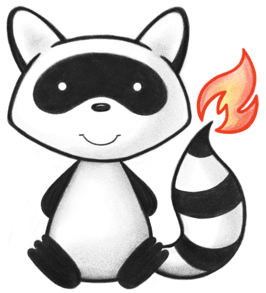
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Address30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.HumanName30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class Practitioner30_40 { 017 018 public static org.hl7.fhir.r4.model.Practitioner convertPractitioner(org.hl7.fhir.dstu3.model.Practitioner src) throws FHIRException { 019 if (src == null) 020 return null; 021 org.hl7.fhir.r4.model.Practitioner tgt = new org.hl7.fhir.r4.model.Practitioner(); 022 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 023 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 024 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 025 if (src.hasActive()) 026 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 027 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) tgt.addName(HumanName30_40.convertHumanName(t)); 028 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 029 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 030 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addAddress(Address30_40.convertAddress(t)); 031 if (src.hasGender()) 032 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 033 if (src.hasBirthDate()) 034 tgt.setBirthDateElement(Date30_40.convertDate(src.getBirthDateElement())); 035 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment30_40.convertAttachment(t)); 036 for (org.hl7.fhir.dstu3.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 037 tgt.addQualification(convertPractitionerQualificationComponent(t)); 038 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCommunication()) 039 tgt.addCommunication(CodeableConcept30_40.convertCodeableConcept(t)); 040 return tgt; 041 } 042 043 public static org.hl7.fhir.dstu3.model.Practitioner convertPractitioner(org.hl7.fhir.r4.model.Practitioner src) throws FHIRException { 044 if (src == null) 045 return null; 046 org.hl7.fhir.dstu3.model.Practitioner tgt = new org.hl7.fhir.dstu3.model.Practitioner(); 047 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 048 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 049 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 050 if (src.hasActive()) 051 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 052 for (org.hl7.fhir.r4.model.HumanName t : src.getName()) tgt.addName(HumanName30_40.convertHumanName(t)); 053 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 054 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 055 for (org.hl7.fhir.r4.model.Address t : src.getAddress()) tgt.addAddress(Address30_40.convertAddress(t)); 056 if (src.hasGender()) 057 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 058 if (src.hasBirthDate()) 059 tgt.setBirthDateElement(Date30_40.convertDate(src.getBirthDateElement())); 060 for (org.hl7.fhir.r4.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment30_40.convertAttachment(t)); 061 for (org.hl7.fhir.r4.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 062 tgt.addQualification(convertPractitionerQualificationComponent(t)); 063 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCommunication()) 064 tgt.addCommunication(CodeableConcept30_40.convertCodeableConcept(t)); 065 return tgt; 066 } 067 068 public static org.hl7.fhir.dstu3.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.r4.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 069 if (src == null) 070 return null; 071 org.hl7.fhir.dstu3.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.dstu3.model.Practitioner.PractitionerQualificationComponent(); 072 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 073 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 074 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 075 if (src.hasCode()) 076 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 077 if (src.hasPeriod()) 078 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 079 if (src.hasIssuer()) 080 tgt.setIssuer(Reference30_40.convertReference(src.getIssuer())); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.r4.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.dstu3.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 085 if (src == null) 086 return null; 087 org.hl7.fhir.r4.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.r4.model.Practitioner.PractitionerQualificationComponent(); 088 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 089 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 090 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 091 if (src.hasCode()) 092 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 093 if (src.hasPeriod()) 094 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 095 if (src.hasIssuer()) 096 tgt.setIssuer(Reference30_40.convertReference(src.getIssuer())); 097 return tgt; 098 } 099}