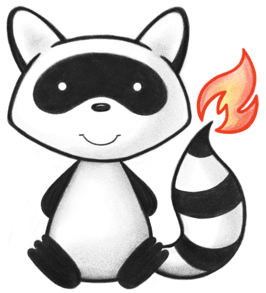
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Quantity30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.dstu3.model.Sequence; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4.model.MolecularSequence; 016 017public class Sequence30_40 { 018 019 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.QualityType> convertQualityType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.QualityType> src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 Enumeration<Sequence.QualityType> tgt = new Enumeration<>(new Sequence.QualityTypeEnumFactory()); 023 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 024 if (src.getValue() == null) { 025 tgt.setValue(null); 026 } else { 027 switch (src.getValue()) { 028 case INDEL: 029 tgt.setValue(Sequence.QualityType.INDEL); 030 break; 031 case SNP: 032 tgt.setValue(Sequence.QualityType.SNP); 033 break; 034 case UNKNOWN: 035 tgt.setValue(Sequence.QualityType.UNKNOWN); 036 break; 037 default: 038 tgt.setValue(Sequence.QualityType.NULL); 039 break; 040 } 041 } 042 return tgt; 043 } 044 045 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.QualityType> convertQualityType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.QualityType> src) throws FHIRException { 046 if (src == null || src.isEmpty()) 047 return null; 048 org.hl7.fhir.r4.model.Enumeration<MolecularSequence.QualityType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new MolecularSequence.QualityTypeEnumFactory()); 049 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 050 if (src.getValue() == null) { 051 tgt.setValue(null); 052 } else { 053 switch (src.getValue()) { 054 case INDEL: 055 tgt.setValue(MolecularSequence.QualityType.INDEL); 056 break; 057 case SNP: 058 tgt.setValue(MolecularSequence.QualityType.SNP); 059 break; 060 case UNKNOWN: 061 tgt.setValue(MolecularSequence.QualityType.UNKNOWN); 062 break; 063 default: 064 tgt.setValue(MolecularSequence.QualityType.NULL); 065 break; 066 } 067 } 068 return tgt; 069 } 070 071 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.RepositoryType> convertRepositoryType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.RepositoryType> src) throws FHIRException { 072 if (src == null || src.isEmpty()) 073 return null; 074 Enumeration<Sequence.RepositoryType> tgt = new Enumeration<>(new Sequence.RepositoryTypeEnumFactory()); 075 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 076 if (src.getValue() == null) { 077 tgt.setValue(null); 078 } else { 079 switch (src.getValue()) { 080 case DIRECTLINK: 081 tgt.setValue(Sequence.RepositoryType.DIRECTLINK); 082 break; 083 case OPENAPI: 084 tgt.setValue(Sequence.RepositoryType.OPENAPI); 085 break; 086 case LOGIN: 087 tgt.setValue(Sequence.RepositoryType.LOGIN); 088 break; 089 case OAUTH: 090 tgt.setValue(Sequence.RepositoryType.OAUTH); 091 break; 092 case OTHER: 093 tgt.setValue(Sequence.RepositoryType.OTHER); 094 break; 095 default: 096 tgt.setValue(Sequence.RepositoryType.NULL); 097 break; 098 } 099 } 100 return tgt; 101 } 102 103 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.RepositoryType> convertRepositoryType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.RepositoryType> src) throws FHIRException { 104 if (src == null || src.isEmpty()) 105 return null; 106 org.hl7.fhir.r4.model.Enumeration<MolecularSequence.RepositoryType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new MolecularSequence.RepositoryTypeEnumFactory()); 107 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 108 if (src.getValue() == null) { 109 tgt.setValue(null); 110 } else { 111 switch (src.getValue()) { 112 case DIRECTLINK: 113 tgt.setValue(MolecularSequence.RepositoryType.DIRECTLINK); 114 break; 115 case OPENAPI: 116 tgt.setValue(MolecularSequence.RepositoryType.OPENAPI); 117 break; 118 case LOGIN: 119 tgt.setValue(MolecularSequence.RepositoryType.LOGIN); 120 break; 121 case OAUTH: 122 tgt.setValue(MolecularSequence.RepositoryType.OAUTH); 123 break; 124 case OTHER: 125 tgt.setValue(MolecularSequence.RepositoryType.OTHER); 126 break; 127 default: 128 tgt.setValue(MolecularSequence.RepositoryType.NULL); 129 break; 130 } 131 } 132 return tgt; 133 } 134 135 public static org.hl7.fhir.dstu3.model.Sequence convertSequence(org.hl7.fhir.r4.model.MolecularSequence src) throws FHIRException { 136 if (src == null) 137 return null; 138 org.hl7.fhir.dstu3.model.Sequence tgt = new org.hl7.fhir.dstu3.model.Sequence(); 139 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 140 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 141 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 142 if (src.hasType()) 143 tgt.setTypeElement(convertSequenceType(src.getTypeElement())); 144 if (src.hasCoordinateSystem()) 145 tgt.setCoordinateSystemElement(Integer30_40.convertInteger(src.getCoordinateSystemElement())); 146 if (src.hasPatient()) 147 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 148 if (src.hasSpecimen()) 149 tgt.setSpecimen(Reference30_40.convertReference(src.getSpecimen())); 150 if (src.hasDevice()) 151 tgt.setDevice(Reference30_40.convertReference(src.getDevice())); 152 if (src.hasPerformer()) 153 tgt.setPerformer(Reference30_40.convertReference(src.getPerformer())); 154 if (src.hasQuantity()) 155 tgt.setQuantity(Quantity30_40.convertQuantity(src.getQuantity())); 156 if (src.hasReferenceSeq()) 157 tgt.setReferenceSeq(convertSequenceReferenceSeqComponent(src.getReferenceSeq())); 158 for (org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceVariantComponent t : src.getVariant()) 159 tgt.addVariant(convertSequenceVariantComponent(t)); 160 if (src.hasObservedSeq()) 161 tgt.setObservedSeqElement(String30_40.convertString(src.getObservedSeqElement())); 162 for (org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceQualityComponent t : src.getQuality()) 163 tgt.addQuality(convertSequenceQualityComponent(t)); 164 if (src.hasReadCoverage()) 165 tgt.setReadCoverageElement(Integer30_40.convertInteger(src.getReadCoverageElement())); 166 for (org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceRepositoryComponent t : src.getRepository()) 167 tgt.addRepository(convertSequenceRepositoryComponent(t)); 168 for (org.hl7.fhir.r4.model.Reference t : src.getPointer()) tgt.addPointer(Reference30_40.convertReference(t)); 169 return tgt; 170 } 171 172 public static org.hl7.fhir.r4.model.MolecularSequence convertSequence(org.hl7.fhir.dstu3.model.Sequence src) throws FHIRException { 173 if (src == null) 174 return null; 175 org.hl7.fhir.r4.model.MolecularSequence tgt = new org.hl7.fhir.r4.model.MolecularSequence(); 176 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 177 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 178 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 179 if (src.hasType()) 180 tgt.setTypeElement(convertSequenceType(src.getTypeElement())); 181 if (src.hasCoordinateSystem()) 182 tgt.setCoordinateSystemElement(Integer30_40.convertInteger(src.getCoordinateSystemElement())); 183 if (src.hasPatient()) 184 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 185 if (src.hasSpecimen()) 186 tgt.setSpecimen(Reference30_40.convertReference(src.getSpecimen())); 187 if (src.hasDevice()) 188 tgt.setDevice(Reference30_40.convertReference(src.getDevice())); 189 if (src.hasPerformer()) 190 tgt.setPerformer(Reference30_40.convertReference(src.getPerformer())); 191 if (src.hasQuantity()) 192 tgt.setQuantity(Quantity30_40.convertQuantity(src.getQuantity())); 193 if (src.hasReferenceSeq()) 194 tgt.setReferenceSeq(convertSequenceReferenceSeqComponent(src.getReferenceSeq())); 195 for (org.hl7.fhir.dstu3.model.Sequence.SequenceVariantComponent t : src.getVariant()) 196 tgt.addVariant(convertSequenceVariantComponent(t)); 197 if (src.hasObservedSeq()) 198 tgt.setObservedSeqElement(String30_40.convertString(src.getObservedSeqElement())); 199 for (org.hl7.fhir.dstu3.model.Sequence.SequenceQualityComponent t : src.getQuality()) 200 tgt.addQuality(convertSequenceQualityComponent(t)); 201 if (src.hasReadCoverage()) 202 tgt.setReadCoverageElement(Integer30_40.convertInteger(src.getReadCoverageElement())); 203 for (org.hl7.fhir.dstu3.model.Sequence.SequenceRepositoryComponent t : src.getRepository()) 204 tgt.addRepository(convertSequenceRepositoryComponent(t)); 205 for (org.hl7.fhir.dstu3.model.Reference t : src.getPointer()) tgt.addPointer(Reference30_40.convertReference(t)); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceQualityComponent convertSequenceQualityComponent(org.hl7.fhir.dstu3.model.Sequence.SequenceQualityComponent src) throws FHIRException { 210 if (src == null) 211 return null; 212 org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceQualityComponent tgt = new org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceQualityComponent(); 213 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 214 if (src.hasType()) 215 tgt.setTypeElement(convertQualityType(src.getTypeElement())); 216 if (src.hasStandardSequence()) 217 tgt.setStandardSequence(CodeableConcept30_40.convertCodeableConcept(src.getStandardSequence())); 218 if (src.hasStart()) 219 tgt.setStartElement(Integer30_40.convertInteger(src.getStartElement())); 220 if (src.hasEnd()) 221 tgt.setEndElement(Integer30_40.convertInteger(src.getEndElement())); 222 if (src.hasScore()) 223 tgt.setScore(Quantity30_40.convertQuantity(src.getScore())); 224 if (src.hasMethod()) 225 tgt.setMethod(CodeableConcept30_40.convertCodeableConcept(src.getMethod())); 226 if (src.hasTruthTP()) 227 tgt.setTruthTPElement(Decimal30_40.convertDecimal(src.getTruthTPElement())); 228 if (src.hasQueryTP()) 229 tgt.setQueryTPElement(Decimal30_40.convertDecimal(src.getQueryTPElement())); 230 if (src.hasTruthFN()) 231 tgt.setTruthFNElement(Decimal30_40.convertDecimal(src.getTruthFNElement())); 232 if (src.hasQueryFP()) 233 tgt.setQueryFPElement(Decimal30_40.convertDecimal(src.getQueryFPElement())); 234 if (src.hasGtFP()) 235 tgt.setGtFPElement(Decimal30_40.convertDecimal(src.getGtFPElement())); 236 if (src.hasPrecision()) 237 tgt.setPrecisionElement(Decimal30_40.convertDecimal(src.getPrecisionElement())); 238 if (src.hasRecall()) 239 tgt.setRecallElement(Decimal30_40.convertDecimal(src.getRecallElement())); 240 if (src.hasFScore()) 241 tgt.setFScoreElement(Decimal30_40.convertDecimal(src.getFScoreElement())); 242 return tgt; 243 } 244 245 public static org.hl7.fhir.dstu3.model.Sequence.SequenceQualityComponent convertSequenceQualityComponent(org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceQualityComponent src) throws FHIRException { 246 if (src == null) 247 return null; 248 org.hl7.fhir.dstu3.model.Sequence.SequenceQualityComponent tgt = new org.hl7.fhir.dstu3.model.Sequence.SequenceQualityComponent(); 249 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 250 if (src.hasType()) 251 tgt.setTypeElement(convertQualityType(src.getTypeElement())); 252 if (src.hasStandardSequence()) 253 tgt.setStandardSequence(CodeableConcept30_40.convertCodeableConcept(src.getStandardSequence())); 254 if (src.hasStart()) 255 tgt.setStartElement(Integer30_40.convertInteger(src.getStartElement())); 256 if (src.hasEnd()) 257 tgt.setEndElement(Integer30_40.convertInteger(src.getEndElement())); 258 if (src.hasScore()) 259 tgt.setScore(Quantity30_40.convertQuantity(src.getScore())); 260 if (src.hasMethod()) 261 tgt.setMethod(CodeableConcept30_40.convertCodeableConcept(src.getMethod())); 262 if (src.hasTruthTP()) 263 tgt.setTruthTPElement(Decimal30_40.convertDecimal(src.getTruthTPElement())); 264 if (src.hasQueryTP()) 265 tgt.setQueryTPElement(Decimal30_40.convertDecimal(src.getQueryTPElement())); 266 if (src.hasTruthFN()) 267 tgt.setTruthFNElement(Decimal30_40.convertDecimal(src.getTruthFNElement())); 268 if (src.hasQueryFP()) 269 tgt.setQueryFPElement(Decimal30_40.convertDecimal(src.getQueryFPElement())); 270 if (src.hasGtFP()) 271 tgt.setGtFPElement(Decimal30_40.convertDecimal(src.getGtFPElement())); 272 if (src.hasPrecision()) 273 tgt.setPrecisionElement(Decimal30_40.convertDecimal(src.getPrecisionElement())); 274 if (src.hasRecall()) 275 tgt.setRecallElement(Decimal30_40.convertDecimal(src.getRecallElement())); 276 if (src.hasFScore()) 277 tgt.setFScoreElement(Decimal30_40.convertDecimal(src.getFScoreElement())); 278 return tgt; 279 } 280 281 public static org.hl7.fhir.dstu3.model.Sequence.SequenceReferenceSeqComponent convertSequenceReferenceSeqComponent(org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceReferenceSeqComponent src) throws FHIRException { 282 if (src == null) 283 return null; 284 org.hl7.fhir.dstu3.model.Sequence.SequenceReferenceSeqComponent tgt = new org.hl7.fhir.dstu3.model.Sequence.SequenceReferenceSeqComponent(); 285 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 286 if (src.hasChromosome()) 287 tgt.setChromosome(CodeableConcept30_40.convertCodeableConcept(src.getChromosome())); 288 if (src.hasGenomeBuild()) 289 tgt.setGenomeBuildElement(String30_40.convertString(src.getGenomeBuildElement())); 290 if (src.hasReferenceSeqId()) 291 tgt.setReferenceSeqId(CodeableConcept30_40.convertCodeableConcept(src.getReferenceSeqId())); 292 if (src.hasReferenceSeqPointer()) 293 tgt.setReferenceSeqPointer(Reference30_40.convertReference(src.getReferenceSeqPointer())); 294 if (src.hasReferenceSeqString()) 295 tgt.setReferenceSeqStringElement(String30_40.convertString(src.getReferenceSeqStringElement())); 296 if (src.hasWindowStart()) 297 tgt.setWindowStartElement(Integer30_40.convertInteger(src.getWindowStartElement())); 298 if (src.hasWindowEnd()) 299 tgt.setWindowEndElement(Integer30_40.convertInteger(src.getWindowEndElement())); 300 return tgt; 301 } 302 303 public static org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceReferenceSeqComponent convertSequenceReferenceSeqComponent(org.hl7.fhir.dstu3.model.Sequence.SequenceReferenceSeqComponent src) throws FHIRException { 304 if (src == null) 305 return null; 306 org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceReferenceSeqComponent tgt = new org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceReferenceSeqComponent(); 307 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 308 if (src.hasChromosome()) 309 tgt.setChromosome(CodeableConcept30_40.convertCodeableConcept(src.getChromosome())); 310 if (src.hasGenomeBuild()) 311 tgt.setGenomeBuildElement(String30_40.convertString(src.getGenomeBuildElement())); 312 if (src.hasReferenceSeqId()) 313 tgt.setReferenceSeqId(CodeableConcept30_40.convertCodeableConcept(src.getReferenceSeqId())); 314 if (src.hasReferenceSeqPointer()) 315 tgt.setReferenceSeqPointer(Reference30_40.convertReference(src.getReferenceSeqPointer())); 316 if (src.hasReferenceSeqString()) 317 tgt.setReferenceSeqStringElement(String30_40.convertString(src.getReferenceSeqStringElement())); 318 if (src.hasWindowStart()) 319 tgt.setWindowStartElement(Integer30_40.convertInteger(src.getWindowStartElement())); 320 if (src.hasWindowEnd()) 321 tgt.setWindowEndElement(Integer30_40.convertInteger(src.getWindowEndElement())); 322 return tgt; 323 } 324 325 public static org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceRepositoryComponent convertSequenceRepositoryComponent(org.hl7.fhir.dstu3.model.Sequence.SequenceRepositoryComponent src) throws FHIRException { 326 if (src == null) 327 return null; 328 org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceRepositoryComponent tgt = new org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceRepositoryComponent(); 329 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 330 if (src.hasType()) 331 tgt.setTypeElement(convertRepositoryType(src.getTypeElement())); 332 if (src.hasUrl()) 333 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 334 if (src.hasName()) 335 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 336 if (src.hasDatasetId()) 337 tgt.setDatasetIdElement(String30_40.convertString(src.getDatasetIdElement())); 338 if (src.hasVariantsetId()) 339 tgt.setVariantsetIdElement(String30_40.convertString(src.getVariantsetIdElement())); 340 if (src.hasReadsetId()) 341 tgt.setReadsetIdElement(String30_40.convertString(src.getReadsetIdElement())); 342 return tgt; 343 } 344 345 public static org.hl7.fhir.dstu3.model.Sequence.SequenceRepositoryComponent convertSequenceRepositoryComponent(org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceRepositoryComponent src) throws FHIRException { 346 if (src == null) 347 return null; 348 org.hl7.fhir.dstu3.model.Sequence.SequenceRepositoryComponent tgt = new org.hl7.fhir.dstu3.model.Sequence.SequenceRepositoryComponent(); 349 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 350 if (src.hasType()) 351 tgt.setTypeElement(convertRepositoryType(src.getTypeElement())); 352 if (src.hasUrl()) 353 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 354 if (src.hasName()) 355 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 356 if (src.hasDatasetId()) 357 tgt.setDatasetIdElement(String30_40.convertString(src.getDatasetIdElement())); 358 if (src.hasVariantsetId()) 359 tgt.setVariantsetIdElement(String30_40.convertString(src.getVariantsetIdElement())); 360 if (src.hasReadsetId()) 361 tgt.setReadsetIdElement(String30_40.convertString(src.getReadsetIdElement())); 362 return tgt; 363 } 364 365 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.SequenceType> convertSequenceType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.SequenceType> src) throws FHIRException { 366 if (src == null || src.isEmpty()) 367 return null; 368 Enumeration<Sequence.SequenceType> tgt = new Enumeration<>(new Sequence.SequenceTypeEnumFactory()); 369 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 370 if (src.getValue() == null) { 371 tgt.setValue(null); 372 } else { 373 switch (src.getValue()) { 374 case AA: 375 tgt.setValue(Sequence.SequenceType.AA); 376 break; 377 case DNA: 378 tgt.setValue(Sequence.SequenceType.DNA); 379 break; 380 case RNA: 381 tgt.setValue(Sequence.SequenceType.RNA); 382 break; 383 default: 384 tgt.setValue(Sequence.SequenceType.NULL); 385 break; 386 } 387 } 388 return tgt; 389 } 390 391 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MolecularSequence.SequenceType> convertSequenceType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Sequence.SequenceType> src) throws FHIRException { 392 if (src == null || src.isEmpty()) 393 return null; 394 org.hl7.fhir.r4.model.Enumeration<MolecularSequence.SequenceType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new MolecularSequence.SequenceTypeEnumFactory()); 395 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 396 if (src.getValue() == null) { 397 tgt.setValue(null); 398 } else { 399 switch (src.getValue()) { 400 case AA: 401 tgt.setValue(MolecularSequence.SequenceType.AA); 402 break; 403 case DNA: 404 tgt.setValue(MolecularSequence.SequenceType.DNA); 405 break; 406 case RNA: 407 tgt.setValue(MolecularSequence.SequenceType.RNA); 408 break; 409 default: 410 tgt.setValue(MolecularSequence.SequenceType.NULL); 411 break; 412 } 413 } 414 return tgt; 415 } 416 417 public static org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceVariantComponent convertSequenceVariantComponent(org.hl7.fhir.dstu3.model.Sequence.SequenceVariantComponent src) throws FHIRException { 418 if (src == null) 419 return null; 420 org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceVariantComponent tgt = new org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceVariantComponent(); 421 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 422 if (src.hasStart()) 423 tgt.setStartElement(Integer30_40.convertInteger(src.getStartElement())); 424 if (src.hasEnd()) 425 tgt.setEndElement(Integer30_40.convertInteger(src.getEndElement())); 426 if (src.hasObservedAllele()) 427 tgt.setObservedAlleleElement(String30_40.convertString(src.getObservedAlleleElement())); 428 if (src.hasReferenceAllele()) 429 tgt.setReferenceAlleleElement(String30_40.convertString(src.getReferenceAlleleElement())); 430 if (src.hasCigar()) 431 tgt.setCigarElement(String30_40.convertString(src.getCigarElement())); 432 if (src.hasVariantPointer()) 433 tgt.setVariantPointer(Reference30_40.convertReference(src.getVariantPointer())); 434 return tgt; 435 } 436 437 public static org.hl7.fhir.dstu3.model.Sequence.SequenceVariantComponent convertSequenceVariantComponent(org.hl7.fhir.r4.model.MolecularSequence.MolecularSequenceVariantComponent src) throws FHIRException { 438 if (src == null) 439 return null; 440 org.hl7.fhir.dstu3.model.Sequence.SequenceVariantComponent tgt = new org.hl7.fhir.dstu3.model.Sequence.SequenceVariantComponent(); 441 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 442 if (src.hasStart()) 443 tgt.setStartElement(Integer30_40.convertInteger(src.getStartElement())); 444 if (src.hasEnd()) 445 tgt.setEndElement(Integer30_40.convertInteger(src.getEndElement())); 446 if (src.hasObservedAllele()) 447 tgt.setObservedAlleleElement(String30_40.convertString(src.getObservedAlleleElement())); 448 if (src.hasReferenceAllele()) 449 tgt.setReferenceAlleleElement(String30_40.convertString(src.getReferenceAlleleElement())); 450 if (src.hasCigar()) 451 tgt.setCigarElement(String30_40.convertString(src.getCigarElement())); 452 if (src.hasVariantPointer()) 453 tgt.setVariantPointer(Reference30_40.convertReference(src.getVariantPointer())); 454 return tgt; 455 } 456}