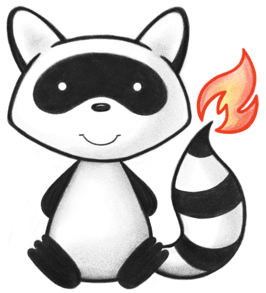
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Id30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r4.model.Enumeration; 019import org.hl7.fhir.r4.model.StructureMap; 020 021public class StructureMap30_40 { 022 023 public static org.hl7.fhir.dstu3.model.StructureMap convertStructureMap(org.hl7.fhir.r4.model.StructureMap src) throws FHIRException { 024 if (src == null) 025 return null; 026 org.hl7.fhir.dstu3.model.StructureMap tgt = new org.hl7.fhir.dstu3.model.StructureMap(); 027 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 028 if (src.hasUrl()) 029 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 030 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 031 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 032 if (src.hasVersion()) 033 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 034 if (src.hasName()) 035 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 036 if (src.hasTitle()) 037 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 038 if (src.hasStatus()) 039 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 040 if (src.hasExperimental()) 041 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 042 if (src.hasDateElement()) 043 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 044 if (src.hasPublisher()) 045 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 046 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 047 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 048 if (src.hasDescription()) 049 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 050 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 051 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 052 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 053 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 054 if (src.hasPurpose()) 055 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 056 if (src.hasCopyright()) 057 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 058 for (org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 059 tgt.addStructure(convertStructureMapStructureComponent(t)); 060 for (org.hl7.fhir.r4.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 061 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 062 tgt.addGroup(convertStructureMapGroupComponent(t)); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.r4.model.StructureMap convertStructureMap(org.hl7.fhir.dstu3.model.StructureMap src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.r4.model.StructureMap tgt = new org.hl7.fhir.r4.model.StructureMap(); 070 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 071 if (src.hasUrl()) 072 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 073 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 074 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 075 if (src.hasVersion()) 076 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 077 if (src.hasName()) 078 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 079 if (src.hasTitle()) 080 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 081 if (src.hasStatus()) 082 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 083 if (src.hasExperimental()) 084 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 085 if (src.hasDateElement()) 086 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 087 if (src.hasPublisher()) 088 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 089 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 090 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 091 if (src.hasDescription()) 092 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 093 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 094 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 095 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 096 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 097 if (src.hasPurpose()) 098 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 099 if (src.hasCopyright()) 100 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 101 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 102 tgt.addStructure(convertStructureMapStructureComponent(t)); 103 for (org.hl7.fhir.dstu3.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 104 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 105 tgt.addGroup(convertStructureMapGroupComponent(t)); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapContextType> convertStructureMapContextType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 Enumeration<StructureMap.StructureMapContextType> tgt = new Enumeration<>(new StructureMap.StructureMapContextTypeEnumFactory()); 113 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 114 if (src.getValue() == null) { 115 tgt.setValue(null); 116 } else { 117 switch (src.getValue()) { 118 case TYPE: 119 tgt.setValue(StructureMap.StructureMapContextType.TYPE); 120 break; 121 case VARIABLE: 122 tgt.setValue(StructureMap.StructureMapContextType.VARIABLE); 123 break; 124 default: 125 tgt.setValue(StructureMap.StructureMapContextType.NULL); 126 break; 127 } 128 } 129 return tgt; 130 } 131 132 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType> convertStructureMapContextType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapContextType> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextTypeEnumFactory()); 136 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case TYPE: 142 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType.TYPE); 143 break; 144 case VARIABLE: 145 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType.VARIABLE); 146 break; 147 default: 148 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType.NULL); 149 break; 150 } 151 } 152 return tgt; 153 } 154 155 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent(); 159 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 160 if (src.hasName()) 161 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 162 if (src.hasExtends()) 163 tgt.setExtendsElement(Id30_40.convertId(src.getExtendsElement())); 164 if (src.hasTypeMode()) 165 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 166 if (src.hasDocumentation()) 167 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 168 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 169 tgt.addInput(convertStructureMapGroupInputComponent(t)); 170 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 171 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 172 return tgt; 173 } 174 175 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 176 if (src == null) 177 return null; 178 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent(); 179 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 180 if (src.hasName()) 181 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 182 if (src.hasExtends()) 183 tgt.setExtendsElement(Id30_40.convertId(src.getExtendsElement())); 184 if (src.hasTypeMode()) 185 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 186 if (src.hasDocumentation()) 187 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 188 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 189 tgt.addInput(convertStructureMapGroupInputComponent(t)); 190 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 191 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 192 return tgt; 193 } 194 195 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 196 if (src == null) 197 return null; 198 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent(); 199 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 200 if (src.hasName()) 201 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 202 if (src.hasType()) 203 tgt.setTypeElement(String30_40.convertString(src.getTypeElement())); 204 if (src.hasMode()) 205 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 206 if (src.hasDocumentation()) 207 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 208 return tgt; 209 } 210 211 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 212 if (src == null) 213 return null; 214 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent(); 215 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 216 if (src.hasName()) 217 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 218 if (src.hasType()) 219 tgt.setTypeElement(String30_40.convertString(src.getTypeElement())); 220 if (src.hasMode()) 221 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 222 if (src.hasDocumentation()) 223 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 224 return tgt; 225 } 226 227 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 228 if (src == null) 229 return null; 230 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent(); 231 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 232 if (src.hasName()) 233 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 234 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 235 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 236 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 237 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 238 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 239 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 240 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 241 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 242 if (src.hasDocumentation()) 243 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 244 return tgt; 245 } 246 247 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 248 if (src == null) 249 return null; 250 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent(); 251 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 252 if (src.hasName()) 253 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 254 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 255 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 256 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 257 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 258 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 259 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 260 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 261 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 262 if (src.hasDocumentation()) 263 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 264 return tgt; 265 } 266 267 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 268 if (src == null) 269 return null; 270 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent(); 271 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 272 if (src.hasName()) 273 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 274 for (org.hl7.fhir.dstu3.model.StringType t : src.getVariable()) tgt.addVariable(t.getValue()); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 279 if (src == null) 280 return null; 281 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent(); 282 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 283 if (src.hasName()) 284 tgt.setNameElement(Id30_40.convertId(src.getNameElement())); 285 for (org.hl7.fhir.r4.model.StringType t : src.getVariable()) tgt.addVariable(t.getValue()); 286 return tgt; 287 } 288 289 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 290 if (src == null) 291 return null; 292 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent(); 293 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 294 if (src.hasContext()) 295 tgt.setContextElement(Id30_40.convertId(src.getContextElement())); 296 if (src.hasMin()) 297 tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 298 if (src.hasMax()) 299 tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 300 if (src.hasType()) 301 tgt.setTypeElement(String30_40.convertString(src.getTypeElement())); 302 if (src.hasDefaultValue()) 303 tgt.setDefaultValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDefaultValue())); 304 if (src.hasElement()) 305 tgt.setElementElement(String30_40.convertString(src.getElementElement())); 306 if (src.hasListMode()) 307 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 308 if (src.hasVariable()) 309 tgt.setVariableElement(Id30_40.convertId(src.getVariableElement())); 310 if (src.hasCondition()) 311 tgt.setConditionElement(String30_40.convertString(src.getConditionElement())); 312 if (src.hasCheck()) 313 tgt.setCheckElement(String30_40.convertString(src.getCheckElement())); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 318 if (src == null) 319 return null; 320 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent(); 321 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 322 if (src.hasContext()) 323 tgt.setContextElement(Id30_40.convertId(src.getContextElement())); 324 if (src.hasMin()) 325 tgt.setMinElement(Integer30_40.convertInteger(src.getMinElement())); 326 if (src.hasMax()) 327 tgt.setMaxElement(String30_40.convertString(src.getMaxElement())); 328 if (src.hasType()) 329 tgt.setTypeElement(String30_40.convertString(src.getTypeElement())); 330 if (src.hasDefaultValue()) 331 tgt.setDefaultValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getDefaultValue())); 332 if (src.hasElement()) 333 tgt.setElementElement(String30_40.convertString(src.getElementElement())); 334 if (src.hasListMode()) 335 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 336 if (src.hasVariable()) 337 tgt.setVariableElement(Id30_40.convertId(src.getVariableElement())); 338 if (src.hasCondition()) 339 tgt.setConditionElement(String30_40.convertString(src.getConditionElement())); 340 if (src.hasCheck()) 341 tgt.setCheckElement(String30_40.convertString(src.getCheckElement())); 342 return tgt; 343 } 344 345 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 346 if (src == null) 347 return null; 348 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent(); 349 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 350 if (src.hasContext()) 351 tgt.setContextElement(Id30_40.convertId(src.getContextElement())); 352 if (src.hasContextType()) 353 tgt.setContextTypeElement(convertStructureMapContextType(src.getContextTypeElement())); 354 if (src.hasElement()) 355 tgt.setElementElement(String30_40.convertString(src.getElementElement())); 356 if (src.hasVariable()) 357 tgt.setVariableElement(Id30_40.convertId(src.getVariableElement())); 358 tgt.setListMode(src.getListMode().stream() 359 .map(StructureMap30_40::convertStructureMapTargetListMode) 360 .collect(Collectors.toList())); 361 if (src.hasListRuleId()) 362 tgt.setListRuleIdElement(Id30_40.convertId(src.getListRuleIdElement())); 363 if (src.hasTransform()) 364 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 365 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 366 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 367 return tgt; 368 } 369 370 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 371 if (src == null) 372 return null; 373 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent(); 374 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 375 if (src.hasContext()) 376 tgt.setContextElement(Id30_40.convertId(src.getContextElement())); 377 if (src.hasContextType()) 378 tgt.setContextTypeElement(convertStructureMapContextType(src.getContextTypeElement())); 379 if (src.hasElement()) 380 tgt.setElementElement(String30_40.convertString(src.getElementElement())); 381 if (src.hasVariable()) 382 tgt.setVariableElement(Id30_40.convertId(src.getVariableElement())); 383 tgt.setListMode(src.getListMode().stream() 384 .map(StructureMap30_40::convertStructureMapTargetListMode) 385 .collect(Collectors.toList())); 386 if (src.hasListRuleId()) 387 tgt.setListRuleIdElement(Id30_40.convertId(src.getListRuleIdElement())); 388 if (src.hasTransform()) 389 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 390 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 391 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 392 return tgt; 393 } 394 395 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 396 if (src == null) 397 return null; 398 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 399 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 400 if (src.hasValue()) 401 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 402 return tgt; 403 } 404 405 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 406 if (src == null) 407 return null; 408 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 409 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 410 if (src.hasValue()) 411 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 412 return tgt; 413 } 414 415 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 416 if (src == null || src.isEmpty()) 417 return null; 418 Enumeration<StructureMap.StructureMapGroupTypeMode> tgt = new Enumeration<>(new StructureMap.StructureMapGroupTypeModeEnumFactory()); 419 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 420 if (src.getValue() == null) { 421 tgt.setValue(null); 422 } else { 423 switch (src.getValue()) { 424 case NONE: 425 tgt.setValue(StructureMap.StructureMapGroupTypeMode.NONE); 426 break; 427 case TYPES: 428 tgt.setValue(StructureMap.StructureMapGroupTypeMode.TYPES); 429 break; 430 case TYPEANDTYPES: 431 tgt.setValue(StructureMap.StructureMapGroupTypeMode.TYPEANDTYPES); 432 break; 433 default: 434 tgt.setValue(StructureMap.StructureMapGroupTypeMode.NULL); 435 break; 436 } 437 } 438 return tgt; 439 } 440 441 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 442 if (src == null || src.isEmpty()) 443 return null; 444 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeModeEnumFactory()); 445 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 446 if (src.getValue() == null) { 447 tgt.setValue(null); 448 } else { 449 switch (src.getValue()) { 450 case NONE: 451 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.NONE); 452 break; 453 case TYPES: 454 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.TYPES); 455 break; 456 case TYPEANDTYPES: 457 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.TYPEANDTYPES); 458 break; 459 default: 460 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.NULL); 461 break; 462 } 463 } 464 return tgt; 465 } 466 467 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 468 if (src == null || src.isEmpty()) 469 return null; 470 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputModeEnumFactory()); 471 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 472 if (src.getValue() == null) { 473 tgt.setValue(null); 474 } else { 475 switch (src.getValue()) { 476 case SOURCE: 477 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.SOURCE); 478 break; 479 case TARGET: 480 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.TARGET); 481 break; 482 default: 483 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.NULL); 484 break; 485 } 486 } 487 return tgt; 488 } 489 490 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 491 if (src == null || src.isEmpty()) 492 return null; 493 Enumeration<StructureMap.StructureMapInputMode> tgt = new Enumeration<>(new StructureMap.StructureMapInputModeEnumFactory()); 494 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 495 if (src.getValue() == null) { 496 tgt.setValue(null); 497 } else { 498 switch (src.getValue()) { 499 case SOURCE: 500 tgt.setValue(StructureMap.StructureMapInputMode.SOURCE); 501 break; 502 case TARGET: 503 tgt.setValue(StructureMap.StructureMapInputMode.TARGET); 504 break; 505 default: 506 tgt.setValue(StructureMap.StructureMapInputMode.NULL); 507 break; 508 } 509 } 510 return tgt; 511 } 512 513 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 514 if (src == null || src.isEmpty()) 515 return null; 516 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelModeEnumFactory()); 517 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 518 if (src.getValue() == null) { 519 tgt.setValue(null); 520 } else { 521 switch (src.getValue()) { 522 case SOURCE: 523 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.SOURCE); 524 break; 525 case QUERIED: 526 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.QUERIED); 527 break; 528 case TARGET: 529 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.TARGET); 530 break; 531 case PRODUCED: 532 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.PRODUCED); 533 break; 534 default: 535 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.NULL); 536 break; 537 } 538 } 539 return tgt; 540 } 541 542 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 543 if (src == null || src.isEmpty()) 544 return null; 545 Enumeration<StructureMap.StructureMapModelMode> tgt = new Enumeration<>(new StructureMap.StructureMapModelModeEnumFactory()); 546 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 547 if (src.getValue() == null) { 548 tgt.setValue(null); 549 } else { 550 switch (src.getValue()) { 551 case SOURCE: 552 tgt.setValue(StructureMap.StructureMapModelMode.SOURCE); 553 break; 554 case QUERIED: 555 tgt.setValue(StructureMap.StructureMapModelMode.QUERIED); 556 break; 557 case TARGET: 558 tgt.setValue(StructureMap.StructureMapModelMode.TARGET); 559 break; 560 case PRODUCED: 561 tgt.setValue(StructureMap.StructureMapModelMode.PRODUCED); 562 break; 563 default: 564 tgt.setValue(StructureMap.StructureMapModelMode.NULL); 565 break; 566 } 567 } 568 return tgt; 569 } 570 571 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 572 if (src == null || src.isEmpty()) 573 return null; 574 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListModeEnumFactory()); 575 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 576 if (src.getValue() == null) { 577 tgt.setValue(null); 578 } else { 579 switch (src.getValue()) { 580 case FIRST: 581 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.FIRST); 582 break; 583 case NOTFIRST: 584 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NOTFIRST); 585 break; 586 case LAST: 587 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.LAST); 588 break; 589 case NOTLAST: 590 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NOTLAST); 591 break; 592 case ONLYONE: 593 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.ONLYONE); 594 break; 595 default: 596 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NULL); 597 break; 598 } 599 } 600 return tgt; 601 } 602 603 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 604 if (src == null || src.isEmpty()) 605 return null; 606 Enumeration<StructureMap.StructureMapSourceListMode> tgt = new Enumeration<>(new StructureMap.StructureMapSourceListModeEnumFactory()); 607 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 608 if (src.getValue() == null) { 609 tgt.setValue(null); 610 } else { 611 switch (src.getValue()) { 612 case FIRST: 613 tgt.setValue(StructureMap.StructureMapSourceListMode.FIRST); 614 break; 615 case NOTFIRST: 616 tgt.setValue(StructureMap.StructureMapSourceListMode.NOTFIRST); 617 break; 618 case LAST: 619 tgt.setValue(StructureMap.StructureMapSourceListMode.LAST); 620 break; 621 case NOTLAST: 622 tgt.setValue(StructureMap.StructureMapSourceListMode.NOTLAST); 623 break; 624 case ONLYONE: 625 tgt.setValue(StructureMap.StructureMapSourceListMode.ONLYONE); 626 break; 627 default: 628 tgt.setValue(StructureMap.StructureMapSourceListMode.NULL); 629 break; 630 } 631 } 632 return tgt; 633 } 634 635 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 636 if (src == null) 637 return null; 638 org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent(); 639 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 640 if (src.hasUrl()) 641 tgt.setUrl(src.getUrl()); 642 if (src.hasMode()) 643 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 644 if (src.hasAlias()) 645 tgt.setAliasElement(String30_40.convertString(src.getAliasElement())); 646 if (src.hasDocumentation()) 647 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 648 return tgt; 649 } 650 651 public static org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 652 if (src == null) 653 return null; 654 org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent(); 655 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 656 if (src.hasUrl()) 657 tgt.setUrl(src.getUrl()); 658 if (src.hasMode()) 659 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 660 if (src.hasAlias()) 661 tgt.setAliasElement(String30_40.convertString(src.getAliasElement())); 662 if (src.hasDocumentation()) 663 tgt.setDocumentationElement(String30_40.convertString(src.getDocumentationElement())); 664 return tgt; 665 } 666 667 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 668 if (src == null || src.isEmpty()) 669 return null; 670 Enumeration<StructureMap.StructureMapTargetListMode> tgt = new Enumeration<>(new StructureMap.StructureMapTargetListModeEnumFactory()); 671 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 672 if (src.getValue() == null) { 673 tgt.setValue(null); 674 } else { 675 switch (src.getValue()) { 676 case FIRST: 677 tgt.setValue(StructureMap.StructureMapTargetListMode.FIRST); 678 break; 679 case SHARE: 680 tgt.setValue(StructureMap.StructureMapTargetListMode.SHARE); 681 break; 682 case LAST: 683 tgt.setValue(StructureMap.StructureMapTargetListMode.LAST); 684 break; 685 case COLLATE: 686 tgt.setValue(StructureMap.StructureMapTargetListMode.COLLATE); 687 break; 688 default: 689 tgt.setValue(StructureMap.StructureMapTargetListMode.NULL); 690 break; 691 } 692 } 693 return tgt; 694 } 695 696 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 697 if (src == null || src.isEmpty()) 698 return null; 699 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListModeEnumFactory()); 700 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 701 if (src.getValue() == null) { 702 tgt.setValue(null); 703 } else { 704 switch (src.getValue()) { 705 case FIRST: 706 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.FIRST); 707 break; 708 case SHARE: 709 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.SHARE); 710 break; 711 case LAST: 712 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.LAST); 713 break; 714 case COLLATE: 715 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.COLLATE); 716 break; 717 default: 718 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.NULL); 719 break; 720 } 721 } 722 return tgt; 723 } 724 725 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> src) throws FHIRException { 726 if (src == null || src.isEmpty()) 727 return null; 728 Enumeration<StructureMap.StructureMapTransform> tgt = new Enumeration<>(new StructureMap.StructureMapTransformEnumFactory()); 729 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 730 if (src.getValue() == null) { 731 tgt.setValue(null); 732 } else { 733 switch (src.getValue()) { 734 case CREATE: 735 tgt.setValue(StructureMap.StructureMapTransform.CREATE); 736 break; 737 case COPY: 738 tgt.setValue(StructureMap.StructureMapTransform.COPY); 739 break; 740 case TRUNCATE: 741 tgt.setValue(StructureMap.StructureMapTransform.TRUNCATE); 742 break; 743 case ESCAPE: 744 tgt.setValue(StructureMap.StructureMapTransform.ESCAPE); 745 break; 746 case CAST: 747 tgt.setValue(StructureMap.StructureMapTransform.CAST); 748 break; 749 case APPEND: 750 tgt.setValue(StructureMap.StructureMapTransform.APPEND); 751 break; 752 case TRANSLATE: 753 tgt.setValue(StructureMap.StructureMapTransform.TRANSLATE); 754 break; 755 case REFERENCE: 756 tgt.setValue(StructureMap.StructureMapTransform.REFERENCE); 757 break; 758 case DATEOP: 759 tgt.setValue(StructureMap.StructureMapTransform.DATEOP); 760 break; 761 case UUID: 762 tgt.setValue(StructureMap.StructureMapTransform.UUID); 763 break; 764 case POINTER: 765 tgt.setValue(StructureMap.StructureMapTransform.POINTER); 766 break; 767 case EVALUATE: 768 tgt.setValue(StructureMap.StructureMapTransform.EVALUATE); 769 break; 770 case CC: 771 tgt.setValue(StructureMap.StructureMapTransform.CC); 772 break; 773 case C: 774 tgt.setValue(StructureMap.StructureMapTransform.C); 775 break; 776 case QTY: 777 tgt.setValue(StructureMap.StructureMapTransform.QTY); 778 break; 779 case ID: 780 tgt.setValue(StructureMap.StructureMapTransform.ID); 781 break; 782 case CP: 783 tgt.setValue(StructureMap.StructureMapTransform.CP); 784 break; 785 default: 786 tgt.setValue(StructureMap.StructureMapTransform.NULL); 787 break; 788 } 789 } 790 return tgt; 791 } 792 793 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> src) throws FHIRException { 794 if (src == null || src.isEmpty()) 795 return null; 796 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransformEnumFactory()); 797 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 798 if (src.getValue() == null) { 799 tgt.setValue(null); 800 } else { 801 switch (src.getValue()) { 802 case CREATE: 803 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CREATE); 804 break; 805 case COPY: 806 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.COPY); 807 break; 808 case TRUNCATE: 809 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.TRUNCATE); 810 break; 811 case ESCAPE: 812 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.ESCAPE); 813 break; 814 case CAST: 815 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CAST); 816 break; 817 case APPEND: 818 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.APPEND); 819 break; 820 case TRANSLATE: 821 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.TRANSLATE); 822 break; 823 case REFERENCE: 824 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.REFERENCE); 825 break; 826 case DATEOP: 827 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.DATEOP); 828 break; 829 case UUID: 830 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.UUID); 831 break; 832 case POINTER: 833 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.POINTER); 834 break; 835 case EVALUATE: 836 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.EVALUATE); 837 break; 838 case CC: 839 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CC); 840 break; 841 case C: 842 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.C); 843 break; 844 case QTY: 845 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.QTY); 846 break; 847 case ID: 848 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.ID); 849 break; 850 case CP: 851 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CP); 852 break; 853 default: 854 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.NULL); 855 break; 856 } 857 } 858 return tgt; 859 } 860}