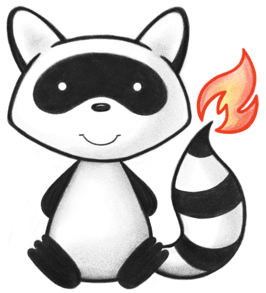
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r4.model.Enumeration; 011import org.hl7.fhir.r4.model.PaymentNotice; 012 013public class PaymentNotice30_40 { 014 015 public static org.hl7.fhir.r4.model.PaymentNotice convertPaymentNotice(org.hl7.fhir.dstu3.model.PaymentNotice src) throws FHIRException { 016 if (src == null) 017 return null; 018 org.hl7.fhir.r4.model.PaymentNotice tgt = new org.hl7.fhir.r4.model.PaymentNotice(); 019 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 022 if (src.hasStatus()) 023 tgt.setStatusElement(convertPaymentNoticeStatus(src.getStatusElement())); 024 if (src.hasRequest()) 025 tgt.setRequest(Reference30_40.convertReference(src.getRequest())); 026 if (src.hasResponse()) 027 tgt.setResponse(Reference30_40.convertReference(src.getResponse())); 028 if (src.hasStatusDate()) 029 tgt.setPaymentDateElement(Date30_40.convertDate(src.getStatusDateElement())); 030 if (src.hasCreated()) 031 tgt.setCreatedElement(DateTime30_40.convertDateTime(src.getCreatedElement())); 032 if (src.hasTarget()) 033 tgt.setRecipient(Reference30_40.convertReference(src.getTarget())); 034 if (src.hasProvider()) 035 tgt.setProvider(Reference30_40.convertReference(src.getProvider())); 036 if (src.hasPaymentStatus()) 037 tgt.setPaymentStatus(CodeableConcept30_40.convertCodeableConcept(src.getPaymentStatus())); 038 return tgt; 039 } 040 041 public static org.hl7.fhir.dstu3.model.PaymentNotice convertPaymentNotice(org.hl7.fhir.r4.model.PaymentNotice src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.dstu3.model.PaymentNotice tgt = new org.hl7.fhir.dstu3.model.PaymentNotice(); 045 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 046 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 047 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 048 if (src.hasStatus()) 049 tgt.setStatusElement(convertPaymentNoticeStatus(src.getStatusElement())); 050 if (src.hasRequest()) 051 tgt.setRequest(Reference30_40.convertReference(src.getRequest())); 052 if (src.hasResponse()) 053 tgt.setResponse(Reference30_40.convertReference(src.getResponse())); 054 if (src.hasPaymentDate()) 055 tgt.setStatusDateElement(Date30_40.convertDate(src.getPaymentDateElement())); 056 if (src.hasCreated()) 057 tgt.setCreatedElement(DateTime30_40.convertDateTime(src.getCreatedElement())); 058 if (src.hasRecipient()) 059 tgt.setTarget(Reference30_40.convertReference(src.getRecipient())); 060 if (src.hasProvider()) 061 tgt.setProvider(Reference30_40.convertReference(src.getProvider())); 062 if (src.hasPaymentStatus()) 063 tgt.setPaymentStatus(CodeableConcept30_40.convertCodeableConcept(src.getPaymentStatus())); 064 return tgt; 065 } 066 067 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.PaymentNotice.PaymentNoticeStatus> convertPaymentNoticeStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus> src) throws FHIRException { 068 if (src == null || src.isEmpty()) 069 return null; 070 Enumeration<PaymentNotice.PaymentNoticeStatus> tgt = new Enumeration<>(new PaymentNotice.PaymentNoticeStatusEnumFactory()); 071 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 072 if (src.getValue() == null) { 073 tgt.setValue(null); 074 } else { 075 switch (src.getValue()) { 076 case ACTIVE: 077 tgt.setValue(PaymentNotice.PaymentNoticeStatus.ACTIVE); 078 break; 079 case CANCELLED: 080 tgt.setValue(PaymentNotice.PaymentNoticeStatus.CANCELLED); 081 break; 082 case DRAFT: 083 tgt.setValue(PaymentNotice.PaymentNoticeStatus.DRAFT); 084 break; 085 case ENTEREDINERROR: 086 tgt.setValue(PaymentNotice.PaymentNoticeStatus.ENTEREDINERROR); 087 break; 088 default: 089 tgt.setValue(PaymentNotice.PaymentNoticeStatus.NULL); 090 break; 091 } 092 } 093 return tgt; 094 } 095 096 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus> convertPaymentNoticeStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.PaymentNotice.PaymentNoticeStatus> src) throws FHIRException { 097 if (src == null || src.isEmpty()) 098 return null; 099 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatusEnumFactory()); 100 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 101 if (src.getValue() == null) { 102 tgt.setValue(null); 103 } else { 104 switch (src.getValue()) { 105 case ACTIVE: 106 tgt.setValue(org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus.ACTIVE); 107 break; 108 case CANCELLED: 109 tgt.setValue(org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus.CANCELLED); 110 break; 111 case DRAFT: 112 tgt.setValue(org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus.DRAFT); 113 break; 114 case ENTEREDINERROR: 115 tgt.setValue(org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus.ENTEREDINERROR); 116 break; 117 default: 118 tgt.setValue(org.hl7.fhir.dstu3.model.PaymentNotice.PaymentNoticeStatus.NULL); 119 break; 120 } 121 } 122 return tgt; 123 } 124}