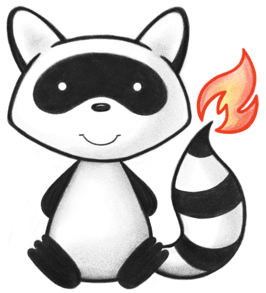
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Dosage30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Annotation30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.SimpleQuantity30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.Enumeration; 014import org.hl7.fhir.r4.model.MedicationDispense; 015 016public class MedicationDispense30_40 { 017 018 public static org.hl7.fhir.dstu3.model.MedicationDispense convertMedicationDispense(org.hl7.fhir.r4.model.MedicationDispense src) throws FHIRException { 019 if (src == null) 020 return null; 021 org.hl7.fhir.dstu3.model.MedicationDispense tgt = new org.hl7.fhir.dstu3.model.MedicationDispense(); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 023 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 024 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 025 for (org.hl7.fhir.r4.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_40.convertReference(t)); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertMedicationDispenseStatus(src.getStatusElement())); 028 if (src.hasCategory()) 029 tgt.setCategory(CodeableConcept30_40.convertCodeableConcept(src.getCategory())); 030 if (src.hasMedication()) 031 tgt.setMedication(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMedication())); 032 if (src.hasSubject()) 033 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 034 if (src.hasContext()) 035 tgt.setContext(Reference30_40.convertReference(src.getContext())); 036 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInformation()) 037 tgt.addSupportingInformation(Reference30_40.convertReference(t)); 038 for (org.hl7.fhir.r4.model.MedicationDispense.MedicationDispensePerformerComponent t : src.getPerformer()) 039 tgt.addPerformer(convertMedicationDispensePerformerComponent(t)); 040 for (org.hl7.fhir.r4.model.Reference t : src.getAuthorizingPrescription()) 041 tgt.addAuthorizingPrescription(Reference30_40.convertReference(t)); 042 if (src.hasType()) 043 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 044 if (src.hasQuantity()) 045 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 046 if (src.hasDaysSupply()) 047 tgt.setDaysSupply(SimpleQuantity30_40.convertSimpleQuantity(src.getDaysSupply())); 048 if (src.hasWhenPrepared()) 049 tgt.setWhenPreparedElement(DateTime30_40.convertDateTime(src.getWhenPreparedElement())); 050 if (src.hasWhenHandedOver()) 051 tgt.setWhenHandedOverElement(DateTime30_40.convertDateTime(src.getWhenHandedOverElement())); 052 if (src.hasDestination()) 053 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 054 for (org.hl7.fhir.r4.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference30_40.convertReference(t)); 055 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 056 for (org.hl7.fhir.r4.model.Dosage t : src.getDosageInstruction()) 057 tgt.addDosageInstruction(Dosage30_40.convertDosage(t)); 058 if (src.hasSubstitution()) 059 tgt.setSubstitution(convertMedicationDispenseSubstitutionComponent(src.getSubstitution())); 060 for (org.hl7.fhir.r4.model.Reference t : src.getDetectedIssue()) 061 tgt.addDetectedIssue(Reference30_40.convertReference(t)); 062 for (org.hl7.fhir.r4.model.Reference t : src.getEventHistory()) 063 tgt.addEventHistory(Reference30_40.convertReference(t)); 064 return tgt; 065 } 066 067 public static org.hl7.fhir.r4.model.MedicationDispense convertMedicationDispense(org.hl7.fhir.dstu3.model.MedicationDispense src) throws FHIRException { 068 if (src == null) 069 return null; 070 org.hl7.fhir.r4.model.MedicationDispense tgt = new org.hl7.fhir.r4.model.MedicationDispense(); 071 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 072 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 073 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 074 for (org.hl7.fhir.dstu3.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_40.convertReference(t)); 075 if (src.hasStatus()) 076 tgt.setStatusElement(convertMedicationDispenseStatus(src.getStatusElement())); 077 if (src.hasCategory()) 078 tgt.setCategory(CodeableConcept30_40.convertCodeableConcept(src.getCategory())); 079 if (src.hasMedication()) 080 tgt.setMedication(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getMedication())); 081 if (src.hasSubject()) 082 tgt.setSubject(Reference30_40.convertReference(src.getSubject())); 083 if (src.hasContext()) 084 tgt.setContext(Reference30_40.convertReference(src.getContext())); 085 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInformation()) 086 tgt.addSupportingInformation(Reference30_40.convertReference(t)); 087 for (org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispensePerformerComponent t : src.getPerformer()) 088 tgt.addPerformer(convertMedicationDispensePerformerComponent(t)); 089 for (org.hl7.fhir.dstu3.model.Reference t : src.getAuthorizingPrescription()) 090 tgt.addAuthorizingPrescription(Reference30_40.convertReference(t)); 091 if (src.hasType()) 092 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 093 if (src.hasQuantity()) 094 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 095 if (src.hasDaysSupply()) 096 tgt.setDaysSupply(SimpleQuantity30_40.convertSimpleQuantity(src.getDaysSupply())); 097 if (src.hasWhenPrepared()) 098 tgt.setWhenPreparedElement(DateTime30_40.convertDateTime(src.getWhenPreparedElement())); 099 if (src.hasWhenHandedOver()) 100 tgt.setWhenHandedOverElement(DateTime30_40.convertDateTime(src.getWhenHandedOverElement())); 101 if (src.hasDestination()) 102 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 103 for (org.hl7.fhir.dstu3.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference30_40.convertReference(t)); 104 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_40.convertAnnotation(t)); 105 for (org.hl7.fhir.dstu3.model.Dosage t : src.getDosageInstruction()) 106 tgt.addDosageInstruction(Dosage30_40.convertDosage(t)); 107 if (src.hasSubstitution()) 108 tgt.setSubstitution(convertMedicationDispenseSubstitutionComponent(src.getSubstitution())); 109 for (org.hl7.fhir.dstu3.model.Reference t : src.getDetectedIssue()) 110 tgt.addDetectedIssue(Reference30_40.convertReference(t)); 111 for (org.hl7.fhir.dstu3.model.Reference t : src.getEventHistory()) 112 tgt.addEventHistory(Reference30_40.convertReference(t)); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispensePerformerComponent convertMedicationDispensePerformerComponent(org.hl7.fhir.r4.model.MedicationDispense.MedicationDispensePerformerComponent src) throws FHIRException { 117 if (src == null) 118 return null; 119 org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispensePerformerComponent tgt = new org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispensePerformerComponent(); 120 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 121 if (src.hasActor()) 122 tgt.setActor(Reference30_40.convertReference(src.getActor())); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r4.model.MedicationDispense.MedicationDispensePerformerComponent convertMedicationDispensePerformerComponent(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispensePerformerComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.r4.model.MedicationDispense.MedicationDispensePerformerComponent tgt = new org.hl7.fhir.r4.model.MedicationDispense.MedicationDispensePerformerComponent(); 130 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 131 if (src.hasActor()) 132 tgt.setActor(Reference30_40.convertReference(src.getActor())); 133 return tgt; 134 } 135 136 private static org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseStatus> convertMedicationDispenseStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus> src) { 137 if (src == null) 138 return null; 139 Enumeration<MedicationDispense.MedicationDispenseStatus> tgt = new Enumeration<>(new MedicationDispense.MedicationDispenseStatusEnumFactory()); 140 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 141 if (src.getValue() == null) { 142 tgt.setValue(null); 143 } else { 144 switch (src.getValue()) { 145 case COMPLETED: 146 tgt.setValue(MedicationDispense.MedicationDispenseStatus.COMPLETED); 147 break; 148 case ENTEREDINERROR: 149 tgt.setValue(MedicationDispense.MedicationDispenseStatus.ENTEREDINERROR); 150 break; 151 case INPROGRESS: 152 tgt.setValue(MedicationDispense.MedicationDispenseStatus.INPROGRESS); 153 break; 154 case NULL: 155 tgt.setValue(MedicationDispense.MedicationDispenseStatus.NULL); 156 break; 157 case ONHOLD: 158 tgt.setValue(MedicationDispense.MedicationDispenseStatus.ONHOLD); 159 break; 160 case PREPARATION: 161 tgt.setValue(MedicationDispense.MedicationDispenseStatus.PREPARATION); 162 break; 163 case STOPPED: 164 tgt.setValue(MedicationDispense.MedicationDispenseStatus.STOPPED); 165 break; 166 } 167 } 168 return tgt; 169 } 170 171 private static org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus> convertMedicationDispenseStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseStatus> src) { 172 if (src == null) 173 return null; 174 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatusEnumFactory()); 175 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 176 if (src.getValue() == null) { 177 tgt.setValue(null); 178 } else { 179 switch (src.getValue()) { 180 case CANCELLED: 181 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.STOPPED); 182 break; 183 case COMPLETED: 184 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.COMPLETED); 185 break; 186 case DECLINED: 187 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.STOPPED); 188 break; 189 case ENTEREDINERROR: 190 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.ENTEREDINERROR); 191 break; 192 case INPROGRESS: 193 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.INPROGRESS); 194 break; 195 case NULL: 196 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.NULL); 197 break; 198 case ONHOLD: 199 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.ONHOLD); 200 break; 201 case PREPARATION: 202 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.PREPARATION); 203 break; 204 case STOPPED: 205 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.STOPPED); 206 break; 207 case UNKNOWN: 208 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseStatus.NULL); 209 break; 210 } 211 } 212 return tgt; 213 } 214 215 public static org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseSubstitutionComponent convertMedicationDispenseSubstitutionComponent(org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseSubstitutionComponent src) throws FHIRException { 216 if (src == null) 217 return null; 218 org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseSubstitutionComponent tgt = new org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseSubstitutionComponent(); 219 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 220 if (src.hasWasSubstituted()) 221 tgt.setWasSubstitutedElement(Boolean30_40.convertBoolean(src.getWasSubstitutedElement())); 222 if (src.hasType()) 223 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 224 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReason()) 225 tgt.addReason(CodeableConcept30_40.convertCodeableConcept(t)); 226 for (org.hl7.fhir.dstu3.model.Reference t : src.getResponsibleParty()) 227 tgt.addResponsibleParty(Reference30_40.convertReference(t)); 228 return tgt; 229 } 230 231 public static org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseSubstitutionComponent convertMedicationDispenseSubstitutionComponent(org.hl7.fhir.r4.model.MedicationDispense.MedicationDispenseSubstitutionComponent src) throws FHIRException { 232 if (src == null) 233 return null; 234 org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseSubstitutionComponent tgt = new org.hl7.fhir.dstu3.model.MedicationDispense.MedicationDispenseSubstitutionComponent(); 235 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 236 if (src.hasWasSubstituted()) 237 tgt.setWasSubstitutedElement(Boolean30_40.convertBoolean(src.getWasSubstitutedElement())); 238 if (src.hasType()) 239 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 240 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReason()) 241 tgt.addReason(CodeableConcept30_40.convertCodeableConcept(t)); 242 for (org.hl7.fhir.r4.model.Reference t : src.getResponsibleParty()) 243 tgt.addResponsibleParty(Reference30_40.convertReference(t)); 244 return tgt; 245 } 246}