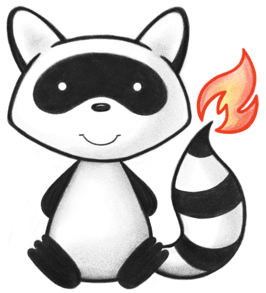
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Ratio30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.dstu3.model.Enumeration; 011import org.hl7.fhir.dstu3.model.Medication; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class Medication30_40 { 015 016 public static org.hl7.fhir.r4.model.Medication convertMedication(org.hl7.fhir.dstu3.model.Medication src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r4.model.Medication tgt = new org.hl7.fhir.r4.model.Medication(); 020 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 021 if (src.hasCode()) 022 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertMedicationStatus(src.getStatusElement())); 025 if (src.hasManufacturer()) 026 tgt.setManufacturer(Reference30_40.convertReference(src.getManufacturer())); 027 if (src.hasForm()) 028 tgt.setForm(CodeableConcept30_40.convertCodeableConcept(src.getForm())); 029 for (org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent t : src.getIngredient()) 030 tgt.addIngredient(convertMedicationIngredientComponent(t)); 031 if (src.hasPackage()) 032 tgt.setBatch(convertMedicationPackageBatchComponent(src.getPackage().getBatchFirstRep())); 033 return tgt; 034 } 035 036 public static org.hl7.fhir.dstu3.model.Medication convertMedication(org.hl7.fhir.r4.model.Medication src) throws FHIRException { 037 if (src == null) 038 return null; 039 org.hl7.fhir.dstu3.model.Medication tgt = new org.hl7.fhir.dstu3.model.Medication(); 040 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 041 if (src.hasCode()) 042 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 043 if (src.hasStatus()) 044 tgt.setStatusElement(convertMedicationStatus(src.getStatusElement())); 045 if (src.hasManufacturer()) 046 tgt.setManufacturer(Reference30_40.convertReference(src.getManufacturer())); 047 if (src.hasForm()) 048 tgt.setForm(CodeableConcept30_40.convertCodeableConcept(src.getForm())); 049 for (org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent t : src.getIngredient()) 050 tgt.addIngredient(convertMedicationIngredientComponent(t)); 051 if (src.hasBatch()) 052 tgt.getPackage().addBatch(convertMedicationPackageBatchComponent(src.getBatch())); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent convertMedicationIngredientComponent(org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent tgt = new org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent(); 060 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 061 if (src.hasItem()) 062 tgt.setItem(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getItem())); 063 if (src.hasIsActive()) 064 tgt.setIsActiveElement(Boolean30_40.convertBoolean(src.getIsActiveElement())); 065 if (src.hasStrength()) 066 tgt.setAmount(Ratio30_40.convertRatio(src.getStrength())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent convertMedicationIngredientComponent(org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent src) throws FHIRException { 071 if (src == null) 072 return null; 073 org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent tgt = new org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent(); 074 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 075 if (src.hasItem()) 076 tgt.setItem(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getItem())); 077 if (src.hasIsActive()) 078 tgt.setIsActiveElement(Boolean30_40.convertBoolean(src.getIsActiveElement())); 079 if (src.hasAmount()) 080 tgt.setStrength(Ratio30_40.convertRatio(src.getAmount())); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent convertMedicationPackageBatchComponent(org.hl7.fhir.r4.model.Medication.MedicationBatchComponent src) throws FHIRException { 085 if (src == null) 086 return null; 087 org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent tgt = new org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent(); 088 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 089 if (src.hasLotNumber()) 090 tgt.setLotNumberElement(String30_40.convertString(src.getLotNumberElement())); 091 if (src.hasExpirationDate()) 092 tgt.setExpirationDateElement(DateTime30_40.convertDateTime(src.getExpirationDateElement())); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.r4.model.Medication.MedicationBatchComponent convertMedicationPackageBatchComponent(org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent src) throws FHIRException { 097 if (src == null) 098 return null; 099 org.hl7.fhir.r4.model.Medication.MedicationBatchComponent tgt = new org.hl7.fhir.r4.model.Medication.MedicationBatchComponent(); 100 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 101 if (src.hasLotNumber()) 102 tgt.setLotNumberElement(String30_40.convertString(src.getLotNumberElement())); 103 if (src.hasExpirationDate()) 104 tgt.setExpirationDateElement(DateTime30_40.convertDateTime(src.getExpirationDateElement())); 105 return tgt; 106 } 107 108 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Medication.MedicationStatus> convertMedicationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Medication.MedicationStatus> src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 Enumeration<Medication.MedicationStatus> tgt = new Enumeration<>(new Medication.MedicationStatusEnumFactory()); 112 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 113 if (src.getValue() == null) { 114 tgt.setValue(null); 115 } else { 116 switch (src.getValue()) { 117 case ACTIVE: 118 tgt.setValue(Medication.MedicationStatus.ACTIVE); 119 break; 120 case INACTIVE: 121 tgt.setValue(Medication.MedicationStatus.INACTIVE); 122 break; 123 case ENTEREDINERROR: 124 tgt.setValue(Medication.MedicationStatus.ENTEREDINERROR); 125 break; 126 default: 127 tgt.setValue(Medication.MedicationStatus.NULL); 128 break; 129 } 130 } 131 return tgt; 132 } 133 134 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Medication.MedicationStatus> convertMedicationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Medication.MedicationStatus> src) throws FHIRException { 135 if (src == null || src.isEmpty()) 136 return null; 137 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Medication.MedicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Medication.MedicationStatusEnumFactory()); 138 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 139 if (src.getValue() == null) { 140 tgt.setValue(null); 141 } else { 142 switch (src.getValue()) { 143 case ACTIVE: 144 tgt.setValue(org.hl7.fhir.r4.model.Medication.MedicationStatus.ACTIVE); 145 break; 146 case INACTIVE: 147 tgt.setValue(org.hl7.fhir.r4.model.Medication.MedicationStatus.INACTIVE); 148 break; 149 case ENTEREDINERROR: 150 tgt.setValue(org.hl7.fhir.r4.model.Medication.MedicationStatus.ENTEREDINERROR); 151 break; 152 default: 153 tgt.setValue(org.hl7.fhir.r4.model.Medication.MedicationStatus.NULL); 154 break; 155 } 156 } 157 return tgt; 158 } 159}