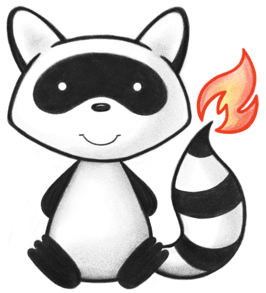
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class GraphDefinition30_40 { 017 018 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode> convertCompartmentCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode> src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.GraphDefinition.CompartmentCodeEnumFactory()); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 023 switch (src.getValue()) { 024 case PATIENT: 025 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.PATIENT); 026 break; 027 case ENCOUNTER: 028 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.ENCOUNTER); 029 break; 030 case RELATEDPERSON: 031 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.RELATEDPERSON); 032 break; 033 case PRACTITIONER: 034 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.PRACTITIONER); 035 break; 036 case DEVICE: 037 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.DEVICE); 038 break; 039 default: 040 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode.NULL); 041 break; 042 } 043 return tgt; 044 } 045 046 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode> convertCompartmentCode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.CompartmentCode> src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCodeEnumFactory()); 050 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 051 switch (src.getValue()) { 052 case PATIENT: 053 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.PATIENT); 054 break; 055 case ENCOUNTER: 056 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.ENCOUNTER); 057 break; 058 case RELATEDPERSON: 059 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.RELATEDPERSON); 060 break; 061 case PRACTITIONER: 062 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.PRACTITIONER); 063 break; 064 case DEVICE: 065 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.DEVICE); 066 break; 067 default: 068 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode.NULL); 069 break; 070 } 071 return tgt; 072 } 073 074 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule> convertGraphCompartmentRule(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule> src) throws FHIRException { 075 if (src == null || src.isEmpty()) 076 return null; 077 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRuleEnumFactory()); 078 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 079 switch (src.getValue()) { 080 case IDENTICAL: 081 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule.IDENTICAL); 082 break; 083 case MATCHING: 084 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule.MATCHING); 085 break; 086 case DIFFERENT: 087 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule.DIFFERENT); 088 break; 089 case CUSTOM: 090 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule.CUSTOM); 091 break; 092 default: 093 tgt.setValue(org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule.NULL); 094 break; 095 } 096 return tgt; 097 } 098 099 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule> convertGraphCompartmentRule(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.GraphDefinition.GraphCompartmentRule> src) throws FHIRException { 100 if (src == null || src.isEmpty()) 101 return null; 102 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRuleEnumFactory()); 103 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 104 switch (src.getValue()) { 105 case IDENTICAL: 106 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule.IDENTICAL); 107 break; 108 case MATCHING: 109 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule.MATCHING); 110 break; 111 case DIFFERENT: 112 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule.DIFFERENT); 113 break; 114 case CUSTOM: 115 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule.CUSTOM); 116 break; 117 default: 118 tgt.setValue(org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule.NULL); 119 break; 120 } 121 return tgt; 122 } 123 124 public static org.hl7.fhir.dstu3.model.GraphDefinition convertGraphDefinition(org.hl7.fhir.r4.model.GraphDefinition src) throws FHIRException { 125 if (src == null) 126 return null; 127 org.hl7.fhir.dstu3.model.GraphDefinition tgt = new org.hl7.fhir.dstu3.model.GraphDefinition(); 128 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 129 if (src.hasUrl()) 130 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 131 if (src.hasVersion()) 132 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 133 if (src.hasName()) 134 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 135 if (src.hasStatus()) 136 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 137 if (src.hasExperimental()) 138 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 139 if (src.hasDateElement()) 140 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 141 if (src.hasPublisher()) 142 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 143 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 144 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 145 if (src.hasDescription()) 146 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 147 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 148 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 149 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 150 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 151 if (src.hasPurpose()) 152 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 153 if (src.hasStart()) 154 tgt.setStartElement(Code30_40.convertCode(src.getStartElement())); 155 if (src.hasProfile()) 156 tgt.setProfile(src.getProfile()); 157 for (org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 158 tgt.addLink(convertGraphDefinitionLinkComponent(t)); 159 return tgt; 160 } 161 162 public static org.hl7.fhir.r4.model.GraphDefinition convertGraphDefinition(org.hl7.fhir.dstu3.model.GraphDefinition src) throws FHIRException { 163 if (src == null) 164 return null; 165 org.hl7.fhir.r4.model.GraphDefinition tgt = new org.hl7.fhir.r4.model.GraphDefinition(); 166 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 167 if (src.hasUrl()) 168 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 169 if (src.hasVersion()) 170 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 171 if (src.hasName()) 172 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 173 if (src.hasStatus()) 174 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 175 if (src.hasExperimental()) 176 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 177 if (src.hasDateElement()) 178 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 179 if (src.hasPublisher()) 180 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 181 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 182 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 183 if (src.hasDescription()) 184 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 185 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 186 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 187 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 188 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 189 if (src.hasPurpose()) 190 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 191 if (src.hasStart()) 192 tgt.setStartElement(Code30_40.convertCode(src.getStartElement())); 193 if (src.hasProfile()) 194 tgt.setProfile(src.getProfile()); 195 for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 196 tgt.addLink(convertGraphDefinitionLinkComponent(t)); 197 return tgt; 198 } 199 200 public static org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent convertGraphDefinitionLinkComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent src) throws FHIRException { 201 if (src == null) 202 return null; 203 org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent tgt = new org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent(); 204 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 205 if (src.hasPath()) 206 tgt.setPathElement(String30_40.convertString(src.getPathElement())); 207 if (src.hasSliceName()) 208 tgt.setSliceNameElement(String30_40.convertString(src.getSliceNameElement())); 209 if (src.hasMin()) 210 tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 211 if (src.hasMax()) 212 tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 213 if (src.hasDescription()) 214 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 215 for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent t : src.getTarget()) 216 tgt.addTarget(convertGraphDefinitionLinkTargetComponent(t)); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent convertGraphDefinitionLinkComponent(org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent src) throws FHIRException { 221 if (src == null) 222 return null; 223 org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent(); 224 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 225 if (src.hasPath()) 226 tgt.setPathElement(String30_40.convertString(src.getPathElement())); 227 if (src.hasSliceName()) 228 tgt.setSliceNameElement(String30_40.convertString(src.getSliceNameElement())); 229 if (src.hasMin()) 230 tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 231 if (src.hasMax()) 232 tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 233 if (src.hasDescription()) 234 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 235 for (org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetComponent t : src.getTarget()) 236 tgt.addTarget(convertGraphDefinitionLinkTargetComponent(t)); 237 return tgt; 238 } 239 240 public static org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent convertGraphDefinitionLinkTargetCompartmentComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent src) throws FHIRException { 241 if (src == null) 242 return null; 243 org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent tgt = new org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent(); 244 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 245 if (src.hasCode()) 246 tgt.setCodeElement(convertCompartmentCode(src.getCodeElement())); 247 if (src.hasRule()) 248 tgt.setRuleElement(convertGraphCompartmentRule(src.getRuleElement())); 249 if (src.hasExpression()) 250 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 251 if (src.hasDescription()) 252 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 253 return tgt; 254 } 255 256 public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent convertGraphDefinitionLinkTargetCompartmentComponent(org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent src) throws FHIRException { 257 if (src == null) 258 return null; 259 org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent(); 260 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 261 if (src.hasCode()) 262 tgt.setCodeElement(convertCompartmentCode(src.getCodeElement())); 263 if (src.hasRule()) 264 tgt.setRuleElement(convertGraphCompartmentRule(src.getRuleElement())); 265 if (src.hasExpression()) 266 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 267 if (src.hasDescription()) 268 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 269 return tgt; 270 } 271 272 public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent convertGraphDefinitionLinkTargetComponent(org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetComponent src) throws FHIRException { 273 if (src == null) 274 return null; 275 org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent(); 276 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 277 if (src.hasType()) 278 tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 279 if (src.hasProfile()) 280 tgt.setProfile(src.getProfile()); 281 for (org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent t : src.getCompartment()) 282 tgt.addCompartment(convertGraphDefinitionLinkTargetCompartmentComponent(t)); 283 for (org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 284 tgt.addLink(convertGraphDefinitionLinkComponent(t)); 285 return tgt; 286 } 287 288 public static org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetComponent convertGraphDefinitionLinkTargetComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent src) throws FHIRException { 289 if (src == null) 290 return null; 291 org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetComponent tgt = new org.hl7.fhir.r4.model.GraphDefinition.GraphDefinitionLinkTargetComponent(); 292 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 293 if (src.hasType()) 294 tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 295 if (src.hasProfile()) 296 tgt.setProfile(src.getProfile()); 297 for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent t : src.getCompartment()) 298 tgt.addCompartment(convertGraphDefinitionLinkTargetCompartmentComponent(t)); 299 for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 300 tgt.addLink(convertGraphDefinitionLinkComponent(t)); 301 return tgt; 302 } 303}