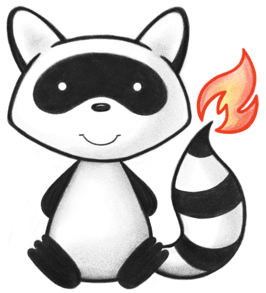
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Instant30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.Enumeration; 012import org.hl7.fhir.r4.model.Slot; 013 014public class Slot30_40 { 015 016 public static org.hl7.fhir.r4.model.Slot convertSlot(org.hl7.fhir.dstu3.model.Slot src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r4.model.Slot tgt = new org.hl7.fhir.r4.model.Slot(); 020 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 023 if (src.hasServiceCategory()) 024 tgt.addServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategory())); 025 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceType()) 026 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 027 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 028 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 029 if (src.hasAppointmentType()) 030 tgt.setAppointmentType(CodeableConcept30_40.convertCodeableConcept(src.getAppointmentType())); 031 if (src.hasSchedule()) 032 tgt.setSchedule(Reference30_40.convertReference(src.getSchedule())); 033 if (src.hasStatus()) 034 tgt.setStatusElement(convertSlotStatus(src.getStatusElement())); 035 if (src.hasStart()) 036 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 037 if (src.hasEnd()) 038 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 039 if (src.hasOverbooked()) 040 tgt.setOverbookedElement(Boolean30_40.convertBoolean(src.getOverbookedElement())); 041 if (src.hasComment()) 042 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu3.model.Slot convertSlot(org.hl7.fhir.r4.model.Slot src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.dstu3.model.Slot tgt = new org.hl7.fhir.dstu3.model.Slot(); 050 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 053 if (src.hasServiceCategory()) 054 tgt.setServiceCategory(CodeableConcept30_40.convertCodeableConcept(src.getServiceCategoryFirstRep())); 055 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceType()) 056 tgt.addServiceType(CodeableConcept30_40.convertCodeableConcept(t)); 057 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) 058 tgt.addSpecialty(CodeableConcept30_40.convertCodeableConcept(t)); 059 if (src.hasAppointmentType()) 060 tgt.setAppointmentType(CodeableConcept30_40.convertCodeableConcept(src.getAppointmentType())); 061 if (src.hasSchedule()) 062 tgt.setSchedule(Reference30_40.convertReference(src.getSchedule())); 063 if (src.hasStatus()) 064 tgt.setStatusElement(convertSlotStatus(src.getStatusElement())); 065 if (src.hasStart()) 066 tgt.setStartElement(Instant30_40.convertInstant(src.getStartElement())); 067 if (src.hasEnd()) 068 tgt.setEndElement(Instant30_40.convertInstant(src.getEndElement())); 069 if (src.hasOverbooked()) 070 tgt.setOverbookedElement(Boolean30_40.convertBoolean(src.getOverbookedElement())); 071 if (src.hasComment()) 072 tgt.setCommentElement(String30_40.convertString(src.getCommentElement())); 073 return tgt; 074 } 075 076 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> src) throws FHIRException { 077 if (src == null || src.isEmpty()) 078 return null; 079 Enumeration<Slot.SlotStatus> tgt = new Enumeration<>(new Slot.SlotStatusEnumFactory()); 080 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 081 if (src.getValue() == null) { 082 tgt.setValue(null); 083 } else { 084 switch (src.getValue()) { 085 case BUSY: 086 tgt.setValue(Slot.SlotStatus.BUSY); 087 break; 088 case FREE: 089 tgt.setValue(Slot.SlotStatus.FREE); 090 break; 091 case BUSYUNAVAILABLE: 092 tgt.setValue(Slot.SlotStatus.BUSYUNAVAILABLE); 093 break; 094 case BUSYTENTATIVE: 095 tgt.setValue(Slot.SlotStatus.BUSYTENTATIVE); 096 break; 097 case ENTEREDINERROR: 098 tgt.setValue(Slot.SlotStatus.ENTEREDINERROR); 099 break; 100 default: 101 tgt.setValue(Slot.SlotStatus.NULL); 102 break; 103 } 104 } 105 return tgt; 106 } 107 108 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Slot.SlotStatus> src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Slot.SlotStatusEnumFactory()); 112 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 113 if (src.getValue() == null) { 114 tgt.setValue(null); 115 } else { 116 switch (src.getValue()) { 117 case BUSY: 118 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSY); 119 break; 120 case FREE: 121 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.FREE); 122 break; 123 case BUSYUNAVAILABLE: 124 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSYUNAVAILABLE); 125 break; 126 case BUSYTENTATIVE: 127 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSYTENTATIVE); 128 break; 129 case ENTEREDINERROR: 130 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.ENTEREDINERROR); 131 break; 132 default: 133 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.NULL); 134 break; 135 } 136 } 137 return tgt; 138 } 139}