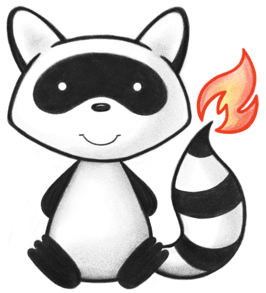
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Id30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 017import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 018import org.hl7.fhir.exceptions.FHIRException; 019 020public class TestScript30_40 { 021 022 public static org.hl7.fhir.r4.model.TestScript convertTestScript(org.hl7.fhir.dstu3.model.TestScript src) throws FHIRException { 023 if (src == null) 024 return null; 025 org.hl7.fhir.r4.model.TestScript tgt = new org.hl7.fhir.r4.model.TestScript(); 026 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 027 if (src.hasUrl()) 028 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 029 if (src.hasIdentifier()) 030 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 031 if (src.hasVersion()) 032 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 033 if (src.hasName()) 034 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 035 if (src.hasTitle()) 036 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 037 if (src.hasStatus()) 038 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 039 if (src.hasExperimental()) 040 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 041 if (src.hasDateElement()) 042 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 043 if (src.hasPublisher()) 044 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 045 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 046 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 047 if (src.hasDescription()) 048 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 049 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 050 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 051 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 052 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 053 if (src.hasPurpose()) 054 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 055 if (src.hasCopyright()) 056 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 057 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 058 tgt.addOrigin(convertTestScriptOriginComponent(t)); 059 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 060 tgt.addDestination(convertTestScriptDestinationComponent(t)); 061 if (src.hasMetadata()) 062 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 063 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 064 tgt.addFixture(convertTestScriptFixtureComponent(t)); 065 for (org.hl7.fhir.dstu3.model.Reference t : src.getProfile()) tgt.addProfile(Reference30_40.convertReference(t)); 066 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 067 tgt.addVariable(convertTestScriptVariableComponent(t)); 068 if (src.hasSetup()) 069 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 070 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent t : src.getTest()) 071 tgt.addTest(convertTestScriptTestComponent(t)); 072 if (src.hasTeardown()) 073 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu3.model.TestScript convertTestScript(org.hl7.fhir.r4.model.TestScript src) throws FHIRException { 078 if (src == null) 079 return null; 080 org.hl7.fhir.dstu3.model.TestScript tgt = new org.hl7.fhir.dstu3.model.TestScript(); 081 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 082 if (src.hasUrl()) 083 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 084 if (src.hasIdentifier()) 085 tgt.setIdentifier(Identifier30_40.convertIdentifier(src.getIdentifier())); 086 if (src.hasVersion()) 087 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 088 if (src.hasName()) 089 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 090 if (src.hasTitle()) 091 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 092 if (src.hasStatus()) 093 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 094 if (src.hasExperimental()) 095 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 096 if (src.hasDateElement()) 097 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 098 if (src.hasPublisher()) 099 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 100 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 101 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 102 if (src.hasDescription()) 103 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 104 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 105 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 106 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 107 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 108 if (src.hasPurpose()) 109 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 110 if (src.hasCopyright()) 111 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 112 for (org.hl7.fhir.r4.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 113 tgt.addOrigin(convertTestScriptOriginComponent(t)); 114 for (org.hl7.fhir.r4.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 115 tgt.addDestination(convertTestScriptDestinationComponent(t)); 116 if (src.hasMetadata()) 117 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 118 for (org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 119 tgt.addFixture(convertTestScriptFixtureComponent(t)); 120 for (org.hl7.fhir.r4.model.Reference t : src.getProfile()) tgt.addProfile(Reference30_40.convertReference(t)); 121 for (org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 122 tgt.addVariable(convertTestScriptVariableComponent(t)); 123 if (src.hasSetup()) 124 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 125 for (org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent t : src.getTest()) 126 tgt.addTest(convertTestScriptTestComponent(t)); 127 if (src.hasTeardown()) 128 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 129 return tgt; 130 } 131 132 public static org.hl7.fhir.r4.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 133 if (src == null) 134 return null; 135 org.hl7.fhir.r4.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptDestinationComponent(); 136 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 137 if (src.hasIndex()) 138 tgt.setIndexElement(Integer30_40.convertInteger(src.getIndexElement())); 139 if (src.hasProfile()) 140 tgt.setProfile(Coding30_40.convertCoding(src.getProfile())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.r4.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 145 if (src == null) 146 return null; 147 org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent(); 148 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 149 if (src.hasIndex()) 150 tgt.setIndexElement(Integer30_40.convertInteger(src.getIndexElement())); 151 if (src.hasProfile()) 152 tgt.setProfile(Coding30_40.convertCoding(src.getProfile())); 153 return tgt; 154 } 155 156 public static org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 157 if (src == null) 158 return null; 159 org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent(); 160 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 161 if (src.hasAutocreate()) 162 tgt.setAutocreateElement(Boolean30_40.convertBoolean(src.getAutocreateElement())); 163 if (src.hasAutodelete()) 164 tgt.setAutodeleteElement(Boolean30_40.convertBoolean(src.getAutodeleteElement())); 165 if (src.hasResource()) 166 tgt.setResource(Reference30_40.convertReference(src.getResource())); 167 return tgt; 168 } 169 170 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 171 if (src == null) 172 return null; 173 org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent(); 174 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 175 if (src.hasAutocreate()) 176 tgt.setAutocreateElement(Boolean30_40.convertBoolean(src.getAutocreateElement())); 177 if (src.hasAutodelete()) 178 tgt.setAutodeleteElement(Boolean30_40.convertBoolean(src.getAutodeleteElement())); 179 if (src.hasResource()) 180 tgt.setResource(Reference30_40.convertReference(src.getResource())); 181 return tgt; 182 } 183 184 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 185 if (src == null) 186 return null; 187 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent(); 188 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 189 if (src.hasRequired()) 190 tgt.setRequiredElement(Boolean30_40.convertBoolean(src.getRequiredElement())); 191 if (src.hasValidated()) 192 tgt.setValidatedElement(Boolean30_40.convertBoolean(src.getValidatedElement())); 193 if (src.hasDescription()) 194 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 195 for (org.hl7.fhir.dstu3.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 196 if (src.hasDestination()) 197 tgt.setDestinationElement(Integer30_40.convertInteger(src.getDestinationElement())); 198 for (org.hl7.fhir.dstu3.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 199 if (src.hasCapabilities()) 200 tgt.setCapabilitiesElement(Reference30_40.convertReferenceToCanonical(src.getCapabilities())); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 205 if (src == null) 206 return null; 207 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent(); 208 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 209 if (src.hasRequired()) 210 tgt.setRequiredElement(Boolean30_40.convertBoolean(src.getRequiredElement())); 211 if (src.hasValidated()) 212 tgt.setValidatedElement(Boolean30_40.convertBoolean(src.getValidatedElement())); 213 if (src.hasDescription()) 214 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 215 for (org.hl7.fhir.r4.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 216 if (src.hasDestination()) 217 tgt.setDestinationElement(Integer30_40.convertInteger(src.getDestinationElement())); 218 for (org.hl7.fhir.r4.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 219 if (src.hasCapabilities()) 220 tgt.setCapabilities(Reference30_40.convertCanonicalToReference(src.getCapabilitiesElement())); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 225 if (src == null) 226 return null; 227 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent(); 228 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 229 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 230 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 231 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 232 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 237 if (src == null) 238 return null; 239 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent(); 240 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 241 for (org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 242 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 243 for (org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 244 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 245 return tgt; 246 } 247 248 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 249 if (src == null) 250 return null; 251 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent(); 252 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 253 if (src.hasUrl()) 254 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 255 if (src.hasDescription()) 256 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 257 return tgt; 258 } 259 260 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 261 if (src == null) 262 return null; 263 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent(); 264 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 265 if (src.hasUrl()) 266 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 267 if (src.hasDescription()) 268 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 269 return tgt; 270 } 271 272 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.r4.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 273 if (src == null) 274 return null; 275 org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent(); 276 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 277 if (src.hasIndex()) 278 tgt.setIndexElement(Integer30_40.convertInteger(src.getIndexElement())); 279 if (src.hasProfile()) 280 tgt.setProfile(Coding30_40.convertCoding(src.getProfile())); 281 return tgt; 282 } 283 284 public static org.hl7.fhir.r4.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 285 if (src == null) 286 return null; 287 org.hl7.fhir.r4.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptOriginComponent(); 288 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 289 if (src.hasIndex()) 290 tgt.setIndexElement(Integer30_40.convertInteger(src.getIndexElement())); 291 if (src.hasProfile()) 292 tgt.setProfile(Coding30_40.convertCoding(src.getProfile())); 293 return tgt; 294 } 295 296 public static org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 297 if (src == null) 298 return null; 299 org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent(); 300 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 301 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent t : src.getAction()) 302 tgt.addAction(convertSetupActionComponent(t)); 303 return tgt; 304 } 305 306 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 307 if (src == null) 308 return null; 309 org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent(); 310 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 311 for (org.hl7.fhir.r4.model.TestScript.SetupActionComponent t : src.getAction()) 312 tgt.addAction(convertSetupActionComponent(t)); 313 return tgt; 314 } 315 316 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 317 if (src == null) 318 return null; 319 org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent(); 320 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 321 for (org.hl7.fhir.r4.model.TestScript.TeardownActionComponent t : src.getAction()) 322 tgt.addAction(convertTeardownActionComponent(t)); 323 return tgt; 324 } 325 326 public static org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 327 if (src == null) 328 return null; 329 org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent(); 330 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 331 for (org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent t : src.getAction()) 332 tgt.addAction(convertTeardownActionComponent(t)); 333 return tgt; 334 } 335 336 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent src) throws FHIRException { 337 if (src == null) 338 return null; 339 org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent(); 340 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 341 if (src.hasName()) 342 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 343 if (src.hasDescription()) 344 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 345 for (org.hl7.fhir.r4.model.TestScript.TestActionComponent t : src.getAction()) 346 tgt.addAction(convertTestActionComponent(t)); 347 return tgt; 348 } 349 350 public static org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent src) throws FHIRException { 351 if (src == null) 352 return null; 353 org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent(); 354 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 355 if (src.hasName()) 356 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 357 if (src.hasDescription()) 358 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 359 for (org.hl7.fhir.dstu3.model.TestScript.TestActionComponent t : src.getAction()) 360 tgt.addAction(convertTestActionComponent(t)); 361 return tgt; 362 } 363 364 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 365 if (src == null) 366 return null; 367 org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent(); 368 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 369 if (src.hasName()) 370 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 371 if (src.hasDefaultValue()) 372 tgt.setDefaultValueElement(String30_40.convertString(src.getDefaultValueElement())); 373 if (src.hasDescription()) 374 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 375 if (src.hasExpression()) 376 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 377 if (src.hasHeaderField()) 378 tgt.setHeaderFieldElement(String30_40.convertString(src.getHeaderFieldElement())); 379 if (src.hasHint()) 380 tgt.setHintElement(String30_40.convertString(src.getHintElement())); 381 if (src.hasPath()) 382 tgt.setPathElement(String30_40.convertString(src.getPathElement())); 383 if (src.hasSourceId()) 384 tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 385 return tgt; 386 } 387 388 public static org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 389 if (src == null) 390 return null; 391 org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent(); 392 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 393 if (src.hasName()) 394 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 395 if (src.hasDefaultValue()) 396 tgt.setDefaultValueElement(String30_40.convertString(src.getDefaultValueElement())); 397 if (src.hasDescription()) 398 tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 399 if (src.hasExpression()) 400 tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 401 if (src.hasHeaderField()) 402 tgt.setHeaderFieldElement(String30_40.convertString(src.getHeaderFieldElement())); 403 if (src.hasHint()) 404 tgt.setHintElement(String30_40.convertString(src.getHintElement())); 405 if (src.hasPath()) 406 tgt.setPathElement(String30_40.convertString(src.getPathElement())); 407 if (src.hasSourceId()) 408 tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 409 return tgt; 410 } 411 412 public static org.hl7.fhir.r4.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent src) throws FHIRException { 413 if (src == null) return null; 414 org.hl7.fhir.r4.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionComponent(); 415 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 416 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 417 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 418 return tgt; 419 } 420 421 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r4.model.TestScript.SetupActionComponent src) throws FHIRException { 422 if (src == null) return null; 423 org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent(); 424 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 425 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 426 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 427 return tgt; 428 } 429 430 public static org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 431 if (src == null) return null; 432 org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent(); 433 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 434 if (src.hasType()) tgt.setType(Coding30_40.convertCoding(src.getType())); 435 if (src.hasResource()) tgt.setResourceElement(Code30_40.convertCode(src.getResourceElement())); 436 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 437 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 438 if (src.hasAccept()) tgt.setAccept(convertContentType(src.getAccept())); 439 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 440 if (src.hasDestination()) tgt.setDestinationElement(Integer30_40.convertInteger(src.getDestinationElement())); 441 if (src.hasEncodeRequestUrl()) 442 tgt.setEncodeRequestUrlElement(Boolean30_40.convertBoolean(src.getEncodeRequestUrlElement())); 443 if (src.hasOrigin()) tgt.setOriginElement(Integer30_40.convertInteger(src.getOriginElement())); 444 if (src.hasParams()) tgt.setParamsElement(String30_40.convertString(src.getParamsElement())); 445 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 446 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 447 if (src.hasRequestId()) tgt.setRequestIdElement(Id30_40.convertId(src.getRequestIdElement())); 448 if (src.hasResponseId()) tgt.setResponseIdElement(Id30_40.convertId(src.getResponseIdElement())); 449 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 450 if (src.hasTargetId()) tgt.setTargetId(src.getTargetId()); 451 if (src.hasUrl()) tgt.setUrlElement(String30_40.convertString(src.getUrlElement())); 452 return tgt; 453 } 454 455 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 456 if (src == null) return null; 457 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent(); 458 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 459 if (src.hasType()) tgt.setType(Coding30_40.convertCoding(src.getType())); 460 if (src.hasResource()) tgt.setResourceElement(Code30_40.convertCode(src.getResourceElement())); 461 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 462 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 463 if (src.hasAccept()) tgt.setAccept(convertContentType(src.getAccept())); 464 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 465 if (src.hasDestination()) tgt.setDestinationElement(Integer30_40.convertInteger(src.getDestinationElement())); 466 if (src.hasEncodeRequestUrl()) 467 tgt.setEncodeRequestUrlElement(Boolean30_40.convertBoolean(src.getEncodeRequestUrlElement())); 468 if (src.hasOrigin()) tgt.setOriginElement(Integer30_40.convertInteger(src.getOriginElement())); 469 if (src.hasParams()) tgt.setParamsElement(String30_40.convertString(src.getParamsElement())); 470 for (org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 471 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 472 if (src.hasRequestId()) tgt.setRequestIdElement(Id30_40.convertId(src.getRequestIdElement())); 473 if (src.hasResponseId()) tgt.setResponseIdElement(Id30_40.convertId(src.getResponseIdElement())); 474 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 475 if (src.hasTargetId()) tgt.setTargetId(src.getTargetId()); 476 if (src.hasUrl()) tgt.setUrlElement(String30_40.convertString(src.getUrlElement())); 477 return tgt; 478 } 479 480 static public String convertContentType(org.hl7.fhir.dstu3.model.TestScript.ContentType src) throws FHIRException { 481 if (src == null) return null; 482 switch (src) { 483 case XML: 484 return "application/fhir+xml"; 485 case JSON: 486 return "application/fhir+json"; 487 case TTL: 488 return "text/turtle"; 489 case NONE: 490 return null; 491 default: 492 return null; 493 } 494 } 495 496 static public org.hl7.fhir.dstu3.model.TestScript.ContentType convertContentType(String src) throws FHIRException { 497 if (src == null) return null; 498 if (src.contains("xml")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.XML; 499 if (src.contains("json")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.JSON; 500 if (src.contains("tu")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.TTL; 501 return org.hl7.fhir.dstu3.model.TestScript.ContentType.NONE; 502 } 503 504 public static org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 505 if (src == null) return null; 506 org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent(); 507 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 508 if (src.hasField()) tgt.setFieldElement(String30_40.convertString(src.getFieldElement())); 509 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 510 return tgt; 511 } 512 513 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 514 if (src == null) return null; 515 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent(); 516 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 517 if (src.hasField()) tgt.setFieldElement(String30_40.convertString(src.getFieldElement())); 518 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 519 return tgt; 520 } 521 522 public static org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 523 if (src == null) return null; 524 org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent(); 525 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 526 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 527 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 528 if (src.hasDirection()) tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 529 if (src.hasCompareToSourceId()) 530 tgt.setCompareToSourceIdElement(String30_40.convertString(src.getCompareToSourceIdElement())); 531 if (src.hasCompareToSourceExpression()) 532 tgt.setCompareToSourceExpressionElement(String30_40.convertString(src.getCompareToSourceExpressionElement())); 533 if (src.hasCompareToSourcePath()) 534 tgt.setCompareToSourcePathElement(String30_40.convertString(src.getCompareToSourcePathElement())); 535 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 536 if (src.hasExpression()) tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 537 if (src.hasHeaderField()) tgt.setHeaderFieldElement(String30_40.convertString(src.getHeaderFieldElement())); 538 if (src.hasMinimumId()) tgt.setMinimumIdElement(String30_40.convertString(src.getMinimumIdElement())); 539 if (src.hasNavigationLinks()) 540 tgt.setNavigationLinksElement(Boolean30_40.convertBoolean(src.getNavigationLinksElement())); 541 if (src.hasOperator()) tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 542 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 543 if (src.hasRequestMethod()) 544 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 545 if (src.hasRequestURL()) tgt.setRequestURLElement(String30_40.convertString(src.getRequestURLElement())); 546 if (src.hasResource()) tgt.setResourceElement(Code30_40.convertCode(src.getResourceElement())); 547 if (src.hasResponse()) tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 548 if (src.hasResponseCode()) tgt.setResponseCodeElement(String30_40.convertString(src.getResponseCodeElement())); 549 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 550 if (src.hasValidateProfileId()) 551 tgt.setValidateProfileIdElement(Id30_40.convertId(src.getValidateProfileIdElement())); 552 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 553 if (src.hasWarningOnly()) tgt.setWarningOnlyElement(Boolean30_40.convertBoolean(src.getWarningOnlyElement())); 554 return tgt; 555 } 556 557 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 558 if (src == null) return null; 559 org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent(); 560 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 561 if (src.hasLabel()) tgt.setLabelElement(String30_40.convertString(src.getLabelElement())); 562 if (src.hasDescription()) tgt.setDescriptionElement(String30_40.convertString(src.getDescriptionElement())); 563 if (src.hasDirection()) tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 564 if (src.hasCompareToSourceId()) 565 tgt.setCompareToSourceIdElement(String30_40.convertString(src.getCompareToSourceIdElement())); 566 if (src.hasCompareToSourceExpression()) 567 tgt.setCompareToSourceExpressionElement(String30_40.convertString(src.getCompareToSourceExpressionElement())); 568 if (src.hasCompareToSourcePath()) 569 tgt.setCompareToSourcePathElement(String30_40.convertString(src.getCompareToSourcePathElement())); 570 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 571 if (src.hasExpression()) tgt.setExpressionElement(String30_40.convertString(src.getExpressionElement())); 572 if (src.hasHeaderField()) tgt.setHeaderFieldElement(String30_40.convertString(src.getHeaderFieldElement())); 573 if (src.hasMinimumId()) tgt.setMinimumIdElement(String30_40.convertString(src.getMinimumIdElement())); 574 if (src.hasNavigationLinks()) 575 tgt.setNavigationLinksElement(Boolean30_40.convertBoolean(src.getNavigationLinksElement())); 576 if (src.hasOperator()) tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 577 if (src.hasPath()) tgt.setPathElement(String30_40.convertString(src.getPathElement())); 578 if (src.hasRequestMethod()) 579 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 580 if (src.hasRequestURL()) tgt.setRequestURLElement(String30_40.convertString(src.getRequestURLElement())); 581 if (src.hasResource()) tgt.setResourceElement(Code30_40.convertCode(src.getResourceElement())); 582 if (src.hasResponse()) tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 583 if (src.hasResponseCode()) tgt.setResponseCodeElement(String30_40.convertString(src.getResponseCodeElement())); 584 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_40.convertId(src.getSourceIdElement())); 585 if (src.hasValidateProfileId()) 586 tgt.setValidateProfileIdElement(Id30_40.convertId(src.getValidateProfileIdElement())); 587 if (src.hasValue()) tgt.setValueElement(String30_40.convertString(src.getValueElement())); 588 if (src.hasWarningOnly()) tgt.setWarningOnlyElement(Boolean30_40.convertBoolean(src.getWarningOnlyElement())); 589 return tgt; 590 } 591 592 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> src) throws FHIRException { 593 if (src == null || src.isEmpty()) return null; 594 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionDirectionTypeEnumFactory()); 595 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 596 if (src.getValue() == null) { 597 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.NULL); 598 } else { 599 switch (src.getValue()) { 600 case RESPONSE: 601 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.RESPONSE); 602 break; 603 case REQUEST: 604 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.REQUEST); 605 break; 606 default: 607 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.NULL); 608 break; 609 } 610 } 611 return tgt; 612 } 613 614 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> src) throws FHIRException { 615 if (src == null || src.isEmpty()) return null; 616 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionTypeEnumFactory()); 617 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 618 if (src.getValue() == null) { 619 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.NULL); 620 } else { 621 switch (src.getValue()) { 622 case RESPONSE: 623 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.RESPONSE); 624 break; 625 case REQUEST: 626 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.REQUEST); 627 break; 628 default: 629 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.NULL); 630 break; 631 } 632 } 633 return tgt; 634 } 635 636 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> src) throws FHIRException { 637 if (src == null || src.isEmpty()) return null; 638 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionOperatorTypeEnumFactory()); 639 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 640 if (src.getValue() == null) { 641 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NULL); 642 } else { 643 switch (src.getValue()) { 644 case EQUALS: 645 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.EQUALS); 646 break; 647 case NOTEQUALS: 648 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTEQUALS); 649 break; 650 case IN: 651 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.IN); 652 break; 653 case NOTIN: 654 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTIN); 655 break; 656 case GREATERTHAN: 657 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.GREATERTHAN); 658 break; 659 case LESSTHAN: 660 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.LESSTHAN); 661 break; 662 case EMPTY: 663 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.EMPTY); 664 break; 665 case NOTEMPTY: 666 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTEMPTY); 667 break; 668 case CONTAINS: 669 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.CONTAINS); 670 break; 671 case NOTCONTAINS: 672 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTCONTAINS); 673 break; 674 case EVAL: 675 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.EVAL); 676 break; 677 default: 678 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NULL); 679 break; 680 } 681 } 682 return tgt; 683 } 684 685 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> src) throws FHIRException { 686 if (src == null || src.isEmpty()) return null; 687 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorTypeEnumFactory()); 688 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 689 if (src.getValue() == null) { 690 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NULL); 691 } else { 692 switch (src.getValue()) { 693 case EQUALS: 694 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EQUALS); 695 break; 696 case NOTEQUALS: 697 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEQUALS); 698 break; 699 case IN: 700 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.IN); 701 break; 702 case NOTIN: 703 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTIN); 704 break; 705 case GREATERTHAN: 706 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.GREATERTHAN); 707 break; 708 case LESSTHAN: 709 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.LESSTHAN); 710 break; 711 case EMPTY: 712 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EMPTY); 713 break; 714 case NOTEMPTY: 715 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEMPTY); 716 break; 717 case CONTAINS: 718 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.CONTAINS); 719 break; 720 case NOTCONTAINS: 721 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTCONTAINS); 722 break; 723 case EVAL: 724 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EVAL); 725 break; 726 default: 727 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NULL); 728 break; 729 } 730 } 731 return tgt; 732 } 733 734 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 735 if (src == null || src.isEmpty()) return null; 736 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCodeEnumFactory()); 737 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 738 if (src.getValue() == null) { 739 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.NULL); 740 } else { 741 switch (src.getValue()) { 742 case DELETE: 743 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.DELETE); 744 break; 745 case GET: 746 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.GET); 747 break; 748 case OPTIONS: 749 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.OPTIONS); 750 break; 751 case PATCH: 752 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.PATCH); 753 break; 754 case POST: 755 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.POST); 756 break; 757 case PUT: 758 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.PUT); 759 break; 760 default: 761 tgt.setValue(org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode.NULL); 762 break; 763 } 764 } 765 return tgt; 766 } 767 768 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 769 if (src == null || src.isEmpty()) return null; 770 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCodeEnumFactory()); 771 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 772 if (src.getValue() == null) { 773 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.NULL); 774 } else { 775 switch (src.getValue()) { 776 case DELETE: 777 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.DELETE); 778 break; 779 case GET: 780 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.GET); 781 break; 782 case OPTIONS: 783 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.OPTIONS); 784 break; 785 case PATCH: 786 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.PATCH); 787 break; 788 case POST: 789 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.POST); 790 break; 791 case PUT: 792 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.PUT); 793 break; 794 default: 795 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.NULL); 796 break; 797 } 798 } 799 return tgt; 800 } 801 802 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 803 if (src == null || src.isEmpty()) return null; 804 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionResponseTypesEnumFactory()); 805 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 806 if (src.getValue() == null) { 807 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NULL); 808 } else { 809 switch (src.getValue()) { 810 case OKAY: 811 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.OKAY); 812 break; 813 case CREATED: 814 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.CREATED); 815 break; 816 case NOCONTENT: 817 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOCONTENT); 818 break; 819 case NOTMODIFIED: 820 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 821 break; 822 case BAD: 823 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.BAD); 824 break; 825 case FORBIDDEN: 826 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.FORBIDDEN); 827 break; 828 case NOTFOUND: 829 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOTFOUND); 830 break; 831 case METHODNOTALLOWED: 832 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 833 break; 834 case CONFLICT: 835 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.CONFLICT); 836 break; 837 case GONE: 838 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.GONE); 839 break; 840 case PRECONDITIONFAILED: 841 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 842 break; 843 case UNPROCESSABLE: 844 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 845 break; 846 default: 847 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NULL); 848 break; 849 } 850 } 851 return tgt; 852 } 853 854 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 855 if (src == null || src.isEmpty()) return null; 856 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypesEnumFactory()); 857 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 858 if (src.getValue() == null) { 859 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NULL); 860 } else { 861 switch (src.getValue()) { 862 case OKAY: 863 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.OKAY); 864 break; 865 case CREATED: 866 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CREATED); 867 break; 868 case NOCONTENT: 869 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOCONTENT); 870 break; 871 case NOTMODIFIED: 872 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 873 break; 874 case BAD: 875 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.BAD); 876 break; 877 case FORBIDDEN: 878 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.FORBIDDEN); 879 break; 880 case NOTFOUND: 881 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTFOUND); 882 break; 883 case METHODNOTALLOWED: 884 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 885 break; 886 case CONFLICT: 887 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CONFLICT); 888 break; 889 case GONE: 890 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.GONE); 891 break; 892 case PRECONDITIONFAILED: 893 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 894 break; 895 case UNPROCESSABLE: 896 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 897 break; 898 default: 899 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NULL); 900 break; 901 } 902 } 903 return tgt; 904 } 905 906 public static org.hl7.fhir.r4.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu3.model.TestScript.TestActionComponent src) throws FHIRException { 907 if (src == null) return null; 908 org.hl7.fhir.r4.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestActionComponent(); 909 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 910 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 911 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 912 return tgt; 913 } 914 915 public static org.hl7.fhir.dstu3.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.r4.model.TestScript.TestActionComponent src) throws FHIRException { 916 if (src == null) return null; 917 org.hl7.fhir.dstu3.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestActionComponent(); 918 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 919 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 920 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 921 return tgt; 922 } 923 924 public static org.hl7.fhir.r4.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent src) throws FHIRException { 925 if (src == null) return null; 926 org.hl7.fhir.r4.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.TeardownActionComponent(); 927 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 928 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 929 return tgt; 930 } 931 932 public static org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r4.model.TestScript.TeardownActionComponent src) throws FHIRException { 933 if (src == null) return null; 934 org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent(); 935 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 936 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 937 return tgt; 938 } 939}