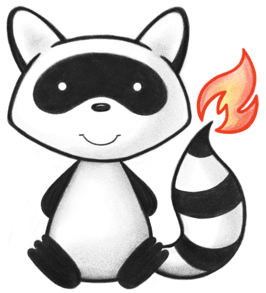
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Address30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.HumanName30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.Enumeration; 014import org.hl7.fhir.r4.model.Person; 015 016public class Person30_40 { 017 018 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 Enumeration<Person.IdentityAssuranceLevel> tgt = new Enumeration<>(new Person.IdentityAssuranceLevelEnumFactory()); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 023 if (src.getValue() == null) { 024 tgt.setValue(null); 025 } else { 026 switch (src.getValue()) { 027 case LEVEL1: 028 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL1); 029 break; 030 case LEVEL2: 031 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL2); 032 break; 033 case LEVEL3: 034 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL3); 035 break; 036 case LEVEL4: 037 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL4); 038 break; 039 default: 040 tgt.setValue(Person.IdentityAssuranceLevel.NULL); 041 break; 042 } 043 } 044 return tgt; 045 } 046 047 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevelEnumFactory()); 051 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 052 if (src.getValue() == null) { 053 tgt.setValue(null); 054 } else { 055 switch (src.getValue()) { 056 case LEVEL1: 057 tgt.setValue(org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel.LEVEL1); 058 break; 059 case LEVEL2: 060 tgt.setValue(org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel.LEVEL2); 061 break; 062 case LEVEL3: 063 tgt.setValue(org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel.LEVEL3); 064 break; 065 case LEVEL4: 066 tgt.setValue(org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel.LEVEL4); 067 break; 068 default: 069 tgt.setValue(org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel.NULL); 070 break; 071 } 072 } 073 return tgt; 074 } 075 076 public static org.hl7.fhir.r4.model.Person convertPerson(org.hl7.fhir.dstu3.model.Person src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.r4.model.Person tgt = new org.hl7.fhir.r4.model.Person(); 080 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 081 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 082 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 083 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) tgt.addName(HumanName30_40.convertHumanName(t)); 084 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 085 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 086 if (src.hasGender()) 087 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 088 if (src.hasBirthDate()) 089 tgt.setBirthDateElement(Date30_40.convertDate(src.getBirthDateElement())); 090 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addAddress(Address30_40.convertAddress(t)); 091 if (src.hasPhoto()) 092 tgt.setPhoto(Attachment30_40.convertAttachment(src.getPhoto())); 093 if (src.hasManagingOrganization()) 094 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 095 if (src.hasActive()) 096 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 097 for (org.hl7.fhir.dstu3.model.Person.PersonLinkComponent t : src.getLink()) 098 tgt.addLink(convertPersonLinkComponent(t)); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.dstu3.model.Person convertPerson(org.hl7.fhir.r4.model.Person src) throws FHIRException { 103 if (src == null) 104 return null; 105 org.hl7.fhir.dstu3.model.Person tgt = new org.hl7.fhir.dstu3.model.Person(); 106 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 107 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 108 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 109 for (org.hl7.fhir.r4.model.HumanName t : src.getName()) tgt.addName(HumanName30_40.convertHumanName(t)); 110 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 111 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 112 if (src.hasGender()) 113 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 114 if (src.hasBirthDate()) 115 tgt.setBirthDateElement(Date30_40.convertDate(src.getBirthDateElement())); 116 for (org.hl7.fhir.r4.model.Address t : src.getAddress()) tgt.addAddress(Address30_40.convertAddress(t)); 117 if (src.hasPhoto()) 118 tgt.setPhoto(Attachment30_40.convertAttachment(src.getPhoto())); 119 if (src.hasManagingOrganization()) 120 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 121 if (src.hasActive()) 122 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 123 for (org.hl7.fhir.r4.model.Person.PersonLinkComponent t : src.getLink()) tgt.addLink(convertPersonLinkComponent(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.r4.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.dstu3.model.Person.PersonLinkComponent src) throws FHIRException { 128 if (src == null) 129 return null; 130 org.hl7.fhir.r4.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.r4.model.Person.PersonLinkComponent(); 131 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 132 if (src.hasTarget()) 133 tgt.setTarget(Reference30_40.convertReference(src.getTarget())); 134 if (src.hasAssurance()) 135 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 136 return tgt; 137 } 138 139 public static org.hl7.fhir.dstu3.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.r4.model.Person.PersonLinkComponent src) throws FHIRException { 140 if (src == null) 141 return null; 142 org.hl7.fhir.dstu3.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.dstu3.model.Person.PersonLinkComponent(); 143 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 144 if (src.hasTarget()) 145 tgt.setTarget(Reference30_40.convertReference(src.getTarget())); 146 if (src.hasAssurance()) 147 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 148 return tgt; 149 } 150}