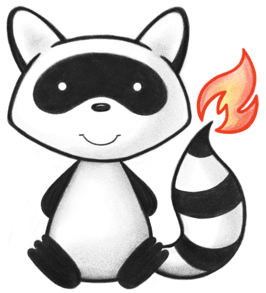
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 016import org.hl7.fhir.dstu3.model.ImplementationGuide; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r4.model.Enumeration; 019 020public class ImplementationGuide30_40 { 021 022 public static org.hl7.fhir.dstu3.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.r4.model.ImplementationGuide src) throws FHIRException { 023 if (src == null) 024 return null; 025 org.hl7.fhir.dstu3.model.ImplementationGuide tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide(); 026 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 027 if (src.hasUrl()) 028 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 029 if (src.hasVersion()) 030 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 031 if (src.hasName()) 032 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 033 if (src.hasStatus()) 034 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 035 if (src.hasExperimental()) 036 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 037 if (src.hasDateElement()) 038 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 039 if (src.hasPublisher()) 040 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 041 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 042 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 043 if (src.hasDescription()) 044 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 045 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 046 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 047 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 048 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 049 if (src.hasCopyright()) 050 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 051 if (src.hasFhirVersion()) 052 for (Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> v : src.getFhirVersion()) { 053 tgt.setFhirVersion(v.asStringValue()); 054 break; 055 } 056 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent t : src.getDependsOn()) 057 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 058 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent t : src.getDefinition().getGrouping()) 059 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 060 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent t : src.getDefinition().getResource()) { 061 findPackage(tgt.getPackage(), t.getGroupingId()).addResource(convertImplementationGuidePackageResourceComponent(t)); 062 } 063 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 064 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 065 if (src.getDefinition().hasPage()) 066 tgt.setPage(convertImplementationGuidePageComponent(src.getDefinition().getPage())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.r4.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu3.model.ImplementationGuide src) throws FHIRException { 071 if (src == null) 072 return null; 073 org.hl7.fhir.r4.model.ImplementationGuide tgt = new org.hl7.fhir.r4.model.ImplementationGuide(); 074 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 075 if (src.hasUrl()) 076 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 077 if (src.hasVersion()) 078 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 079 if (src.hasName()) 080 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 081 if (src.hasStatus()) 082 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 083 if (src.hasExperimental()) 084 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 085 if (src.hasDateElement()) 086 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 087 if (src.hasPublisher()) 088 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 089 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 090 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 091 if (src.hasDescription()) 092 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 093 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 094 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 095 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 096 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 097 if (src.hasCopyright()) 098 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 099 if (src.hasFhirVersion()) 100 tgt.addFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 101 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 102 tgt.addDependsOn(convertImplementationGuideDependencyComponent(t)); 103 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 104 tgt.getDefinition().addGrouping(convertImplementationGuidePackageComponent(tgt.getDefinition(), t)); 105 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 106 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 107 if (src.hasPage()) 108 tgt.getDefinition().setPage(convertImplementationGuidePageComponent(src.getPage())); 109 return tgt; 110 } 111 112 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent src) throws FHIRException { 113 if (src == null) 114 return null; 115 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 116 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 117 tgt.setType(org.hl7.fhir.dstu3.model.ImplementationGuide.GuideDependencyType.REFERENCE); 118 if (src.hasUri()) 119 tgt.setUri(src.getUri()); 120 return tgt; 121 } 122 123 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 124 if (src == null) 125 return null; 126 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent(); 127 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 128 if (src.hasUri()) 129 tgt.setUri(src.getUri()); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 134 if (src == null) 135 return null; 136 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 137 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 138 if (src.hasType()) 139 tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 140 if (src.hasProfile()) 141 tgt.setProfile(Reference30_40.convertCanonicalToReference(src.getProfileElement())); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 146 if (src == null) 147 return null; 148 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 149 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 150 if (src.hasType()) 151 tgt.setTypeElement(Code30_40.convertCode(src.getTypeElement())); 152 if (src.hasProfile()) 153 tgt.setProfileElement(Reference30_40.convertReferenceToCanonical(src.getProfile())); 154 return tgt; 155 } 156 157 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent src) throws FHIRException { 158 if (src == null) 159 return null; 160 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent(); 161 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 162 tgt.setId(src.getId()); 163 if (src.hasName()) 164 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 165 if (src.hasDescription()) 166 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 167 return tgt; 168 } 169 170 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionComponent context, org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 171 if (src == null) 172 return null; 173 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent(); 174 tgt.setId("p" + (context.getGrouping().size() + 1)); 175 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 176 if (src.hasName()) 177 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 178 if (src.hasDescription()) 179 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 180 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) { 181 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tn = convertImplementationGuidePackageResourceComponent(t); 182 tn.setGroupingId(tgt.getId()); 183 context.addResource(tn); 184 } 185 return tgt; 186 } 187 188 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 189 if (src == null) 190 return null; 191 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent(); 192 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 193 if (src.hasExampleFor()) { 194 org.hl7.fhir.r4.model.Type t = ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getExampleFor()); 195 tgt.setExample(t instanceof org.hl7.fhir.r4.model.Reference ? new org.hl7.fhir.r4.model.CanonicalType(((org.hl7.fhir.r4.model.Reference) t).getReference()) : t); 196 } else if (src.hasExample()) 197 tgt.setExample(new org.hl7.fhir.r4.model.BooleanType(src.getExample())); 198 if (src.hasName()) 199 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 200 if (src.hasDescription()) 201 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 202 if (src.hasSourceReference()) 203 tgt.setReference(Reference30_40.convertReference(src.getSourceReference())); 204 else if (src.hasSourceUriType()) 205 tgt.setReference(new org.hl7.fhir.r4.model.Reference(src.getSourceUriType().getValue())); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent src) throws FHIRException { 210 if (src == null) 211 return null; 212 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 213 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 214 if (src.hasExampleCanonicalType()) { 215 if (src.hasExampleCanonicalType()) 216 tgt.setExampleFor(Reference30_40.convertCanonicalToReference(src.getExampleCanonicalType())); 217 tgt.setExample(true); 218 } else if (src.hasExampleBooleanType()) 219 tgt.setExample(src.getExampleBooleanType().getValue()); 220 else 221 tgt.setExample(false); 222 if (src.hasName()) 223 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 224 if (src.hasDescription()) 225 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 226 if (src.hasReference()) 227 tgt.setSource(Reference30_40.convertReference(src.getReference())); 228 return tgt; 229 } 230 231 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent src) throws FHIRException { 232 if (src == null) 233 return null; 234 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent(); 235 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 236 if (src.hasNameUrlType()) 237 tgt.setSource(src.getNameUrlType().getValue()); 238 if (src.hasTitle()) 239 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 240 if (src.hasGeneration()) 241 tgt.setKind(convertPageGeneration(src.getGeneration())); 242 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent t : src.getPage()) 243 tgt.addPage(convertImplementationGuidePageComponent(t)); 244 return tgt; 245 } 246 247 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 248 if (src == null) 249 return null; 250 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent(); 251 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 252 if (src.hasSource()) 253 tgt.setName(convertUriToUrl(src.getSourceElement())); 254 if (src.hasTitle()) 255 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 256 if (src.hasKind()) 257 tgt.setGeneration(convertPageGeneration(src.getKind())); 258 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 259 tgt.addPage(convertImplementationGuidePageComponent(t)); 260 return tgt; 261 } 262 263 static public org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration convertPageGeneration(org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind kind) { 264 switch (kind) { 265 case PAGE: 266 return org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration.HTML; 267 default: 268 return org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration.GENERATED; 269 } 270 } 271 272 static public org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind convertPageGeneration(org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration generation) { 273 switch (generation) { 274 case HTML: 275 return org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind.PAGE; 276 default: 277 return org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind.RESOURCE; 278 } 279 } 280 281 public static org.hl7.fhir.r4.model.UrlType convertUriToUrl(org.hl7.fhir.dstu3.model.UriType src) throws FHIRException { 282 org.hl7.fhir.r4.model.UrlType tgt = new org.hl7.fhir.r4.model.UrlType(src.getValue()); 283 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 284 return tgt; 285 } 286 287 static public org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent findPackage(List<ImplementationGuide.ImplementationGuidePackageComponent> definition, String id) { 288 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t : definition) 289 if (t.hasId() && t.getId().equals(id)) 290 return t; 291 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t1 = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent(); 292 t1.setName("Default Package"); 293 t1.setId(id); 294 return t1; 295 } 296}