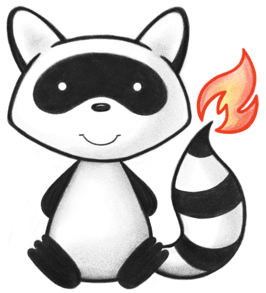
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Schedule30_40 { 013 014 public static org.hl7.fhir.dstu3.model.Schedule convertSchedule(org.hl7.fhir.r4.model.Schedule src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.dstu3.model.Schedule tgt = new org.hl7.fhir.dstu3.model.Schedule(); 018 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 021 if (src.hasActive()) 022 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 023 if (src.hasServiceCategory()) 024 tgt.setServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategoryFirstRep())); 025 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceType()) 026 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 027 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) 028 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 029 for (org.hl7.fhir.r4.model.Reference t : src.getActor()) tgt.addActor(Reference30_40.convertReference(t)); 030 if (src.hasPlanningHorizon()) 031 tgt.setPlanningHorizon(Period30_40.convertPeriod(src.getPlanningHorizon())); 032 if (src.hasComment()) 033 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 034 return tgt; 035 } 036 037 public static org.hl7.fhir.r4.model.Schedule convertSchedule(org.hl7.fhir.dstu3.model.Schedule src) throws FHIRException { 038 if (src == null) 039 return null; 040 org.hl7.fhir.r4.model.Schedule tgt = new org.hl7.fhir.r4.model.Schedule(); 041 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 042 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 043 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 044 if (src.hasActive()) 045 tgt.setActiveElement(Boolean30_40.convertBoolean(src.getActiveElement())); 046 if (src.hasServiceCategory()) 047 tgt.addServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategory())); 048 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceType()) 049 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 050 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 051 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 052 for (org.hl7.fhir.dstu3.model.Reference t : src.getActor()) tgt.addActor(Reference30_40.convertReference(t)); 053 if (src.hasPlanningHorizon()) 054 tgt.setPlanningHorizon(Period30_40.convertPeriod(src.getPlanningHorizon())); 055 if (src.hasComment()) 056 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 057 return tgt; 058 } 059}