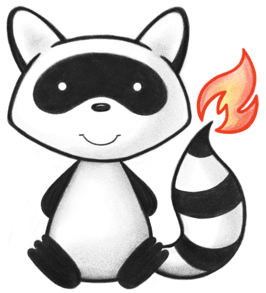
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Address30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class Location30_40 { 015 016 public static org.hl7.fhir.r4.model.Location convertLocation(org.hl7.fhir.dstu3.model.Location src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r4.model.Location tgt = new org.hl7.fhir.r4.model.Location(); 020 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 025 if (src.hasOperationalStatus()) 026 tgt.setOperationalStatus(Coding30_40.convertCoding(src.getOperationalStatus())); 027 if (src.hasName()) 028 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 029 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 030 if (src.hasDescription()) 031 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 032 if (src.hasMode()) 033 tgt.setModeElement(convertLocationMode(src.getModeElement())); 034 if (src.hasType()) 035 tgt.addType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 036 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 037 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 038 if (src.hasAddress()) 039 tgt.setAddress(Address30_40.convertAddress(src.getAddress())); 040 if (src.hasPhysicalType()) 041 tgt.setPhysicalType(CodeableConcept30_40.convertCodeableConcept(src.getPhysicalType())); 042 if (src.hasPosition()) 043 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 044 if (src.hasManagingOrganization()) 045 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 046 if (src.hasPartOf()) 047 tgt.setPartOf(Reference30_40.convertReference(src.getPartOf())); 048 for (org.hl7.fhir.dstu3.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_40.convertReference(t)); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.dstu3.model.Location convertLocation(org.hl7.fhir.r4.model.Location src) throws FHIRException { 053 if (src == null) 054 return null; 055 org.hl7.fhir.dstu3.model.Location tgt = new org.hl7.fhir.dstu3.model.Location(); 056 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 057 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 059 if (src.hasStatus()) 060 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 061 if (src.hasOperationalStatus()) 062 tgt.setOperationalStatus(Coding30_40.convertCoding(src.getOperationalStatus())); 063 if (src.hasName()) 064 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 065 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 066 if (src.hasDescription()) 067 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 068 if (src.hasMode()) 069 tgt.setModeElement(convertLocationMode(src.getModeElement())); 070 if (src.hasType()) 071 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getTypeFirstRep())); 072 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 073 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 074 if (src.hasAddress()) 075 tgt.setAddress(Address30_40.convertAddress(src.getAddress())); 076 if (src.hasPhysicalType()) 077 tgt.setPhysicalType(CodeableConcept30_40.convertCodeableConcept(src.getPhysicalType())); 078 if (src.hasPosition()) 079 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 080 if (src.hasManagingOrganization()) 081 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 082 if (src.hasPartOf()) 083 tgt.setPartOf(Reference30_40.convertReference(src.getPartOf())); 084 for (org.hl7.fhir.r4.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_40.convertReference(t)); 085 return tgt; 086 } 087 088 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Location.LocationModeEnumFactory()); 092 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 093 switch (src.getValue()) { 094 case INSTANCE: 095 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationMode.INSTANCE); 096 break; 097 case KIND: 098 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationMode.KIND); 099 break; 100 default: 101 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationMode.NULL); 102 break; 103 } 104 return tgt; 105 } 106 107 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationMode> src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Location.LocationModeEnumFactory()); 111 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 112 switch (src.getValue()) { 113 case INSTANCE: 114 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.INSTANCE); 115 break; 116 case KIND: 117 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.KIND); 118 break; 119 default: 120 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.NULL); 121 break; 122 } 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r4.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.dstu3.model.Location.LocationPositionComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.r4.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.r4.model.Location.LocationPositionComponent(); 130 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 131 if (src.hasLongitude()) 132 tgt.setLongitudeElement(Decimal30_40.convertDecimal(src.getLongitudeElement())); 133 if (src.hasLatitude()) 134 tgt.setLatitudeElement(Decimal30_40.convertDecimal(src.getLatitudeElement())); 135 if (src.hasAltitude()) 136 tgt.setAltitudeElement(Decimal30_40.convertDecimal(src.getAltitudeElement())); 137 return tgt; 138 } 139 140 public static org.hl7.fhir.dstu3.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.r4.model.Location.LocationPositionComponent src) throws FHIRException { 141 if (src == null) 142 return null; 143 org.hl7.fhir.dstu3.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.dstu3.model.Location.LocationPositionComponent(); 144 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 145 if (src.hasLongitude()) 146 tgt.setLongitudeElement(Decimal30_40.convertDecimal(src.getLongitudeElement())); 147 if (src.hasLatitude()) 148 tgt.setLatitudeElement(Decimal30_40.convertDecimal(src.getLatitudeElement())); 149 if (src.hasAltitude()) 150 tgt.setAltitudeElement(Decimal30_40.convertDecimal(src.getAltitudeElement())); 151 return tgt; 152 } 153 154 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> src) throws FHIRException { 155 if (src == null || src.isEmpty()) 156 return null; 157 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Location.LocationStatusEnumFactory()); 158 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 159 switch (src.getValue()) { 160 case ACTIVE: 161 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationStatus.ACTIVE); 162 break; 163 case SUSPENDED: 164 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationStatus.SUSPENDED); 165 break; 166 case INACTIVE: 167 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationStatus.INACTIVE); 168 break; 169 default: 170 tgt.setValue(org.hl7.fhir.r4.model.Location.LocationStatus.NULL); 171 break; 172 } 173 return tgt; 174 } 175 176 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Location.LocationStatus> src) throws FHIRException { 177 if (src == null || src.isEmpty()) 178 return null; 179 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Location.LocationStatusEnumFactory()); 180 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 181 switch (src.getValue()) { 182 case ACTIVE: 183 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.ACTIVE); 184 break; 185 case SUSPENDED: 186 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.SUSPENDED); 187 break; 188 case INACTIVE: 189 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.INACTIVE); 190 break; 191 default: 192 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.NULL); 193 break; 194 } 195 return tgt; 196 } 197}