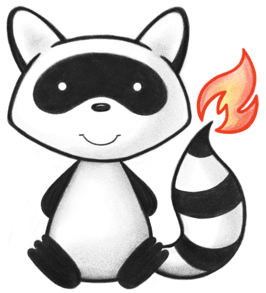
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Extension30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 017import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 018import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r4.model.Enumeration; 021import org.hl7.fhir.r4.model.Questionnaire; 022 023public class Questionnaire30_40 { 024 025 public static org.hl7.fhir.r4.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu3.model.Questionnaire src) throws FHIRException { 026 if (src == null) 027 return null; 028 org.hl7.fhir.r4.model.Questionnaire tgt = new org.hl7.fhir.r4.model.Questionnaire(); 029 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 030 if (src.hasUrl()) 031 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 032 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 033 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 034 if (src.hasVersion()) 035 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 036 if (src.hasName()) 037 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 038 if (src.hasTitle()) 039 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 040 if (src.hasStatus()) 041 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 042 if (src.hasExperimental()) 043 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 044 if (src.hasDateElement()) 045 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 046 if (src.hasPublisher()) 047 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 048 if (src.hasDescription()) 049 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 050 if (src.hasPurpose()) 051 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 052 if (src.hasApprovalDate()) 053 tgt.setApprovalDateElement(Date30_40.convertDate(src.getApprovalDateElement())); 054 if (src.hasLastReviewDate()) 055 tgt.setLastReviewDateElement(Date30_40.convertDate(src.getLastReviewDateElement())); 056 if (src.hasEffectivePeriod()) 057 tgt.setEffectivePeriod(Period30_40.convertPeriod(src.getEffectivePeriod())); 058 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 059 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 060 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 061 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 062 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 063 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 064 if (src.hasCopyright()) 065 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 066 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 067 for (org.hl7.fhir.dstu3.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 068 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 069 tgt.addItem(convertQuestionnaireItemComponent(t)); 070 return tgt; 071 } 072 073 public static org.hl7.fhir.dstu3.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r4.model.Questionnaire src) throws FHIRException { 074 if (src == null) 075 return null; 076 org.hl7.fhir.dstu3.model.Questionnaire tgt = new org.hl7.fhir.dstu3.model.Questionnaire(); 077 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 078 if (src.hasUrl()) 079 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 080 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 081 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 082 if (src.hasVersion()) 083 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 084 if (src.hasName()) 085 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 086 if (src.hasTitle()) 087 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 088 if (src.hasStatus()) 089 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 090 if (src.hasExperimental()) 091 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 092 if (src.hasDateElement()) 093 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 094 if (src.hasPublisher()) 095 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 096 if (src.hasDescription()) 097 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 098 if (src.hasPurpose()) 099 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 100 if (src.hasApprovalDate()) 101 tgt.setApprovalDateElement(Date30_40.convertDate(src.getApprovalDateElement())); 102 if (src.hasLastReviewDate()) 103 tgt.setLastReviewDateElement(Date30_40.convertDate(src.getLastReviewDateElement())); 104 if (src.hasEffectivePeriod()) 105 tgt.setEffectivePeriod(Period30_40.convertPeriod(src.getEffectivePeriod())); 106 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 107 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 108 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 109 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 110 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 111 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 112 if (src.hasCopyright()) 113 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 114 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 115 for (org.hl7.fhir.r4.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 116 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 117 tgt.addItem(convertQuestionnaireItemComponent(t)); 118 return tgt; 119 } 120 121 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 122 if (src == null) 123 return null; 124 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent(); 125 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 126 if (src.hasLinkId()) 127 tgt.setLinkIdElement(String30_40.convertString(src.getLinkIdElement())); 128 if (src.hasDefinition()) 129 tgt.setDefinitionElement(Uri30_40.convertUri(src.getDefinitionElement())); 130 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 131 if (src.hasPrefix()) 132 tgt.setPrefixElement(String30_40.convertString(src.getPrefixElement())); 133 if (src.hasText()) 134 tgt.setTextElement(String30_40.convertString(src.getTextElement())); 135 if (src.hasType()) 136 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 137 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 138 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 139 if (src.hasRequired()) 140 tgt.setRequiredElement(Boolean30_40.convertBoolean(src.getRequiredElement())); 141 if (src.hasRepeats()) 142 tgt.setRepeatsElement(Boolean30_40.convertBoolean(src.getRepeatsElement())); 143 if (src.hasReadOnly()) 144 tgt.setReadOnlyElement(Boolean30_40.convertBoolean(src.getReadOnlyElement())); 145 if (src.hasMaxLength()) 146 tgt.setMaxLengthElement(Integer30_40.convertInteger(src.getMaxLengthElement())); 147 if (src.hasAnswerValueSet()) 148 tgt.setOptions(Reference30_40.convertCanonicalToReference(src.getAnswerValueSetElement())); 149 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 150 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 151 if (src.hasInitial()) 152 tgt.setInitial(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getInitialFirstRep().getValue())); 153 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 154 tgt.addItem(convertQuestionnaireItemComponent(t)); 155 return tgt; 156 } 157 158 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 159 if (src == null) 160 return null; 161 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent(); 162 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 163 if (src.hasLinkId()) 164 tgt.setLinkIdElement(String30_40.convertString(src.getLinkIdElement())); 165 if (src.hasDefinition()) 166 tgt.setDefinitionElement(Uri30_40.convertUri(src.getDefinitionElement())); 167 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_40.convertCoding(t)); 168 if (src.hasPrefix()) 169 tgt.setPrefixElement(String30_40.convertString(src.getPrefixElement())); 170 if (src.hasText()) 171 tgt.setTextElement(String30_40.convertString(src.getTextElement())); 172 if (src.hasType()) 173 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 174 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 175 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 176 tgt.setEnableBehavior(Questionnaire.EnableWhenBehavior.ANY); 177 if (src.hasRequired()) 178 tgt.setRequiredElement(Boolean30_40.convertBoolean(src.getRequiredElement())); 179 if (src.hasRepeats()) 180 tgt.setRepeatsElement(Boolean30_40.convertBoolean(src.getRepeatsElement())); 181 if (src.hasReadOnly()) 182 tgt.setReadOnlyElement(Boolean30_40.convertBoolean(src.getReadOnlyElement())); 183 if (src.hasMaxLength()) 184 tgt.setMaxLengthElement(Integer30_40.convertInteger(src.getMaxLengthElement())); 185 if (src.hasOptions()) 186 tgt.setAnswerValueSetElement(Reference30_40.convertReferenceToCanonical(src.getOptions())); 187 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 188 tgt.addAnswerOption(convertQuestionnaireItemOptionComponent(t)); 189 if (src.hasInitial()) 190 tgt.addInitial().setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getInitial())); 191 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 192 tgt.addItem(convertQuestionnaireItemComponent(t)); 193 for (org.hl7.fhir.dstu3.model.Extension t : src.getModifierExtension()) { 194 tgt.addModifierExtension(Extension30_40.convertExtension(t)); 195 } 196 return tgt; 197 } 198 199 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 200 if (src == null) 201 return null; 202 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 203 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 204 if (src.hasQuestion()) 205 tgt.setQuestionElement(String30_40.convertString(src.getQuestionElement())); 206 if (src.hasOperator() && src.getOperator() == org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.EXISTS) 207 tgt.setHasAnswer(src.getAnswerBooleanType().getValue()); 208 else if (src.hasAnswer()) 209 tgt.setAnswer(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getAnswer())); 210 return tgt; 211 } 212 213 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 214 if (src == null) 215 return null; 216 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 217 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 218 if (src.hasQuestion()) 219 tgt.setQuestionElement(String30_40.convertString(src.getQuestionElement())); 220 if (src.hasHasAnswer()) { 221 tgt.setOperator(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.EXISTS); 222 if (src.hasHasAnswerElement()) 223 tgt.setAnswer(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getHasAnswerElement())); 224 } else if (src.hasAnswer()) { 225 tgt.setOperator(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.EQUAL); 226 if (src.hasAnswer()) 227 tgt.setAnswer(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getAnswer())); 228 } 229 return tgt; 230 } 231 232 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 233 if (src == null) 234 return null; 235 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 236 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 237 if (src.hasValue()) 238 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 239 return tgt; 240 } 241 242 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 243 if (src == null) 244 return null; 245 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent(); 246 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 247 if (src.hasValue()) 248 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 249 return tgt; 250 } 251 252 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 253 if (src == null || src.isEmpty()) 254 return null; 255 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 256 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 257 if (src.getValue() == null) { 258 tgt.setValue(null); 259 } else { 260 switch (src.getValue()) { 261 case GROUP: 262 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 263 break; 264 case DISPLAY: 265 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 266 break; 267 case QUESTION: 268 tgt.setValue(Questionnaire.QuestionnaireItemType.QUESTION); 269 break; 270 case BOOLEAN: 271 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 272 break; 273 case DECIMAL: 274 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 275 break; 276 case INTEGER: 277 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 278 break; 279 case DATE: 280 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 281 break; 282 case DATETIME: 283 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 284 break; 285 case TIME: 286 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 287 break; 288 case STRING: 289 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 290 break; 291 case TEXT: 292 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 293 break; 294 case URL: 295 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 296 break; 297 case CHOICE: 298 tgt.setValue(Questionnaire.QuestionnaireItemType.CHOICE); 299 break; 300 case OPENCHOICE: 301 tgt.setValue(Questionnaire.QuestionnaireItemType.OPENCHOICE); 302 break; 303 case ATTACHMENT: 304 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 305 break; 306 case REFERENCE: 307 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 308 break; 309 case QUANTITY: 310 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 311 break; 312 default: 313 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 314 break; 315 } 316 } 317 return tgt; 318 } 319 320 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 321 if (src == null || src.isEmpty()) 322 return null; 323 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 324 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 325 if (src.getValue() == null) { 326 tgt.setValue(null); 327 } else { 328 switch (src.getValue()) { 329 case GROUP: 330 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.GROUP); 331 break; 332 case DISPLAY: 333 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DISPLAY); 334 break; 335 case QUESTION: 336 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.QUESTION); 337 break; 338 case BOOLEAN: 339 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.BOOLEAN); 340 break; 341 case DECIMAL: 342 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DECIMAL); 343 break; 344 case INTEGER: 345 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.INTEGER); 346 break; 347 case DATE: 348 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DATE); 349 break; 350 case DATETIME: 351 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DATETIME); 352 break; 353 case TIME: 354 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.TIME); 355 break; 356 case STRING: 357 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.STRING); 358 break; 359 case TEXT: 360 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.TEXT); 361 break; 362 case URL: 363 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.URL); 364 break; 365 case CHOICE: 366 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.CHOICE); 367 break; 368 case OPENCHOICE: 369 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.OPENCHOICE); 370 break; 371 case ATTACHMENT: 372 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.ATTACHMENT); 373 break; 374 case REFERENCE: 375 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.REFERENCE); 376 break; 377 case QUANTITY: 378 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.QUANTITY); 379 break; 380 default: 381 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.NULL); 382 break; 383 } 384 } 385 return tgt; 386 } 387}