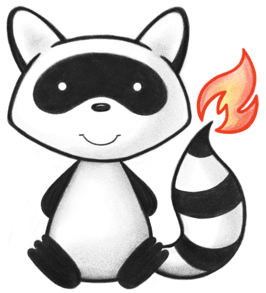
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.SimpleQuantity30_40; 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.r4.model.Enumeration; 010import org.hl7.fhir.r4.model.SupplyDelivery; 011 012public class SupplyDelivery30_40 { 013 014 public static org.hl7.fhir.r4.model.SupplyDelivery convertSupplyDelivery(org.hl7.fhir.dstu3.model.SupplyDelivery src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.r4.model.SupplyDelivery tgt = new org.hl7.fhir.r4.model.SupplyDelivery(); 018 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 019 if (src.hasIdentifier()) 020 tgt.addIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 021 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_40.convertReference(t)); 022 for (org.hl7.fhir.dstu3.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_40.convertReference(t)); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertSupplyDeliveryStatus(src.getStatusElement())); 025 if (src.hasPatient()) 026 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 027 if (src.hasType()) 028 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 029 if (src.hasSuppliedItem()) 030 tgt.setSuppliedItem(convertSupplyDeliverySuppliedItemComponent(src.getSuppliedItem())); 031 if (src.hasOccurrence()) 032 tgt.setOccurrence(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getOccurrence())); 033 if (src.hasSupplier()) 034 tgt.setSupplier(Reference30_40.convertReference(src.getSupplier())); 035 if (src.hasDestination()) 036 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 037 for (org.hl7.fhir.dstu3.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference30_40.convertReference(t)); 038 return tgt; 039 } 040 041 public static org.hl7.fhir.dstu3.model.SupplyDelivery convertSupplyDelivery(org.hl7.fhir.r4.model.SupplyDelivery src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.dstu3.model.SupplyDelivery tgt = new org.hl7.fhir.dstu3.model.SupplyDelivery(); 045 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 046 if (src.hasIdentifier()) 047 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifierFirstRep())); 048 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_40.convertReference(t)); 049 for (org.hl7.fhir.r4.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_40.convertReference(t)); 050 if (src.hasStatus()) 051 tgt.setStatusElement(convertSupplyDeliveryStatus(src.getStatusElement())); 052 if (src.hasPatient()) 053 tgt.setPatient(Reference30_40.convertReference(src.getPatient())); 054 if (src.hasType()) 055 tgt.setType(CodeableConcept30_40.convertCodeableConcept(src.getType())); 056 if (src.hasSuppliedItem()) 057 tgt.setSuppliedItem(convertSupplyDeliverySuppliedItemComponent(src.getSuppliedItem())); 058 if (src.hasOccurrence()) 059 tgt.setOccurrence(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getOccurrence())); 060 if (src.hasSupplier()) 061 tgt.setSupplier(Reference30_40.convertReference(src.getSupplier())); 062 if (src.hasDestination()) 063 tgt.setDestination(Reference30_40.convertReference(src.getDestination())); 064 for (org.hl7.fhir.r4.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference30_40.convertReference(t)); 065 return tgt; 066 } 067 068 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliveryStatus> convertSupplyDeliveryStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus> src) throws FHIRException { 069 if (src == null || src.isEmpty()) 070 return null; 071 Enumeration<SupplyDelivery.SupplyDeliveryStatus> tgt = new Enumeration<>(new SupplyDelivery.SupplyDeliveryStatusEnumFactory()); 072 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 073 if (src.getValue() == null) { 074 tgt.setValue(null); 075 } else { 076 switch (src.getValue()) { 077 case INPROGRESS: 078 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.INPROGRESS); 079 break; 080 case COMPLETED: 081 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.COMPLETED); 082 break; 083 case ABANDONED: 084 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.ABANDONED); 085 break; 086 case ENTEREDINERROR: 087 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.ENTEREDINERROR); 088 break; 089 default: 090 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.NULL); 091 break; 092 } 093 } 094 return tgt; 095 } 096 097 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus> convertSupplyDeliveryStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliveryStatus> src) throws FHIRException { 098 if (src == null || src.isEmpty()) 099 return null; 100 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatusEnumFactory()); 101 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 102 if (src.getValue() == null) { 103 tgt.setValue(null); 104 } else { 105 switch (src.getValue()) { 106 case INPROGRESS: 107 tgt.setValue(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus.INPROGRESS); 108 break; 109 case COMPLETED: 110 tgt.setValue(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus.COMPLETED); 111 break; 112 case ABANDONED: 113 tgt.setValue(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus.ABANDONED); 114 break; 115 case ENTEREDINERROR: 116 tgt.setValue(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus.ENTEREDINERROR); 117 break; 118 default: 119 tgt.setValue(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliveryStatus.NULL); 120 break; 121 } 122 } 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent convertSupplyDeliverySuppliedItemComponent(org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent tgt = new org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent(); 130 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 131 if (src.hasQuantity()) 132 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 133 if (src.hasItem()) 134 tgt.setItem(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getItem())); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent convertSupplyDeliverySuppliedItemComponent(org.hl7.fhir.r4.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent src) throws FHIRException { 139 if (src == null) 140 return null; 141 org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent tgt = new org.hl7.fhir.dstu3.model.SupplyDelivery.SupplyDeliverySuppliedItemComponent(); 142 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 143 if (src.hasQuantity()) 144 tgt.setQuantity(SimpleQuantity30_40.convertSimpleQuantity(src.getQuantity())); 145 if (src.hasItem()) 146 tgt.setItem(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getItem())); 147 return tgt; 148 } 149}