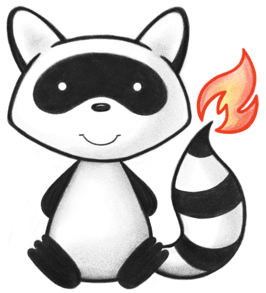
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Address30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Attachment30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.HumanName30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r4.model.CodeableConcept; 017 018public class RelatedPerson30_40 { 019 020 public static org.hl7.fhir.dstu3.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.r4.model.RelatedPerson src) throws FHIRException { 021 if (src == null) 022 return null; 023 org.hl7.fhir.dstu3.model.RelatedPerson tgt = new org.hl7.fhir.dstu3.model.RelatedPerson(); 024 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 025 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) { 026 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 027 } 028 if (src.hasActive()) { 029 if (src.hasActiveElement()) 030 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 031 } 032 if (src.hasPatient()) { 033 if (src.hasPatient()) 034 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 035 } 036 List<CodeableConcept> relationships = src.getRelationship(); 037 if (relationships.size() > 0) { 038 tgt.setRelationship(CodeableConcept30_40.convertCodeableConcept(relationships.get(0))); 039 if (relationships.size() > 1) { 040 } 041 } 042 for (org.hl7.fhir.r4.model.HumanName t : src.getName()) { 043 tgt.addName(HumanName30_40.convertHumanName(t)); 044 } 045 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) { 046 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 047 } 048 if (src.hasGender()) { 049 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 050 } 051 if (src.hasBirthDate()) { 052 tgt.setBirthDate(src.getBirthDate()); 053 } 054 for (org.hl7.fhir.r4.model.Address t : src.getAddress()) { 055 tgt.addAddress(Address30_40.convertAddress(t)); 056 } 057 for (org.hl7.fhir.r4.model.Attachment t : src.getPhoto()) { 058 tgt.addPhoto(Attachment30_40.convertAttachment(t)); 059 } 060 if (src.hasPeriod()) { 061 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 062 } 063 return tgt; 064 } 065 066 public static org.hl7.fhir.r4.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.dstu3.model.RelatedPerson src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.r4.model.RelatedPerson tgt = new org.hl7.fhir.r4.model.RelatedPerson(); 070 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 071 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) { 072 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 073 } 074 if (src.hasActive()) { 075 if (src.hasActiveElement()) 076 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 077 } 078 if (src.hasPatient()) { 079 if (src.hasPatient()) 080 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 081 } 082 if (src.hasRelationship()) { 083 if (src.hasRelationship()) 084 tgt.addRelationship(CodeableConcept30_40.convertCodeableConcept(src.getRelationship())); 085 } 086 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) { 087 tgt.addName(HumanName30_40.convertHumanName(t)); 088 } 089 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) { 090 tgt.addTelecom(ContactPoint30_40.convertContactPoint(t)); 091 } 092 if (src.hasGender()) { 093 tgt.setGenderElement(Enumerations30_40.convertAdministrativeGender(src.getGenderElement())); 094 } 095 if (src.hasBirthDate()) { 096 tgt.setBirthDate(src.getBirthDate()); 097 } 098 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) { 099 tgt.addAddress(Address30_40.convertAddress(t)); 100 } 101 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPhoto()) { 102 tgt.addPhoto(Attachment30_40.convertAttachment(t)); 103 } 104 if (src.hasPeriod()) { 105 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 106 } 107 return tgt; 108 } 109}