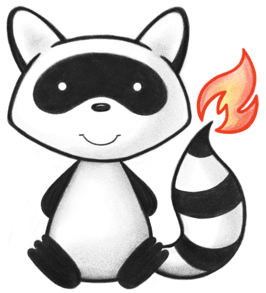
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ParameterDefinition30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.RelatedArtifact30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.TriggerDefinition30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 017import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 018import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 019import org.hl7.fhir.dstu3.model.ContactDetail; 020import org.hl7.fhir.dstu3.model.Contributor.ContributorType; 021import org.hl7.fhir.exceptions.FHIRException; 022 023public class Library30_40 { 024 025 public static org.hl7.fhir.dstu3.model.Library convertLibrary(org.hl7.fhir.r4.model.Library src) throws FHIRException { 026 if (src == null) 027 return null; 028 org.hl7.fhir.dstu3.model.Library tgt = new org.hl7.fhir.dstu3.model.Library(); 029 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 030 if (src.hasUrl()) 031 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 032 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 033 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 034 if (src.hasVersion()) 035 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 036 if (src.hasName()) 037 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 038 if (src.hasTitle()) 039 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 040 if (src.hasStatus()) 041 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 042 if (src.hasExperimental()) 043 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 044 if (src.hasType()) 045 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 046 if (src.hasDateElement()) 047 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 048 if (src.hasPublisher()) 049 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 050 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 051 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 052 if (src.hasDescription()) 053 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 054 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 055 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 056 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 057 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 058 if (src.hasPurpose()) 059 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 060 if (src.hasUsage()) 061 tgt.setUsageElement(String30_40.convertString(src.getUsageElement())); 062 if (src.hasCopyright()) 063 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 064 if (src.hasApprovalDate()) 065 tgt.setApprovalDateElement(Date30_40.convertDate(src.getApprovalDateElement())); 066 if (src.hasLastReviewDate()) 067 tgt.setLastReviewDateElement(Date30_40.convertDate(src.getLastReviewDateElement())); 068 if (src.hasEffectivePeriod()) 069 tgt.setEffectivePeriod(Period30_40.convertPeriod(src.getEffectivePeriod())); 070 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getTopic()) 071 tgt.addTopic(CodeableConcept30_40.convertCodeableConcept(t)); 072 for (org.hl7.fhir.r4.model.ContactDetail t : src.getAuthor()) { 073 org.hl7.fhir.dstu3.model.Contributor c = new org.hl7.fhir.dstu3.model.Contributor(); 074 c.setType(ContributorType.AUTHOR); 075 c.addContact(ContactDetail30_40.convertContactDetail(t)); 076 tgt.addContributor(c); 077 } 078 for (org.hl7.fhir.r4.model.ContactDetail t : src.getEditor()) { 079 org.hl7.fhir.dstu3.model.Contributor c = new org.hl7.fhir.dstu3.model.Contributor(); 080 c.setType(ContributorType.EDITOR); 081 c.addContact(ContactDetail30_40.convertContactDetail(t)); 082 tgt.addContributor(c); 083 } 084 for (org.hl7.fhir.r4.model.ContactDetail t : src.getReviewer()) { 085 org.hl7.fhir.dstu3.model.Contributor c = new org.hl7.fhir.dstu3.model.Contributor(); 086 c.setType(ContributorType.REVIEWER); 087 c.addContact(ContactDetail30_40.convertContactDetail(t)); 088 tgt.addContributor(c); 089 } 090 for (org.hl7.fhir.r4.model.ContactDetail t : src.getEndorser()) { 091 org.hl7.fhir.dstu3.model.Contributor c = new org.hl7.fhir.dstu3.model.Contributor(); 092 c.setType(ContributorType.ENDORSER); 093 c.addContact(ContactDetail30_40.convertContactDetail(t)); 094 tgt.addContributor(c); 095 } 096 for (org.hl7.fhir.r4.model.RelatedArtifact t : src.getRelatedArtifact()) 097 tgt.addRelatedArtifact(RelatedArtifact30_40.convertRelatedArtifact(t)); 098 for (org.hl7.fhir.r4.model.ParameterDefinition t : src.getParameter()) 099 tgt.addParameter(ParameterDefinition30_40.convertParameterDefinition(t)); 100 for (org.hl7.fhir.r4.model.DataRequirement t : src.getDataRequirement()) 101 tgt.addDataRequirement(TriggerDefinition30_40.convertDataRequirement(t)); 102 for (org.hl7.fhir.r4.model.Attachment t : src.getContent()) tgt.addContent(Attachment30_40.convertAttachment(t)); 103 return tgt; 104 } 105 106 public static org.hl7.fhir.r4.model.Library convertLibrary(org.hl7.fhir.dstu3.model.Library src) throws FHIRException { 107 if (src == null) 108 return null; 109 org.hl7.fhir.r4.model.Library tgt = new org.hl7.fhir.r4.model.Library(); 110 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 111 if (src.hasUrl()) 112 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 113 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 114 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 115 if (src.hasVersion()) 116 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 117 if (src.hasName()) 118 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 119 if (src.hasTitle()) 120 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 121 if (src.hasStatus()) 122 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 123 if (src.hasExperimental()) 124 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 125 if (src.hasType()) 126 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 127 if (src.hasDateElement()) 128 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 129 if (src.hasPublisher()) 130 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 131 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 132 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 133 if (src.hasDescription()) 134 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 135 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 136 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 137 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 138 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 139 if (src.hasPurpose()) 140 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 141 if (src.hasUsage()) 142 tgt.setUsageElement(String30_40.convertString(src.getUsageElement())); 143 if (src.hasCopyright()) 144 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 145 if (src.hasApprovalDate()) 146 tgt.setApprovalDateElement(Date30_40.convertDate(src.getApprovalDateElement())); 147 if (src.hasLastReviewDate()) 148 tgt.setLastReviewDateElement(Date30_40.convertDate(src.getLastReviewDateElement())); 149 if (src.hasEffectivePeriod()) 150 tgt.setEffectivePeriod(Period30_40.convertPeriod(src.getEffectivePeriod())); 151 for (org.hl7.fhir.dstu3.model.Contributor t : src.getContributor()) { 152 if (t.getType() == ContributorType.AUTHOR) 153 for (ContactDetail c : t.getContact()) tgt.addAuthor(ContactDetail30_40.convertContactDetail(c)); 154 if (t.getType() == ContributorType.EDITOR) 155 for (ContactDetail c : t.getContact()) tgt.addEditor(ContactDetail30_40.convertContactDetail(c)); 156 if (t.getType() == ContributorType.REVIEWER) 157 for (ContactDetail c : t.getContact()) tgt.addReviewer(ContactDetail30_40.convertContactDetail(c)); 158 if (t.getType() == ContributorType.ENDORSER) 159 for (ContactDetail c : t.getContact()) tgt.addEndorser(ContactDetail30_40.convertContactDetail(c)); 160 } 161 for (org.hl7.fhir.dstu3.model.RelatedArtifact t : src.getRelatedArtifact()) 162 tgt.addRelatedArtifact(RelatedArtifact30_40.convertRelatedArtifact(t)); 163 for (org.hl7.fhir.dstu3.model.ParameterDefinition t : src.getParameter()) 164 tgt.addParameter(ParameterDefinition30_40.convertParameterDefinition(t)); 165 for (org.hl7.fhir.dstu3.model.DataRequirement t : src.getDataRequirement()) 166 tgt.addDataRequirement(TriggerDefinition30_40.convertDataRequirement(t)); 167 for (org.hl7.fhir.dstu3.model.Attachment t : src.getContent()) tgt.addContent(Attachment30_40.convertAttachment(t)); 168 return tgt; 169 } 170}