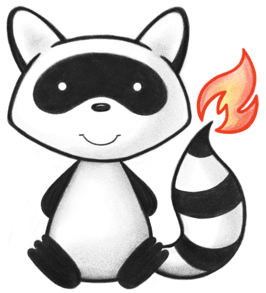
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Ratio30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.SimpleQuantity30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Substance30_40 { 013 014 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatusEnumFactory()); 018 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 019 switch (src.getValue()) { 020 case ACTIVE: 021 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.ACTIVE); 022 break; 023 case INACTIVE: 024 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.INACTIVE); 025 break; 026 case ENTEREDINERROR: 027 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 028 break; 029 default: 030 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.NULL); 031 break; 032 } 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 037 if (src == null || src.isEmpty()) 038 return null; 039 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatusEnumFactory()); 040 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 041 switch (src.getValue()) { 042 case ACTIVE: 043 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.ACTIVE); 044 break; 045 case INACTIVE: 046 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.INACTIVE); 047 break; 048 case ENTEREDINERROR: 049 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 050 break; 051 default: 052 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.NULL); 053 break; 054 } 055 return tgt; 056 } 057 058 public static org.hl7.fhir.r4.model.Substance convertSubstance(org.hl7.fhir.dstu3.model.Substance src) throws FHIRException { 059 if (src == null) 060 return null; 061 org.hl7.fhir.r4.model.Substance tgt = new org.hl7.fhir.r4.model.Substance(); 062 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 063 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 064 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 065 if (src.hasStatus()) 066 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 067 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 068 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 069 if (src.hasCode()) 070 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 071 if (src.hasDescription()) 072 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 073 for (org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 074 tgt.addInstance(convertSubstanceInstanceComponent(t)); 075 for (org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 076 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.dstu3.model.Substance convertSubstance(org.hl7.fhir.r4.model.Substance src) throws FHIRException { 081 if (src == null) 082 return null; 083 org.hl7.fhir.dstu3.model.Substance tgt = new org.hl7.fhir.dstu3.model.Substance(); 084 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 085 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 086 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 087 if (src.hasStatus()) 088 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 089 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 090 tgt.addCategory(CodeableConcept30_40.convertCodeableConcept(t)); 091 if (src.hasCode()) 092 tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 093 if (src.hasDescription()) 094 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 095 for (org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 096 tgt.addInstance(convertSubstanceInstanceComponent(t)); 097 for (org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 098 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 103 if (src == null) 104 return null; 105 org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent(); 106 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 107 if (src.hasQuantity()) 108 tgt.setQuantity(Ratio30_40.convertRatio(src.getQuantity())); 109 if (src.hasSubstance()) 110 tgt.setSubstance(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSubstance())); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 115 if (src == null) 116 return null; 117 org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent(); 118 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 119 if (src.hasQuantity()) 120 tgt.setQuantity(Ratio30_40.convertRatio(src.getQuantity())); 121 if (src.hasSubstance()) 122 tgt.setSubstance(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getSubstance())); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent(); 130 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 131 if (src.hasIdentifier()) 132 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 133 if (src.hasExpiry()) 134 tgt.setExpiryElement(DateTime30_40.convertDateTime(src.getExpiryElement())); 135 if (src.hasQuantity()) 136 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 137 return tgt; 138 } 139 140 public static org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent src) throws FHIRException { 141 if (src == null) 142 return null; 143 org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent(); 144 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 145 if (src.hasIdentifier()) 146 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 147 if (src.hasExpiry()) 148 tgt.setExpiryElement(DateTime30_40.convertDateTime(src.getExpiryElement())); 149 if (src.hasQuantity()) 150 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 151 return tgt; 152 } 153}