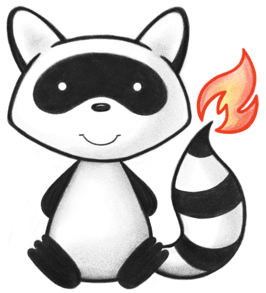
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 016import org.hl7.fhir.exceptions.FHIRException; 017 018public class SearchParameter30_40 { 019 020 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> convertSearchComparator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator> src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.SearchComparatorEnumFactory()); 024 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 025 switch (src.getValue()) { 026 case EQ: 027 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.EQ); 028 break; 029 case NE: 030 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.NE); 031 break; 032 case GT: 033 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.GT); 034 break; 035 case LT: 036 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.LT); 037 break; 038 case GE: 039 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.GE); 040 break; 041 case LE: 042 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.LE); 043 break; 044 case SA: 045 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.SA); 046 break; 047 case EB: 048 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.EB); 049 break; 050 case AP: 051 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.AP); 052 break; 053 default: 054 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.NULL); 055 break; 056 } 057 return tgt; 058 } 059 060 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator> convertSearchComparator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> src) throws FHIRException { 061 if (src == null || src.isEmpty()) 062 return null; 063 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.SearchParameter.SearchComparatorEnumFactory()); 064 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 065 switch (src.getValue()) { 066 case EQ: 067 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.EQ); 068 break; 069 case NE: 070 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.NE); 071 break; 072 case GT: 073 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.GT); 074 break; 075 case LT: 076 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.LT); 077 break; 078 case GE: 079 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.GE); 080 break; 081 case LE: 082 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.LE); 083 break; 084 case SA: 085 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.SA); 086 break; 087 case EB: 088 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.EB); 089 break; 090 case AP: 091 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.AP); 092 break; 093 default: 094 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator.NULL); 095 break; 096 } 097 return tgt; 098 } 099 100 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCodeEnumFactory()); 104 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 105 switch (src.getValue()) { 106 case MISSING: 107 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.MISSING); 108 break; 109 case EXACT: 110 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.EXACT); 111 break; 112 case CONTAINS: 113 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.CONTAINS); 114 break; 115 case NOT: 116 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.NOT); 117 break; 118 case TEXT: 119 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.TEXT); 120 break; 121 case IN: 122 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.IN); 123 break; 124 case NOTIN: 125 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.NOTIN); 126 break; 127 case BELOW: 128 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.BELOW); 129 break; 130 case ABOVE: 131 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.ABOVE); 132 break; 133 case TYPE: 134 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.TYPE); 135 break; 136 default: 137 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode.NULL); 138 break; 139 } 140 return tgt; 141 } 142 143 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode> src) throws FHIRException { 144 if (src == null || src.isEmpty()) 145 return null; 146 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.SearchModifierCodeEnumFactory()); 147 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 148 switch (src.getValue()) { 149 case MISSING: 150 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.MISSING); 151 break; 152 case EXACT: 153 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.EXACT); 154 break; 155 case CONTAINS: 156 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.CONTAINS); 157 break; 158 case NOT: 159 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NOT); 160 break; 161 case TEXT: 162 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.TEXT); 163 break; 164 case IN: 165 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.IN); 166 break; 167 case NOTIN: 168 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NOTIN); 169 break; 170 case BELOW: 171 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.BELOW); 172 break; 173 case ABOVE: 174 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.ABOVE); 175 break; 176 case TYPE: 177 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.TYPE); 178 break; 179 default: 180 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NULL); 181 break; 182 } 183 return tgt; 184 } 185 186 public static org.hl7.fhir.dstu3.model.SearchParameter convertSearchParameter(org.hl7.fhir.r4.model.SearchParameter src) throws FHIRException { 187 if (src == null) 188 return null; 189 org.hl7.fhir.dstu3.model.SearchParameter tgt = new org.hl7.fhir.dstu3.model.SearchParameter(); 190 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 191 if (src.hasUrl()) 192 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 193 if (src.hasVersion()) 194 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 195 if (src.hasName()) 196 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 197 if (src.hasStatus()) 198 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 199 if (src.hasExperimental()) 200 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 201 if (src.hasDateElement()) 202 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 203 if (src.hasPublisher()) 204 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 205 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 206 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 207 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 208 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 209 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 210 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 211 if (src.hasPurpose()) 212 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 213 if (src.hasCode()) 214 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 215 for (org.hl7.fhir.r4.model.CodeType t : src.getBase()) tgt.addBase(t.getValue()); 216 if (src.hasType()) 217 tgt.setTypeElement(Enumerations30_40.convertSearchParamType(src.getTypeElement())); 218 if (src.hasDerivedFrom()) 219 tgt.setDerivedFrom(src.getDerivedFrom()); 220 if (src.hasDescription()) 221 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 222 if (src.hasExpression()) 223 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 224 if (src.hasXpath()) 225 tgt.setXpathElement(String30_40.convertString(src.getXpathElement())); 226 if (src.hasXpathUsage()) 227 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 228 for (org.hl7.fhir.r4.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 229 tgt.setComparator(src.getComparator().stream() 230 .map(SearchParameter30_40::convertSearchComparator) 231 .collect(Collectors.toList())); 232 tgt.setModifier(src.getModifier().stream() 233 .map(SearchParameter30_40::convertSearchModifierCode) 234 .collect(Collectors.toList())); 235 for (org.hl7.fhir.r4.model.StringType t : src.getChain()) tgt.addChain(t.getValue()); 236 for (org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 237 tgt.addComponent(convertSearchParameterComponentComponent(t)); 238 return tgt; 239 } 240 241 public static org.hl7.fhir.r4.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu3.model.SearchParameter src) throws FHIRException { 242 if (src == null) 243 return null; 244 org.hl7.fhir.r4.model.SearchParameter tgt = new org.hl7.fhir.r4.model.SearchParameter(); 245 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 246 if (src.hasUrl()) 247 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 248 if (src.hasVersion()) 249 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 250 if (src.hasName()) 251 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 252 if (src.hasStatus()) 253 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 254 if (src.hasExperimental()) 255 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 256 if (src.hasDateElement()) 257 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 258 if (src.hasPublisher()) 259 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 260 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 261 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 262 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 263 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 264 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 265 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 266 if (src.hasPurpose()) 267 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 268 if (src.hasCode()) 269 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 270 for (org.hl7.fhir.dstu3.model.CodeType t : src.getBase()) tgt.addBase(t.getValue()); 271 if (src.hasType()) 272 tgt.setTypeElement(Enumerations30_40.convertSearchParamType(src.getTypeElement())); 273 if (src.hasDerivedFrom()) 274 tgt.setDerivedFrom(src.getDerivedFrom()); 275 if (src.hasDescription()) 276 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 277 if (src.hasExpression()) 278 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 279 if (src.hasXpath()) 280 tgt.setXpathElement(String30_40.convertString(src.getXpathElement())); 281 if (src.hasXpathUsage()) 282 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 283 for (org.hl7.fhir.dstu3.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 284 tgt.setComparator(src.getComparator().stream() 285 .map(SearchParameter30_40::convertSearchComparator) 286 .collect(Collectors.toList())); 287 tgt.setModifier(src.getModifier().stream() 288 .map(SearchParameter30_40::convertSearchModifierCode) 289 .collect(Collectors.toList())); 290 for (org.hl7.fhir.dstu3.model.StringType t : src.getChain()) tgt.addChain(t.getValue()); 291 for (org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 292 tgt.addComponent(convertSearchParameterComponentComponent(t)); 293 return tgt; 294 } 295 296 public static org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 297 if (src == null) 298 return null; 299 org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent(); 300 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 301 if (src.hasDefinition()) 302 tgt.setDefinition(Reference30_40.convertCanonicalToReference(src.getDefinitionElement())); 303 if (src.hasExpression()) 304 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 305 return tgt; 306 } 307 308 public static org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 309 if (src == null) 310 return null; 311 org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent(); 312 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 313 if (src.hasDefinition()) 314 tgt.setDefinitionElement(Reference30_40.convertReferenceToCanonical(src.getDefinition())); 315 if (src.hasExpression()) 316 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 317 return tgt; 318 } 319 320 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType> src) throws FHIRException { 321 if (src == null || src.isEmpty()) 322 return null; 323 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.XPathUsageTypeEnumFactory()); 324 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 325 switch (src.getValue()) { 326 case NORMAL: 327 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NORMAL); 328 break; 329 case PHONETIC: 330 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.PHONETIC); 331 break; 332 case NEARBY: 333 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NEARBY); 334 break; 335 case DISTANCE: 336 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.DISTANCE); 337 break; 338 case OTHER: 339 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.OTHER); 340 break; 341 default: 342 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NULL); 343 break; 344 } 345 return tgt; 346 } 347 348 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> src) throws FHIRException { 349 if (src == null || src.isEmpty()) 350 return null; 351 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageTypeEnumFactory()); 352 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 353 switch (src.getValue()) { 354 case NORMAL: 355 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.NORMAL); 356 break; 357 case PHONETIC: 358 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.PHONETIC); 359 break; 360 case NEARBY: 361 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.NEARBY); 362 break; 363 case DISTANCE: 364 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.DISTANCE); 365 break; 366 case OTHER: 367 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.OTHER); 368 break; 369 default: 370 tgt.setValue(org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType.NULL); 371 break; 372 } 373 return tgt; 374 } 375}