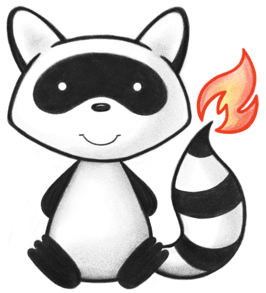
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.dstu3.model.TestReport; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class TestReport30_40 { 016 017 public static org.hl7.fhir.r4.model.TestReport convertTestReport(org.hl7.fhir.dstu3.model.TestReport src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.r4.model.TestReport tgt = new org.hl7.fhir.r4.model.TestReport(); 021 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 022 if (src.hasIdentifier()) 023 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 024 if (src.hasName()) 025 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertTestReportStatus(src.getStatusElement())); 028 if (src.hasTestScript()) 029 tgt.setTestScript(Reference30_40.convertReference(src.getTestScript())); 030 if (src.hasResult()) 031 tgt.setResultElement(convertTestReportResult(src.getResultElement())); 032 if (src.hasScore()) 033 tgt.setScoreElement(Decimal30_40.convertDecimal(src.getScoreElement())); 034 if (src.hasTester()) 035 tgt.setTesterElement(String30_40.convertString(src.getTesterElement())); 036 if (src.hasIssued()) 037 tgt.setIssuedElement(DateTime30_40.convertDateTime(src.getIssuedElement())); 038 for (org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantComponent t : src.getParticipant()) 039 tgt.addParticipant(convertTestReportParticipantComponent(t)); 040 if (src.hasSetup()) 041 tgt.setSetup(convertTestReportSetupComponent(src.getSetup())); 042 for (org.hl7.fhir.dstu3.model.TestReport.TestReportTestComponent t : src.getTest()) 043 tgt.addTest(convertTestReportTestComponent(t)); 044 if (src.hasTeardown()) 045 tgt.setTeardown(convertTestReportTeardownComponent(src.getTeardown())); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.dstu3.model.TestReport convertTestReport(org.hl7.fhir.r4.model.TestReport src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.dstu3.model.TestReport tgt = new org.hl7.fhir.dstu3.model.TestReport(); 053 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 054 if (src.hasIdentifier()) 055 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 056 if (src.hasName()) 057 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 058 if (src.hasStatus()) 059 tgt.setStatusElement(convertTestReportStatus(src.getStatusElement())); 060 if (src.hasTestScript()) 061 tgt.setTestScript(Reference30_40.convertReference(src.getTestScript())); 062 if (src.hasResult()) 063 tgt.setResultElement(convertTestReportResult(src.getResultElement())); 064 if (src.hasScore()) 065 tgt.setScoreElement(Decimal30_40.convertDecimal(src.getScoreElement())); 066 if (src.hasTester()) 067 tgt.setTesterElement(String30_40.convertString(src.getTesterElement())); 068 if (src.hasIssued()) 069 tgt.setIssuedElement(DateTime30_40.convertDateTime(src.getIssuedElement())); 070 for (org.hl7.fhir.r4.model.TestReport.TestReportParticipantComponent t : src.getParticipant()) 071 tgt.addParticipant(convertTestReportParticipantComponent(t)); 072 if (src.hasSetup()) 073 tgt.setSetup(convertTestReportSetupComponent(src.getSetup())); 074 for (org.hl7.fhir.r4.model.TestReport.TestReportTestComponent t : src.getTest()) 075 tgt.addTest(convertTestReportTestComponent(t)); 076 if (src.hasTeardown()) 077 tgt.setTeardown(convertTestReportTeardownComponent(src.getTeardown())); 078 return tgt; 079 } 080 081 public static org.hl7.fhir.r4.model.TestReport.TestReportParticipantComponent convertTestReportParticipantComponent(org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantComponent src) throws FHIRException { 082 if (src == null) 083 return null; 084 org.hl7.fhir.r4.model.TestReport.TestReportParticipantComponent tgt = new org.hl7.fhir.r4.model.TestReport.TestReportParticipantComponent(); 085 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 086 if (src.hasType()) 087 tgt.setTypeElement(convertTestReportParticipantType(src.getTypeElement())); 088 if (src.hasUri()) 089 tgt.setUriElement(Uri30_40.convertUri(src.getUriElement())); 090 if (src.hasDisplay()) 091 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantComponent convertTestReportParticipantComponent(org.hl7.fhir.r4.model.TestReport.TestReportParticipantComponent src) throws FHIRException { 096 if (src == null) 097 return null; 098 org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantComponent(); 099 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 100 if (src.hasType()) 101 tgt.setTypeElement(convertTestReportParticipantType(src.getTypeElement())); 102 if (src.hasUri()) 103 tgt.setUriElement(Uri30_40.convertUri(src.getUriElement())); 104 if (src.hasDisplay()) 105 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantType> convertTestReportParticipantType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportParticipantType> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 Enumeration<TestReport.TestReportParticipantType> tgt = new Enumeration<>(new TestReport.TestReportParticipantTypeEnumFactory()); 113 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 114 if (src.getValue() == null) { 115 tgt.setValue(null); 116 } else { 117 switch (src.getValue()) { 118 case TESTENGINE: 119 tgt.setValue(TestReport.TestReportParticipantType.TESTENGINE); 120 break; 121 case CLIENT: 122 tgt.setValue(TestReport.TestReportParticipantType.CLIENT); 123 break; 124 case SERVER: 125 tgt.setValue(TestReport.TestReportParticipantType.SERVER); 126 break; 127 default: 128 tgt.setValue(TestReport.TestReportParticipantType.NULL); 129 break; 130 } 131 } 132 return tgt; 133 } 134 135 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportParticipantType> convertTestReportParticipantType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportParticipantType> src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportParticipantType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestReport.TestReportParticipantTypeEnumFactory()); 139 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 140 if (src.getValue() == null) { 141 tgt.setValue(null); 142 } else { 143 switch (src.getValue()) { 144 case TESTENGINE: 145 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportParticipantType.TESTENGINE); 146 break; 147 case CLIENT: 148 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportParticipantType.CLIENT); 149 break; 150 case SERVER: 151 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportParticipantType.SERVER); 152 break; 153 default: 154 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportParticipantType.NULL); 155 break; 156 } 157 } 158 return tgt; 159 } 160 161 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportResult> convertTestReportResult(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportResult> src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportResult> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestReport.TestReportResultEnumFactory()); 165 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 166 if (src.getValue() == null) { 167 tgt.setValue(null); 168 } else { 169 switch (src.getValue()) { 170 case PASS: 171 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportResult.PASS); 172 break; 173 case FAIL: 174 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportResult.FAIL); 175 break; 176 case PENDING: 177 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportResult.PENDING); 178 break; 179 default: 180 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportResult.NULL); 181 break; 182 } 183 } 184 return tgt; 185 } 186 187 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportResult> convertTestReportResult(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportResult> src) throws FHIRException { 188 if (src == null || src.isEmpty()) 189 return null; 190 Enumeration<TestReport.TestReportResult> tgt = new Enumeration<>(new TestReport.TestReportResultEnumFactory()); 191 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 192 if (src.getValue() == null) { 193 tgt.setValue(null); 194 } else { 195 switch (src.getValue()) { 196 case PASS: 197 tgt.setValue(TestReport.TestReportResult.PASS); 198 break; 199 case FAIL: 200 tgt.setValue(TestReport.TestReportResult.FAIL); 201 break; 202 case PENDING: 203 tgt.setValue(TestReport.TestReportResult.PENDING); 204 break; 205 default: 206 tgt.setValue(TestReport.TestReportResult.NULL); 207 break; 208 } 209 } 210 return tgt; 211 } 212 213 public static org.hl7.fhir.r4.model.TestReport.TestReportSetupComponent convertTestReportSetupComponent(org.hl7.fhir.dstu3.model.TestReport.TestReportSetupComponent src) throws FHIRException { 214 if (src == null) 215 return null; 216 org.hl7.fhir.r4.model.TestReport.TestReportSetupComponent tgt = new org.hl7.fhir.r4.model.TestReport.TestReportSetupComponent(); 217 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 218 for (org.hl7.fhir.dstu3.model.TestReport.SetupActionComponent t : src.getAction()) 219 tgt.addAction(convertSetupActionComponent(t)); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.dstu3.model.TestReport.TestReportSetupComponent convertTestReportSetupComponent(org.hl7.fhir.r4.model.TestReport.TestReportSetupComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.dstu3.model.TestReport.TestReportSetupComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TestReportSetupComponent(); 227 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 228 for (org.hl7.fhir.r4.model.TestReport.SetupActionComponent t : src.getAction()) 229 tgt.addAction(convertSetupActionComponent(t)); 230 return tgt; 231 } 232 233 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportStatus> convertTestReportStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportStatus> src) throws FHIRException { 234 if (src == null || src.isEmpty()) 235 return null; 236 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestReport.TestReportStatusEnumFactory()); 237 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(null); 240 } else { 241 switch (src.getValue()) { 242 case COMPLETED: 243 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.COMPLETED); 244 break; 245 case INPROGRESS: 246 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.INPROGRESS); 247 break; 248 case WAITING: 249 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.WAITING); 250 break; 251 case STOPPED: 252 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.STOPPED); 253 break; 254 case ENTEREDINERROR: 255 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.ENTEREDINERROR); 256 break; 257 default: 258 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportStatus.NULL); 259 break; 260 } 261 } 262 return tgt; 263 } 264 265 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportStatus> convertTestReportStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportStatus> src) throws FHIRException { 266 if (src == null || src.isEmpty()) 267 return null; 268 Enumeration<TestReport.TestReportStatus> tgt = new Enumeration<>(new TestReport.TestReportStatusEnumFactory()); 269 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 270 if (src.getValue() == null) { 271 tgt.setValue(null); 272 } else { 273 switch (src.getValue()) { 274 case COMPLETED: 275 tgt.setValue(TestReport.TestReportStatus.COMPLETED); 276 break; 277 case INPROGRESS: 278 tgt.setValue(TestReport.TestReportStatus.INPROGRESS); 279 break; 280 case WAITING: 281 tgt.setValue(TestReport.TestReportStatus.WAITING); 282 break; 283 case STOPPED: 284 tgt.setValue(TestReport.TestReportStatus.STOPPED); 285 break; 286 case ENTEREDINERROR: 287 tgt.setValue(TestReport.TestReportStatus.ENTEREDINERROR); 288 break; 289 default: 290 tgt.setValue(TestReport.TestReportStatus.NULL); 291 break; 292 } 293 } 294 return tgt; 295 } 296 297 public static org.hl7.fhir.r4.model.TestReport.TestReportTeardownComponent convertTestReportTeardownComponent(org.hl7.fhir.dstu3.model.TestReport.TestReportTeardownComponent src) throws FHIRException { 298 if (src == null) 299 return null; 300 org.hl7.fhir.r4.model.TestReport.TestReportTeardownComponent tgt = new org.hl7.fhir.r4.model.TestReport.TestReportTeardownComponent(); 301 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 302 for (org.hl7.fhir.dstu3.model.TestReport.TeardownActionComponent t : src.getAction()) 303 tgt.addAction(convertTeardownActionComponent(t)); 304 return tgt; 305 } 306 307 public static org.hl7.fhir.dstu3.model.TestReport.TestReportTeardownComponent convertTestReportTeardownComponent(org.hl7.fhir.r4.model.TestReport.TestReportTeardownComponent src) throws FHIRException { 308 if (src == null) 309 return null; 310 org.hl7.fhir.dstu3.model.TestReport.TestReportTeardownComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TestReportTeardownComponent(); 311 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 312 for (org.hl7.fhir.r4.model.TestReport.TeardownActionComponent t : src.getAction()) 313 tgt.addAction(convertTeardownActionComponent(t)); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.dstu3.model.TestReport.TestReportTestComponent convertTestReportTestComponent(org.hl7.fhir.r4.model.TestReport.TestReportTestComponent src) throws FHIRException { 318 if (src == null) 319 return null; 320 org.hl7.fhir.dstu3.model.TestReport.TestReportTestComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TestReportTestComponent(); 321 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 322 if (src.hasName()) 323 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 324 if (src.hasDescription()) 325 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 326 for (org.hl7.fhir.r4.model.TestReport.TestActionComponent t : src.getAction()) 327 tgt.addAction(convertTestActionComponent(t)); 328 return tgt; 329 } 330 331 public static org.hl7.fhir.r4.model.TestReport.TestReportTestComponent convertTestReportTestComponent(org.hl7.fhir.dstu3.model.TestReport.TestReportTestComponent src) throws FHIRException { 332 if (src == null) 333 return null; 334 org.hl7.fhir.r4.model.TestReport.TestReportTestComponent tgt = new org.hl7.fhir.r4.model.TestReport.TestReportTestComponent(); 335 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 336 if (src.hasName()) 337 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 338 if (src.hasDescription()) 339 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 340 for (org.hl7.fhir.dstu3.model.TestReport.TestActionComponent t : src.getAction()) 341 tgt.addAction(convertTestActionComponent(t)); 342 return tgt; 343 } 344 345 public static org.hl7.fhir.r4.model.TestReport.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu3.model.TestReport.SetupActionComponent src) throws FHIRException { 346 if (src == null) return null; 347 org.hl7.fhir.r4.model.TestReport.SetupActionComponent tgt = new org.hl7.fhir.r4.model.TestReport.SetupActionComponent(); 348 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 349 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 350 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 351 return tgt; 352 } 353 354 public static org.hl7.fhir.dstu3.model.TestReport.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r4.model.TestReport.SetupActionComponent src) throws FHIRException { 355 if (src == null) return null; 356 org.hl7.fhir.dstu3.model.TestReport.SetupActionComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.SetupActionComponent(); 357 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 358 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 359 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 360 return tgt; 361 } 362 363 public static org.hl7.fhir.r4.model.TestReport.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu3.model.TestReport.SetupActionOperationComponent src) throws FHIRException { 364 if (src == null) return null; 365 org.hl7.fhir.r4.model.TestReport.SetupActionOperationComponent tgt = new org.hl7.fhir.r4.model.TestReport.SetupActionOperationComponent(); 366 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 367 if (src.hasResult()) tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 368 if (src.hasMessage()) tgt.setMessageElement(MarkDown30_40.convertMarkdown(src.getMessageElement())); 369 if (src.hasDetail()) tgt.setDetailElement(Uri30_40.convertUri(src.getDetailElement())); 370 return tgt; 371 } 372 373 public static org.hl7.fhir.dstu3.model.TestReport.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r4.model.TestReport.SetupActionOperationComponent src) throws FHIRException { 374 if (src == null) return null; 375 org.hl7.fhir.dstu3.model.TestReport.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.SetupActionOperationComponent(); 376 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 377 if (src.hasResult()) tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 378 if (src.hasMessage()) tgt.setMessageElement(MarkDown30_40.convertMarkdown(src.getMessageElement())); 379 if (src.hasDetail()) tgt.setDetailElement(Uri30_40.convertUri(src.getDetailElement())); 380 return tgt; 381 } 382 383 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportActionResult> convertTestReportActionResult(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportActionResult> src) throws FHIRException { 384 if (src == null || src.isEmpty()) return null; 385 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportActionResult> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestReport.TestReportActionResultEnumFactory()); 386 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 387 if (src.getValue() == null) { 388 tgt.setValue(null); 389} else { 390 switch(src.getValue()) { 391 case PASS: 392 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.PASS); 393 break; 394 case SKIP: 395 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.SKIP); 396 break; 397 case FAIL: 398 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.FAIL); 399 break; 400 case WARNING: 401 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.WARNING); 402 break; 403 case ERROR: 404 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.ERROR); 405 break; 406 default: 407 tgt.setValue(org.hl7.fhir.r4.model.TestReport.TestReportActionResult.NULL); 408 break; 409 } 410} 411 return tgt; 412 } 413 414 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportActionResult> convertTestReportActionResult(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestReport.TestReportActionResult> src) throws FHIRException { 415 if (src == null || src.isEmpty()) return null; 416 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestReport.TestReportActionResult> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestReport.TestReportActionResultEnumFactory()); 417 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 418 if (src.getValue() == null) { 419 tgt.setValue(null); 420} else { 421 switch(src.getValue()) { 422 case PASS: 423 tgt.setValue(TestReport.TestReportActionResult.PASS); 424 break; 425 case SKIP: 426 tgt.setValue(TestReport.TestReportActionResult.SKIP); 427 break; 428 case FAIL: 429 tgt.setValue(TestReport.TestReportActionResult.FAIL); 430 break; 431 case WARNING: 432 tgt.setValue(TestReport.TestReportActionResult.WARNING); 433 break; 434 case ERROR: 435 tgt.setValue(TestReport.TestReportActionResult.ERROR); 436 break; 437 default: 438 tgt.setValue(TestReport.TestReportActionResult.NULL); 439 break; 440 } 441} 442 return tgt; 443 } 444 445 public static org.hl7.fhir.r4.model.TestReport.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu3.model.TestReport.SetupActionAssertComponent src) throws FHIRException { 446 if (src == null) return null; 447 org.hl7.fhir.r4.model.TestReport.SetupActionAssertComponent tgt = new org.hl7.fhir.r4.model.TestReport.SetupActionAssertComponent(); 448 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 449 if (src.hasResult()) tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 450 if (src.hasMessage()) tgt.setMessageElement(MarkDown30_40.convertMarkdown(src.getMessageElement())); 451 if (src.hasDetail()) tgt.setDetailElement(String30_40.convertString(src.getDetailElement())); 452 return tgt; 453 } 454 455 public static org.hl7.fhir.dstu3.model.TestReport.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r4.model.TestReport.SetupActionAssertComponent src) throws FHIRException { 456 if (src == null) return null; 457 org.hl7.fhir.dstu3.model.TestReport.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.SetupActionAssertComponent(); 458 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 459 if (src.hasResult()) tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 460 if (src.hasMessage()) tgt.setMessageElement(MarkDown30_40.convertMarkdown(src.getMessageElement())); 461 if (src.hasDetail()) tgt.setDetailElement(String30_40.convertString(src.getDetailElement())); 462 return tgt; 463 } 464 465 public static org.hl7.fhir.r4.model.TestReport.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu3.model.TestReport.TestActionComponent src) throws FHIRException { 466 if (src == null) return null; 467 org.hl7.fhir.r4.model.TestReport.TestActionComponent tgt = new org.hl7.fhir.r4.model.TestReport.TestActionComponent(); 468 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 469 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 470 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 471 return tgt; 472 } 473 474 public static org.hl7.fhir.dstu3.model.TestReport.TestActionComponent convertTestActionComponent(org.hl7.fhir.r4.model.TestReport.TestActionComponent src) throws FHIRException { 475 if (src == null) return null; 476 org.hl7.fhir.dstu3.model.TestReport.TestActionComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TestActionComponent(); 477 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 478 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 479 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 480 return tgt; 481 } 482 483 public static org.hl7.fhir.r4.model.TestReport.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu3.model.TestReport.TeardownActionComponent src) throws FHIRException { 484 if (src == null) return null; 485 org.hl7.fhir.r4.model.TestReport.TeardownActionComponent tgt = new org.hl7.fhir.r4.model.TestReport.TeardownActionComponent(); 486 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 487 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 488 return tgt; 489 } 490 491 public static org.hl7.fhir.dstu3.model.TestReport.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r4.model.TestReport.TeardownActionComponent src) throws FHIRException { 492 if (src == null) return null; 493 org.hl7.fhir.dstu3.model.TestReport.TeardownActionComponent tgt = new org.hl7.fhir.dstu3.model.TestReport.TeardownActionComponent(); 494 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 495 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 496 return tgt; 497 } 498}