reference30_40.convertReference(src.getExpressedBy())); 041 for (org.hl7.fhir.r4.model.Reference t : src.getAddresses()) tgt.addAddresses(Reference30_40.convertReference(t)); 042 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 043 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getOutcomeCode()) 044 tgt.addOutcomeCode(CodeableConcept30_40.convertCodeableConcept(t)); 045 for (org.hl7.fhir.r4.model.Reference t : src.getOutcomeReference()) 046 tgt.addOutcomeReference(Reference30_40.convertReference(t)); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.r4.model.Goal convertGoal(org.hl7.fhir.dstu3.model.Goal src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r4.model.Goal tgt = new org.hl7.fhir.r4.model.Goal(); 054 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 055 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 056 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 057 if (src.hasStatus()) 058 tgt.setLifecycleStatusElement(convertGoalStatus(src.getStatusElement())); 059 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 060 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 061 if (src.hasPriority()) 062 tgt.setPriority(CodeableConcept30_40.convertCodeableConcept(src.getPriority())); 063 if (src.hasDescription()) 064 tgt.setDescription(CodeableConcept30_40.convertCodeableConcept(src.getDescription())); 065 if (src.hasSubject()) 066 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 067 if (src.hasStart()) 068 tgt.setStart(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getStart())); 069 if (src.hasTarget()) 070 tgt.addTarget(convertGoalTargetComponent(src.getTarget())); 071 if (src.hasStatusDate()) 072 tgt.setStatusDateElement(Date30_40.convertDate(src.getStatusDateElement())); 073 if (src.hasStatusReason()) 074 tgt.setStatusReasonElement(String30_40.convertString(src.getStatusReasonElement())); 075 if (src.hasExpressedBy()) 076 tgt.setExpressedBy(Reference30_40.convertReference(src.getExpressedBy())); 077 for (org.hl7.fhir.dstu3.model.Reference t : src.getAddresses()) 078 tgt.addAddresses(Reference30_40.convertReference(t)); 079 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 080 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getOutcomeCode()) 081 tgt.addOutcomeCode(CodeableConcept30_40.convertCodeableConcept(t)); 082 for (org.hl7.fhir.dstu3.model.Reference t : src.getOutcomeReference()) 083 tgt.addOutcomeReference(Reference30_40.convertReference(t)); 084 return tgt; 085 } 086 087 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus> convertGoalStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Goal.GoalStatus> src) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Goal.GoalLifecycleStatusEnumFactory()); 091 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 092 switch (src.getValue()) { 093 case PROPOSED: 094 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.PROPOSED); 095 break; 096 case ACCEPTED: 097 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACCEPTED); 098 break; 099 case PLANNED: 100 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.PLANNED); 101 break; 102 case INPROGRESS: 103 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACTIVE); 104 break; 105 case ONTARGET: 106 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACTIVE); 107 break; 108 case AHEADOFTARGET: 109 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACTIVE); 110 break; 111 case BEHINDTARGET: 112 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACTIVE); 113 break; 114 case SUSTAINING: 115 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ACTIVE); 116 break; 117 case ACHIEVED: 118 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.COMPLETED); 119 break; 120 case ONHOLD: 121 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ONHOLD); 122 break; 123 case CANCELLED: 124 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.CANCELLED); 125 break; 126 case ENTEREDINERROR: 127 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.ENTEREDINERROR); 128 break; 129 case REJECTED: 130 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.REJECTED); 131 break; 132 default: 133 tgt.setValue(org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus.NULL); 134 break; 135 } 136 return tgt; 137 } 138 139 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Goal.GoalStatus> convertGoalStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Goal.GoalLifecycleStatus> src) throws FHIRException { 140 if (src == null || src.isEmpty()) 141 return null; 142 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Goal.GoalStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Goal.GoalStatusEnumFactory()); 143 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 144 switch (src.getValue()) { 145 case PROPOSED: 146 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.PROPOSED); 147 break; 148 case ACCEPTED: 149 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.ACCEPTED); 150 break; 151 case PLANNED: 152 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.PLANNED); 153 break; 154 case ACTIVE: 155 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.INPROGRESS); 156 break; 157 case COMPLETED: 158 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.ACHIEVED); 159 break; 160 case ONHOLD: 161 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.ONHOLD); 162 break; 163 case CANCELLED: 164 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.CANCELLED); 165 break; 166 case ENTEREDINERROR: 167 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.ENTEREDINERROR); 168 break; 169 case REJECTED: 170 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.REJECTED); 171 break; 172 default: 173 tgt.setValue(org.hl7.fhir.dstu3.model.Goal.GoalStatus.NULL); 174 break; 175 } 176 return tgt; 177 } 178 179 public static org.hl7.fhir.r4.model.Goal.GoalTargetComponent convertGoalTargetComponent(org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent src) throws FHIRException { 180 if (src == null) 181 return null; 182 org.hl7.fhir.r4.model.Goal.GoalTargetComponent tgt = new org.hl7.fhir.r4.model.Goal.GoalTargetComponent(); 183 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 184 if (src.hasMeasure()) 185 tgt.setMeasure(CodeableConcept30_40.convertCodeableConcept(src.getMeasure())); 186 if (src.hasDetail()) 187 tgt.setDetail(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDetail())); 188 if (src.hasDue()) 189 tgt.setDue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDue())); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent convertGoalTargetComponent(org.hl7.fhir.r4.model.Goal.GoalTargetComponent src) throws FHIRException { 194 if (src == null) 195 return null; 196 org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent tgt = new org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent(); 197 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 198 if (src.hasMeasure()) 199 tgt.setMeasure(CodeableConcept30_40.convertCodeableConcept(src.getMeasure())); 200 if (src.hasDetail()) 201 tgt.setDetail(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDetail())); 202 if (src.hasDue()) 203 tgt.setDue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDue())); 204 return tgt; 205 } 206}