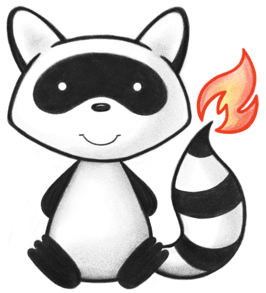
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.PositiveInt30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.CodeableConcept; 012 013public class Media30_40 { 014 015 private static final String CODE_SYSTEM_MEDIA_TYPE = "http://terminology.hl7.org/CodeSystem/media-type"; 016 017 public static org.hl7.fhir.dstu3.model.Media convertMedia(org.hl7.fhir.r4.model.Media src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.dstu3.model.Media tgt = new org.hl7.fhir.dstu3.model.Media(); 021 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) { 023 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 024 } 025 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) { 026 tgt.addBasedOn(Reference30_40.convertReference(t)); 027 } 028 if (src.hasType()) { 029 CodeableConcept type = src.getType(); 030 for (org.hl7.fhir.r4.model.Coding c : type.getCoding()) { 031 if (CODE_SYSTEM_MEDIA_TYPE.equals(c.getSystem())) { 032 tgt.setType(org.hl7.fhir.dstu3.model.Media.DigitalMediaType.fromCode(c.getCode().replace("image", "photo"))); 033 break; 034 } 035 } 036 } 037 if (src.hasModality()) { 038 if (src.hasModality()) 039 tgt.setSubtype(CodeableConcept30_40.convertCodeableConcept(src.getModality())); 040 } 041 if (src.hasView()) { 042 if (src.hasView()) 043 tgt.setView(CodeableConcept30_40.convertCodeableConcept(src.getView())); 044 } 045 if (src.hasSubject()) { 046 if (src.hasSubject()) 047 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 048 } 049 if (src.hasEncounter()) { 050 if (src.hasEncounter()) 051 tgt.setContext(Reference30_40.convertReference(src.getEncounter())); 052 } 053 if (src.hasCreated()) { 054 if (src.hasCreated()) 055 tgt.setOccurrence(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getCreated())); 056 } 057 if (src.hasOperator()) { 058 if (src.hasOperator()) 059 tgt.setOperator(Reference30_40.convertReference(src.getOperator())); 060 } 061 for (CodeableConcept t : src.getReasonCode()) { 062 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 063 } 064 if (src.hasBodySite()) { 065 if (src.hasBodySite()) 066 tgt.setBodySite(CodeableConcept30_40.convertCodeableConcept(src.getBodySite())); 067 } 068 if (src.hasDevice()) { 069 if (src.hasDevice()) 070 tgt.setDevice(Reference30_40.convertReference(src.getDevice())); 071 } 072 if (src.hasHeight()) { 073 if (src.hasHeightElement()) 074 tgt.setHeightElement(PositiveInt30_40.convertPositiveInt(src.getHeightElement())); 075 } 076 if (src.hasWidth()) { 077 if (src.hasWidthElement()) 078 tgt.setWidthElement(PositiveInt30_40.convertPositiveInt(src.getWidthElement())); 079 } 080 if (src.hasFrames()) { 081 if (src.hasFramesElement()) 082 tgt.setFramesElement(PositiveInt30_40.convertPositiveInt(src.getFramesElement())); 083 } 084 if (src.hasDuration()) { 085 tgt.setDuration(src.getDuration().intValue()); 086 } 087 if (src.hasContent()) { 088 if (src.hasContent()) 089 tgt.setContent(Attachment30_40.convertAttachment(src.getContent())); 090 } 091 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) { 092 tgt.addNote(Annotation30_40.convertAnnotation(t)); 093 } 094 return tgt; 095 } 096 097 public static org.hl7.fhir.r4.model.Media convertMedia(org.hl7.fhir.dstu3.model.Media src) throws FHIRException { 098 if (src == null) 099 return null; 100 org.hl7.fhir.r4.model.Media tgt = new org.hl7.fhir.r4.model.Media(); 101 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 102 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) { 103 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 104 } 105 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) { 106 tgt.addBasedOn(Reference30_40.convertReference(t)); 107 } 108 if (src.hasType()) { 109 org.hl7.fhir.r4.model.Coding coding = new org.hl7.fhir.r4.model.Coding(); 110 coding.setSystem(CODE_SYSTEM_MEDIA_TYPE); 111 coding.setCode(src.getType().toCode().replace("photo", "image")); 112 CodeableConcept codeableConcept = new CodeableConcept(coding); 113 tgt.setType(codeableConcept); 114 } 115 if (src.hasSubtype()) { 116 if (src.hasSubtype()) 117 tgt.setModality(CodeableConcept30_40.convertCodeableConcept(src.getSubtype())); 118 } 119 if (src.hasView()) { 120 if (src.hasView()) 121 tgt.setView(CodeableConcept30_40.convertCodeableConcept(src.getView())); 122 } 123 if (src.hasSubject()) { 124 if (src.hasSubject()) 125 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 126 } 127 if (src.hasContext()) { 128 if (src.hasContext()) 129 tgt.setEncounter(Reference30_40.convertReference(src.getContext())); 130 } 131 if (src.hasOccurrence()) { 132 if (src.hasOccurrence()) 133 tgt.setCreated(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getOccurrence())); 134 } 135 if (src.hasOperator()) { 136 if (src.hasOperator()) 137 tgt.setOperator(Reference30_40.convertReference(src.getOperator())); 138 } 139 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) { 140 tgt.addReasonCode(CodeableConcept30_40.convertCodeableConcept(t)); 141 } 142 if (src.hasBodySite()) { 143 if (src.hasBodySite()) 144 tgt.setBodySite(CodeableConcept30_40.convertCodeableConcept(src.getBodySite())); 145 } 146 if (src.hasDevice()) { 147 if (src.hasDevice()) 148 tgt.setDevice(Reference30_40.convertReference(src.getDevice())); 149 } 150 if (src.hasHeight()) { 151 if (src.hasHeightElement()) 152 tgt.setHeightElement(PositiveInt30_40.convertPositiveInt(src.getHeightElement())); 153 } 154 if (src.hasWidth()) { 155 if (src.hasWidthElement()) 156 tgt.setWidthElement(PositiveInt30_40.convertPositiveInt(src.getWidthElement())); 157 } 158 if (src.hasFrames()) { 159 if (src.hasFramesElement()) 160 tgt.setFramesElement(PositiveInt30_40.convertPositiveInt(src.getFramesElement())); 161 } 162 if (src.hasDuration()) { 163 if (src.hasDuration()) 164 tgt.setDuration(src.getDuration()); 165 } 166 if (src.hasContent()) { 167 if (src.hasContent()) 168 tgt.setContent(Attachment30_40.convertAttachment(src.getContent())); 169 } 170 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) { 171 tgt.addNote(Annotation30_40.convertAnnotation(t)); 172 } 173 tgt.setStatus(org.hl7.fhir.r4.model.Media.MediaStatus.COMPLETED); 174 return tgt; 175 } 176}